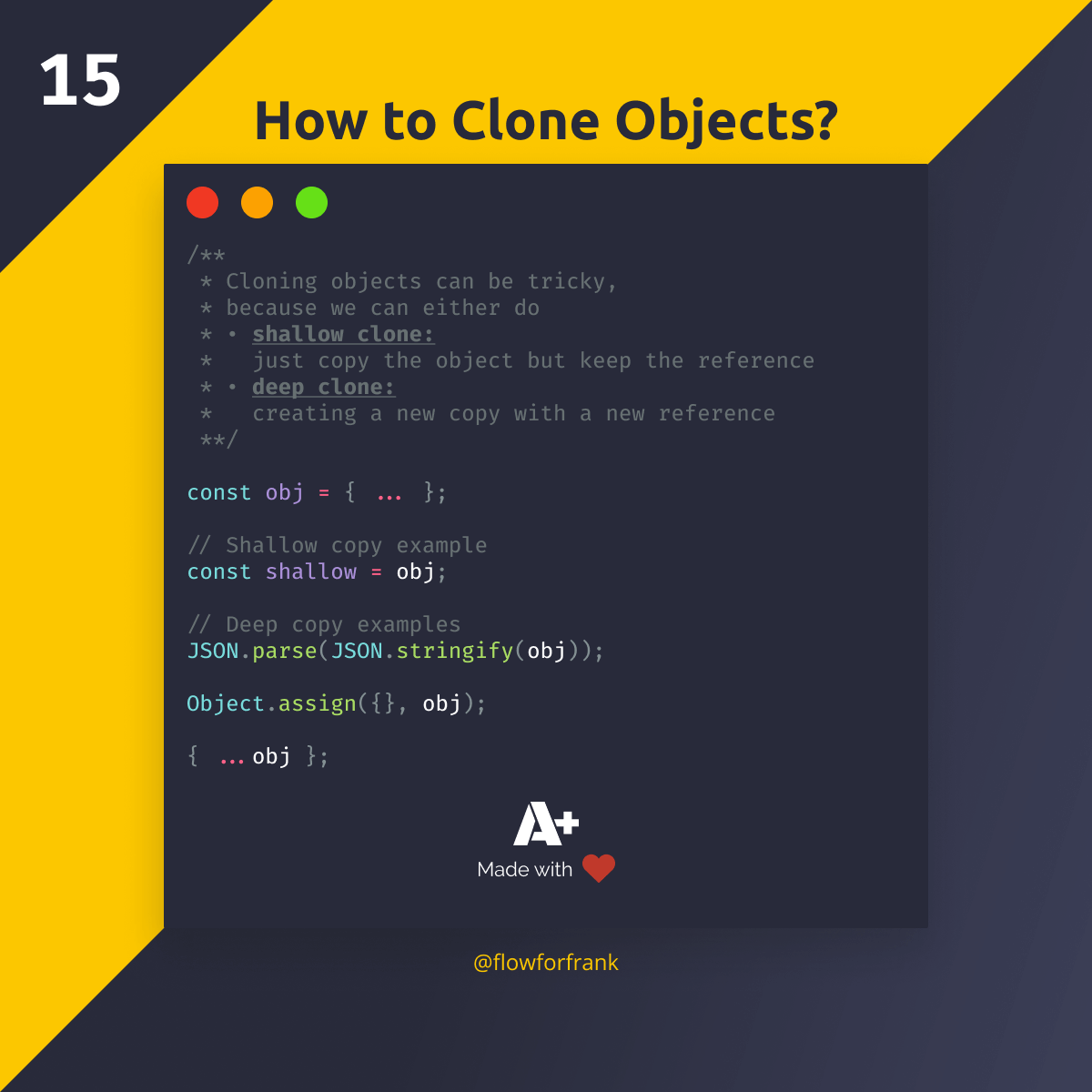
3 Simple Examples to Copy Objects in JavaScript
Copying objects in JavaScript can be tricky because we can either do a shallow copy (just copy the object but keep the reference) or a deep copy. (creating a new copy with a new reference)
To copy an object in JavaScript, you have three options:
- Use the spread operator
- Use
JSON.stringify
andJSON.parse
- Use
Object.assign
with an empty object
All of the above methods can be used to perform a deep copy. To shallow copy an object, and keep the reference, you can do a simple assignment:
const obj = { ... };
// Shallow copy can be done by using assignment:
const shallow = obj;
To deep copy an object and create a new reference for it, you can use the following options:
// Using JSON.parse & JSON.stringify
JSON.parse(JSON.stringify(obj));
Object.assign({}, obj);
// Using Object.assign
const shallow = Object.assign({}, obj);
// Using object spread
const deep = { ...obj };
Note that you can also perform a shallow copy with Object.assign
if you pass an existing object as the first parameter:
const obj = { ... };
const shallow = Object.assign(obj, obj2);
The difference between shallow and deep copy
Shallow copy means you copy the object and keep the reference, while deep copy means you copy the object with a new reference.
Why is this important for us? Image the following situation. You have the internal state of your application stored in an object that is reflected in your user interface. You pass the state to a child component, where you modify the format of one of the properties.
// The root state contains a timestamp
const state = {
timestamp: 1655718139739
}
// In your child component you want to display a formatted date
const passedState = state
// This will also modify the original object
passedState.timestamp = format(state.timestamp)
This will not only modify the format inside the child component but everywhere else since the two objects share the same reference. When you modify passedState
, you also modify state
.
This is why you usually want to go with a deep copy, where you create a new reference while copying the object. To fix the above error, we can use the above-mentioned solutions:
// The root state contains a timestamp
const state = {
timestamp: 1655718139739
}
// This time, we performed a deep copy
const passedState = { ...state }
// This will NOT modify the original object
passedState.timestamp = format(state.timestamp)
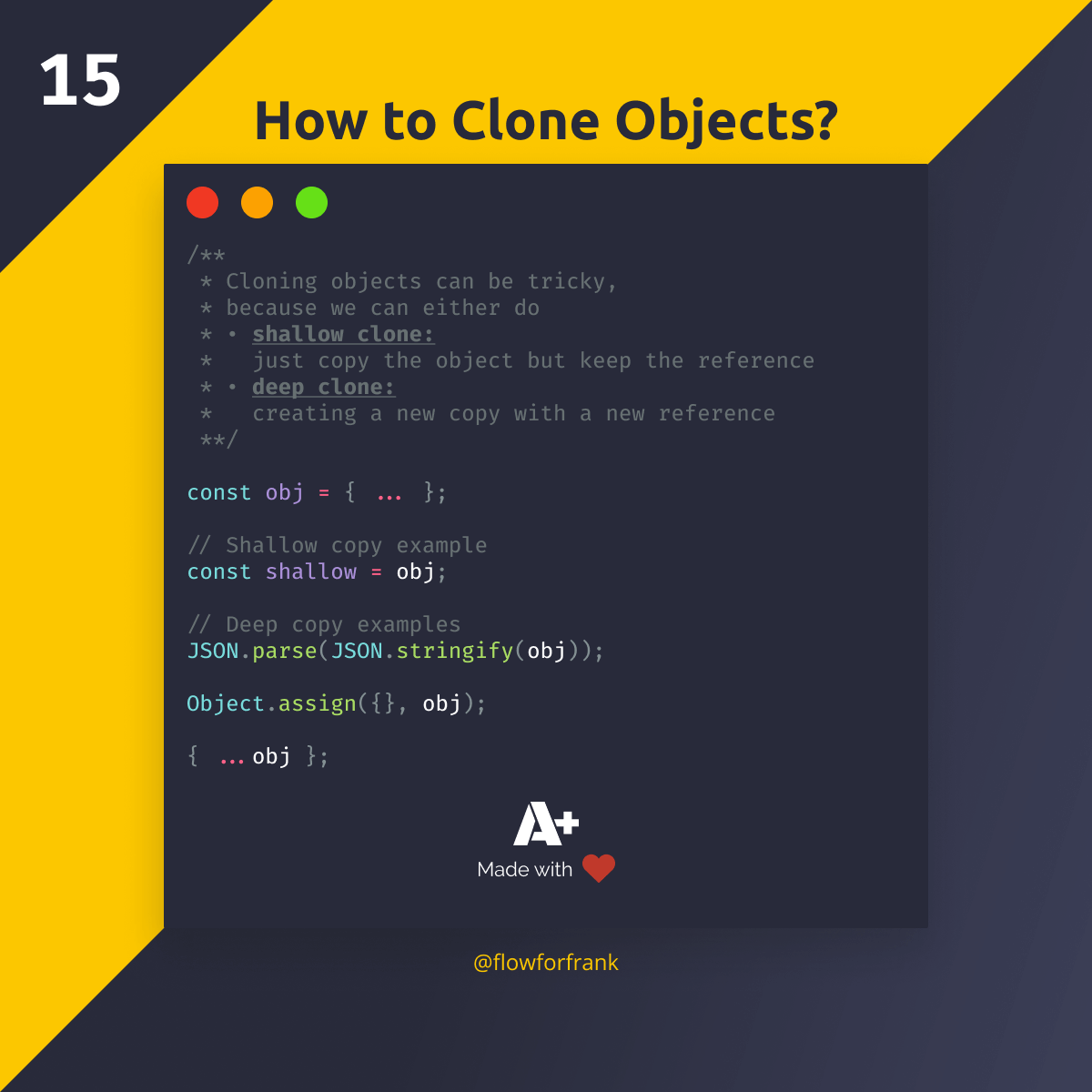
Resources:
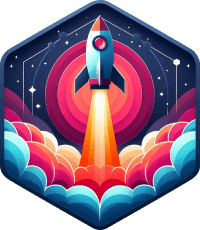
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: