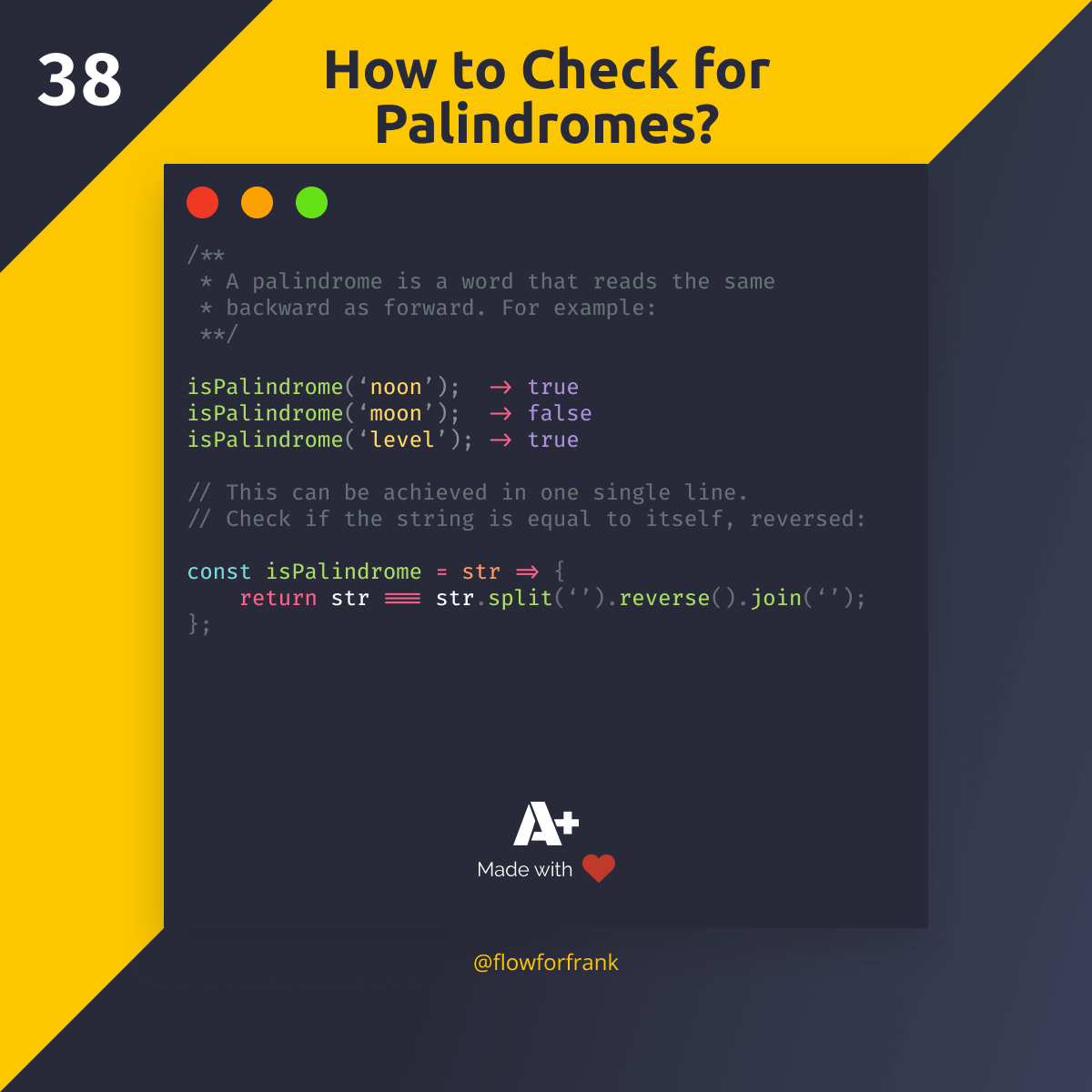
How to Check for Palindromes in JavaScript
A palindrome is a sequence of characters that reads the same backwards as forward, such as "noon" or "level".
To check if a word is a palindrome, we can create a function for it, the implementation can vary, but probably the shortest solution is using a combination of split
, reverse
and join
:
const isPalindrome = str => str === str.split('').reverse().join('');
isPalindrome('noon'); -> true
isPalindrome('moon'); -> false
isPalindrome('level'); -> true
It works by checking if the passed string is equal to itself in reverse order. This is achieved by first splitting the string into letter which gives us an array, this array is reversed, then joined back together. In terms of performance, the best way is probably using a for
loop:
const isPalindrome = str => {
for (let i = 0; i < str.length / 2; i++) {
if (str[i] !== str[str.length - 1 - i]) {
return false;
}
}
return true;
};
This works by looping through the string, starting from the beginning, and checking if the character at position i
, is equal to the same position, but starting from the end. If it's not, we can simply break from the loop by returning false, otherwise, we are dealing with a palindrome.
Also note that you only need to loop till the half of the string, otherwise every character would be checked twice. This is why we go till str.length / 2
in the for
loop.
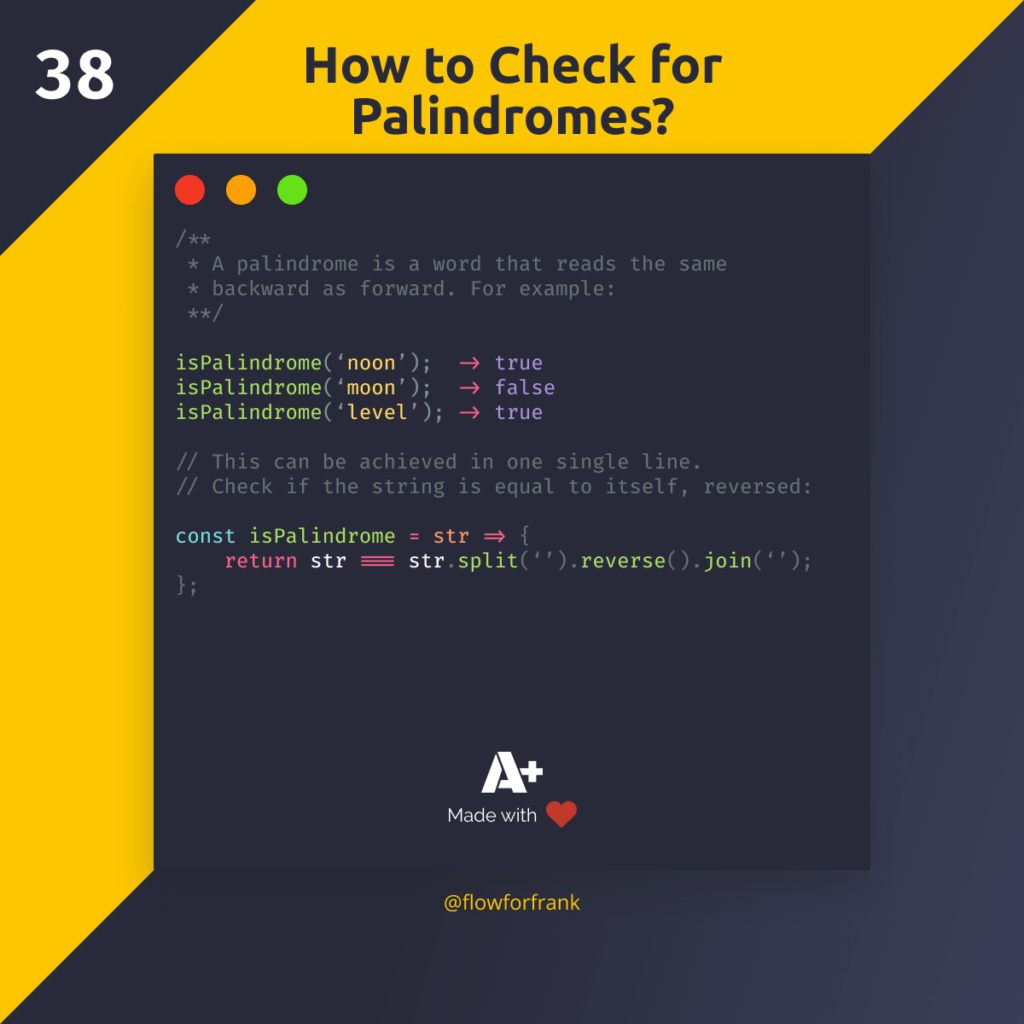
Resources:
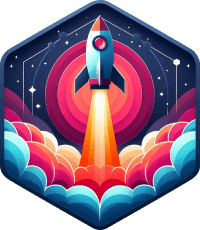
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: