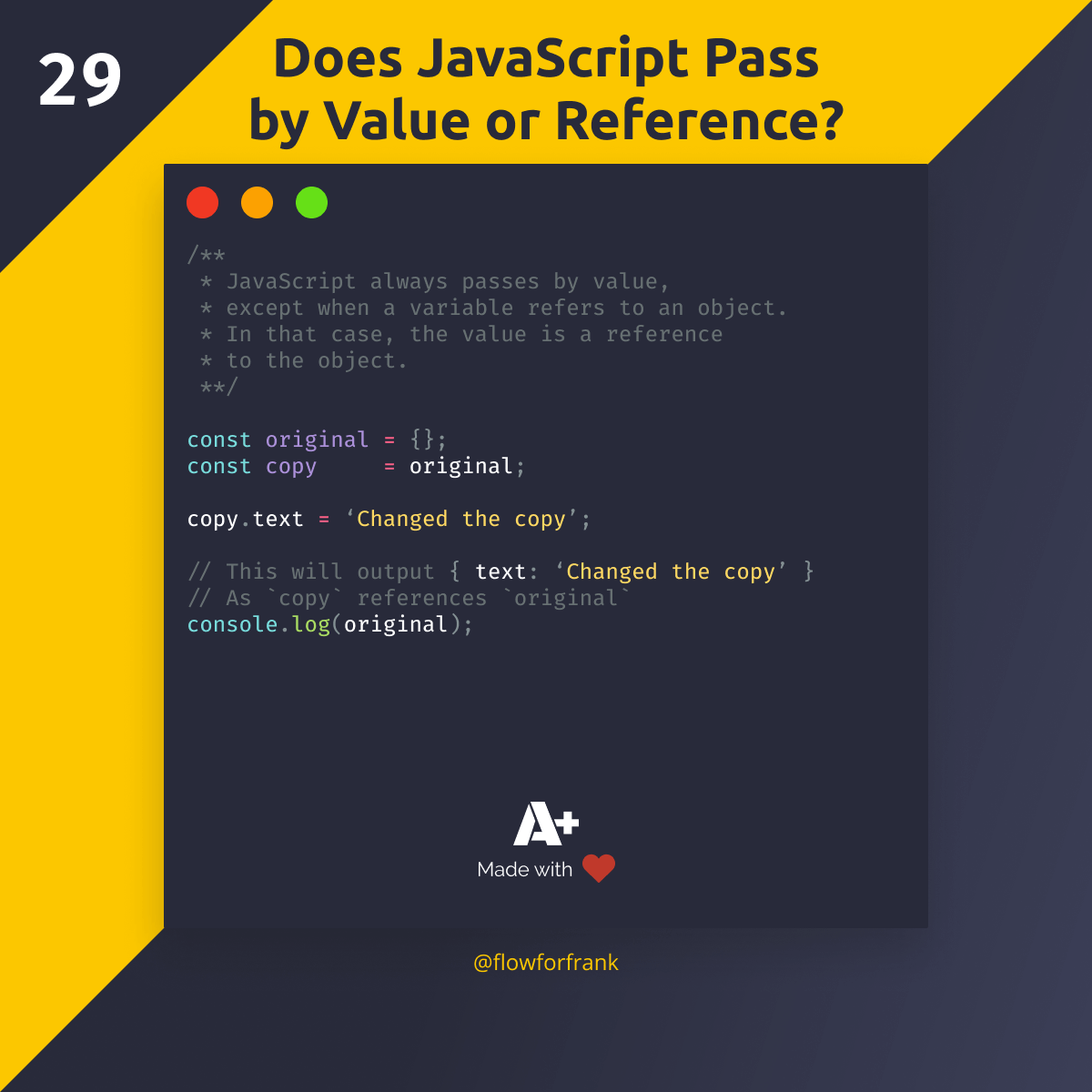
Does JavaScript Pass by Value or Reference?
JavaScript always passes by value, except when a variable refers to an object. In that case, the value is a reference to the object.
const original = {};
const copy = original;
copy.text = 'Changed the copy';
// This will output { text: βChanged the copyβ }
// As `copy` references `original`
console.log(original);
You can try it out with the example above. Even though you've changed copy
, as the reference is pointing to original
, it will be changed too. In order to do deep copy an object β meaning you also create a new reference to detach from the original β you can use one of the examples below:
// Using JSON.parse & JSON.stringify
JSON.parse(JSON.stringify(obj));
// Using Object.assign
Object.assign({}, obj);
// Using object spread
{ ...obj };
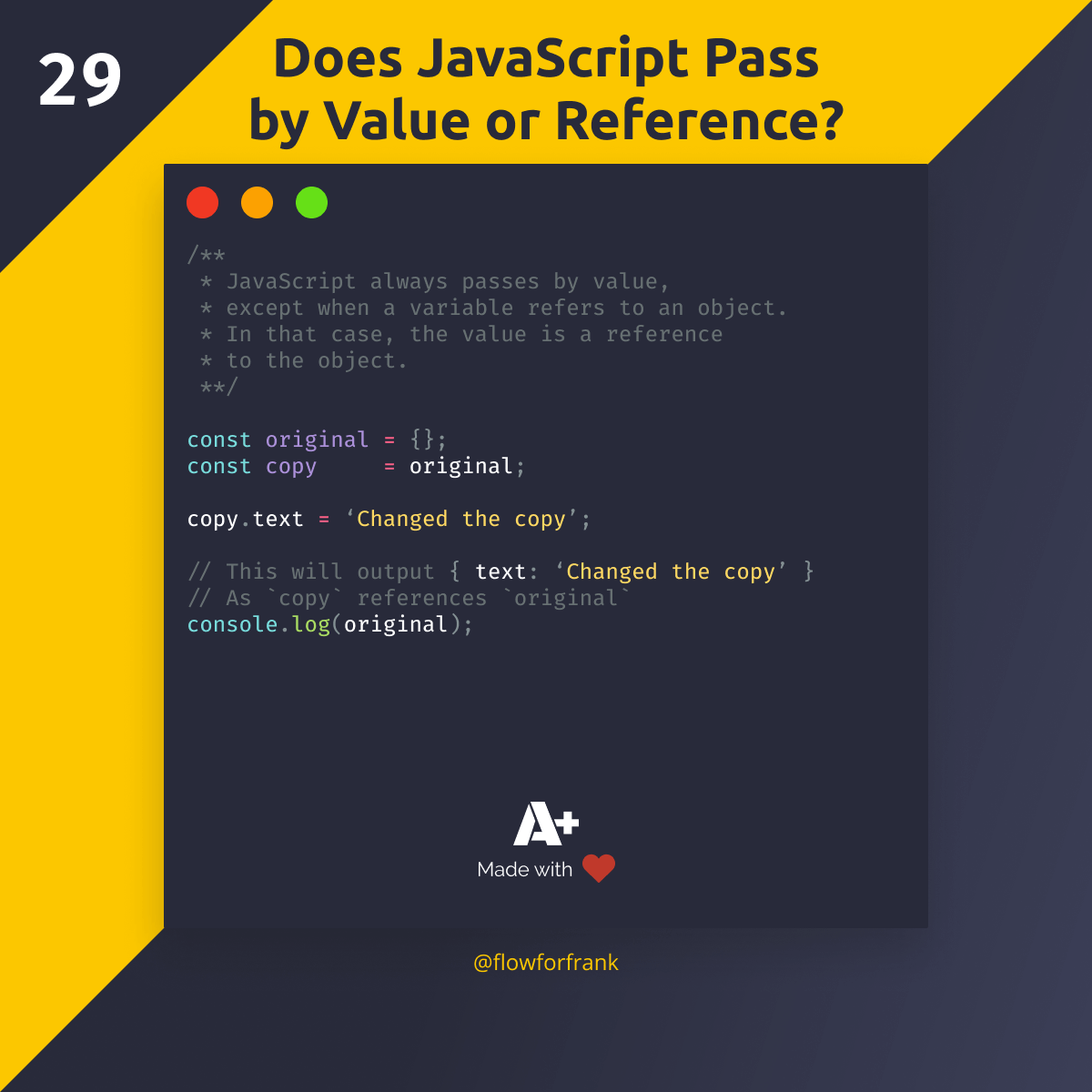
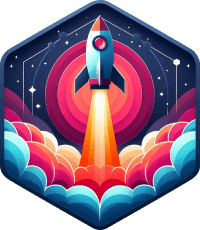
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: