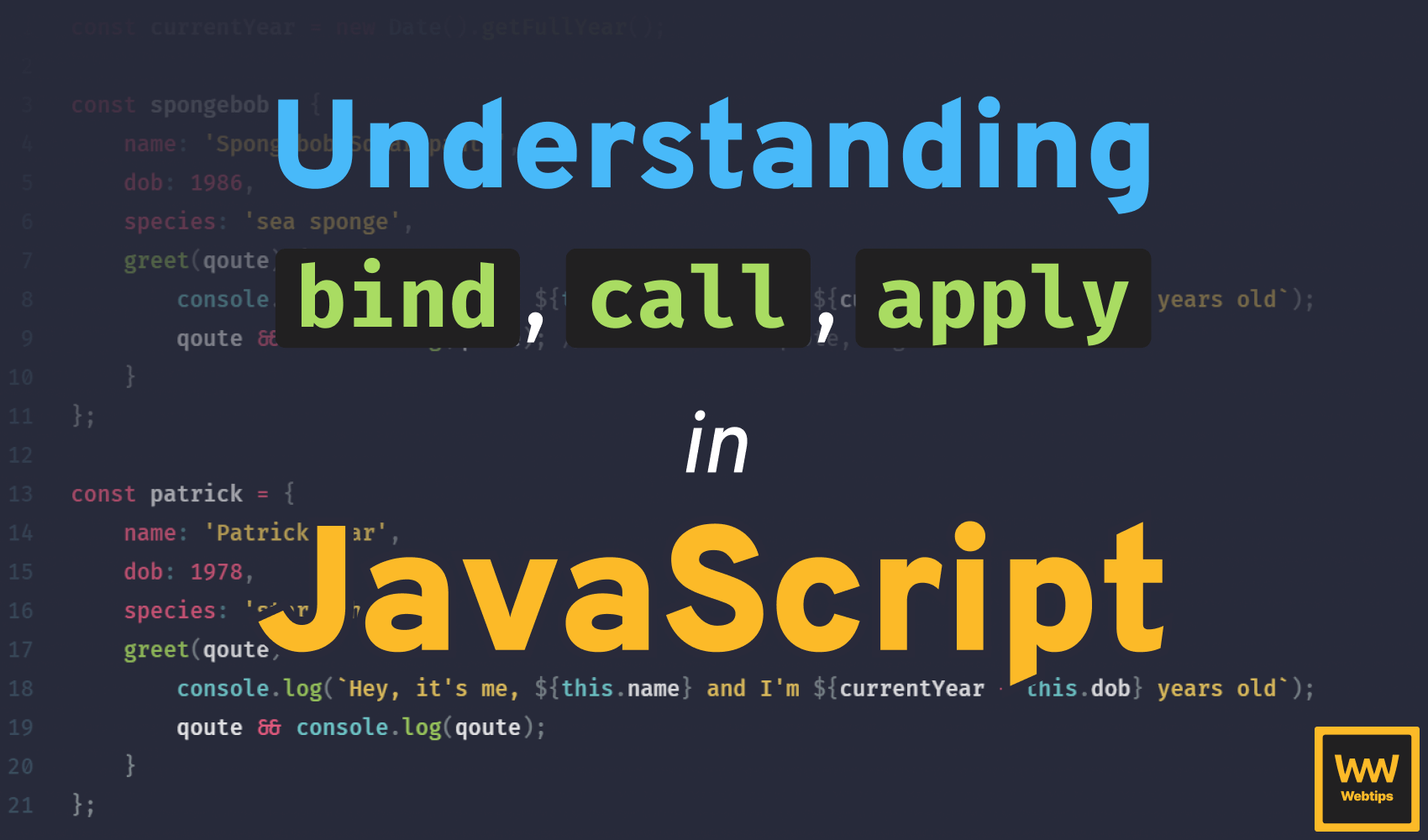
Understanding Bind, Call and Apply in JavaScript
Just as applying cold water to a burned area in the real world, we can also apply additional information to our function calls in the digital world through various function methods. In this tutorial, we'll take an in-depth look at what apply
, call
, and bind
are in JavaScript and how they work.
This tutorial heavily uses the this
keyword in JavaScript. If you're unfamiliar with how the this
keyword works, check out the tutorial below first.
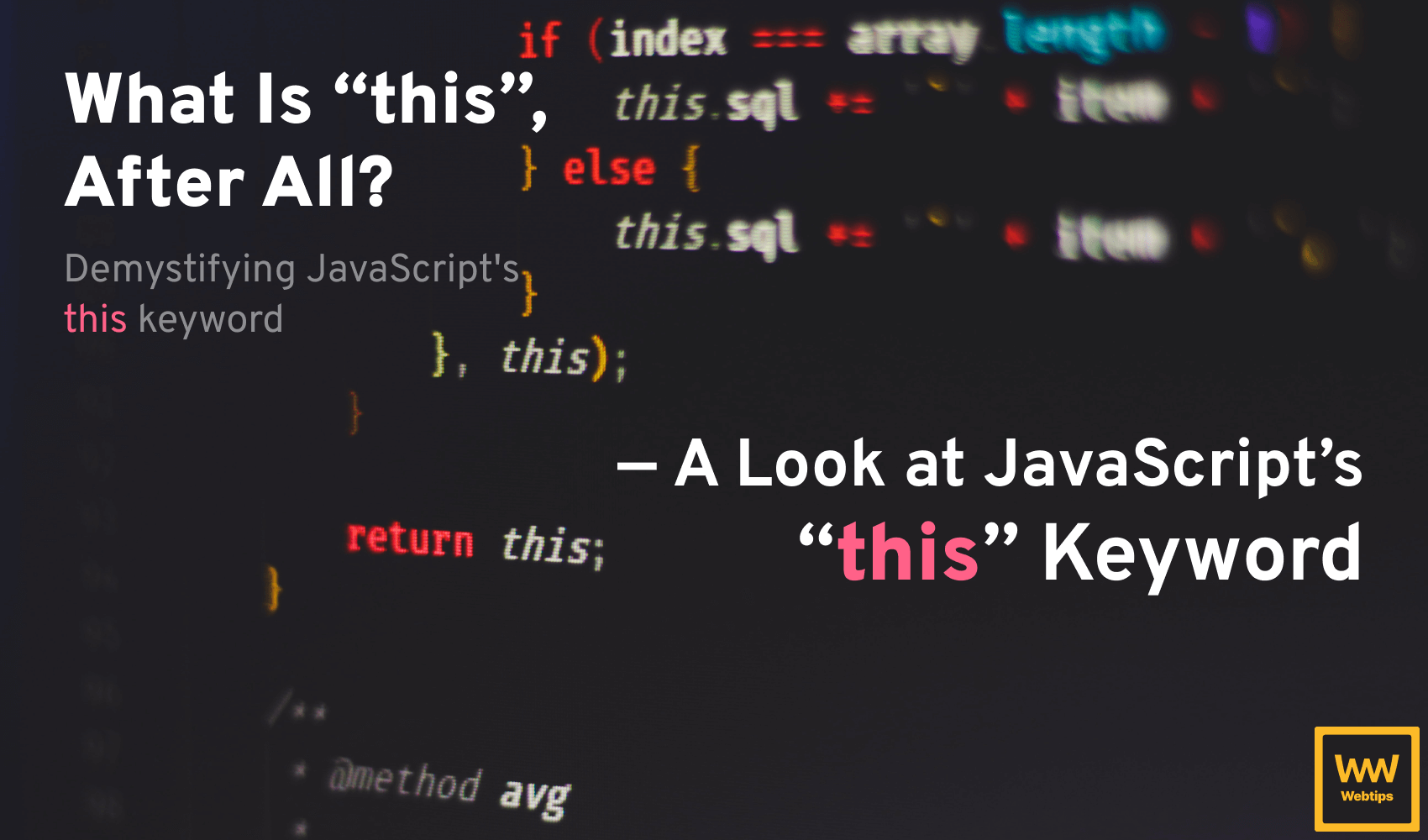
Letβs go in order, and start with bind. But first, we need some code to demonstrate all three of them, so we'll use the following two objects as examples:
const currentYear = new Date().getFullYear()
const spongebob = {
name: 'Spongebob Squarepants',
dob: 1986,
species: 'sea sponge',
greet(quote) {
console.log(`Hey, it's me, ${this.name} and I'm ${currentYear - this.dob} years old`)
quote && console.log(quote) // If we have a quote, log it out
}
}
const patrick = {
name: 'Patrick Star',
dob: 1978,
species: 'starfish',
greet(quote) {
console.log(`Hey, it's me, ${this.name} and I'm ${currentYear - this.dob} years old`)
quote && console.log(quote)
}
}
To demonstrate the functionality of bind
, call
, and apply
, we'll see how we can modify the context (the properties) of the above objects, such as their name
, dob
, or species
properties. We also have a greet
function, so that we can demonstrate how to modify the context of object methods too.
Bind
bind
is used in JavaScript to bind certain contexts to a function. When we have a function called lofi
and we call it like lofi.bind(chill)
, we are creating a new function where the context of this
is set to the value of chill
. Keep in mind that this does not modify the original function nor will it call:
index.js
data.js
The above code example demonstrates that bind
does not change the actual function but creates a brand-new one. On line 4, we use the bind
method to create a new context. However, when calling the greet
function on line 5, we still get the original context.
To use the modified context, we need to assign the bind
call to a variable. This is what we did on line 8. When we call greetPatrick()
the second time, we get back Patrickβs details because of the bound context, even though we are calling spongbob.greet
.
Call
Unlike bind
, call
will call the function immediately with the specified context. Letβs take a look at a couple of examples to better understand how call
differs from bind
. Take a look at the following code:
index.js
data.js
- Line 4: We call the
greet
method with thecall
function method, passing inpatrick
as a parameter (for the context). This will immediately execute the function β unlikebind
β creating aconsole.log
. - Line 7: When assigning the
call
method to a variable, we'll also get another line logged to the console as the function is executed again, regardless of the variable assignment. - Line 10: If we log the
greetPatrick
variable to the console, it'll returnundefined
. This is because thegreet
method doesn't return anything. If we don't have an explicit return statement in a function, for example,return true
,undefined
is returned implicitly. - Line 13: When we pass more than one parameter to
call
, the rest will be forwarded to the function. Notice that thegreet
method accepts aquote
parameter. The second string will be passed as the quote. This line is essentially equivalent to the following:
spongebob.greet('One eternity later')

Apply
The apply
function method is similar to call
. The only difference between the two is that call
accepts a list of arguments, while apply
accepts an array of arguments:
patrick.greet.apply(patrick, ['One eternity later']);
Note the difference between call
and apply
. One is called with an array while the other is not. There's no difference between their functionality; the only difference is the way they accept arguments. If we were to have multiple arguments they would look like the following:
// Accepts list of arguments
spongebob.greet.call(spongebob, '2000 years later', 'One eternity later');
// Accepts array of arguments
patrick.greet.apply(patrick, ['2000 years later', 'One eternity later']);
Conclusion
Letβs recap everything and draw a conclusion.
- Use
bind
when you want to bind a context to a function you want to call later. - Use
call
orapply
if you want to invoke the function immediately.
And the greatest question when it comes to call
and apply
: which one should you use? It really depends on your personal choice. However, if we look at which one performs better, call
executes faster most of the time:
Is there anything else you'd like to know about bind
, call
, and apply
in JavaScript? Let us know in the comments below! The use of the this
keyword heavily ties into this topic. If you're unfamiliar with how this works, be sure to check out the following tutorial:
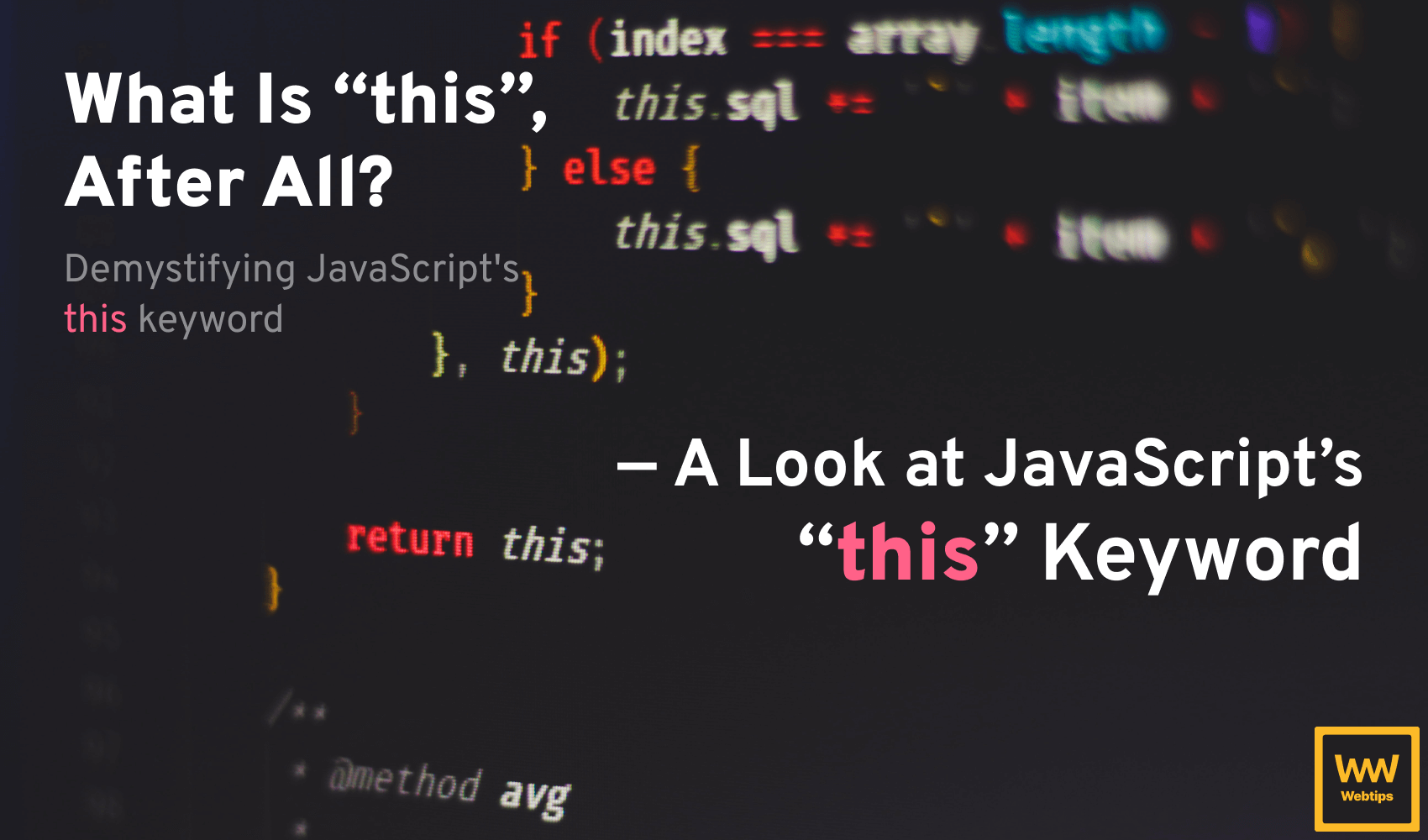
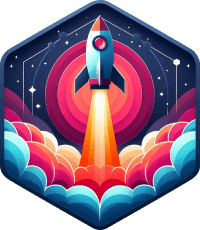
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: