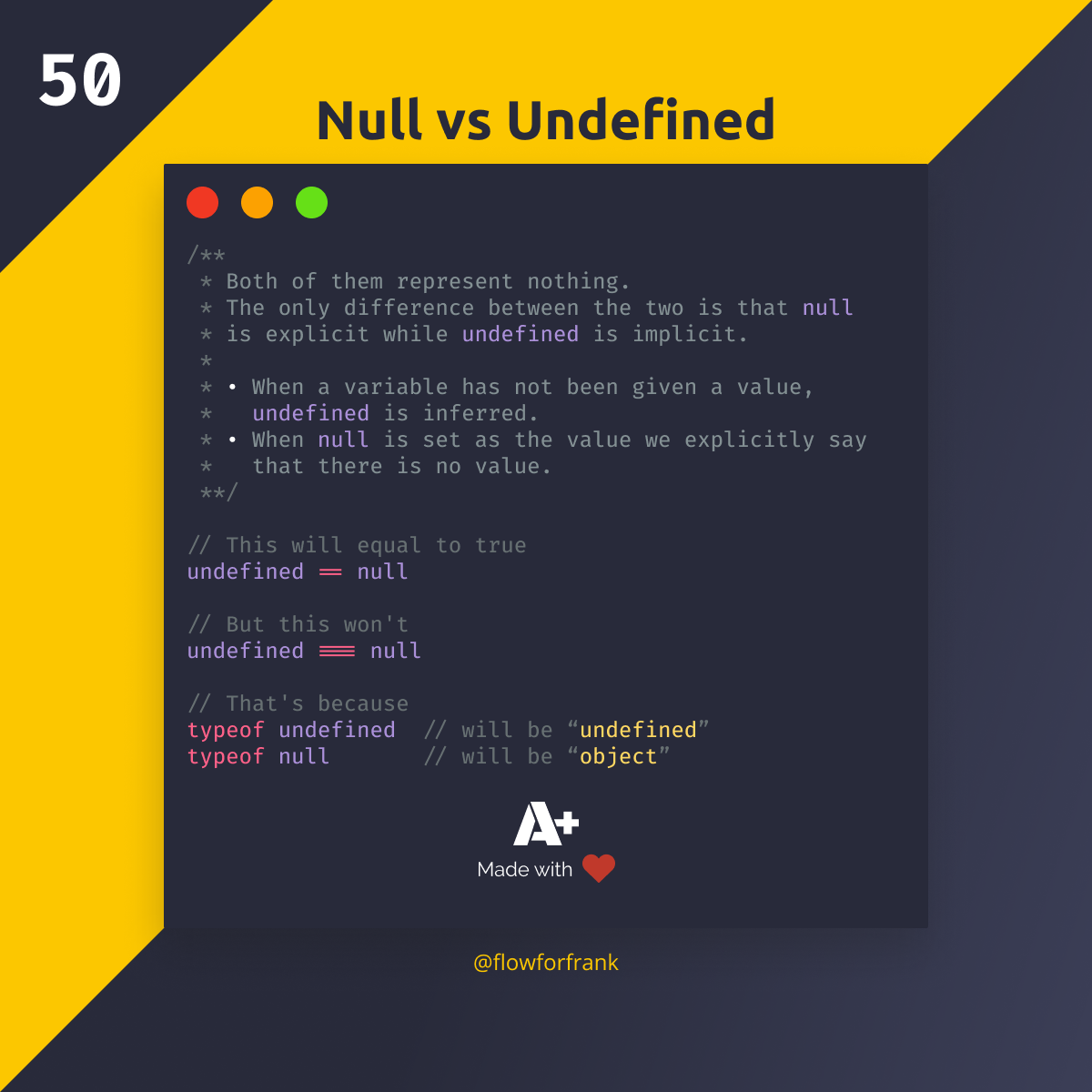
JS Tips, Tricks, and Snippets
Get useful code snippets into your codebase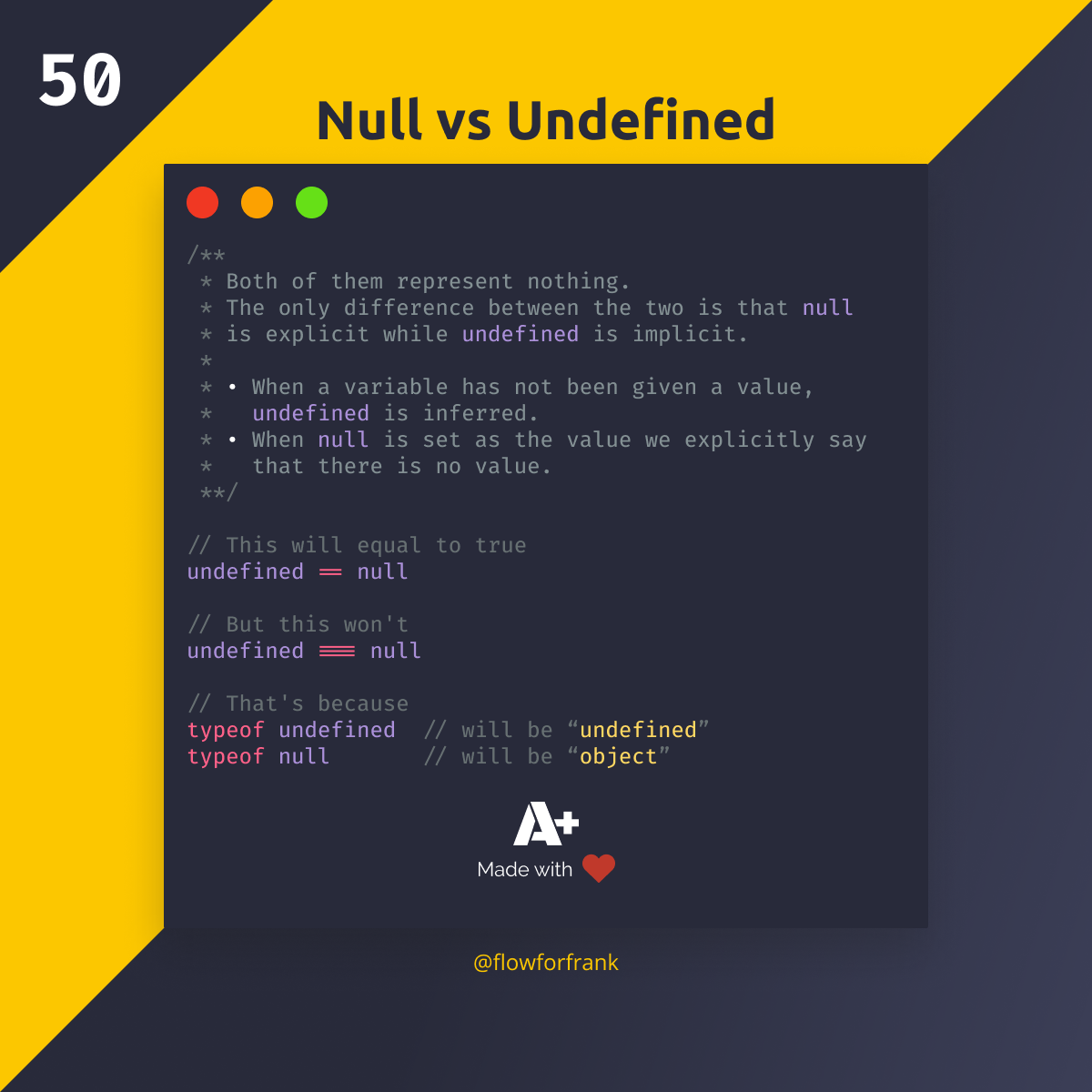
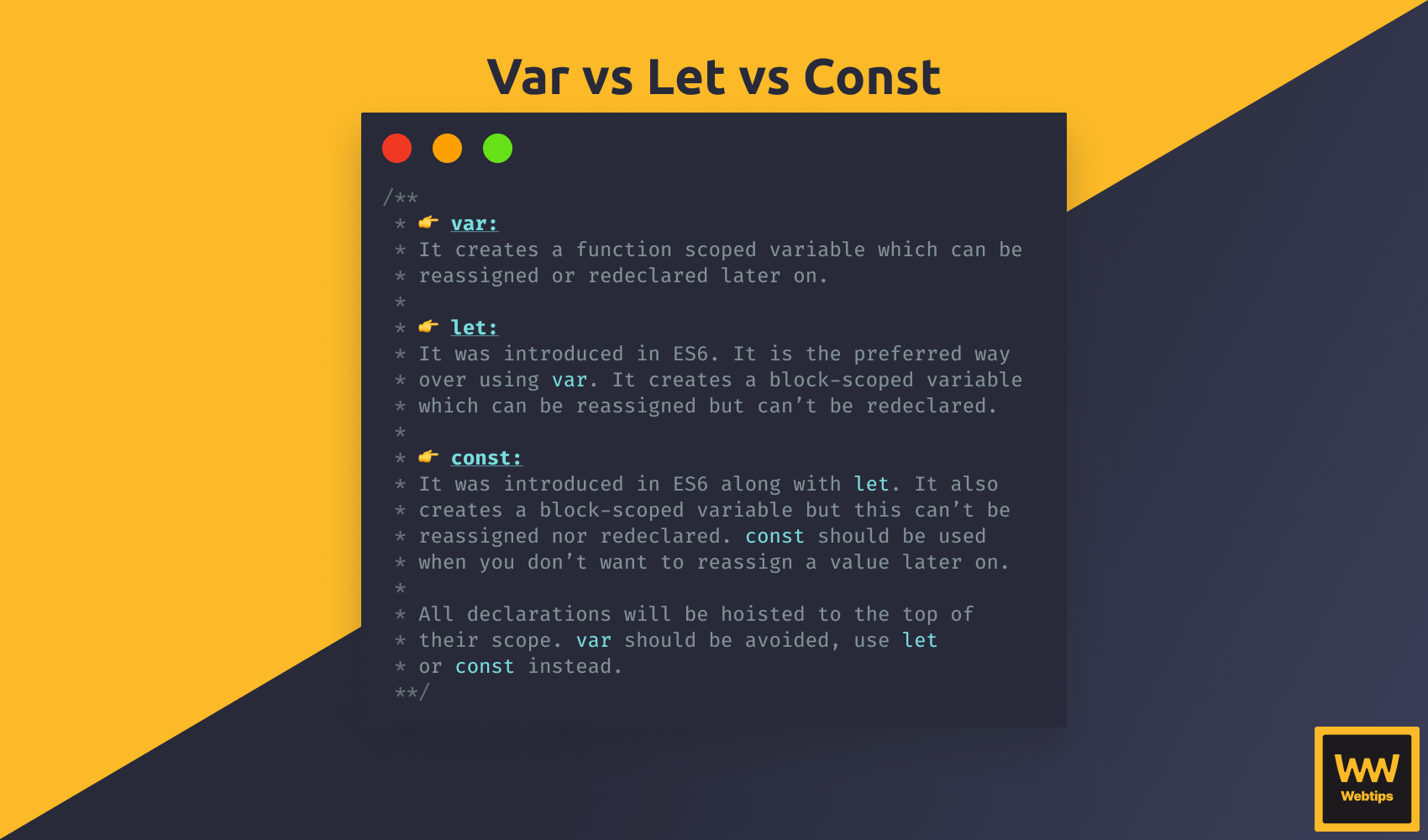
What are the Differences Between var, let, and const?
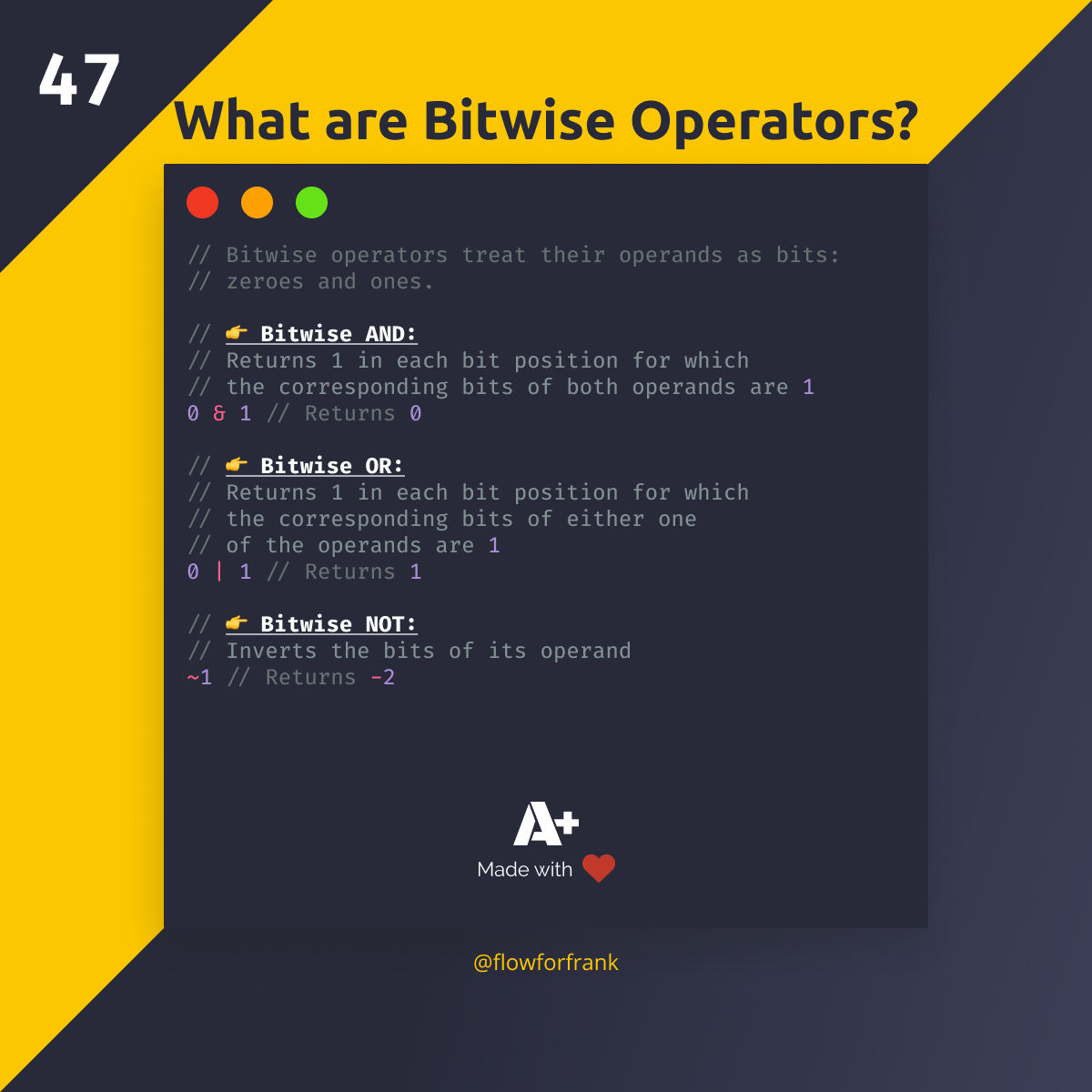
What are Bitwise Operators?
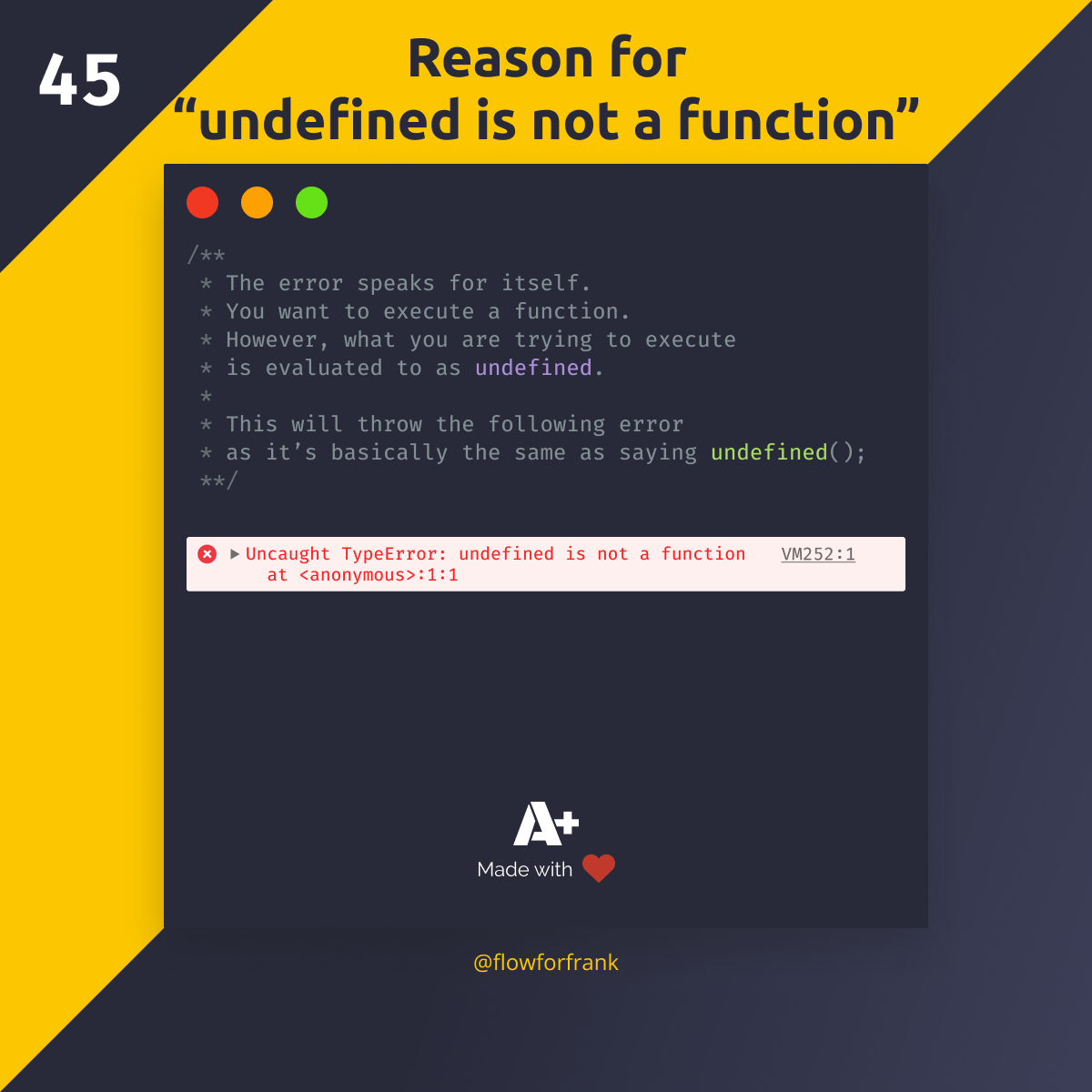
Why Do We Get "undefined is not a function" in JavaScript?
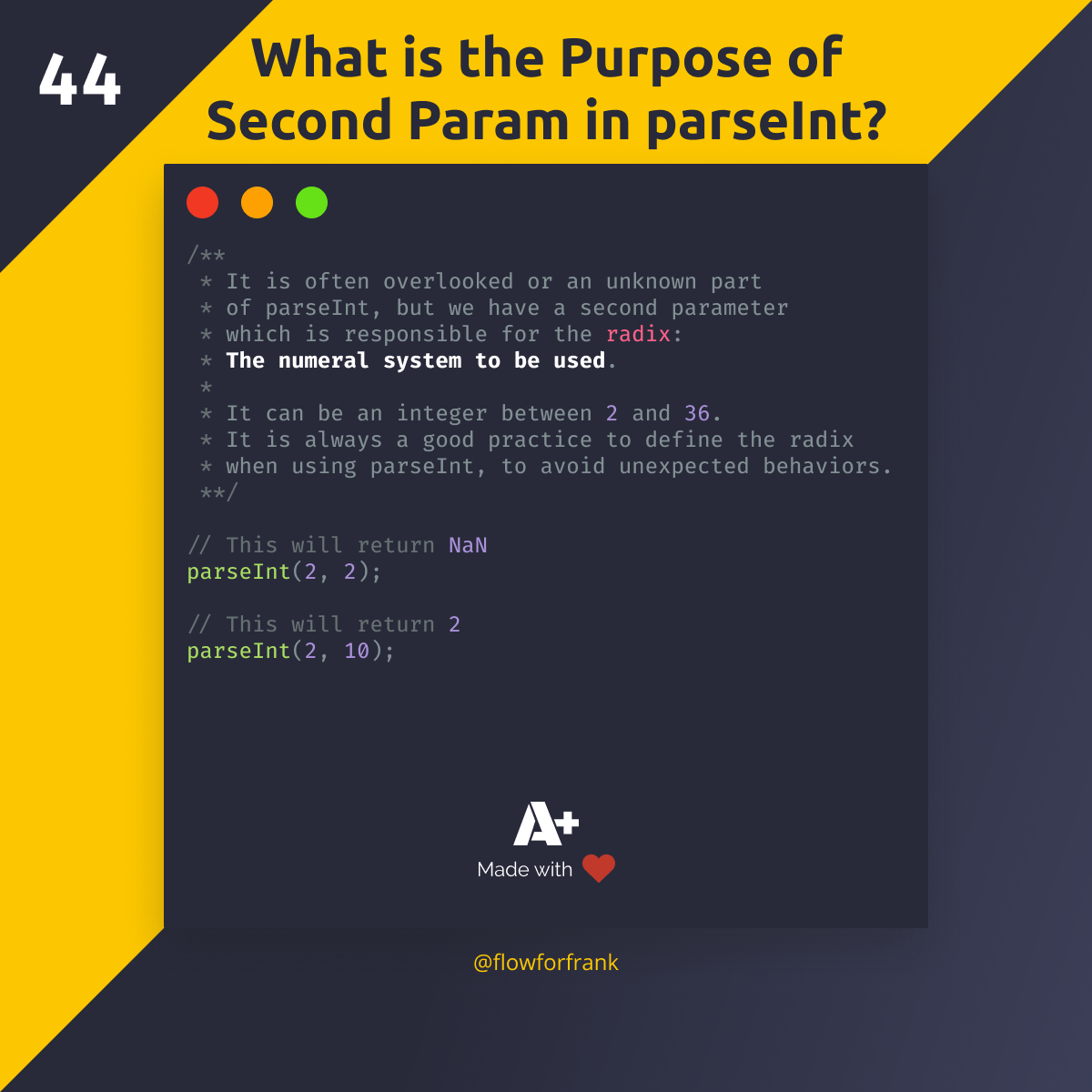
What is the Purpose of the Second Param in parseInt?
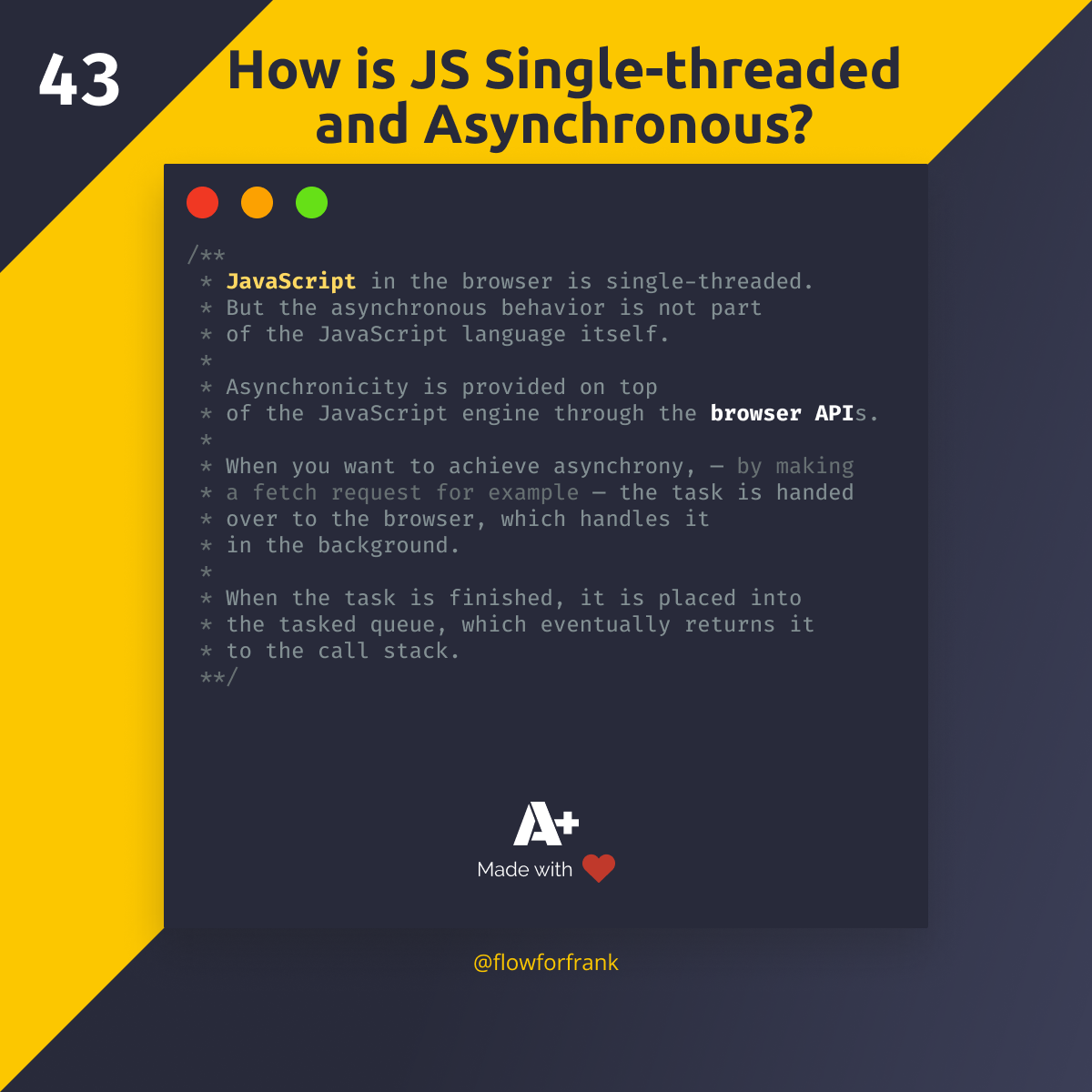
How is JavaScript single-threaded and asynchronous?
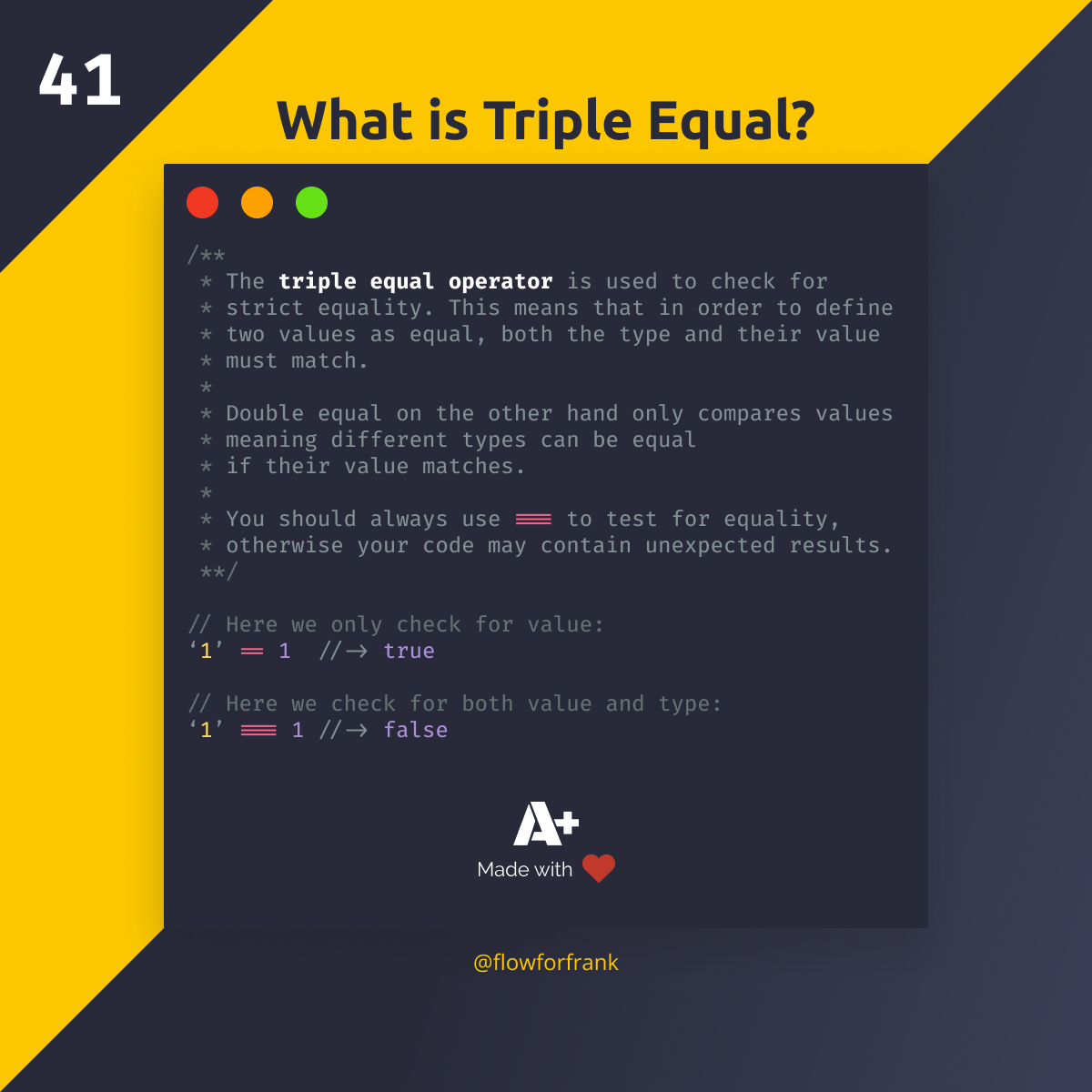
What is Triple Equal in JavaScript?
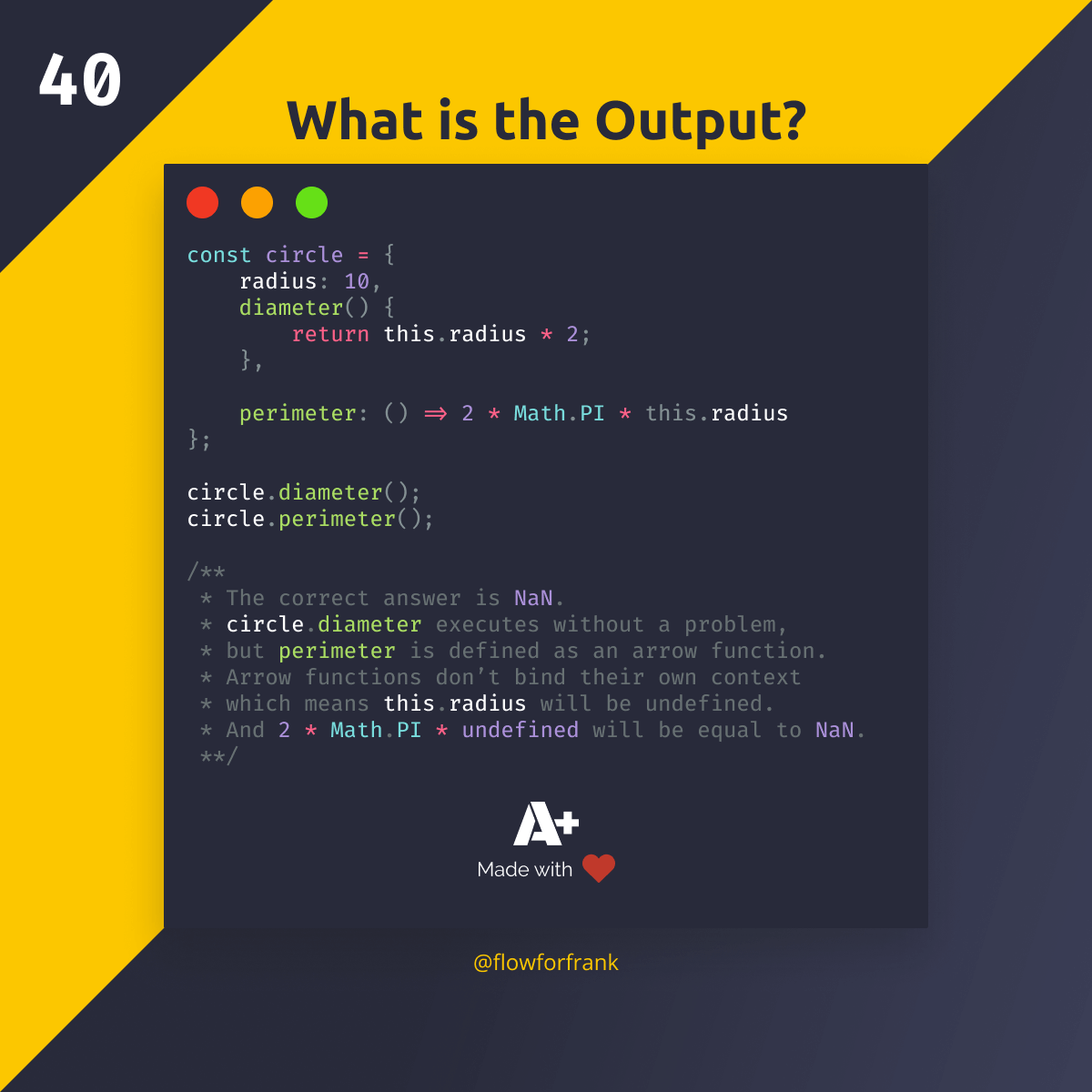
What is the Output of this Code?
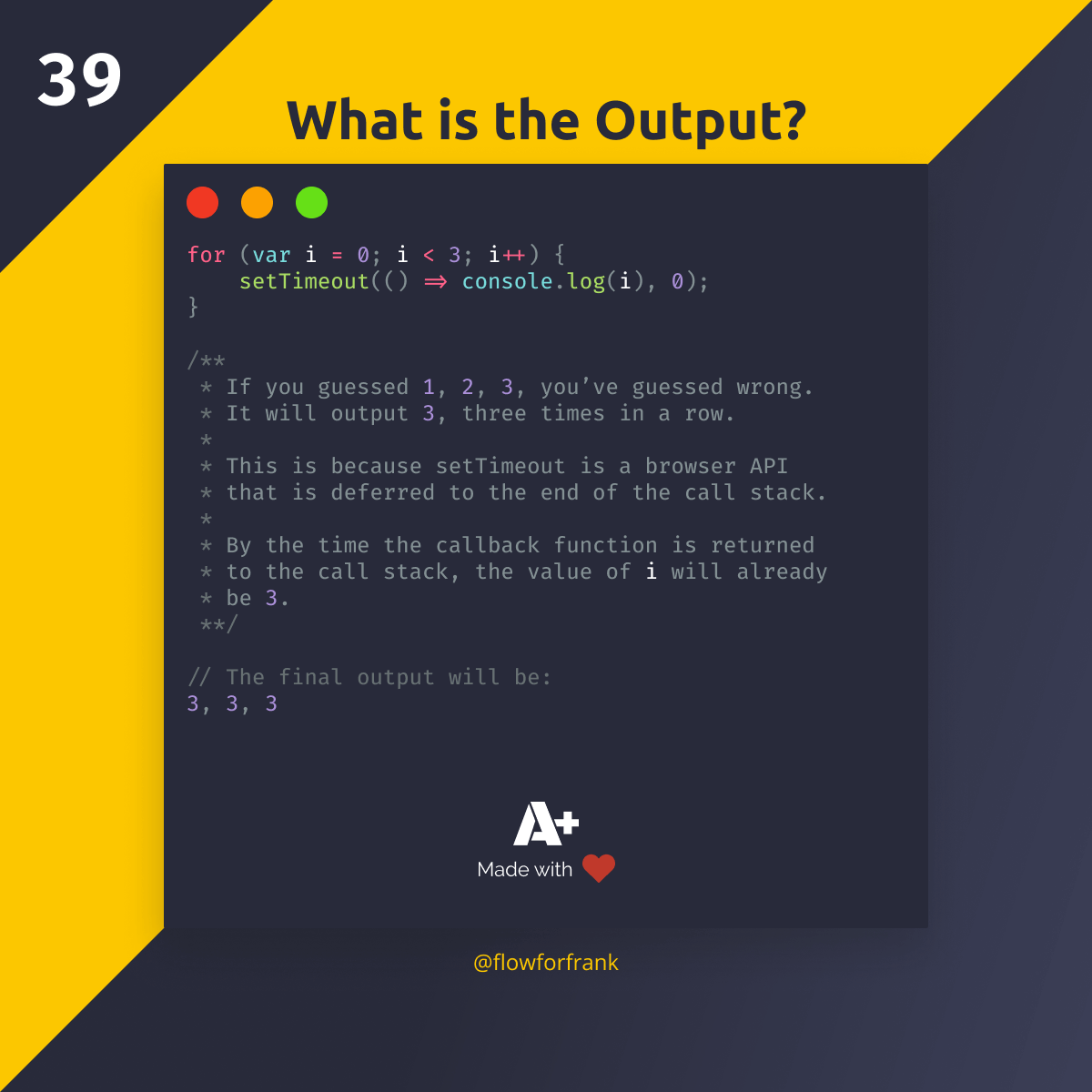
setTimeout in a for loop
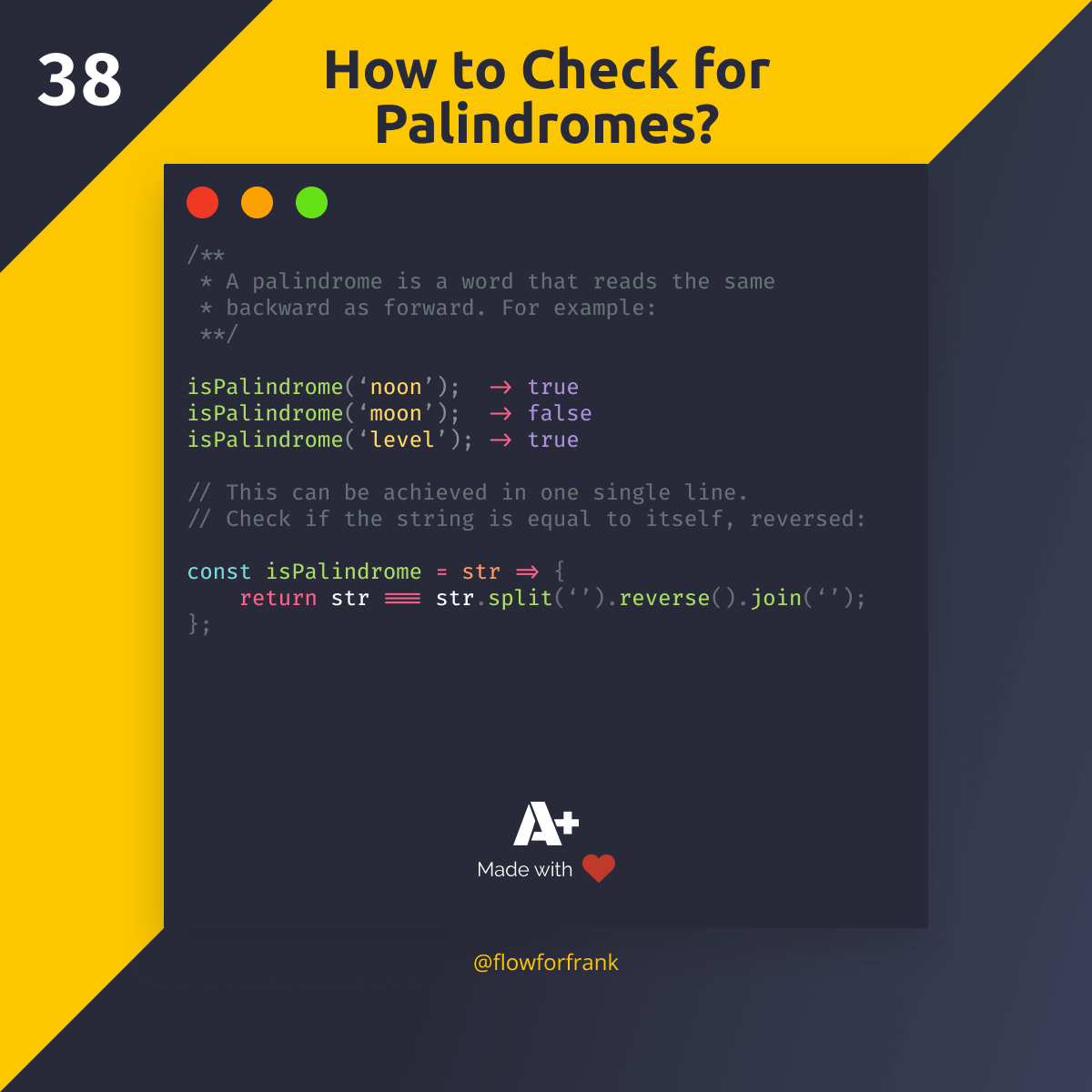
How to Check for Palindromes in JavaScript
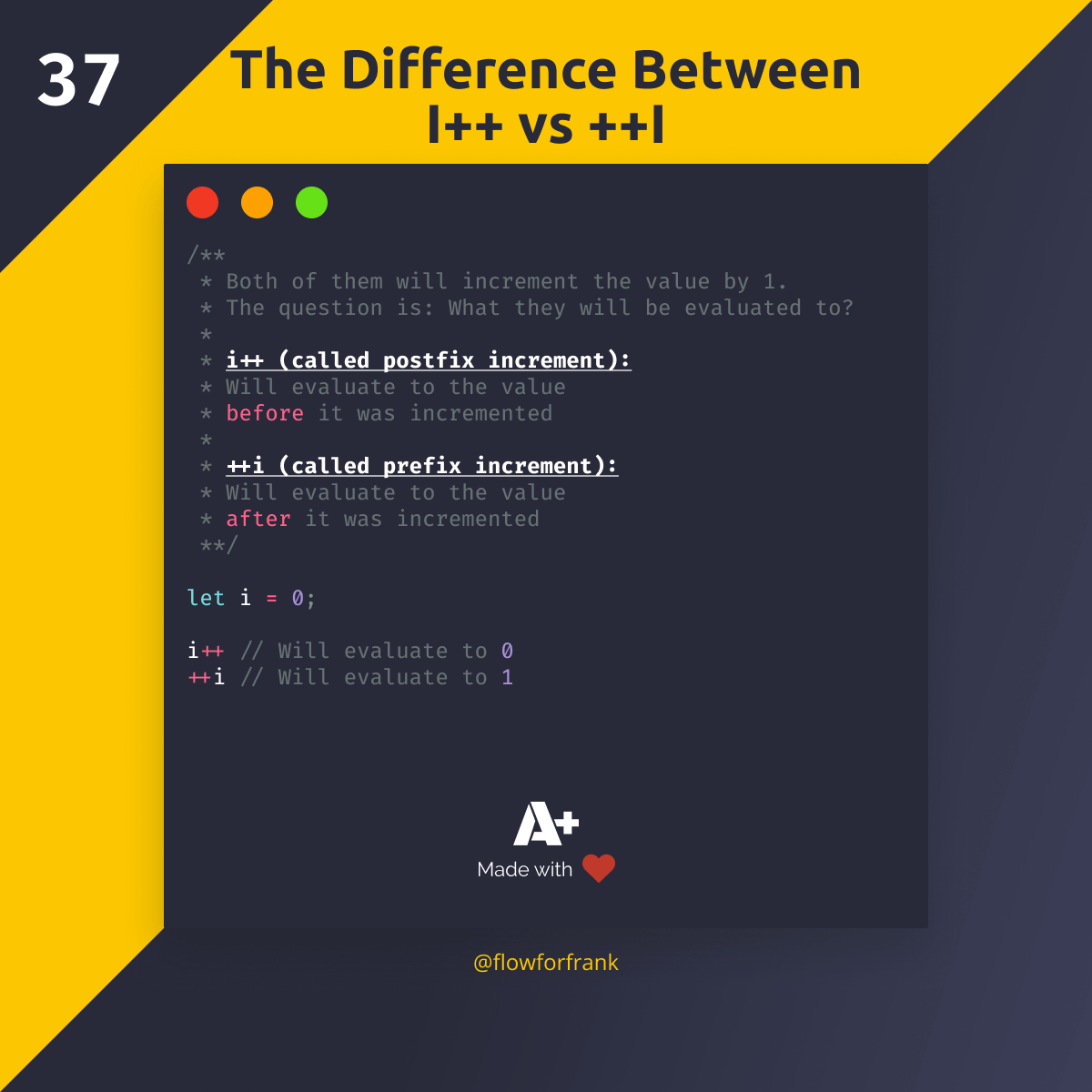
The Difference Between Postfix and Prefix Increment
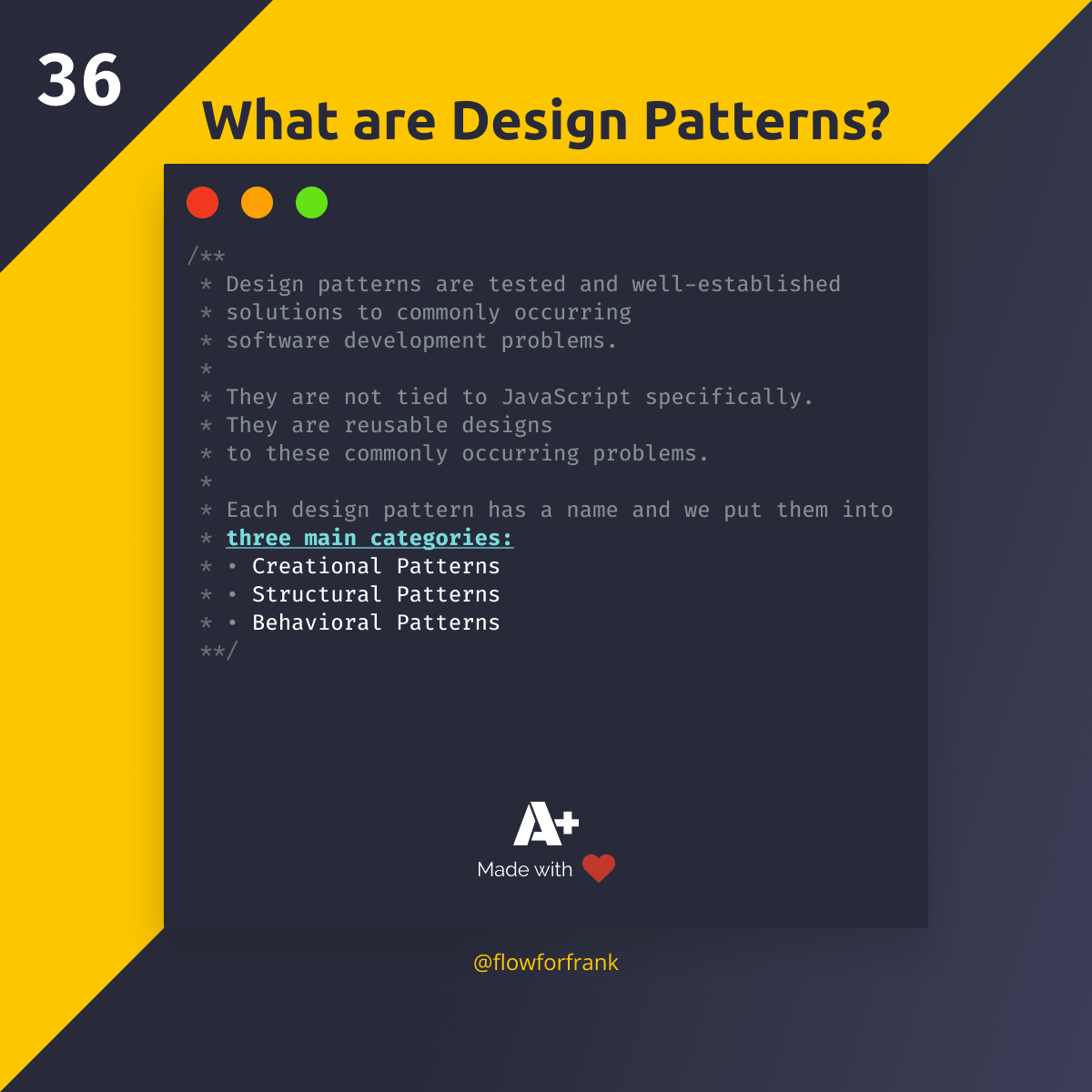
What are Design Patterns
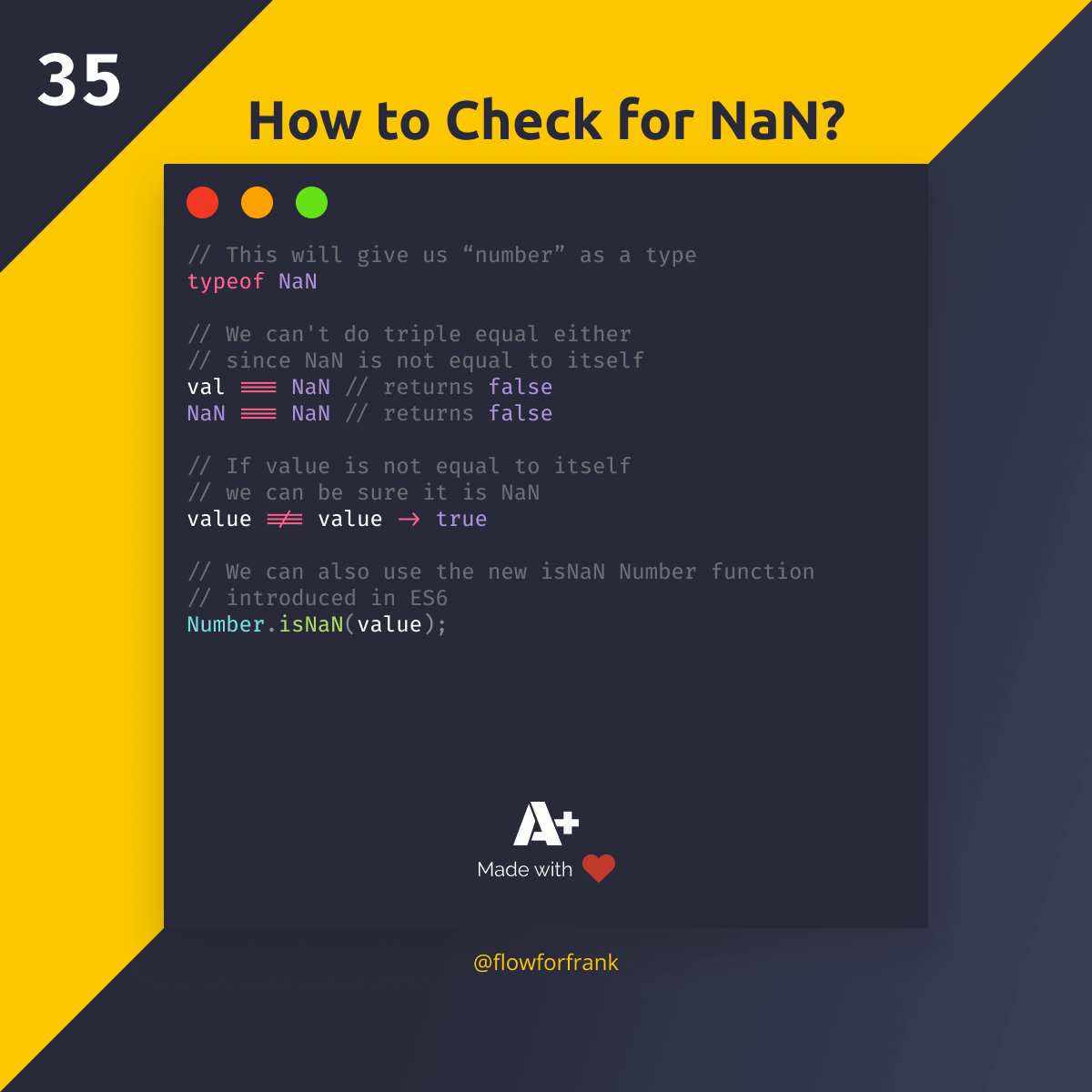
How to Check NaN in JavaScript
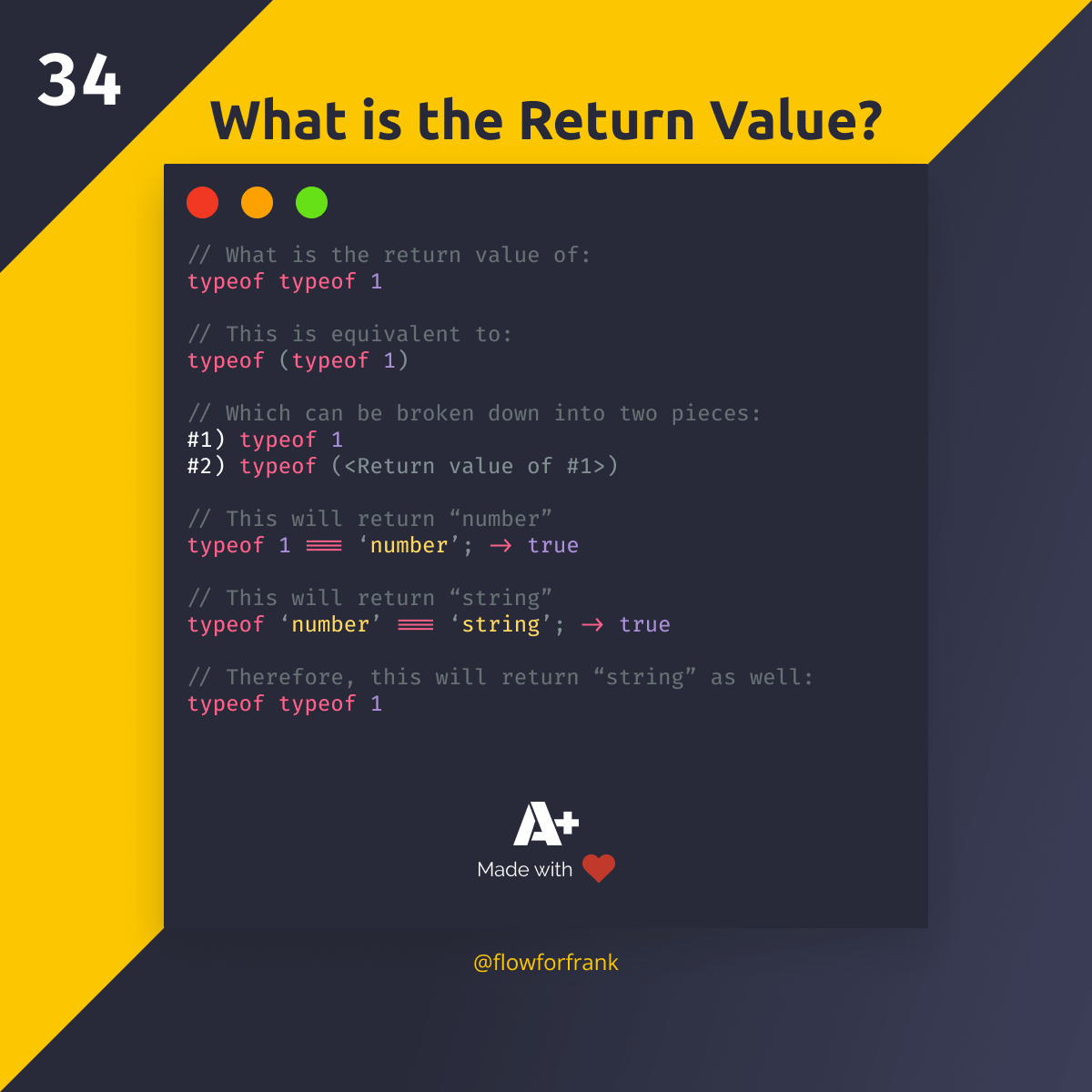
What is the Return Value of typeof typeof 1?
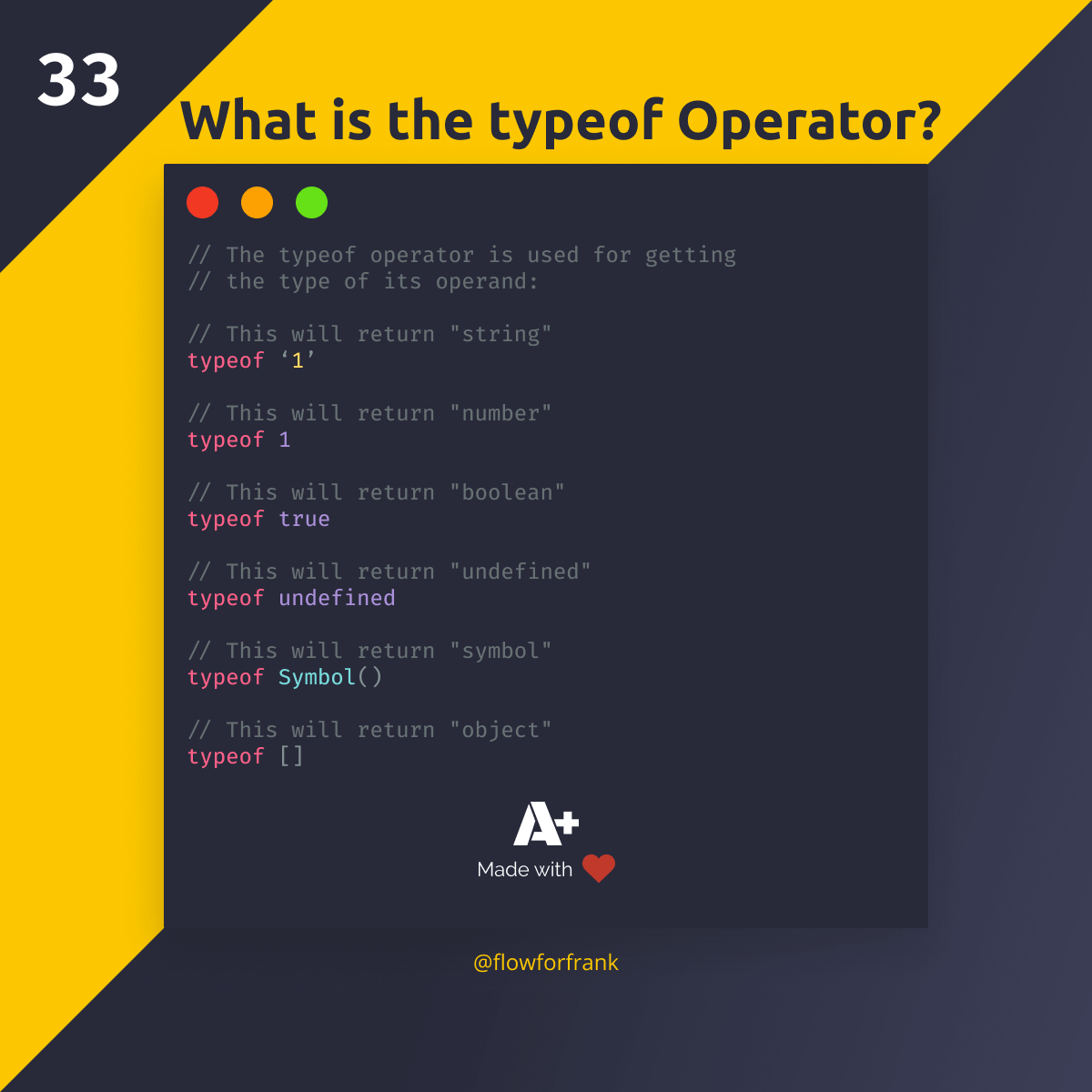
The typeof Operator in JavaScript
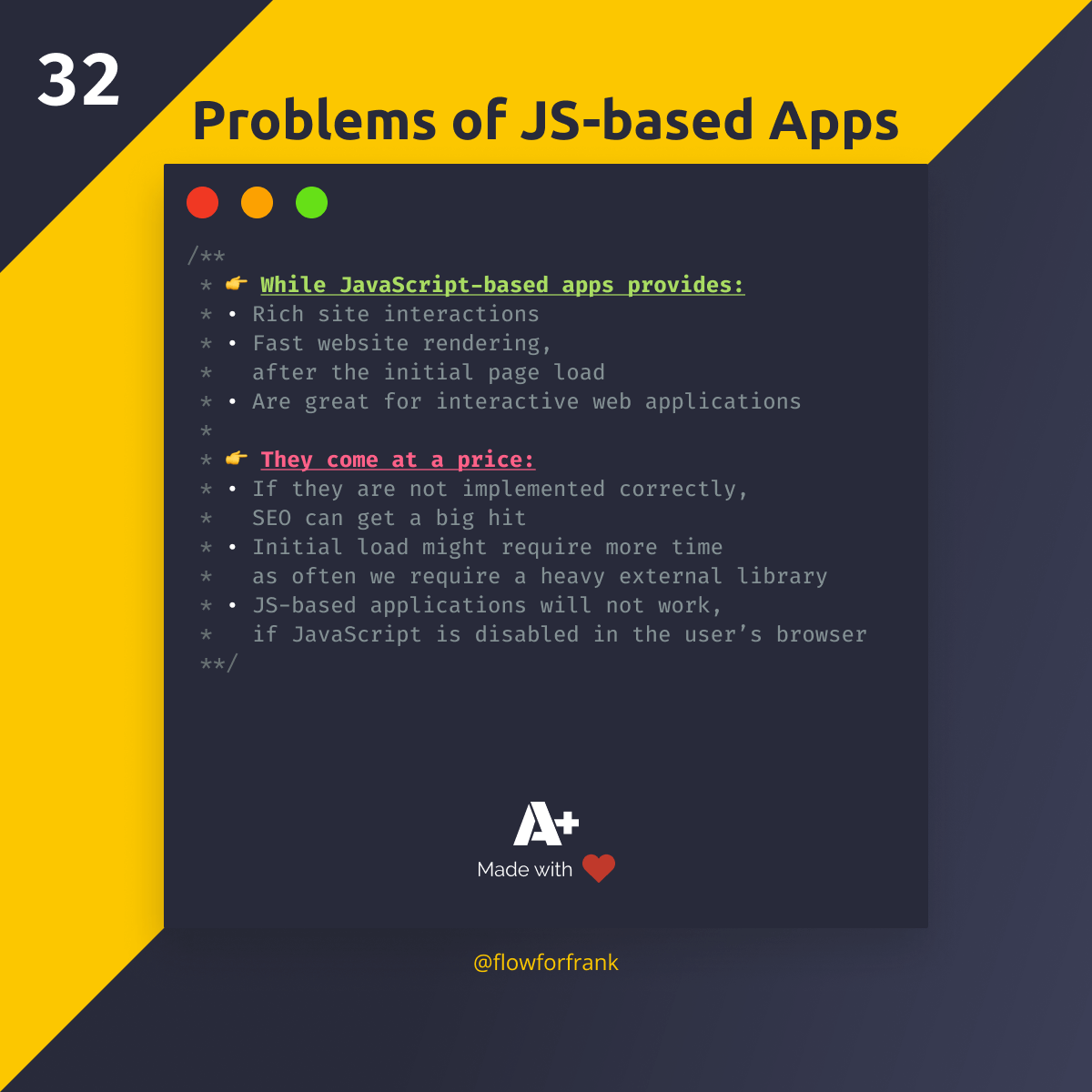
The Problems with JavaScript-based Applications
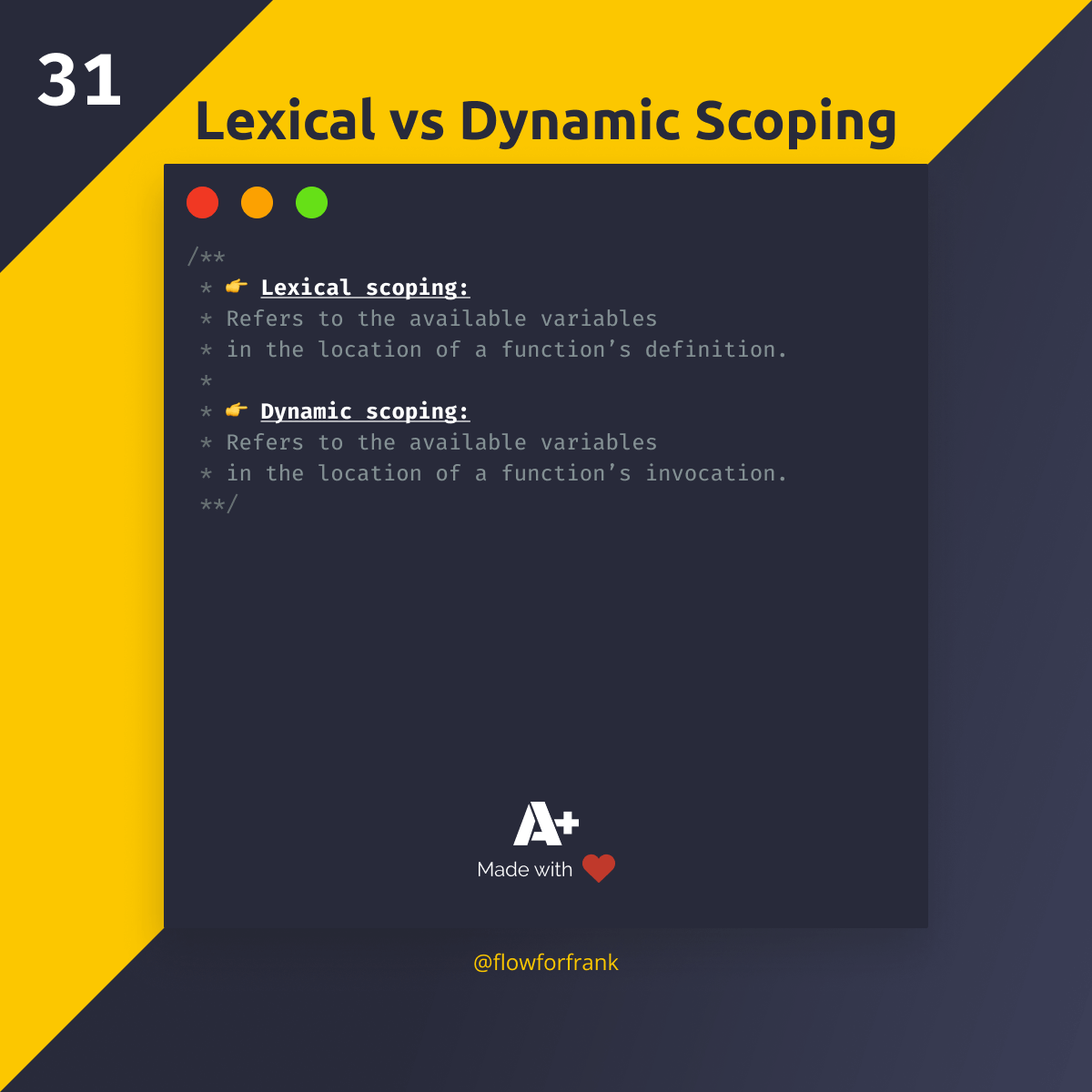
The Difference Between Lexical and Dynamic Scoping
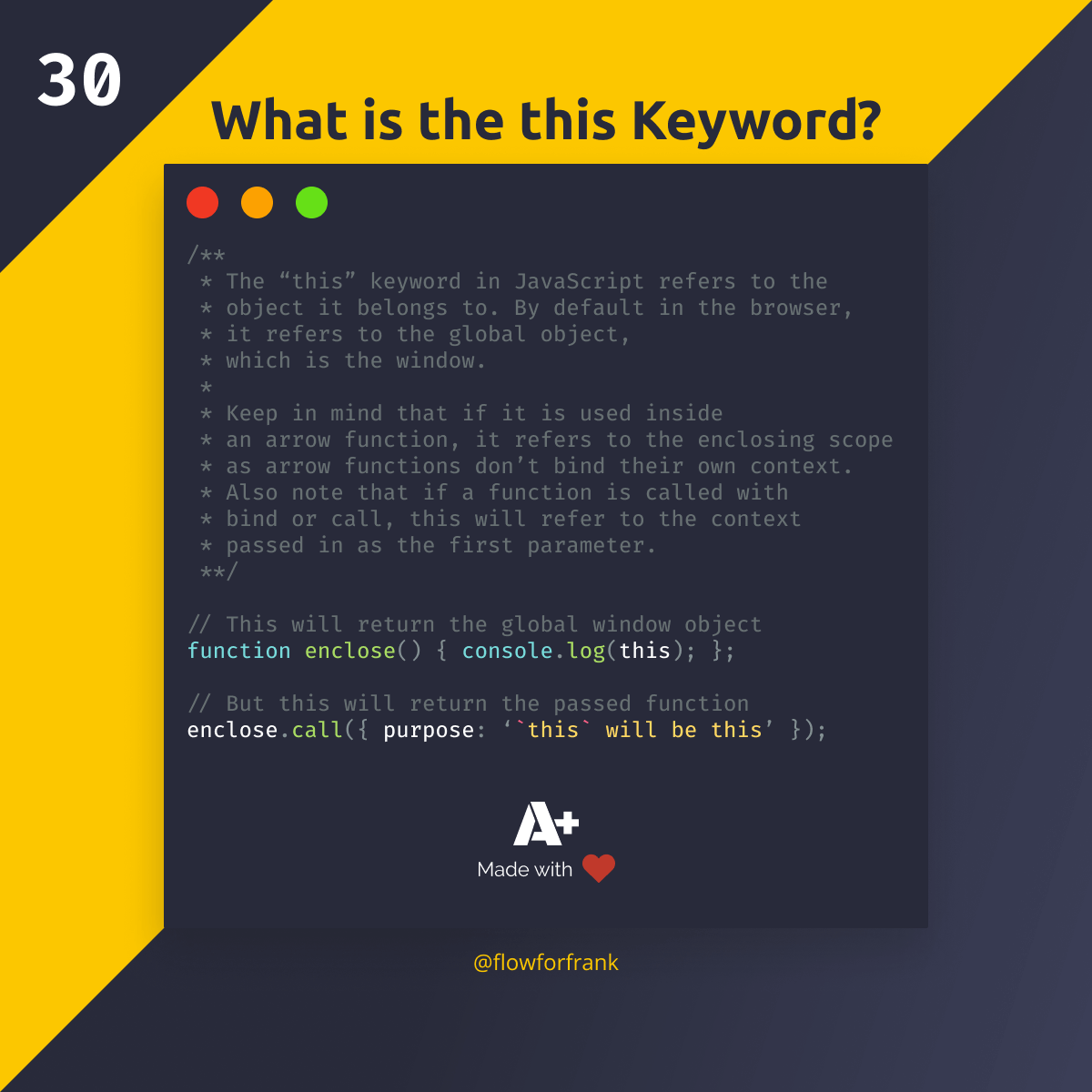
What is the this Keyword in JavaScript?
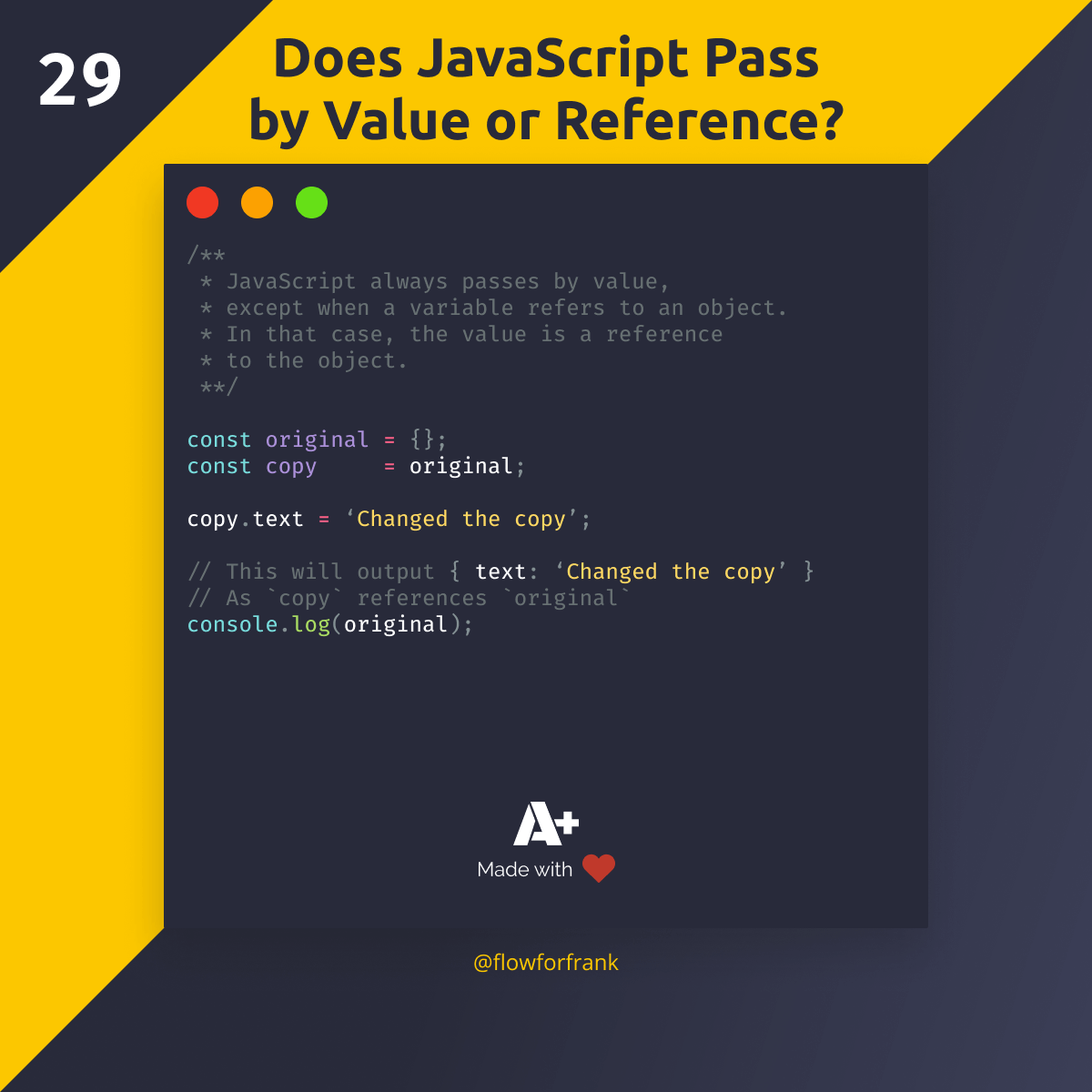
Does JavaScript Pass by Value or Reference?
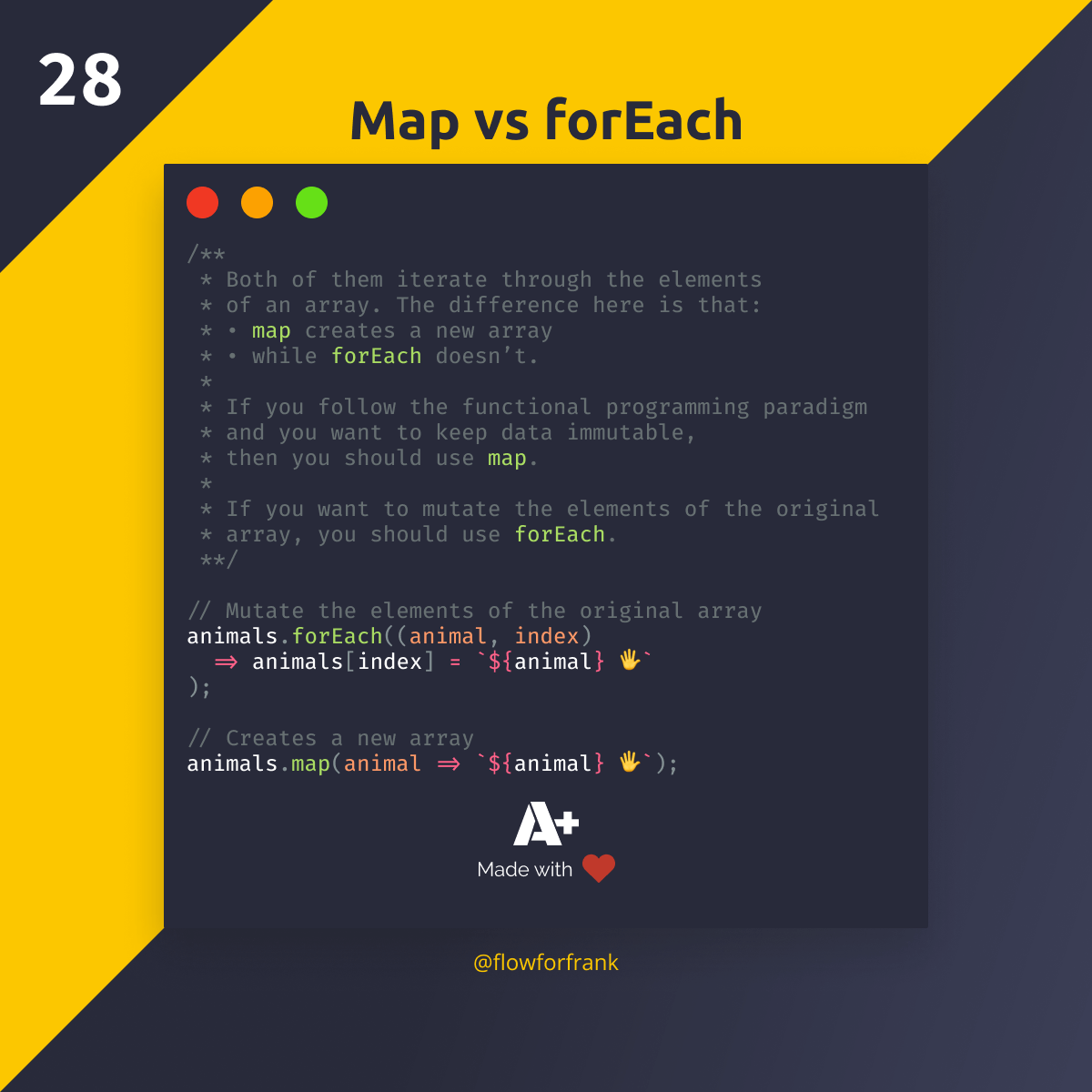
Map vs forEach in JavaScript
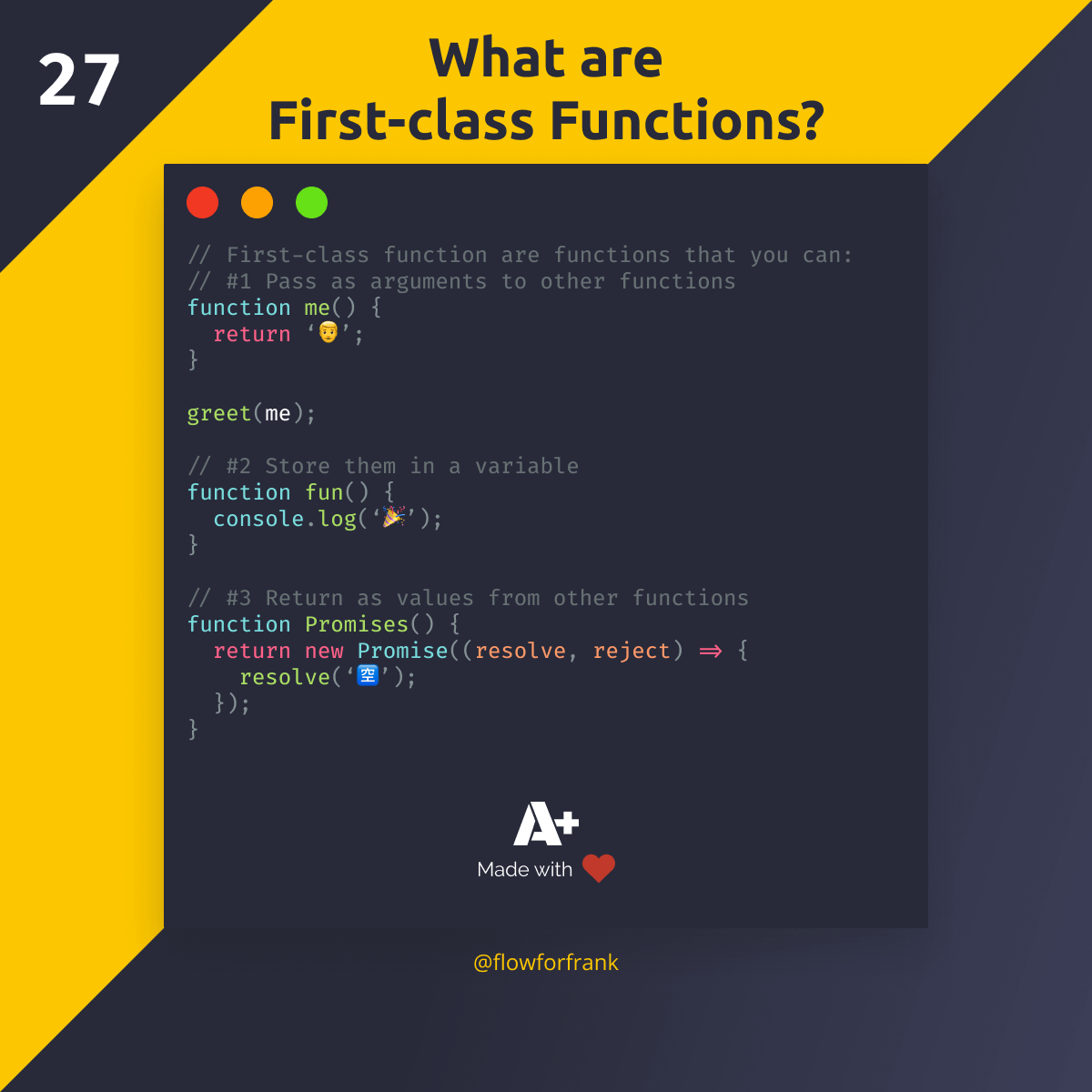