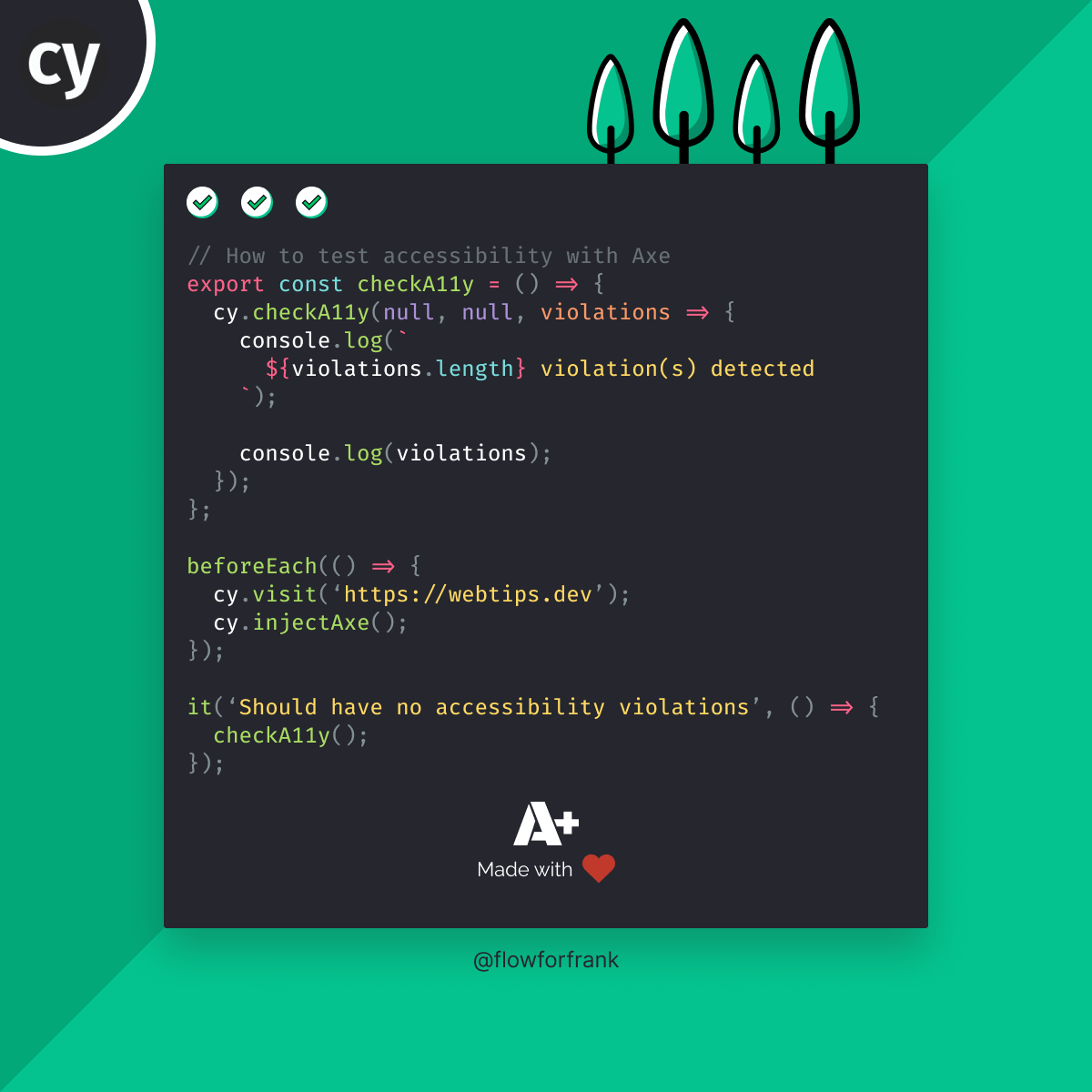
How to Test Accessibility with Cypress
Accessibility is an important topic when it comes to usability. To make your website accessible to the widest possible audience, you want to ensure that your website is easily perceivable, understandable, and navigable on multiple devices.
To test accessibility with Cypress, you can use the Axe plugin which helps you catch violations with a single Cypress command. But in order to do that, we first need to set up the plugin.
Setting Up Axe
To add Axe to Cypress, we will need to install two packages, axe-core
, and the plugin itself:
npm i --save-dev axe-core
npm i --save-dev cypress-axe
You will also need to import the plugin in your support file, after your commands:
import './commands'
import 'cypress-axe'
And we are all set and ready. Now we can use Axe inside Cypress. By default, however, accessibility violations that are logged are not user friendly. To make our work a little bit easier, let's also create a custom command that we can later reuse throughout our spec files and log more information about the violations.
Adding a Custom Command for Axe
To create a custom command, create a new file called accessibility.js
, and export the following function that you can later import in your spec files:
export const checkA11y = options => {
cy.checkA11y(null, options, violations => {
console.log(`${violations.length} violation(s) detected`);
console.table(violations);
});
};
This file exports a function called checkA11y
. A11y is short for accessibility. It calls the Cypress command that is provided by the plugin. This command can take three arguments:
- A context that tells Axe which part of the DOM we are interested in. Since we want to test the whole page, we can leave this
null
. Otherwise, you can specify a DOM selector here. - Custom options that specify how we want Axe to behave. For the full list of available options, see the resources section at the end of the article. It also includes a special
includedImpacts
option, which can be used to filter violations based on impact. We will take a look at how we can use this later on. If you don't want to override the default behavior, you can also keep this onenull
. - A callback function that defines what should happen if there are accessibility violations. Here we only want to log additional information to the console.
Now let's take a look at how we can use this function in our test cases.

Using Axe
First, you want to inject the plugin into the test file to make sure that cy.checkA11y
is available. You can do this using cy.injectAxe
:
import { checkA11y } from '../accessibility'
describe('Accessibility', () => {
before(() => {
cy.visit('https://webtips.dev');
cy.injectAxe();
});
it('Should have no a11y violations', () => checkA11y());
});
Then you can call the imported custom accessibility function to check for violations. If you would like to override the default behavior, you can pass an options object to the function:
it('Should have no a11y violations', () => {
checkA11y({
includedImpacts: ['critical']
});
});
As discussed earlier, the options object can also take a special key called includedImpacts
. The above function call will only catch critical accessibility violations. Apart from "critical", you can also pass "minor", "moderate", or "serious".
Want to learn Cypress from end to end? Check out my Cypress course on Educative where I cover everything:

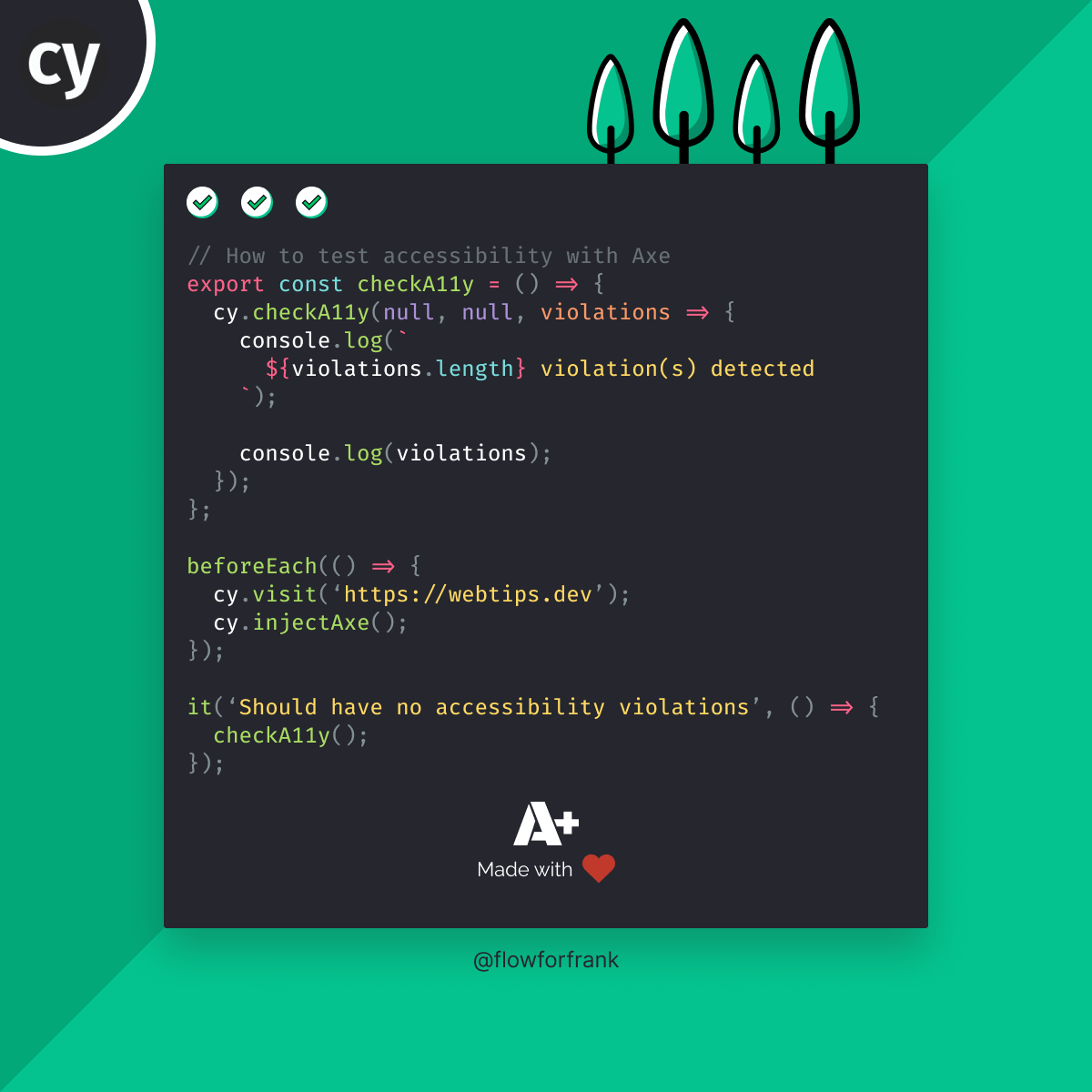
Resources:
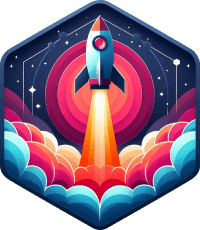
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: