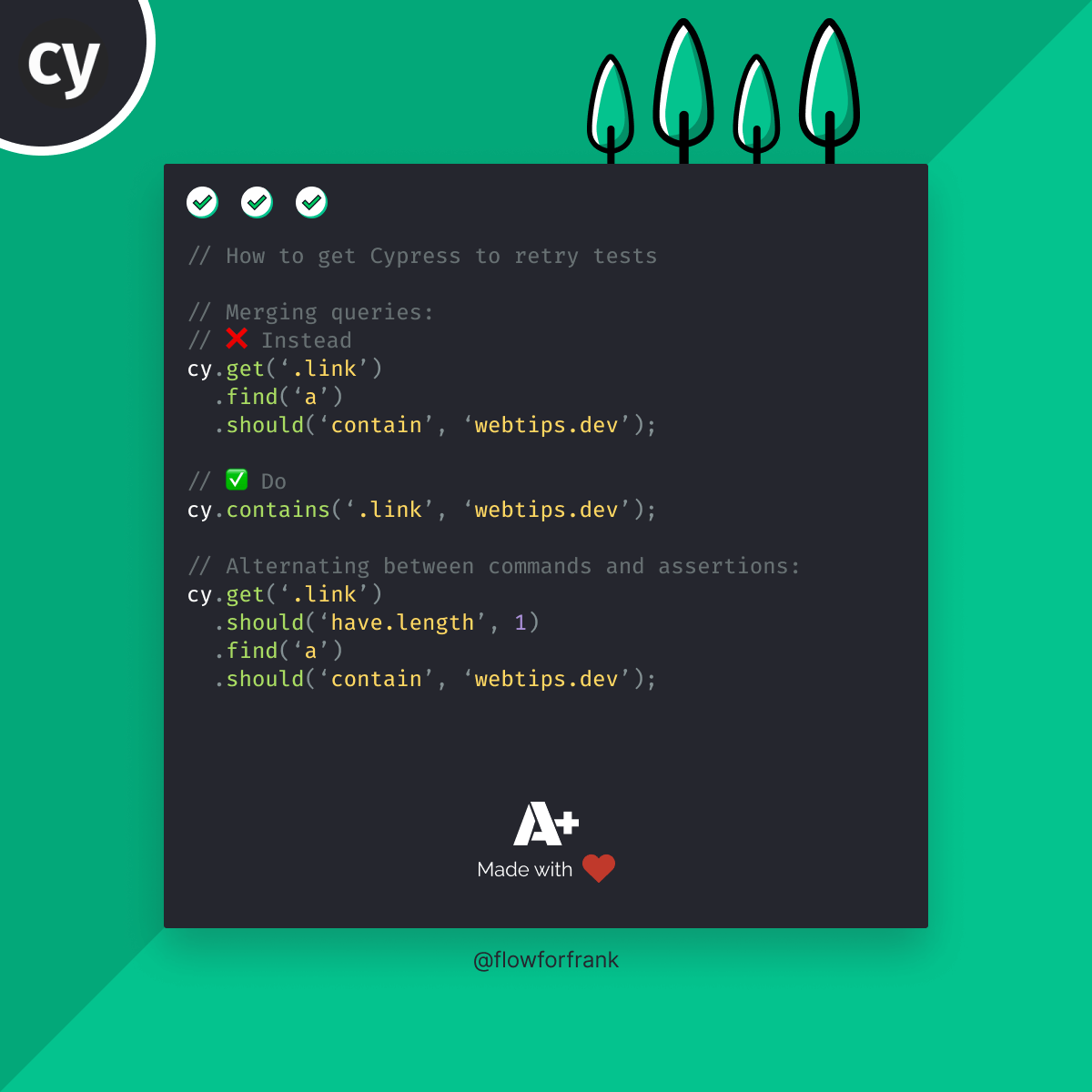
How to Get Cypress to Retry Tests
Cypress automatically retries your tests in most cases, but there are also several ways you can ensure that tests are retried correctly, such as:
- Merging queries
- Alternating between commands and assertions
- Increasing timeouts
- Configuring test retries globally
Merging queries
Merging multiple queries into one is one way. This is useful because Cypress only retries the last command before an assertion automatically. For example, let's say we have the following query:
cy.get('.link')
.find('a')
.should('contain', 'webtips.dev');
Here we want to query .link
elements and want to find anchors inside them to verify that they contain a certain text. The problem here is that Cypress will only retry the last command before the assertion, so only .find
will be retried automatically, but not cy.get
. To battle this, we can rewrite the above verification to the following:
cy.contains('.link', 'webtips.dev');
Now we have combined multiple commands into cy.contains
which is automatically retried as a single command.
Alternating between commands and assertions
Another way to force retries is by alternating between commands and assertions. As mentioned earlier, Cypress only retries the last command automatically before an assertion, so in order to retry multiple commands, we can add an assertion after each command. Let's take the previous example, and see how we can rewrite it to maximize the use of retry-ability:
// β Instead
cy.get('.link')
.find('a')
.should('contain', 'webtips.dev');
// β
Do
cy.get('.link')
.should('have.length', 1)
.find('a')
.should('contain', 'webtips.dev');
Notice that in the second example, we have an additional .should
right after cy.get
to alternate between command and assertions. Now both cy.get
and .find
will be retried automatically.

Increasing timeouts
You can increase your timeouts globally by setting the defaultCommandTimeout
property in your cypress.json
configuration file (By default, this is set to 4000 milliseconds). Or you can also increase timeouts for individual commands, like so:
cy.get('.link', { timeout: 10000 }); // Retry for 10 seconds
cy.get('.link', { timeout: 0 }); // Disable retries
It's important to point out that you should only increase timeouts as a last resort, and you should try to enforce retries in other ways as discussed in this tutorial.
Configuring test retries globally
Lastly, you can also configure retries globally through your cypress.json
configuration file with the retries
property:
{
"retries": {
"runMode": 2, // Used for cypress run, defaults to 0
"openMode": 0 // Used for cypress open, defaults to 0
}
}
You can also define the number of retries, regardless of mode by simply passing a number to the retries
property:
{
"retries": 2
}
Want to learn Cypress from end to end? Check out my Cypress course on Educative where I cover everything:

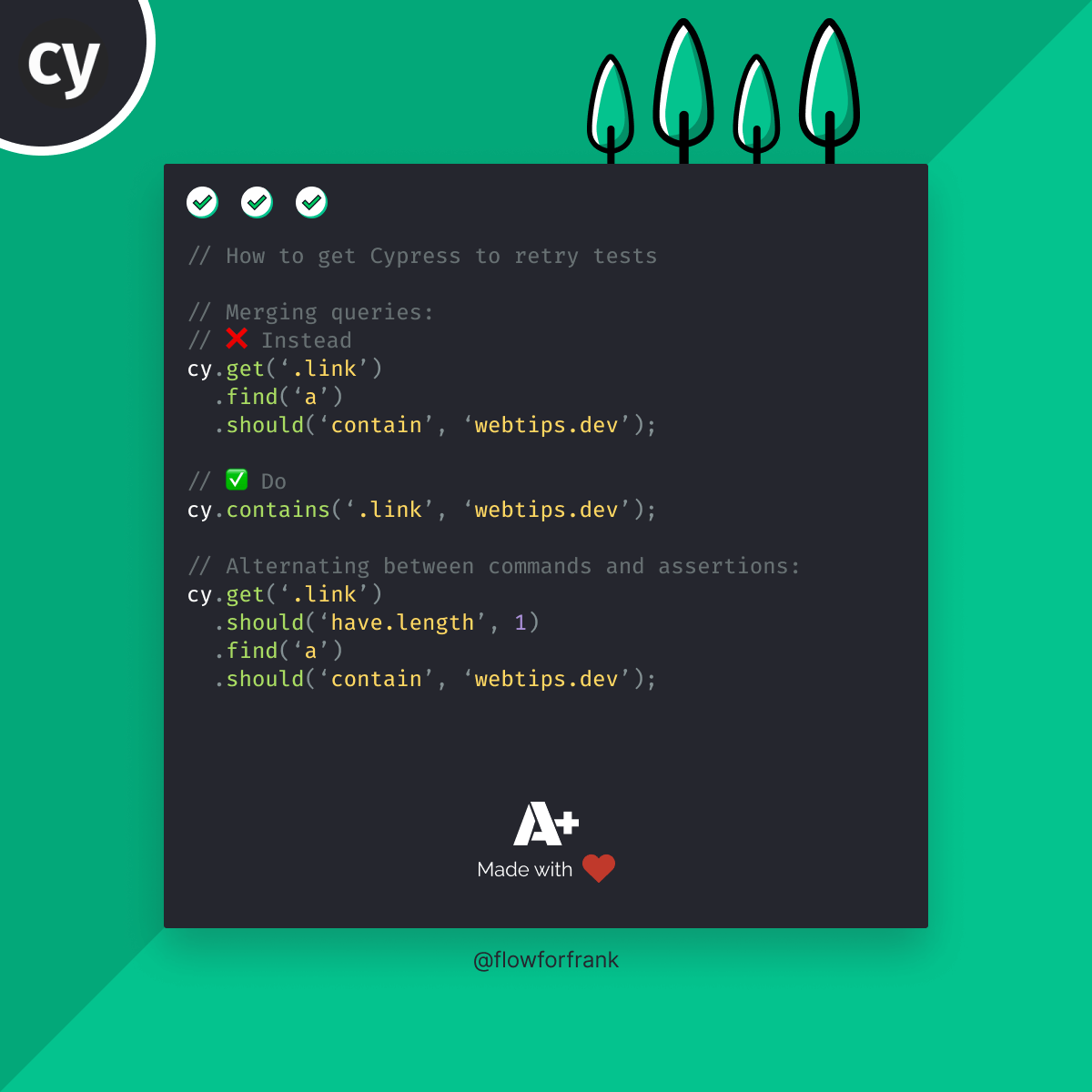
Resources:
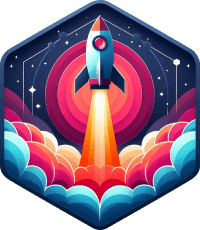
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: