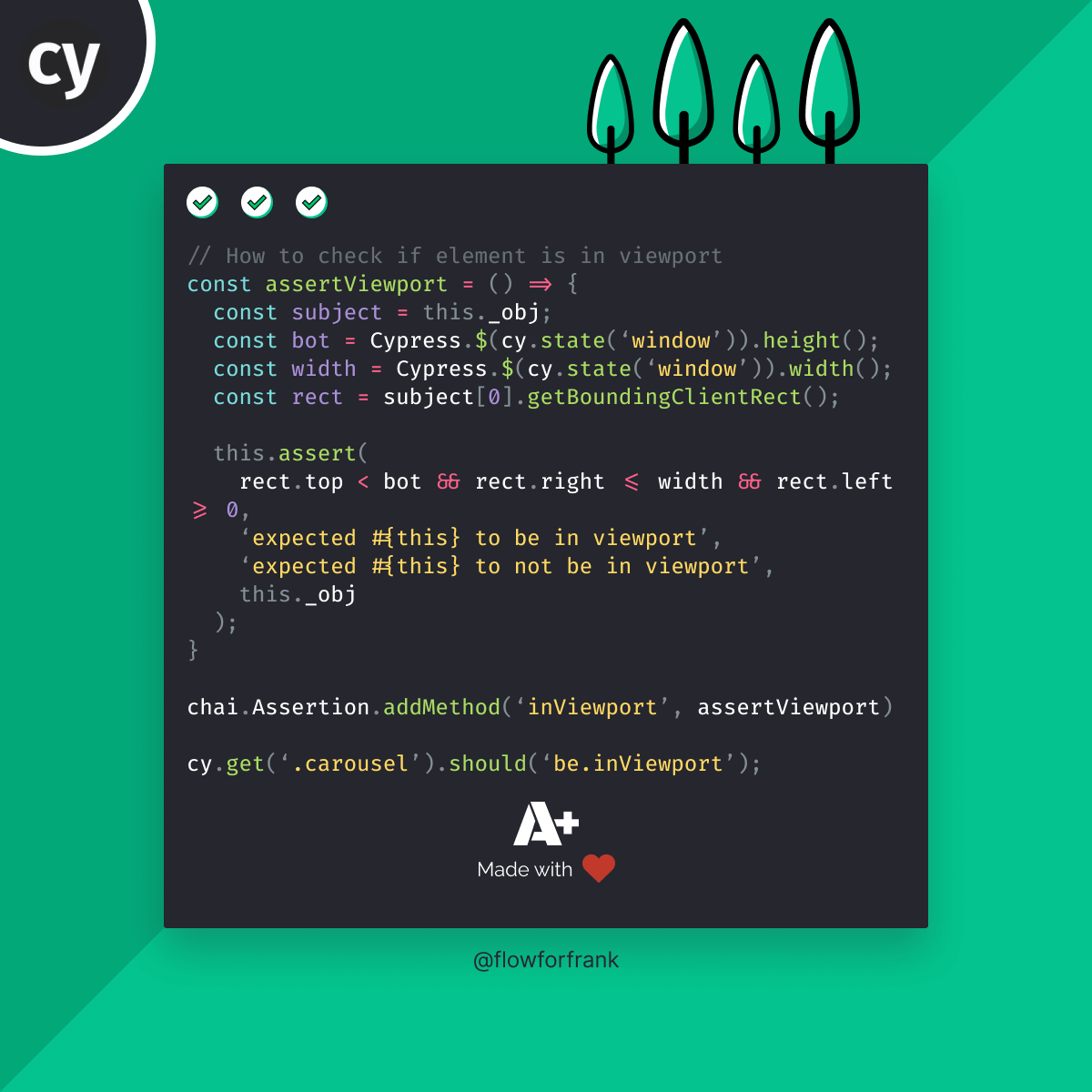
How to Check if Element is in Viewport in Cypress
To check if an element is in the viewport in Cypress, we can add a custom assertion to Chai using the support
folder. Create a new file called inViewport.js
and export the following function:
export default _chai => {
function assertIsInViewport() {
const subject = this._obj;
const bottom = Cypress.$(cy.state('window')).height();
const width = Cypress.$(cy.state('window')).width();
const rect = subject[0].getBoundingClientRect();
this.assert(
rect.top < bottom && rect.right <= width && rect.left >= 0,
'expected #{this} to be in the viewport',
'expected #{this} to not be in the viewport',
this._obj
);
}
_chai.Assertion.addMethod('inViewport', assertIsInViewport);
}
This function will add a new assertion to Chai. Using this.assert
, we can verify if the subject (the element that the assertion is chained from) is within the viewport or not. We can also define error messages as the second and third parameters of this function, and even pass the subject using #{this}
.
In order to use this new assertion, inside the index.js
file in your support
folder, import it, and assign it to Chai:
import inViewport from './inViewport'
before(() => {
chai.use(inViewport);
});
This before
hook will run once before all test files and adds the new assertion to Chai. Now you can call these assertions in the following way in your test cases:
cy.get('h1').should('be.inViewport');
cy.get('h1').should('not.be.inViewport');
Want to learn Cypress from end to end? Check out my Cypress course on Educative where I cover everything:

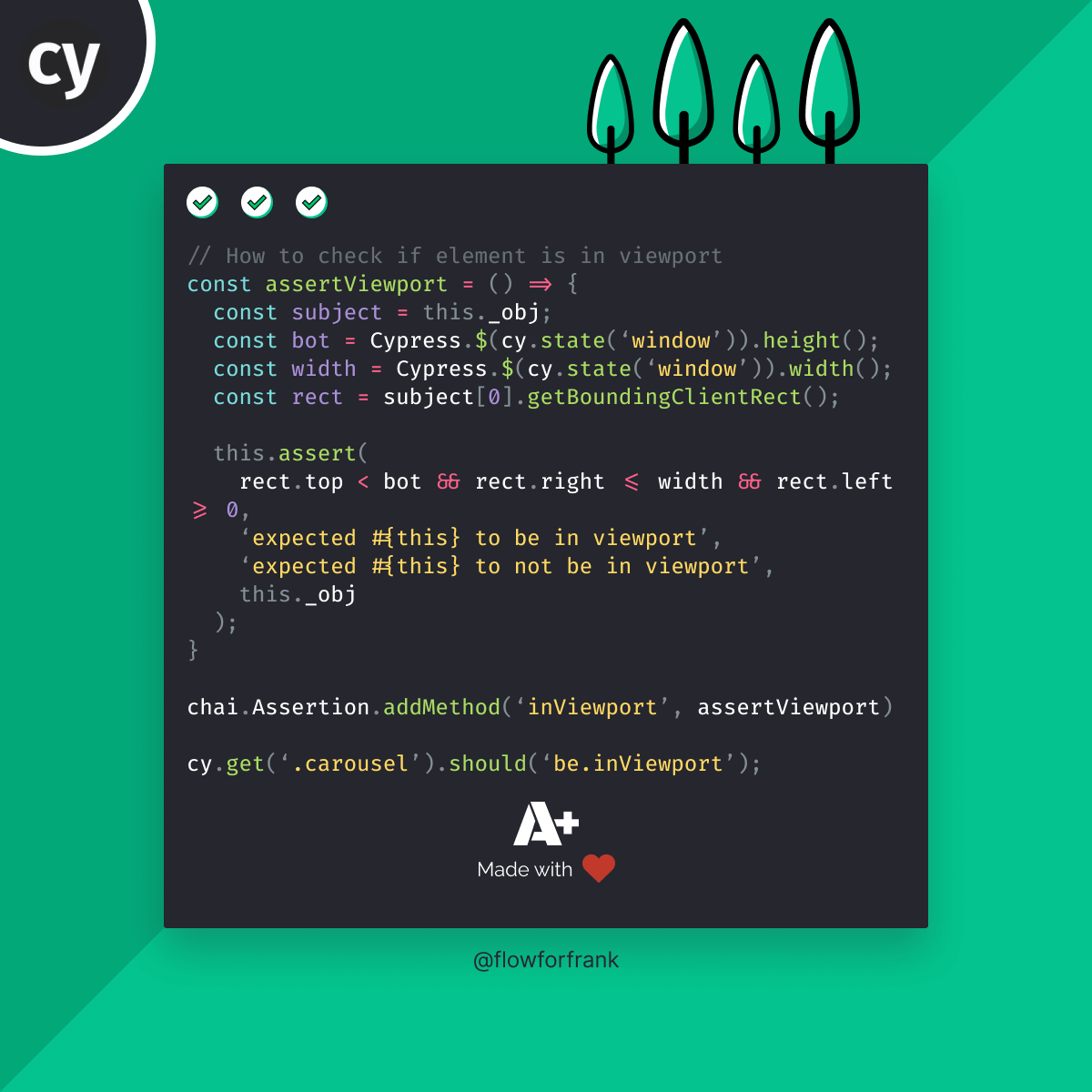
Resources:
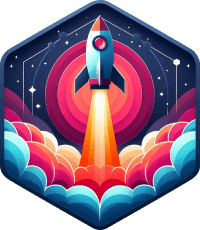
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: