How to Fix "Unexpected token o in JSON at position 1"
In order to fix the "Uncaught SyntaxError: Unexpected token o in JSON at position 1" error in JavaScript, you need to make you are passing a valid JSON string to JSON.parse
. Take a look at the following example:
// β Don't
// This will result in "Uncaught SyntaxError: Unexpected token o in JSON at position 1"
JSON.parse({})
// βοΈ Do ensure that you pass a valid JSON
const obj = JSON.stringify({})
JSON.parse(obj)
The error happens when JSON.parse
gets an invalid JSON string. The reason this error message happens is that you try to pass an empty JavaScript object to JSON.parse
, resulting in the error.
The object will be converted into a string, and the first character of the converted string happens to be the letter "o". Try to convert an empty object to a string in the following way, and pass the converted string to JSON.parse
. You will get the exact same error.
// Converting the empty object to a string
String({})
<- '[object Object]'
// Will result in the same error message
JSON.parse('[object Object]')
If you know the value you are passing to JSON.parse
is already a JavaScript object, then you don't need to use JSON.parse
. On the other hand, if you want to convert a value into valid JSON, you need to use JSON.stringify
:
// Use JSON.stringify to convert values to JSON
JSON.stringify({ ... })
The General Way to Fix the Error
Most of the time, you may not know upfront the value of the variable you are passing to JSON.parse
, as the data may be originating from a server. A general way to ensure that you don't run into this error is to use a try-catch
where you try to parse the passed value, and catch the error if there is any.
try {
JSON.parse({})
} catch (error) {
// This will log out: "SyntaxError: Unexpected token o in JSON at position 1"
console.log(error)
}
You can also introduce the following helper function in your codebase, which does the same thing internally. Simply pass the value that you would pass to JSON.parse
, and it will either return the parsed value or null
.
const parseJSON = json => {
try {
return JSON.parse(json)
} catch (error) {
return null
}
}
parseJSON({}) // Returns null
parseJSON('{}') // Returns {}
You can also use online tools such as JSONLint or JSON Formatter & Validator, in order to validate whether the value you are passing is a valid JSON or not.
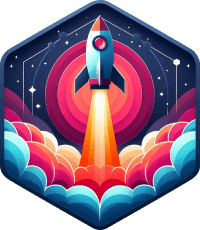
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: