Testing Disabled Buttons in React Testing Library
To test whether your buttons are disabled or not in React Testing Library, you need to use the toHaveAttribute
or the toBeDisabled
assertion in the following way:
import { render, screen } from '@testing-library/react'
it('should render a disabled button', () => {
render(<Cta disabled={true}>Learn more!</Cta>)
expect(screen.getByTestId('button')).toHaveAttribute('disabled')
expect(screen.getByTestId('button')).toBeDisabled()
})
toHaveAttribute
also accepts a second parameter for the value if you need to test specific attribute values.
Prefer using getByTestId
to avoid breaking your unit tests by small code changes. You can also test the opposite and verify if your buttons are not disabled by prepending a not
to either of the assertions:
import { render, screen } from '@testing-library/react'
it('should render a disabled button', () => {
render(<Cta>Learn more!</Cta>)
expect(screen.getByTestId('button')).not.toHaveAttribute('disabled')
expect(screen.getByTestId('button')).not.toBeDisabled()
})
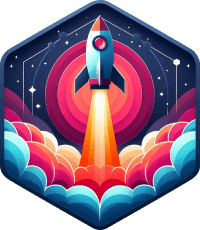
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: