Properly Testing Button Clicks in React Testing Library
In order to test button click events in React Testing Library, we need to use the fireEvent
API:
import { fireEvent, render, screen } from '@testing-library/react'
it('Should expand and collapse an accordion', () => {
render(
<div>
<p>Content</p>
<button data-testid="accordionButton">Expand</button>
</div>
)
// Prefer using getByTestId or getByRole
const button = screen.getByTestId('accordionButton')
const button = screen.getByRole('button')
const button = screen.getByText('Expand')
expect(screen.queryByText('Content')).toBeNull()
fireEvent.click(button)
expect(screen.getByText('Content')).toBeInTheDocument()
fireEvent.click(button)
expect(screen.queryByText('My Content')).toBeNull()
})
Once you have the rendered component, you will need to grab the button using screen.getByTestId
. You can also use other methods such as getByRole
or getByText
, but preferably, you want to use getByTestId
to avoid breaking your test cases by changing the copy.
Then you can call fireEvent.click
and pass the queried button to simulate a click event. Notice that you can fire the same event multiple times. In the above example, we try to verify if the accordion can be opened and closed with two different click events.
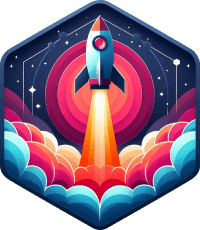
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: