How to Refresh Pages in React With One Line of Code
To refresh a page in React, you don't need React itself β just plain JavaScript. Call window.location.reload
, which triggers a full page reload.
export const App = () => {
const refresh = () => window.location.reload(true)
return (
<button onClick={refresh}>Refresh</button>
)
}
This functions like a refresh button. Note that we pass true
to the reload
method. This non-standard feature is supported only in Firefox, instructing the browser to bypass the cache and force a reload.
Using a boolean parameter for location.reload
is ignored by all other browsers except Firefox.
Alternatively, we can achieve this using an inline onClick
handler:
<button onClick={() => window.location.reload(true)}>Refresh</button>
However, in most cases, consider updating your state with useState
instead of a full page reload for a better user experience. Use the above solution only when a complete page reload is necessary.
Refresh by Updating the State
If you don't want a full page reload, refresh the page by updating the state. In the following example, we have a table where values are calculated based on other fields. Try changing the first and last name cells in the interactive widget below to update the state.
Table.jsx
App.jsx
data.js
Using the useState
hook, we can refresh the table and the page without a page reload. The updateTable
function is called on input changes, updating both the state and UI.
For demonstration purposes, the above code has been shortened. In a real-world scenario, make sure to use thead
and tbody
for correct semantics.
Refresh by Resetting State
Another approach to page refresh without a full reload is to reset the state back to its original values. This can be done with a reset button that calls the updater function of the useState
hook with the initial values, essentially, resetting the page. Update the code in the interactive widget above according to the following to reset the state:
return (
<React.Fragment>
...
</table>
<button onClick={() => setUsers(data)}>Reload</button>
</React.Fragment>
)
Don't forget to wrap the table and button elements in a fragment and pass the initial object to setUsers
to reset the state.

Maintaining State After Refresh
There can also be cases where we want to do a full page reload, but keep track of the state after the reload. This can be achieved through the use of the Local Storage API. Local storage helps us keep data in the user's browser. For this, we'll need to introduce the following two functionalities:
- Capture the updated state and store it in local storage.
- Create a
useEffect
hook to reset the state to its previous value on page load.
Table.jsx
App.jsx
data.js
Try changing the values in the table in the above code example, then click on the "Refresh Page" button. The state will be saved through page reloads. The state is saved on line:21 and reset on line:29.
Note that we need to use JSON.stringify
and JSON.parse
to save and retrieve the data from Local Storage. The API handles only strings, so we need to handle conversions.
Once the state is set, we can use JSON.parse
to turn the string back into a JavaScript object and pass it to setUsers
to update the state.
Refresh Every X Seconds
Last but not least, it's also possible to refresh the page every X seconds, if you need to achieve automatic refreshes. This can be done by wrapping the very first solution into a setTimeout
in the following way:
setTimeout(() => {
window.location.reload()
}, 10_000)
This will refresh the page every 10 seconds. When the page is reloaded, the setTimeout
will be called automatically, which executes the wrapped code after a set of milliseconds, passed as the second parameter. In our case, this is set to 10 seconds or 10,000 milliseconds.
Note that you can use underscore in numbers for JavaScript to make them more readable. The above example is equivalent to 10000
.
Summary
In short, for a full-page reload in React, use window.location.reload
. Otherwise, consider the following:
- Refresh page without a reload: Use
useState
to update the internal state. - Refresh page to initial value: Call the
useState
updater function with initial values. - Maintain state after reload: Use local storage to store and restore the state.
- Refresh page every X seconds: Wrap your code in a
setTimeout
.
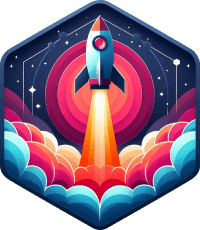
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: