How to Use Inline Styles in React
When it comes to styling components in React, the recommended way is to use CSS stylesheets. However, in a small number of cases, you may need to use inline styles. To use inline styles in React, we need to pass an object to the style
attribute on the JSX element:
const App = () => {
return (
<h1 style={{ color: 'yellow' }}>
This heading has inline styles
</h1>
)
}
Notice the double curly braces. The outer one is defining the JavaScript expression, while the inner ones are for the actual object that is passed to the expression. The CSS properties in this case are the object keys, while the object values are the styles associated with the CSS property.
Outsourcing Styles to Objects
To improve upon the previous example, we can outsource the inline style into a JavaScript object above the render method. This improves readability and maintainability. Take the following as an example:
const App = () => {
const styles = {
color: 'yellow',
backgroundColor: 'black'
}
return (
<h1 style={styles}>
This heading has inline styles
</h1>
)
}
When using inline CSS in JavaScript, dashed CSS properties must be converted to camelCase.
Note that this time, the object is passed to the style
attribute, so instead of the {{ ... }}
annotation, only one curly brace is used.
Using Multiple Inline Styles
When using multiple inline styles, it is common practice to define the styles in one object and create a nested object for each element with the styles defined. For example, the following component attaches styles to both h1
and h2
elements:
const App = () => {
const styles = {
h1: {
color: 'red',
backgroundColor: 'black'
},
h2: {
color: 'yellow'
}
}
return (
<React.Fragment>
<h1 style={styles.h1}>
This heading has inline styles
</h1>
<h2 style={styles.h2}>And this one too</h2>
</React.Fragment>
)
}

Why You Should Not Use Inline Styles
In most cases, you should avoid using inline styles and instead use regular CSS classes, whether they are imported as a module, used by Tailwind, or CSS in JS. When it comes to using inline styles, keep in mind that you don't want to use them for the following reasons:
- Hard to maintain: Using inline styles makes the code harder to read and maintain. They are also hard to debug and modify, and combining all of these can slow down the development process.
- Impacts performance: A large number of inline styles also negatively affect performance. They increase the size of the HTML file and prevent browsers from caching styles for faster page loads. This results in slower load times.
- Not reusable: It's easy to end up with code duplication when using inline styles, as it's hard to spot which parts to extract. This again leads to code that is harder to maintain.
- Has limitations: Inline styles also have limitations, such as the inability to use pseudo-classes or media queries. This limits the number of possible use cases with inline styles.
When You Should Use Inline Styles
So the question comes, when should I use inline styles? There can be two situations when using inline styles is reasonable:
- Dynamic styles: Dynamically generating styles is only possible through JavaScript. A common use case for this is animating the styles of an element on scroll to create interactive interfaces.
- Overriding third-party CSS: Another use case for inline styles is overriding existing CSS applied through third-party scripts or services. When you don't have control over the CSS and overriding styles with higher specificity is not an option, inline styles can be used as a last resort.
In conclusion, this means that whenever you need to use inline styles, use them sparingly and with caution. Use them as a last resort.
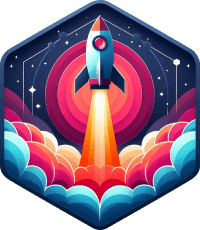
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: