How to Test onChange Events in React Testing Library
To test onChange
events in React Testing Library, use the fireEvent.change
API in the following way:
import React from 'react'
import { fireEvent, render, screen } from '@testing-library/react'
describe('onChange events', () => {
it('should test onChange behavior', () => {
render(<Dropdown />)
const select = screen.getByRole('select')
const testingLibrary = screen.getByRole('option', { name: 'React Testing Library' })
const jest = screen.getByRole('option', { name: 'Jest' })
fireEvent.change(select, { target: { value: 'π' } })
expect(select.value).toBe('π')
expect(testingLibrary.selected).toBe(true)
fireEvent.change(select, { target: { value: 'π΄' } })
expect(select.value).toBe('π΄')
expect(jest.selected).toBe(true)
})
})
To select an option from an HTML select
element, you will need to grab the element with either screen.getByTestId
or screen.getByRole
, and pass it over to fireEvent.change
with a target.value
object for targeting the right option.
Notice that you can also grab elements by specifying the value of their attributes inside an object, passed as a second parameter to screen.getByRole
.
Finally, to test if the correct element is selected, we can use select.value
and option.selected
for assertions.
expect(select.value).toBe('π΄')
expect(jest.selected).toBe(true)
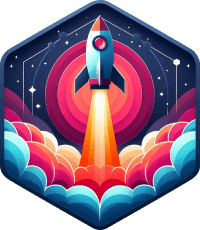
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: