3 Ways to Get Query Params in React (Without Router Too)
To get query params in React without using React Router, we can use the URLSearchParams API. Take the following URL as an example:
webtips.dev?tutorial=react
In order to get the value of the query param from the above URL, let's create a new URLSearchParams object and use its get
method with the name of the query param:
const Component = () => {
const searchParams = new URLSearchParams(document.location.search)
return (
<div>Tutorial: {searchParams.get('tutorial')}</div>
)
}
You will need to pass the name of the query param as a string to the get
method to retrieve its value. You can do this with as many parameters as needed. Make sure you pass document.location.search
to the URLSearchParams call in order to get the right query params.
With React Router v6
If you are using the latest version of React Router, it has a built-in useSearchParams
hook that we can use to get query parameters. You can use the hook in the following way:
import { useSearchParams } from 'react-router-dom'
const Component = () => {
const [searchParams, setSearchParams] = useSearchParams()
return (
<div>Tutorial: {searchParams.get('tutorial')}</div>
)
}
You can also use this hook to set query parameters with the second setSearchParams
function.
With React Router v5
If you are still using a lower version of React Router than v6, then you will need to use the useLocation
hook in combination with the URLSearchParams API, similar to the first example:
import { useLocation } from 'react-router-dom'
const Component = () => {
const search = useLocation().search
const searchParams = new URLSearchParams(search)
return (
<div>Tutorial: {searchParams.get('tutorial')}</div>
)
}
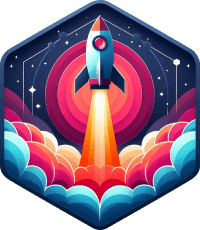
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: