Two Ways to Force Types in TypeScript
There are two ways to force types in TypeScript (also known as type casting or type conversion). Using the bracket syntax, or using the as
keyword. For example:
// Using bracket syntax
const age = <string>user.age
// Using the "as" keyword
const age = user.age as number
In the above code example, we are transforming one type into another, for example, a number to a string, or a string to a number. In both cases, the end result will be the same, there are no differences.
One thing to keep in mind is to stick to one or the other for consistency. In case you don't know the type upfront, it is also a common practice to use the any
type to avoid type errors:
// Using bracket syntax
const age = <any>user.age
// Using the "as" keyword
const age = user.age as any
Only use the any
type as the last resort, and if you are sure your application won't run into type errors.
Type Conversions in TypeScript
Of course, you can also go the other way around and convert types instead of forcing them. You can use the same type conversion method in TypeScript you would use in plain JavaScript. That is, for the above example, we can use the String
or Number
object to convert strings to numbers and vice versa.
// Converting a string to a number
const age: number = Number(user.age)
// Converting a number to a string
const age: string = String(user.age)
// Make sure you pass a valid numbe to Number
const age: number = Number('invalid') // This will result in NaN
Note that in case you pass an invalid number to the Number
object, you will get NaN
as a result. You can also convert types into booleans using the Boolean
object:
Boolean(1) // Returns true
Boolean('0') // Returns true
Boolean([]) // Returns true
Boolean(null) // Returns false
Boolean(undefined) // Returns false
Boolean(0) // Returns false
Boolean(NaN) // Returns false
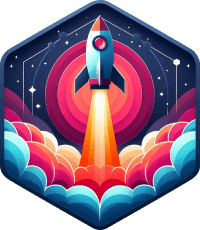
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies:
Courses

Understanding TypeScript

Mastering TypeScript
