How to Fix "Maximum call stack size exceeded" Errors in JS
The "Uncaught RangeError: Maximum call stack size exceeded" error occurs in JavaScript when you don't specify an exit condition for your recursive function. Take the following as an example:
const test = () => test()
// β This will throw "Uncaught RangeError: Maximum call stack size exceeded"
test()
This function will call itself indefinitely, causing the "Maximum call stack size exceeded" error. You may also come across this error in other forms, such as the below, but the cause will be the same.
"Uncaught InternalError: Too much recursion"
To fix the error, we will need to provide an exit condition. You can do this by wrapping the call inside a conditional statement. Take the following code as an example:
const countDown = num => {
if (num > 0) {
console.log(num)
countDown(num - 1)
}
}
This is also a recursive function that calls itself and will log out numbers until it reaches 0. Notice that we call the function with the "same argument - 1". This means that at a certain call, the condition will not be met, meaning we will stop executing the function. This way, we won't reach the maximum call stack size, as we have an exit condition.
Infinite loops
The "Maximum call stack size exceeded" error can not only happen with recursive functions, but with infinite loops as well. Most commonly, this can happen while using a while
loop.
A common way to ensure that your while loop has an exit condition is to decrement a value at each iteration. Take a look at the following example:
Once i
reaches 0, it will be evaluated as false
, meaning we will stop executing the while loop. In this case, this is our exit condition.
Make sure you always define an exit condition to avoid running into infinite loops.
Want to learn more about recursion in JavaScript? Check out the below tutorial.
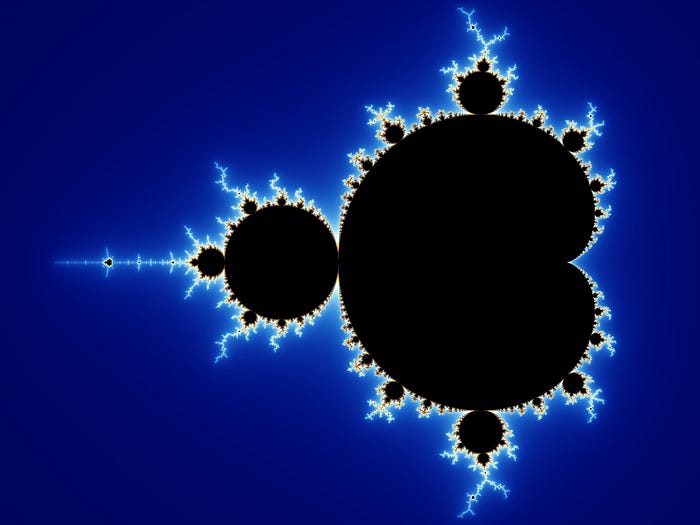
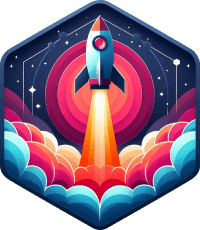
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: