How to Fix "Illegal return statement" Errors in JavaScript
The "Uncaught SyntaxError: Illegal return statement" error occurs, whenever JavaScript comes across a return
statement outside of a function. The return
keyword can only be used inside of functions. To resolve the error, make sure you don't use a return
statement outside of functions.
// β Don't use return outside of functions
return 'Hello World! π'
// βοΈ Do
const greet = () => {
return 'Hello World! π'
}
// βοΈ Or using a shorthand
const greet = () => 'Hello World! π'
Using the return
keyword inside block scope such as inside if
statements or for
loops are also not valid (if they are outside of a function).
// β Don't
if (user) {
return `Hello ${user}! π`
}
// β Don't
for (let i = 100; i > 0; i--) {
return i
}
In case you need to return a value from a loop conditionally, you need to introduce a variable outside of the loop, and then assign the value to the declared variable. And in case you need to break from a loop conditionally, then you can use the break
keyword.
// Returning a value conditionally from a loop
let value
for (let i = 0; i < 100; i++) {
if (conditionToBeMet) {
value = i
}
}
// now have the value of i
console.log(value)
// Breaking conditionally from a loop
for (let i = 100; i > 0; i--) {
if (i < 50) {
break
}
console.log(i)
}
In case you have nested loops and you want to only break from one of them, you can use a labeled statement, where you label your loop with a name, and pass the name after the break
keyword. This way, you can control which loop should be stopped.
outerLoop: {
for (let i = 0; i < 100; i++) {
innerLoop: {
for (let j = 0; j < 100; j++) {
break innerLoop
}
}
}
}
You may also come across a similar error message "Uncaught SyntaxError: Unexpected token 'return'". This error occurs whenever you have a syntax error in your application right above a return
statement. For example:
const greet = user => {
const message = 'Welcome ' + user +
return message
}
Here we have an unexpected plus sign after the string, which means JavaScript will not expect the return
keyword from the next line. In short:
Whenever you get an "Unexpected token 'return'" error in JavaScript, it means there's a syntax error (unclosed string, extra operator, missing keyword) right before your return
statement.
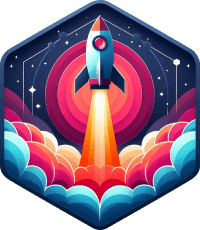
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: