How to Fix "Functions are not valid as React child" Errors
The "Functions are not valid as React child" error happens in React, whenever you try to return a function from your component, instead of calling the function. It may also happen if you return the reference to a component instead of calling it as a JSX tag.
const fn = () => { ... }
const Component = () => { ... }
// π΄ This will throw the above error
const App = () => {
return fn
}
// π’ Call the executed function instead
const App = () => {
return fn()
}
// π΄ This will throw the above error
const App = () => {
return Component
}
// π’ Call it as a component
const App = () => {
return <Component />
}
This happens because you return a reference to the function, instead of returning the return value of the function, which you can do by invoking it.
In the second example, we try to render a component, but we return the function instead. In this case, make sure you return it as a JSX tag, instead of referencing the function.
You may also come across the same issue when using React Router. The Route
component expects a component to be rendered for the element
prop. Make sure you pass a component for the prop, instead of passing a reference to the component.
// π΄ This will throw the above error
<Route path="/" element={Component} />
// π’ Pass the component instead
<Route path="/" element={<Component />} />
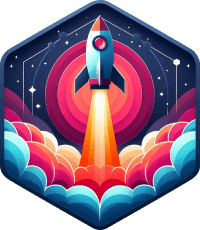
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: