Fix "cannot read properties of null (reading length)" in JS
The "Uncaught TypeError: Cannot read properties of null (reading 'length')" error occurs in JavaScript, whenever you try to use the length
property of an array (or string) on a variable that is null
.
This usually happens when you receive your data from a database, and you don't check whether the data exists in the first place. Take a look at the following example:
// Trying to fetch updates from a database that returns null
const updates = null
// β This will throw: Uncaught TypeError: Cannot read properties of null (reading 'length')
if (!updates.length) {
console.log('You are up to date! π')
}
// The above translates to:
if (!null.length) { ... }
We expect the updates
variable to be an array, but a null
is returned and so we get the above-mentioned error. This is because null
doesn't have a length
property. Try to run null.length
in your console and you will get the same error.
How to Fix the Error?
In order to fix the error, we need to make sure that the array is not null
. In order to do this, the simplest way is to use optional chaining.
// βοΈ Even if updates is null, this will work
if (!updates?.length) {
console.log('You are up to date! π')
}
// The above translates to:
if (!null?.length) { ... }
You can use optional chaining by introducing a question mark after the variable. This ensures that the property will only be read if the variable indeed has a value.
We can also use a logical OR, or use the built-in Array.isArray
method to check prior to using the length
property to prevent running into issues.
// Using logical OR
const updates = null || []
// Using Array.isArray
if (Array.isArray(updates) && !updates.length) { ... }
In the first example, we fallback to an empty array, if the value retrieved, is null
. This ensures that we will always be working with an array.
The second example uses Array.isArray
to first check if we are working with an array. If this part evaluates to false
, we won't check the length
property, therefore no error will be thrown. We can also use logical OR in place where we want to work with the length
property:
if (!(updates || []).length) { ... }
Working With Strings
Strings also have a length
property. The same rules apply to them. Prefer using optional chaining, or a logical OR to fallback to default values. The only difference here, is you want to provide an empty string as a fallback value:
// Using optional chaining
const string = null
if (!string?.length) { ... }
// Using logical OR
const string = null || ''
// Using the typeof operator to
if (typeof string === 'string') {
const length = string.length
}
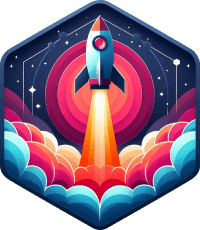
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: