Extending Types in TypeScript The Right Way
Extending types in TypeScript can be done by using the &
operator. This is called intersection types, which can be used for combining two or more different types together.
// βοΈ Using different types
type Fruit = {
sweet: boolean
}
type Vegetable = {
berry: boolean
}
type Tomato = Fruit & Vegetable
// βοΈ Using interfaces
interface Fruit {
sweet: boolean
}
interface Vegetable {
berry: boolean
}
type Tomato = Fruit & Vegetable;
// β This is not valid
interface Tomato = Fruit & Vegetable
The Tomato
type will now contain both fields from the other two types. You can combine as many types together this way as needed. This syntax can also be used with interfaces. However, if you want to define your type as an interface, then you will need to use the extends
keyword.
type Fruit = {
sweet: boolean
}
interface Vegetable {
berry: boolean
}
interface Tomato extends Fruit, Vegetable {
}
Notice that for extending multiple interfaces, you can comma-separate them instead of using the &
operator. You also have the option to extend interfaces with types using the extends
keyword, not just interfaces, or you can even combine both of them. However, for following best practices and keeping things consistent, it is recommended to stick to one or the other.
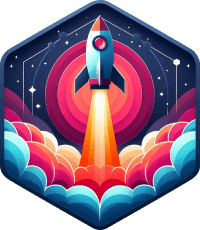
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies:
Courses

Understanding TypeScript

Mastering TypeScript
