How to Extend Multiple Classes in TypeScript With Mixins
You cannot extend multiple classes at once using the extends
keyword in TypeScript. However, you can use a mixin β a special function that can be used for extending multiple classes.
// This will throw an error: "Classes can only extend a single class."
class Tomato extends Vegetable, Fruit { ... }
Whenever you try to extend a class with multiple other classes, you will get the following error: "Classes can only extend a single class.". To resolve this, create the following function in your codebase that you can use for extending multiple classes:
const applyMixins = (baseClass: any, extendedClasses: any[]) => {
extendedClasses.forEach(extendedClass => {
Object.getOwnPropertyNames(extendedClass.prototype).forEach(name => {
Object.defineProperty(
baseClass.prototype,
name,
Object.getOwnPropertyDescriptor(extendedClass.prototype, name) || Object.create(null)
)
})
})
}
The way this function works is it expects a class that needs to be extended with other classes, and an array of classes where the extension should come from. It loops through each class and copies the properties and methods over to the base class.
This way, you will have the extended properties and methods available for you to use on your base class. You can call this function in the following way:
class Enemy {
attack() { ... }
}
class Boss {
ultimateAttack() { ... }
}
class Entity implements Enemy, Boss {
spawn() { ... }
}
applyMixins(Entity, [Enemy, Boss])
const boss = new Entity()
boss.spawn()
boss.attack()
boss.ultimateAttack()
Call the function with the class that needs to be extended, and pass the classes as an array that you want to extend from. Now you are able to call both methods from both classes on the extended class.
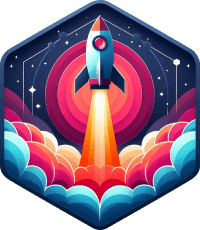
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies:
Courses

Understanding TypeScript

Mastering TypeScript
