4 Ways to Convert Strings to Booleans in TypeScript
To convert a string to a boolean in TypeScript, the easiest way is to wrap your value in the built-in JSON.parse
function. This function will convert the string into a boolean and return either true
or false
, depending on the input.
const booleanTrue: boolean = JSON.parse('true') // Returns true
const booleanFalse: boolean = JSON.parse('false') // Returns false
Alternatively, you can create a helper function to manually return true
or false
, based on the provided argument.
const booleanify = (value: string): boolean => {
const truthy: string[] = [
'true',
'True',
'1'
]
return truthy.includes(value)
}
booleanify('true') // Returns true
booleanify('True') // Returns true
booleanify('1') // Returns true
booleanify('false') // Returns false
booleanify('0') // Returns false
Here, we can utilize the includes
method on an array of strings. This way, we can handle capitalized strings or evaluate "1" as true
. Anything that is included in the array will return true
, while everything else will return false
.
There are, of course, many different ways to achieve the same result. In case you prefer a different solution, here are three other ways to convert strings to booleans in TypeScript, listed in order of recommendation.
Using Strict Equality
Another approach is to use the strict equality operator to check if your string values are equal to either "true" or "false" and create a boolean from that. Note that you might want to convert your string to lowercase first, in case you receive the text capitalized; otherwise, you may end up with an incorrect value.
const stringTrue: string = 'true'
const stringCapitalizted: string = 'True'
const booleanTrue: boolean = stringTrue === 'true' // Returns true
const booleanFalse: boolean = stringCapitalizted === 'true' // Returns false
const booleanLowercase: boolean = stringCapitalizted.toLowerCase() === 'true' // Returns true
Strict equality checks both the type and value of the variable, whereas the double equal operator only checks the value. Therefore, you always want to use strict equality in your code to avoid false positives.
// β Returns true, as only the value is checked
'1' == 1
// βοΈ Returns false, as both value and type are checked
'1' === 1
Using the Boolean Object
There are two more ways to convert strings to booleans, however, due to their nature, they will produce false positives. One method is to wrap your value in a Boolean
object, which converts the value into a boolean and returns true
or false
if an empty string is provided.
const booleanTrue: boolean = Boolean('true') // Returns true
const booleanFalse: boolean = Boolean('false') // Returns true
const booleanOne: boolean = Boolean('1') // Returns true
const booleanZero: boolean = Boolean('0') // Returns true
const booleanSpace: boolean = Boolean(' ') // Returns true
const booleanEmpty: boolean = Boolean('') // Returns false
Note that all non-empty strings will return true
, and only empty strings will return false
in this case, as all strings with a length are considered truthy values.

Using Double Negation
Lastly, another way to convert strings to booleans is to use double negation. Similar to the Boolean object, you'll get the same behavior where all strings will be true
, regardless of their values, except for empty strings.
const booleanTrue: boolean = !!'true' // Returns true
const booleanFalse: boolean = !!'false' // Returns true
const booleanOne: boolean = !!'1' // Returns true
const booleanZero: boolean = !!'0' // Returns true
const booleanSpace: boolean = !!' ' // Returns true
const booleanEmpty: boolean = !!'' // Returns false
Both the Boolean
object and double negation can be used to convert any value into a boolean, not just strings.
Conclusion
In conclusion, there are various correct ways to convert strings to booleans in TypeScript. So which one should you use? It depends on your use case, but for the most part, prefer using the strict operator. Use the other approaches according to the following:
booleanify
: This function is reliable as you can specify which values to treat astrue
and which values to treat asfalse
.- Working with
true
andfalse
strings: When you only need to handletrue
andfalse
as strings, useJSON.parse
. - Using Boolean and double negation: Only use the
Boolean
object and double negation if you don't need to work withtrue
andfalse
as strings. Among all the options, these ones are the least reliable when it comes to handling string-based boolean values.
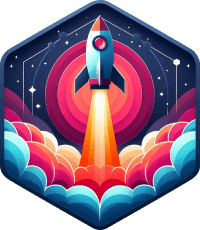
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies:
Courses

Understanding TypeScript

Mastering TypeScript
