3 Ways to Conditionally Apply Classes in React
When working with React components, you will often need to work with conditional classes. In this tutorial, I will show you how to approach the problem in three different ways: using libraries, custom utility functions, or inline arrays.
Using classNames
The most common way to apply class names conditionally in React is by using the classnames
library. This library was created specifically for conditionally joining class names together:
// Run `npm i classnames` in your terminal to install the package
import classNames from 'classnames'
// Using strings
<div className={classNames(
'relative mb-4',
!isVisible && 'hidden'
)} />
// Using arrays
<div className={classNames([
'relative mb-4',
!isVisible && 'hidden'
])} />
// Using objects
<div className={classNames(
'relative mb-4',
{ hidden: !isVisible }
)} />
You can also try the clsx
library, which is a smaller alternative to classnames
.
When working with objects, notice that the key represents the class name, while the property is used for evaluating the condition. In the above example, a .hidden
class will be applied if !isVisible
evaluates to true
.
Using a Custom Utility Function
If you are more concerned about the performance and the footprint of your NPM packages, you can also create the following custom utility function inside your project:
const classNames = array => array?.filter(Boolean).join(' ')
// Using in JSX
<div className={classNames([
'relative mb-4',
!isVisible && 'hidden'
])} />
This works exactly like classnames
or clsx
, but without an additional dependency. The only difference is that it only accepts an array and uses filter(Boolean)
to filter out all falsy values, then joins it back together into a single string using join(' ')
.

Using Inline Arrays
Last but not least, for very simple solutions, we can also use a simple logical AND or ternary with an inline array in the following ways:
<div className={[
'relative mb-4',
hidden && !isVisible
].join(' ')} />
// Using an outsource variable
const containerClass = ['relative mb-4', !isVisible && 'hidden'].join(' ')
// Using with ternary
const containerClass = ['relative mb-4', !isVisible ? 'hidden' : ''].join(' ')
<div className={containerClass} />
A ternary can also be used when different types of classes are needed depending on the condition. For example, the following code switches between two classes depending on the value of the isActive
state:
<div className={isActive ? 'hamburger-close' : 'hamburger-open'} />
Which Solution to Use?
To help you decide on which approach you should take, you can use the following flow:
# Generally speaking, a library will be suitable for most situations
libary -> custom function -> inline array
- Using libraries: Use for most cases. If you are concerned about size but still want to use a flexible third-party package, go with
clsx
. - Using custom utility functions: Use this approach if you are concerned about the JavaScript performance of your application, and you want to keep both the number of dependencies and your JavaScript code to a bare minimum.
- Using inline arrays: Only use as a last resort for simple classes or if your codebase contains only a handful of conditional classes.
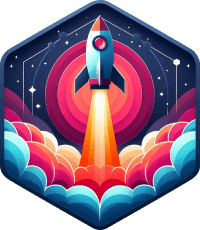
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: