How to Create Associative Arrays in JavaScript
Associative arrays are a collection of key-value pairs, a type of array where named keys are used in place of numbered indexes. Unlike other programming languages, JavaScript does not have associative arrays. Instead, objects can be used.
Note that objects are denoted by curly braces, and named keys are used instead of indexes. Each key with its value is called a property. To add new properties (entries) to the object, you can use either a dot or bracket notation.
// Using dot notation
user.role = 'admin'
// Using bracket notation
user['role'] = 'admin'
In general, you want to use the dot notation. When you are working with variables and need dynamic names for your object properties, that's when the bracket notation can come in handy.
const property = 'role'
// The user object will now have a "role" property
user[property] = 'admin'
Make sure you follow the same naming rules for object properties that you would follow for variables. For example, you cannot start the name of a property with a number.
Traversing with Objects
As objects work quite differently compared to arrays, it means you cannot traverse through objects in the same way you would do with an array.
To loop through an object, you can use a combination of Object.keys
, and a forEach
loop in the following way:
// This will log out
// - name John
// - age 30
// - job Developer
Object.keys(user).forEach(key => console.log(key, user[key]))
Object.keys
return an array of strings, containing the named keys of the object. We can also use this to get the length of an object if needed.
// Returns the length of the object based on the number of keys
Object.keys(user).length
We can also get only the values using Object.values
, and we can also convert the key-value pairs into arrays using Object.entries
.
// Returns ['name', 'age', 'job']
Object.keys(user)
// Returns ['John', 30, 'Developer']
Object.values(user)
// Returns [['name', 'John'], ['age', 30], ['job', 'Developer']]
Object.entries(user)
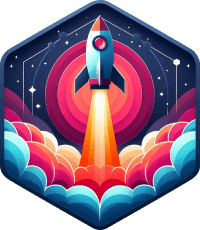
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: