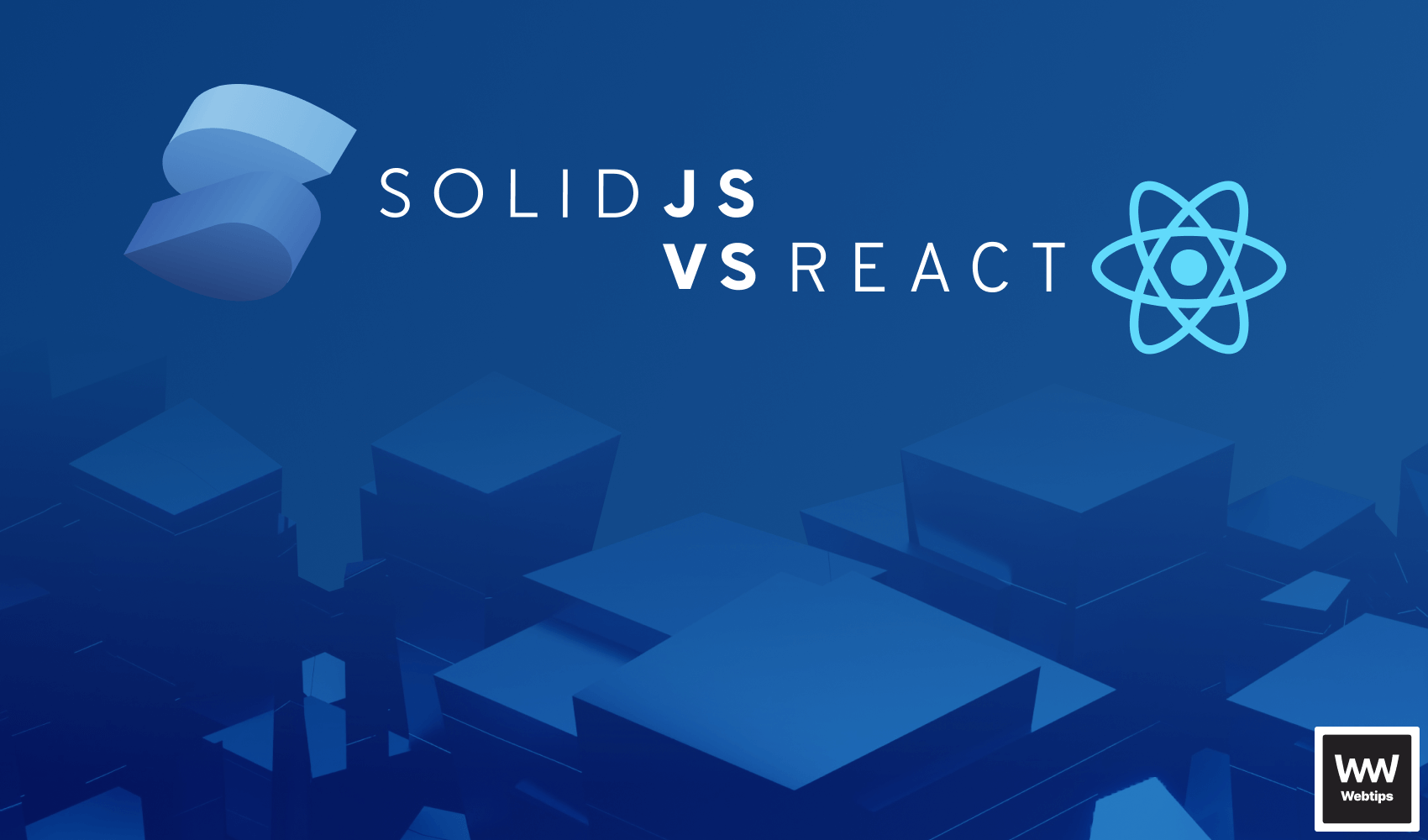
SolidJS vs React: Should You Make the Switch?
SolidJS is an open source, reactive declarative JavaScript library with an API similar to React. It was created by Ryan Carniato in 2018 and reached the stable v1.0 version in 2021. In SolidJS, components are JavaScript functions that return JSX just like in React.
// This is a valid component in both React and Solid
const App = () => <h1>π</h1>
Unlike React, SolidJS doesn't use a virtual DOM. Instead, templates are compiled down to real DOM nodes and updated with reactions. When state changes, only the code that depends on it will rerun.
How to Setup a New Solid Project
To get started with SolidJS, run the following commands inside your terminal to bootstrap a new project.
# For using JavaScript
- npx degit solidjs/templates/js solid-app
# For using TypeScript
- npx degit solidjs/templates/ts solid-app
- npm i
- npm run dev
In case you want to start working with SolidJS from absolute zero or in an existing project, you need to install the following dependencies to your project:
npm i solid-js babel-preset-solid
Then, you will need to set up Solid as a preset in your babelrc
config file, which you can do so by adding it to your presets array.
"presets": ["solid"]
In case you also want to work with TypeScript, you will need to extend your compilerOptions
in your tsconfig
file to support Solid's JSX syntax.
"compilerOptions": {
"jsx": "preserve",
"jsxImportSource": "solid-js"
}
Working with SolidJS
To get a basic overview of how Solid works, let's create the same starter app in both React and SolidJS. They share many similarities, the main differences are how hooks are handled in SolidJS.
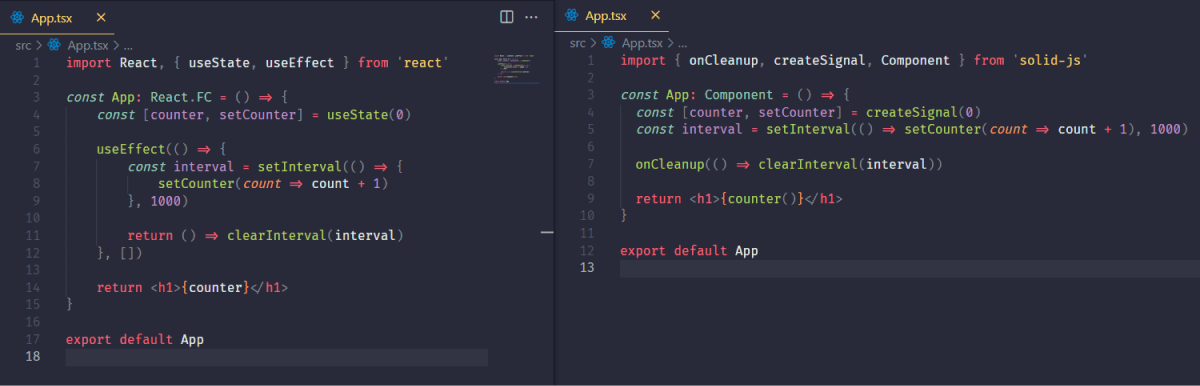
While React hooks use the "use" prefix, SolidJS uses the "create" prefix. In place of useState
, we have createSignal
, and in place of useEffect
, we have createEffect
. But what you will notice, is that in the above example, we don't have a createEffect
call.
In React, setInterval
triggers a state change, which causes the component to rerender. This is why we need to call setInterval calls inside useEffect hooks.
This is because unlike in React, the Solid component is only called once, so there's no need to use a createEffect
hook. In case you need to create side effects or run code when your data changes, you can use the createEffect
hook.
const [counter, setCounter] = createSignal(0)
// The equivalent of useEffect in Solid
createEffect(() => {
console.log(counter())
})
Notice that in SolidJS, you don't need a dependency array for a createEffect
call. This is because the compiler automatically detects any dependencies referenced inside the call.
SolidJS also comes with a built-in createMemo
function that can be used to memoize computation-heavy function calls.
There are two other main differences that we need to point out when it comes to SolidJS vs React. Unlike in React, Solid uses the class
property to set class names for DOM elements. It also supports a classList
property which can be used to conditionally set class names.
<div classList={{ active: isCurrent }}>Setting class names conditionall</button>
Here, an active
class will be added to the div in case the isCurrent
variable is evaluated to true
. Therefore, the property accepts an object where the key is the class name to be set, and the value is a boolean.
The other main difference is that unlike in React, when you assign a JSX expression to a variable, you actually get back a DOM element, instead of an abstraction.
const dom = <div>π</div>
// This will return an actual div as a DOM element, as oppose to a function call
console.log(dom)
It's also worth noting that Solid supports the use of Fragments. Similar to React, you can use an empty tag to define a fragment.
<>
<h1>Title</h1>
<h2>Subtitle</h2>
<>
Conditional Rendering
SolidJS also comes with a couple of handful built-in components for handling control flows. When it comes to conditional rendering, there is a built-in Show
component you can use to tell Solid when to render a component, and even what to render as a fallback if the condition is false.
import { Show } from 'solid-js'
<Show when={isLoggedIn} fallback={<h1>Welcome guest</h1>}>
<h1>Welcome {user}</h1>
</Show>
When you need to handle multiple conditions at once, there is also a built-in Switch
component that we can use just like a switch statement.
import { Switch, Match } from 'solid-js'
<Switch fallback={<Dashboard />}>
<Match when={route === "recipes"}>
<Recipes />
</Match>
<Match when={route === "ingredients"}>
<Ingredients />
</Match>
</Switch>
Loops
It also has a built-in For
component for handling loops over a collection of items. Notice that unlike in React, you don't need a special key
prop for keeping track of elements. Just like Show
, it also accepts a fallback content in case there is nothing to render.
import { For } from 'solid-js'
<For each={item} fallback={<div>Nothing to render π€·</div>}>
{(item, index) => <div>#{index} {item}</div>}
</For>
Lifecycle
SolidJS also comes with two lifecycle methods called onMount
, and onCleanup
that are executed at the beginning and end of a component's lifecycle respectively.
onMount(() => { ... })
onCleanup(() => { ... })
There is also a third, onError
method that we can use to register an error handler that executes when an error occurs in one of the child components.

Performance of SolidJS vs React
On its homepage, solid claims to be the fastest among many JavaScript libraries due to its small footprint. But how does it compare to React in terms of performance?
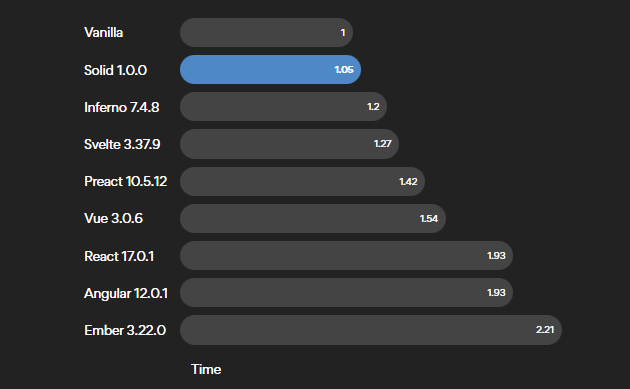
In order to find out, I build both starter apps to benchmark their initial load, as well as their bundle sizes. Here are the results:
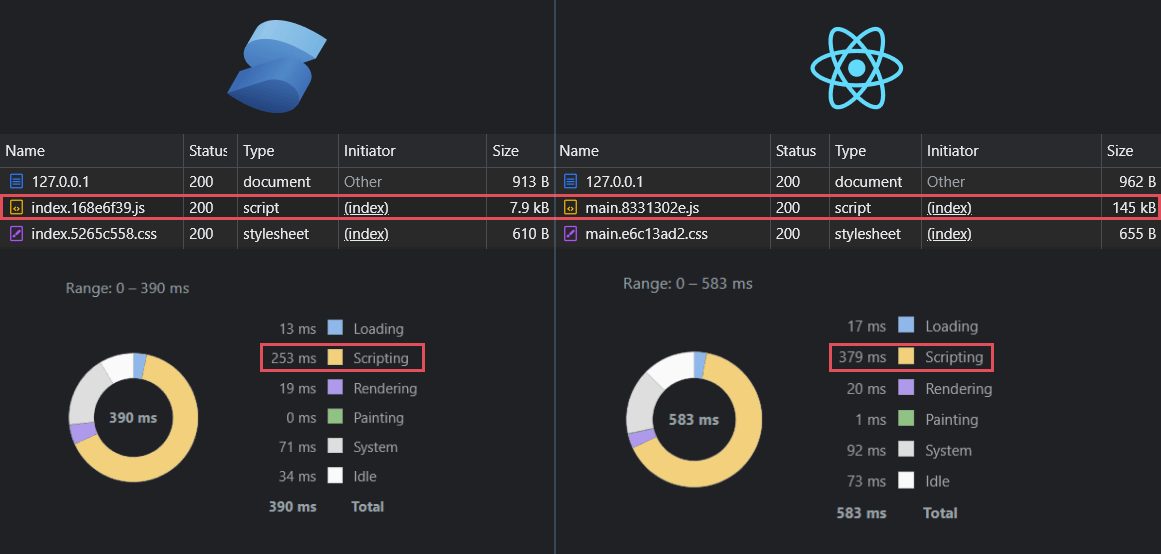
Highlighted in red, there is a huge difference in bundle size, mainly due to the fact that the library itself is small (almost 95% smaller in this simple example). It's important to mention, however, that as an app grows, this difference likely will be smaller.
Scripting performance also shows a ~50% difference where solid performs better than React. So what does this mean? Should you make the switch from React to SolidJS? Is SolidJS better than React?
Conclusion
There are many advantages of SolidJS compared to React. As mentioned above, it has a much smaller footprint due to the overall size of the library and has some built-in features which make it more beginner-friendly, such as its built-in Show
or For
/Switch
components.
However, there's also a downside to SolidJS. It is still relatively new. React has been around for almost a decade, and it has an enormous community behind it. It has been starred around 200K times on GitHub, compared to SolidJS with 20K stars at the writing of this article.
This also means whatever issue you are facing with React, you will likely find the answer. There are also many job openings looking for specifically React developers, which is not true for SolidJS.
When it comes to production builds, you may not want to switch over to Solid just yet, but it is nonetheless a great library for other projects and can outperform React in many areas.
If you would like to play around with SolidJS right inside your browser, I highly recommend giving a visit to its online playground to get a look and feel. Do you already have experience with SolidJS? Let us know your thoughts on it in the comments below! Thank you for reading through, happy coding!
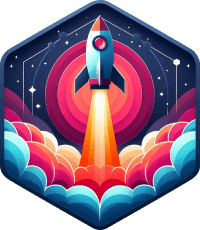
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: