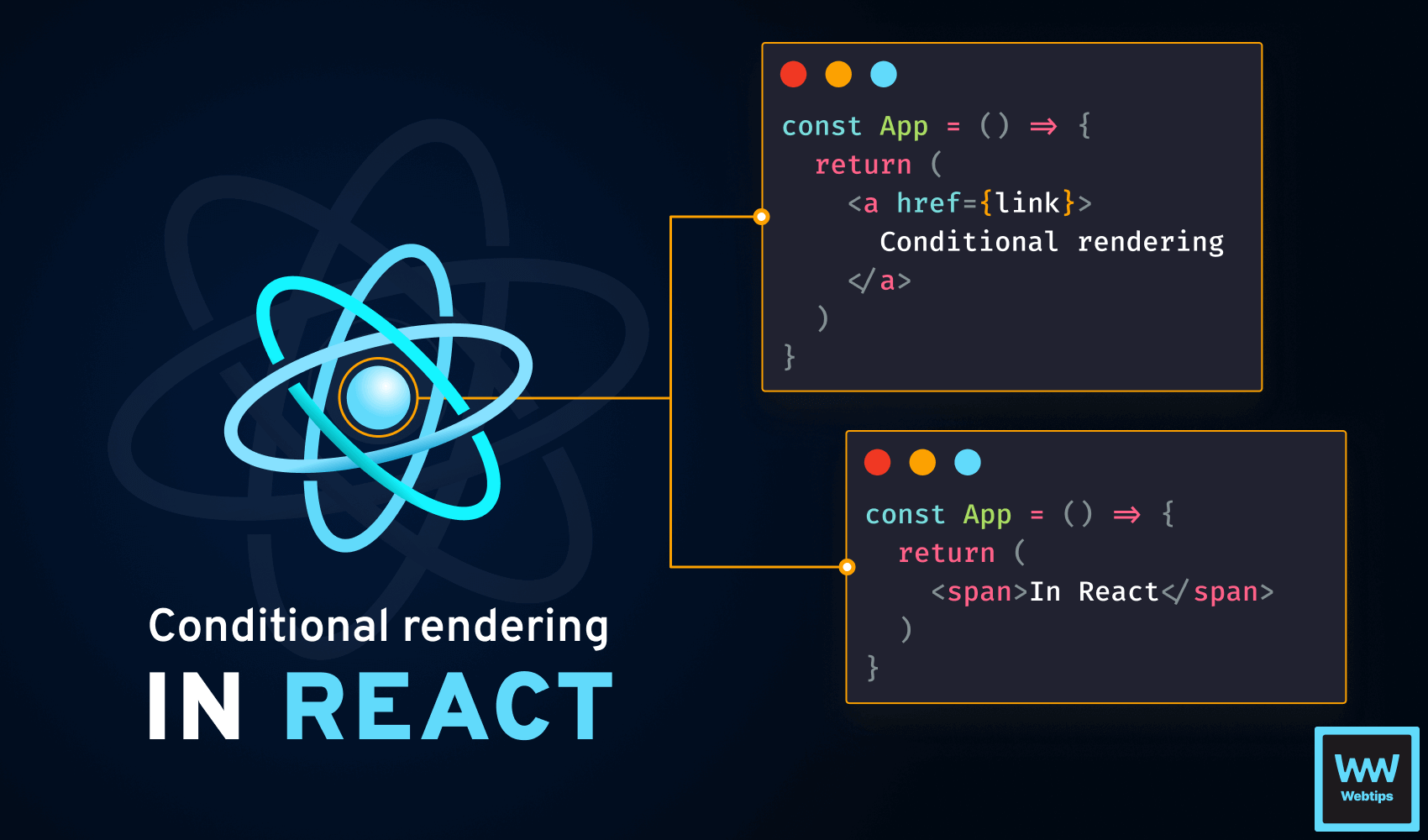
How to Render Anchors Without Href in React
When working with anchors in React, you may find yourself in a situation where you need to render an anchor without a link if data is missing.
However, anchors are used for hyperlinks. Therefore, to keep your document accessible and semantically correct, you want to render a different element in place of the anchor if no link is present.
Conditionally Rendering Anchors
This can be achieved in React with conditional rendering. Conditional rendering is the act of rendering elements on the page based on different conditions. The following example will render an a
element if the link
is present:
const App = () => {
const link = ''
return link
? <a href={link}>Article title</a>
: <span>Article title</span>;
}
In case the link
is missing, the component will render a span
that will display the same text but without a hyperlink associated with it.
Another common way to conditionally render an anchor without href
in React is using a custom component that renders a link or a different element based on the presence of a prop, such as href
. Take the following example:
const Link = ({ href, children }) => href
? <a href={href}>{children}</a>
: <span>{children}</span>
const App = () => {
const link = ''
return <Link href={link}>Article title</Link>
}
Here, the Link
component expects an optional href
prop, and based on its presence, it will render an anchor with the passed href
, or a span
if the href
is missing. We can take this example one step further and create a higher-order component that can conditionally wrap other elements as well.
Anchors without href
are not focusable and will not be part of the tabbing order.
Rendering Anchors with HOC
Higher-order components (HOC for short), are a pattern used for reusing component logic. A common example of a HOC in React is a conditional wrapper that wraps elements conditionally. Take the following example:
const ConditionalWrapper = ({ condition, wrapper, children }) => {
return condition ? wrapper(children) : children
}
const App = () => {
const link = ''
return (
<ConditionalWrapper
condition={link}
wrapper={children => (
<a href={link}>{children}</a>
)}
>
<span>Article title</span>
</ConditionalWrapper>
)
}
In this example, if the link
is present, the span
inside the ConditionalWrapper
component will be wrapped with an anchor. We can define any wrapper for this component using the wrapper
prop. The wrapper
prop must always return a JSX element that should be used for wrapping.
In our case, it wraps the span
inside of an anchor. Note that unlike with conditional rendering, in this case, the span
will be present at all times.
// Output with conditional rendering:
// With link:
<a href="...">Article title</a>
// Without link:
<span>Article title</span>
// ----------------------------------
// Output with HOC
// With link:
<a href="...">
<span>Article title</span>
</a>
// Without link:
<span>Article title</span>

Summary
In summary, avoid rendering a
tags with href
. Anchors without links in the document are not focusable and will not be in the tabbing order. Instead, try to use conditional rendering or a higher-order component. You can decide which one to use based on the following points:
- If you are concerned about DOM size, go with conditional rendering.
- If you need to wrap multiple elements with different components and you need reusability, go with a HOC.
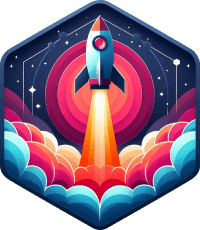
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: