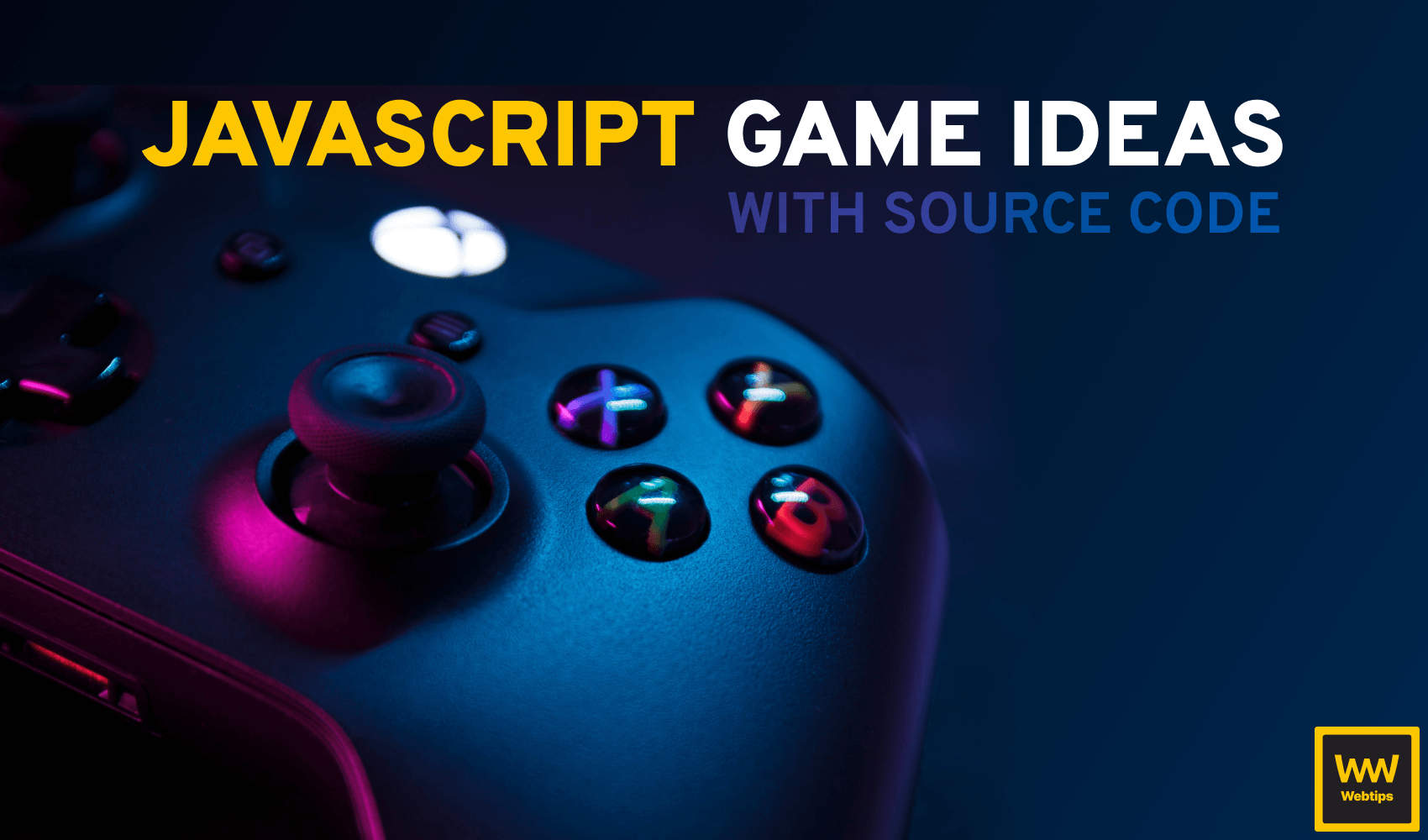
12 JavaScript Game Ideas with Source Code
If you're into game development and you already have some basic JavaScript knowledge, then you're at the right place. This article collects some JavaScript game ideas with tutorials and source code included.
The following tutorials will help you learn more about building games in JavaScript, and give you some ideas on what project to take on next. Let's start with the simpler, easier games to make, the ones that can be created entirely with vanilla JavaScript, then we'll move on to more advanced stuff.
Vanilla JavaScript Game Ideas
The following game ideas can all be recreated using only vanilla JavaScript, but later on, we'll also look at more complex ideas for which we can use a game framework. We'll leave a link to the tutorial for every game idea, and you'll also find links to the GitHub projects there.
Create a Hangman
Building a hangman is a fun project to take on, and we can learn a lot along the way. Working with game logic, randomization, and switching between game assets. This tutorial is available on Webtips as part of a preview lesson from our JavaScript course.
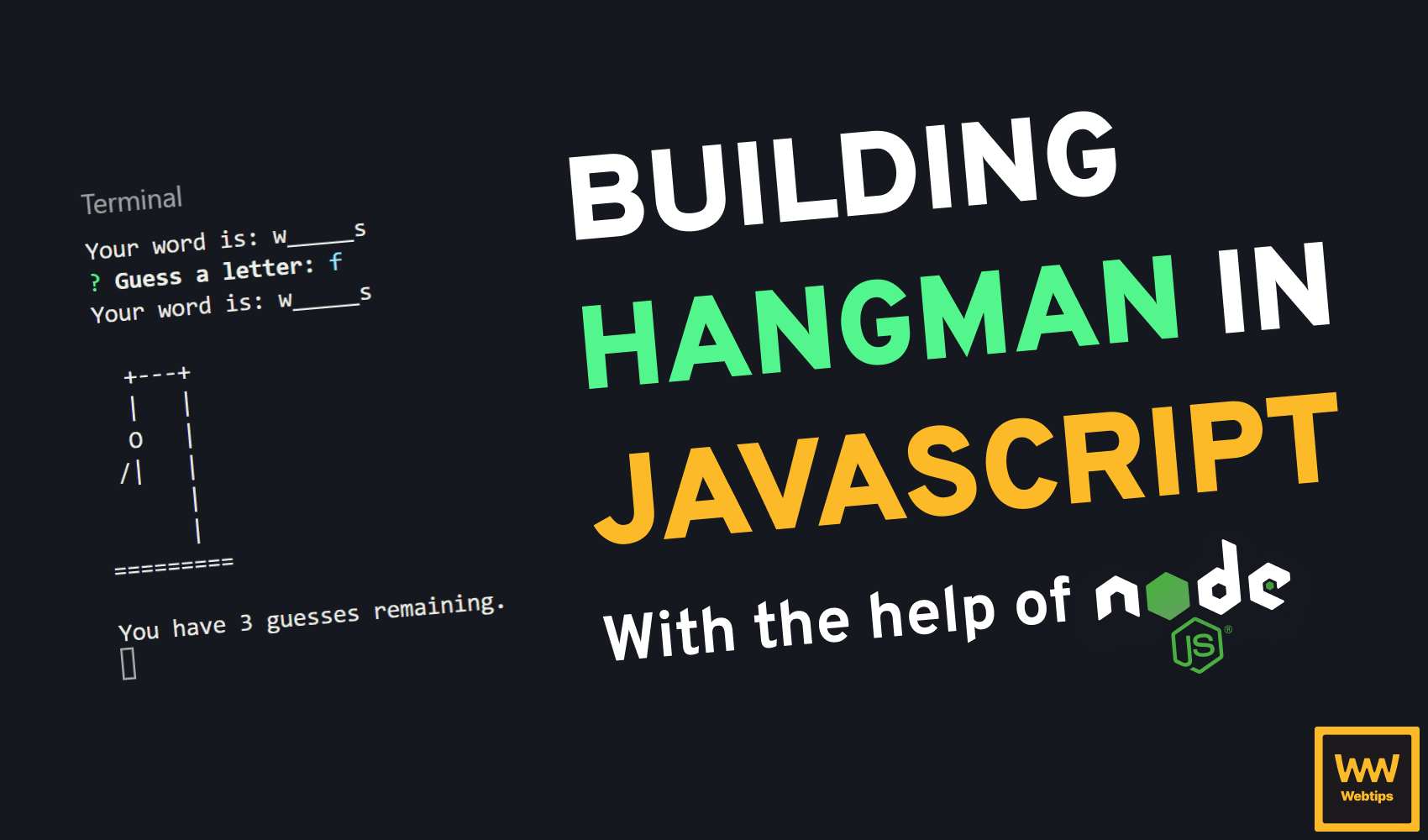
π You'll learn:
Working in Node
CJS vs ESM format
Regular expressions
Game logic flow
Using Inquirer
Handling terminal input and output
Create a Memory Game
One of our recent tutorials covers how to create a memory game to improve your memory and your JavaScript skills. You'll learn about how to flip cards, and how to interact with them. We'll also see how to work with timers and game states.
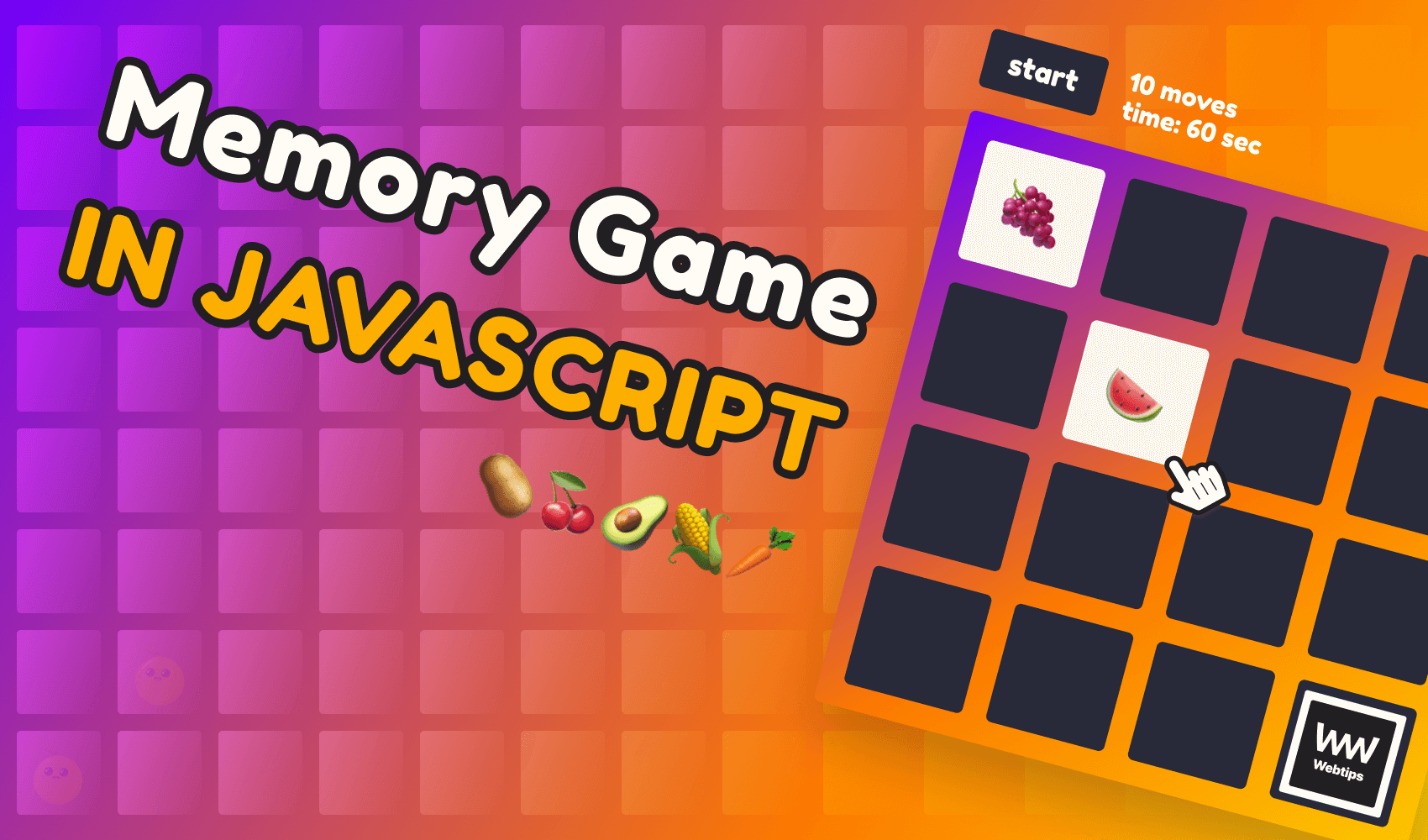
π You'll learn:
Working with DOM
Event listeners
CSS animations and transforms
Shuffling algorithms and randomization
setTimeout
andsetInterval
Handling game states
Create a Tic-Tac-Toe Game
Next, we have the classic tic-tac-toe game. In this tutorial, you can learn more about grids, event listeners, and working with a game state. Here you'll see how to create turn-based games. The entire logic for the game will fit into less than 100 lines of code.
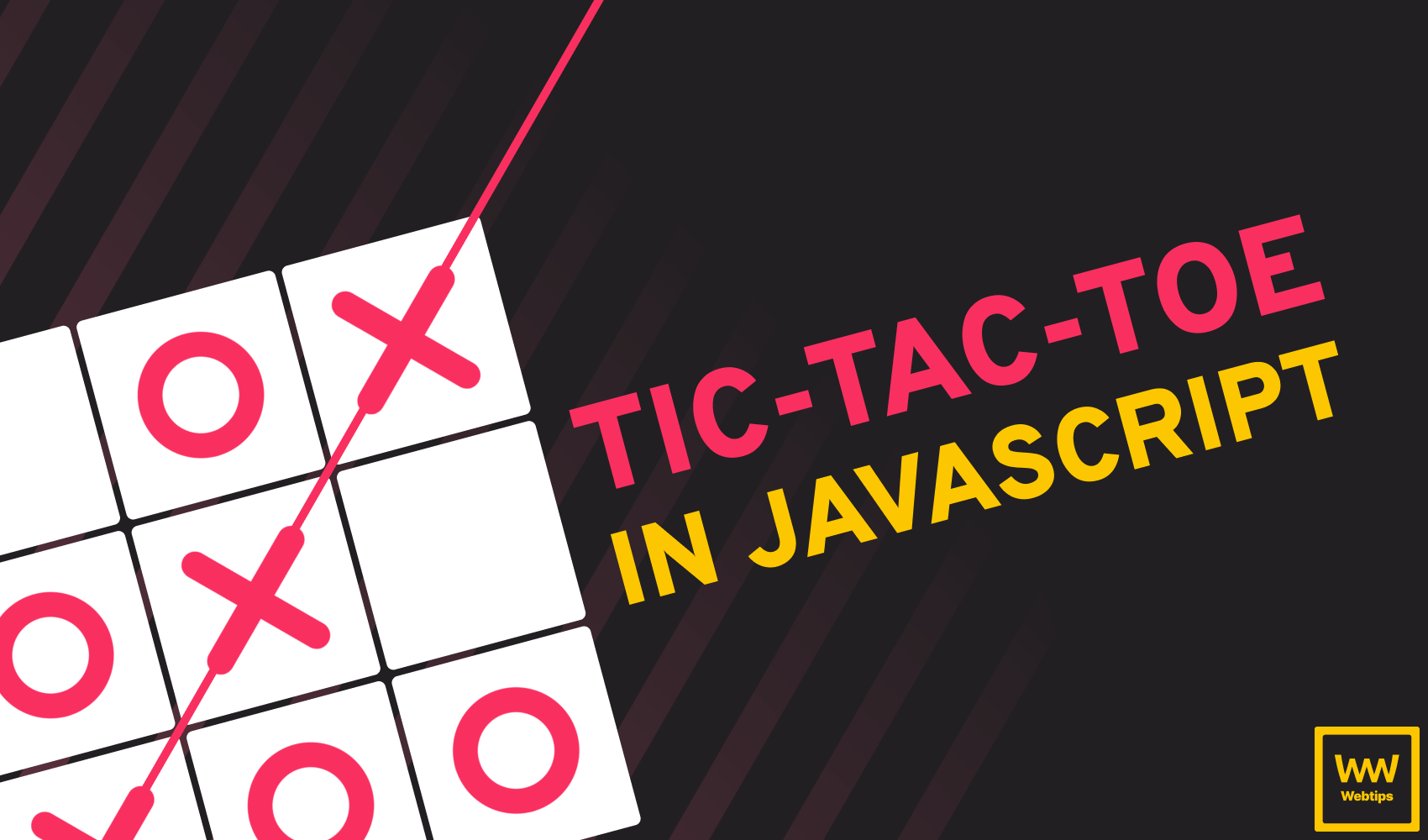
π You'll learn:
DOM manipulation
Array methods
CSS Grid
Pseudo-selectors and elements
State handling
Restart functionality
Create a Snake Game from Checkboxes
Want to look into creating a snake game? This is made entirely with checkboxes and a radio button. In this project, you'll learn a lot about working with arrays and manipulating DOM elements. We'll also look into how to create a scoring system.
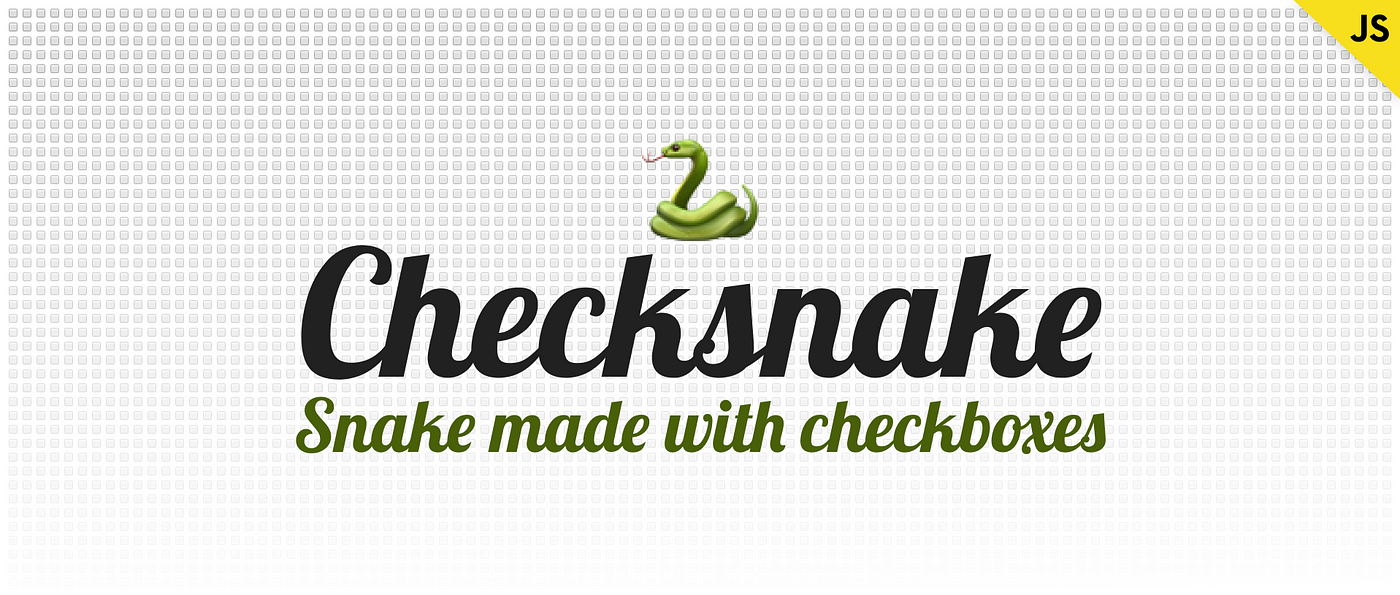
π You'll learn:
World generation
Keyboard input
Character movement
Working with configurations
Randomization
Scoring system
CSS animations
Game over state
Create Particle Effects
Want to create particle effects for a game? For this, you can use the canvas element. This tutorial is not a game on its own, but it teaches you a fundamental part of game development, how to create particle effects. This project will tackle this from the point of fireworks.
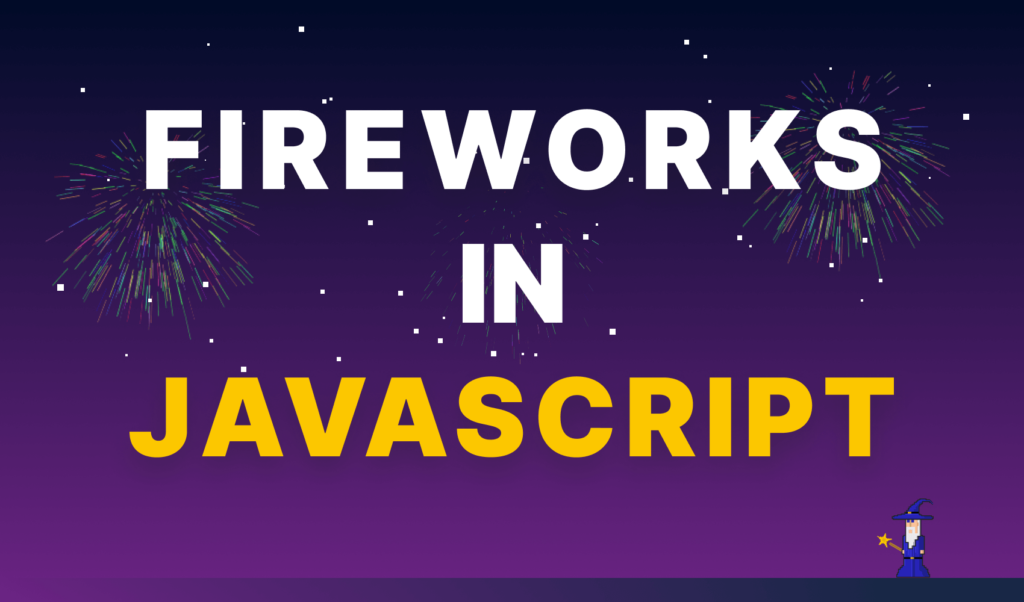
π You'll learn:
Working with canvas
Particle effects
Canvas mouse actions
Canvas gradients
JavaScript animations
Calculating distance in JavaScript
Create a Rock, Paper, Scissors Game
The following game ideas can be found on Youtube, as we have yet to create tutorials for these games on Webtips. The first one is rock, paper scissors, created by WebDevSimplied that you can play against your computer.
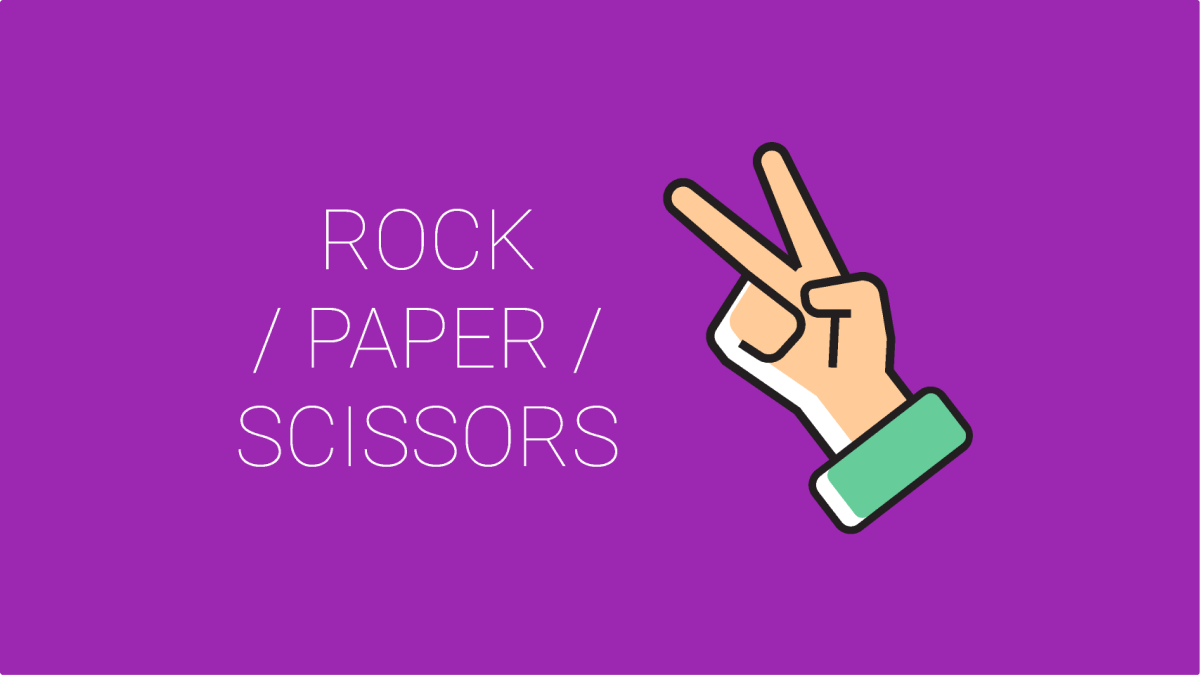
π You'll learn:
How to simplify complex game logic
CSS Grid and Flexbox
Randomization
Create a Quiz Game
Also created by the same channel, is a quiz game that teaches you what steps you need to take when building out JavaScript games, or any other project for that matter. It teaches you how to create a quiz game that you can later easily extend and customize.
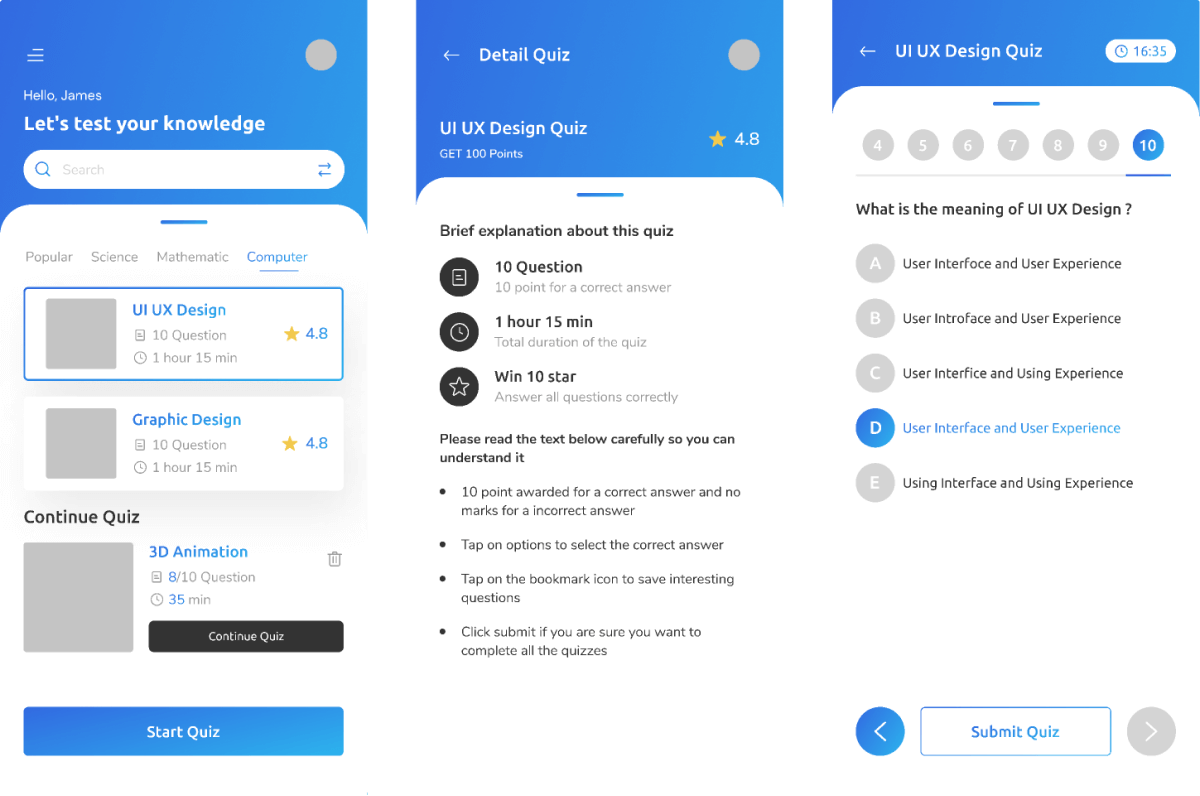
π You'll learn:
Dynamic display
Working with child elements
Array randomization
CSS variables
Create a Minesweeper
Looking for an even more complex game idea? Minesweeper it's then. Created by The Coding Train who is all about coding challenges, in this tutorial, you'll learn how to work with cells, loops, arrays, shapes, and there will be some math involved too.
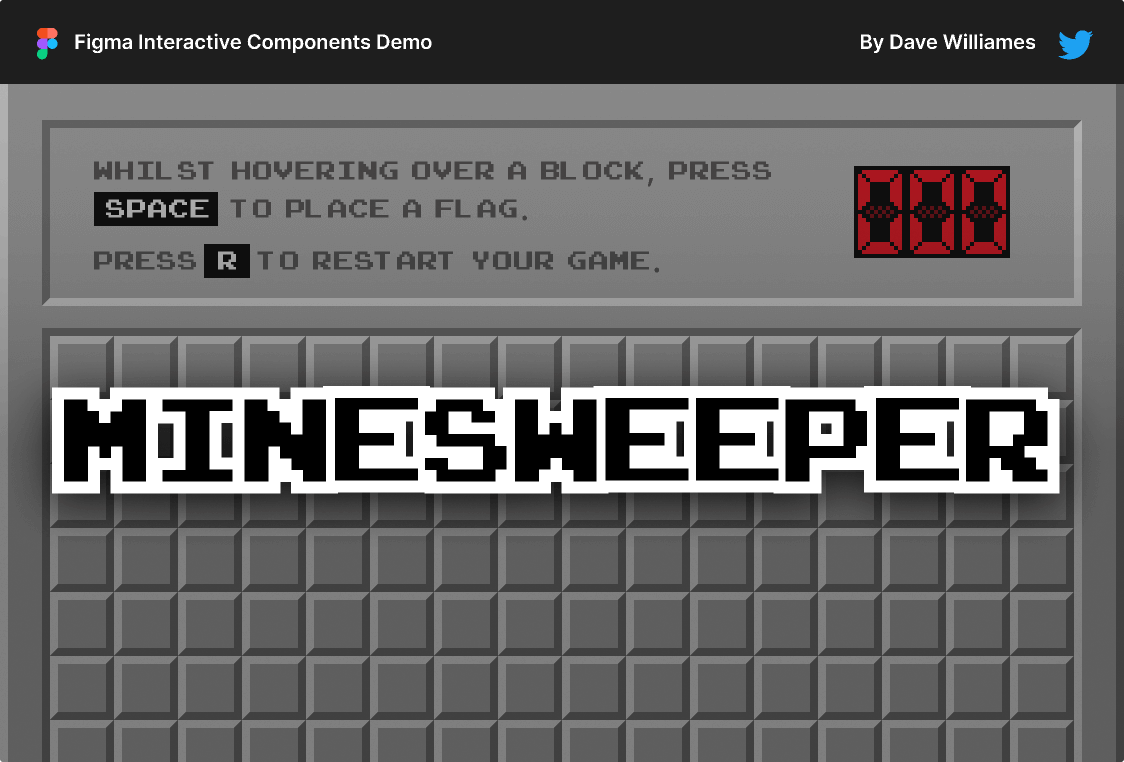
π You'll learn:
Working with grids
Nested loops
Detecting neighboring cells
Flood fill algorithm
Create a Word Scramble
For the last vanilla JavaScript game idea, you can only find the source code on Codepen. This project takes on the challenge to create a word scramble game. Work with multiple difficulty levels, randomization, and event listeners for interaction.
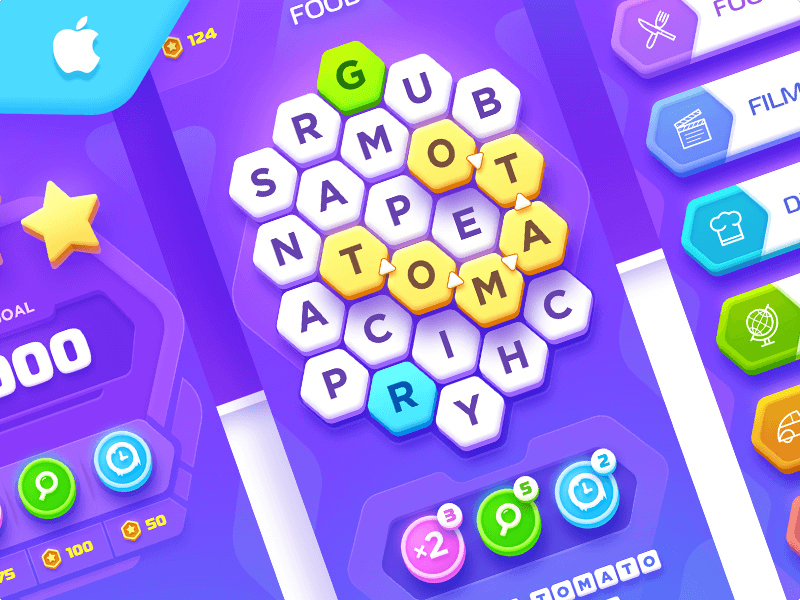
π You'll learn:
Leveling system
Reset functionality
DOM manipulation
Working with random values
Phaser Game Ideas
Now let's move to more advanced concepts. For the following 3 game ideas, we're going to use Phaser, a JavaScript Game Framework to rebuild classic games, such as Atari's Breakout or Mario.
Remake Mario
Mario, first created in 1985 is now a classic platformer game. In this tutorial, you'll learn about working with tiles: generating a world with tilemap, loading it into the game, and working with tilemap objects. You'll also learn about animating sprites, camera movement, and particle effects. This tutorial is broken down into three parts.
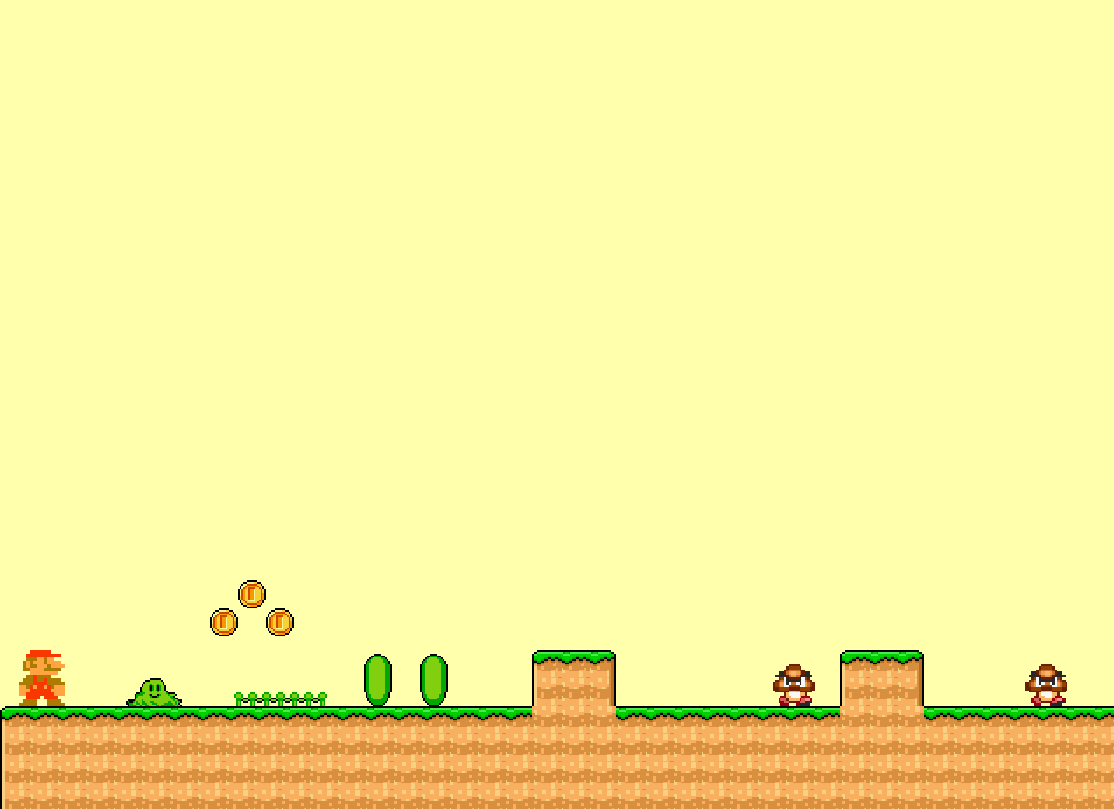
π You'll learn:
Phaser 3 basics
Working with tilemaps and sprites
Camera movement
Character movement
Game objects
Interactibles
Remake Chrome's Dino Game
Chrome's Dino comes online when you go offline. Just like Mario, this tutorial is also broken down into three parts due to its complexity. A lot of concepts are covered in this series starting from building out a game UI and generating game objects, all the way to handling game logic with timers.
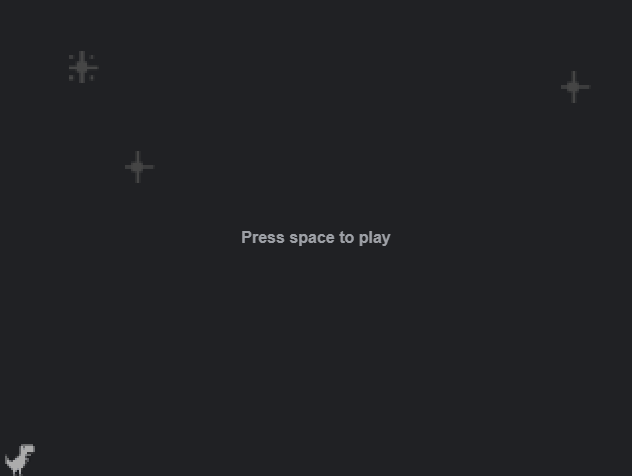
π You'll learn:
Creating game UI
Side scroll behavior
Working with Timers
Collision detection
Dynamically spawning game objects
High score system
Create Atari's Breakout
Last but not least, in this tutorial, you'll learn how you can recreate the famous Atari Breakout game, again with the help of Phaser. We'll look at configuring the game, preloading assets, creating the world with physics, displaying texts, adding collision detection, and much more.
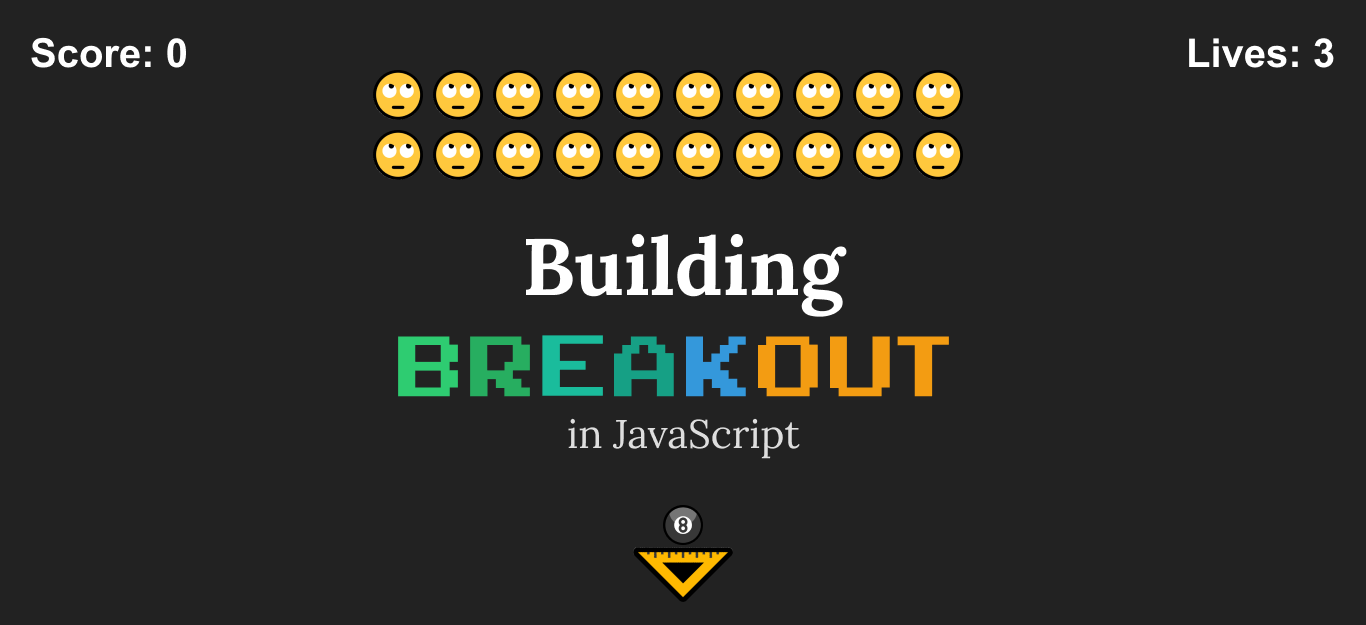
π You'll learn:
Working in Phaser
Loading game assets
Groups and colliders
Working with game text

Summary
Hope you found the right game idea for your next project. If you're looking for more JavaScript project ideas β not necessarily just game ideas β check out our 100 JavaScript project ideas with takeaways and resources such as designs and tutorials, so can only focus on coding. Thank you for reading through, happy coding! π¨βπ»
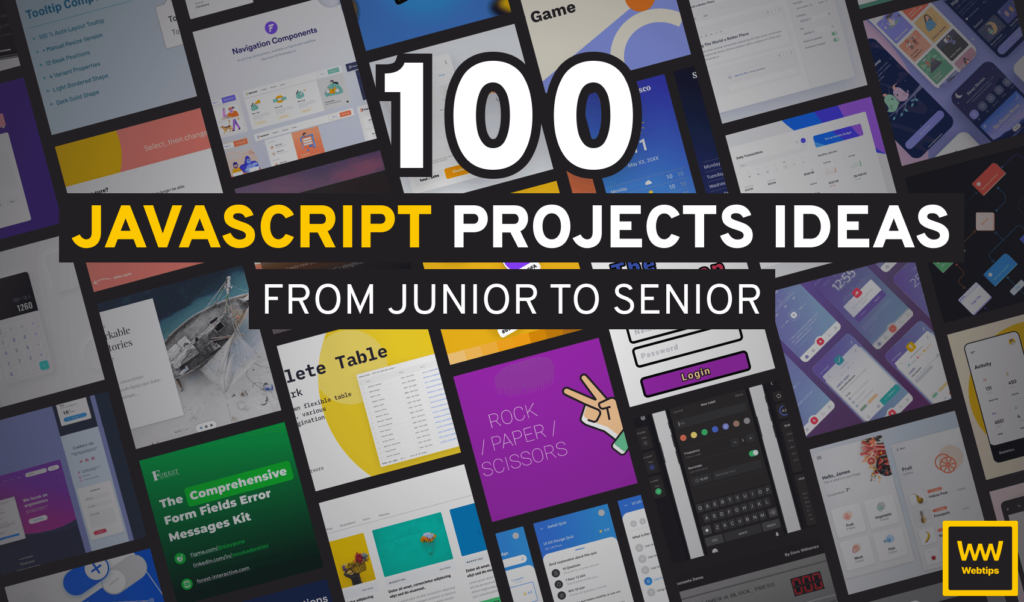
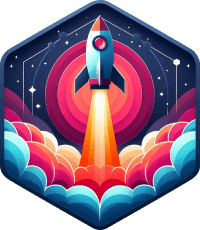
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies:
Courses

Game Development in JS/TS - The Complete Guide

HTML5 Game Development
