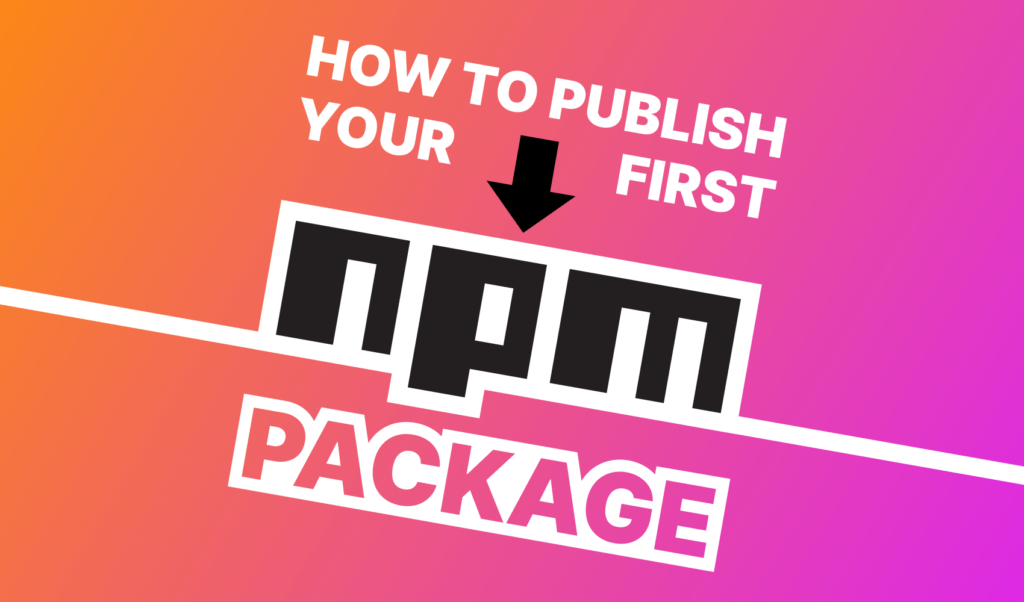
How to Publish Your First NPM Package
The Node Package Manager — or npm for short — has been helping developers since 2010 to create and distribute packages with ease, such as React or Svelte. At the writing of this tutorial, it has almost 1.5 million packages for you to use in your next project.
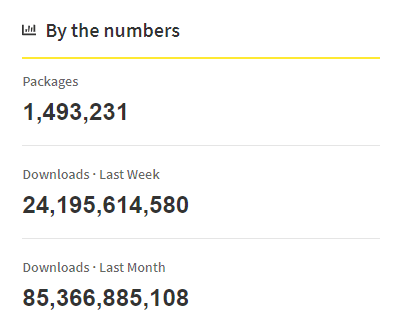
These packages can be installed in any project through CLI with the npm i <package-name>
command. You can also install them globally by appending a -g
flag to the command:
npm i -g <package-name>
This means you can use the package outside of your project, anywhere on your computer. When packages are used as dependencies of a project, NPM can install multiple packages using the package.json
file, which also keeps track of the version of the dependencies.
{
"name": "npm",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC"
}
Making Your First NPM Package
To publish your own package, you need to create a package first. You can create a new empty NPM project by running npm init -y
. This will create a package.json
file with the above properties.
You may want to fill out all the fields as they will be visible on npm. You can view different packages on npmjs.com. You also want to create a README.md
file where you offer documentation to your visitors on how they can use your package.
Make sure the main
file exists — in this case, index.js
— as this will be resolved, once someone tries to import your package with an import
statement. Also don’t forget to export
your package in the file, so it can be imported.
Publishing Packages With NP
To publish an NPM package, we are going to use another package, called np. It is created specifically for publishing NPM packages. This is because as part of publishing, you may want to go over a number of different steps, that you may forget to do each time, such as:
- Running the test to verify your changes
- Updating your
package.json
version number, using semantic versioning - Creating the appropriate git tag for the version
- Pushing the changes both to GitHub and NPM
- Writing the changelog for the release
To start creating NPM packages in the first hand, you need to register an account on npmjs, if you haven’t got one already. Go to npmjs.com/signup and create a new account.
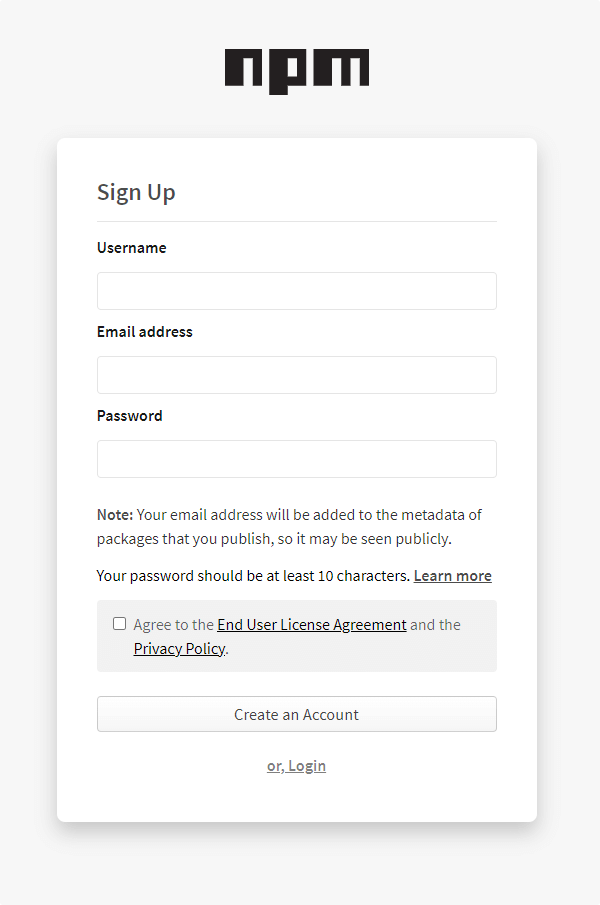
Make sure you verify your email address after account creation, otherwise you may get 403 errors while trying to publish your package.
Once you’ve signed up, you can log in to your account inside your project through the command line, by running npm login
.
After you’re logged in, install np globally by running npm i -g np
. Then by running np
in your project directory, you can start the process of publishing packages.
What are the prerequisites?
Before you start publishing, however, you need to make sure of a couple of things:
- You need to have a GitHub repository attached to your project. Otherwise the
prerequisite check
step will fail. - You also need to have a clean working directory, so make sure you either commit or stash your changes.
np
will also run thenpm run test
command. By default, this will makenp
fail because of theexit 1
command at the end. Make sure you remove that.
"scripts": {
- "test": "echo \"Error: no test specified\" && exit 1"
+ "test": "echo \"Error: no test specified\""
},
Now that you have everything set up, you can run np
to start publishing.
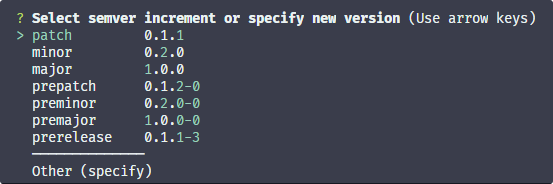
First, np
will ask you how you want to increment the version number. It uses semantic versioning. After you choose a version, it will start running the checks for each step.
If you get 403 errors asking if you are logged in as the correct user, you may trying to publish a package that already exists on npm. You can check if a package exists by simply navigating to npmjs.com/package/<package-name>. If the package exists, you need to rename the name field in your package.json
file.
If all the names you come up with are already taken, you can also scope the package to your username, and name the package as @user/package-name
.
If you still get 403 errors because of two-factor authentication, you can disable it through the CLI by running np
with the --no-2fa
flag.
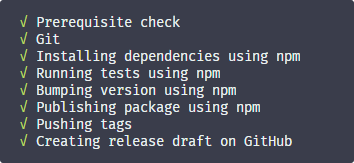
If everything goes well, you should see all the above steps turning green. After it finished creating a release draft on GitHub, it will automatically open a browser tab for you with all your changes included as a description for the release.
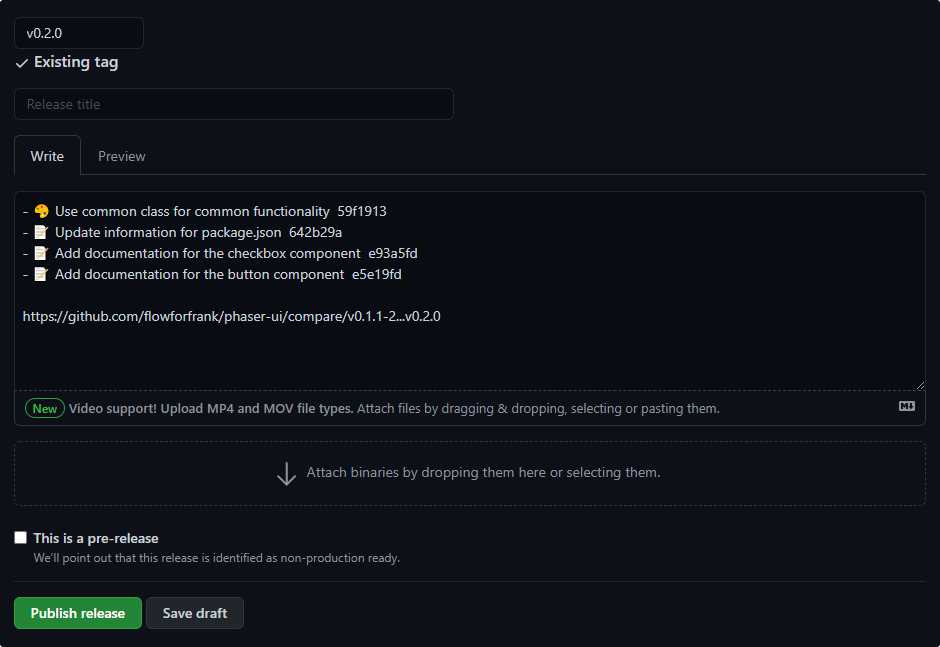
How to test packages locally
Now, whenever you publish completely new packages, or major updates, you may also want to test your changes locally before deploying a new version. To do this, you can use the npm link
command. This is used for symlinking packages. Simply run npm link
in your package folder, then jump into another project where you want to try out your package. You can then run npm link <package-name>
to link it to your project.
- cd package-folder
- npm link
- cd project-folder
- npm link package-folder
Any changes you make to package-folder
, will also be reflected while you use that package in the project-folder
.
How to deploy only the distribution files
If you try to pull your npm package with npm i <package-name>
, you may notice that your whole project is distributed, and this may not be what you want. Rather, you only want the distribution files to be deployed, so others can pull in your transpiled and minified version of your package.
In order to do this, you can specify the files you want to copy in your package.json
file using the files
array. For example, to only copy the files from your dist
folder, you would do:
"files": [
"dist"
]
Note that files specified here cannot be excluded through your .gitignore
or .npmignore
file. Regardless of what you specify in your files
array, some files will always be included, such as your package.json
or README
/ LICENSE
files. Likewise, some files will always be ignored, such as .git
or your node_modules
.

Summary
And now you should have your new NPM package ready to use by everyone. You can view your package on npmjs under your profile, as well as directly visiting npmjs.com/package/<your-package-name>
.
When publishing new packages, make sure you always include thorough documentation with examples as well as a changelog on what was changed in your version. If you want contributions, it’s also a good thing to add a CODE_OF_CONDUCT.md
.
Do you have experience with publishing NPM packages? Let us know your thoughts in the comments below! Thank you for taking the time to read this article, happy deploying!
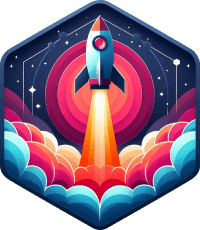
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: