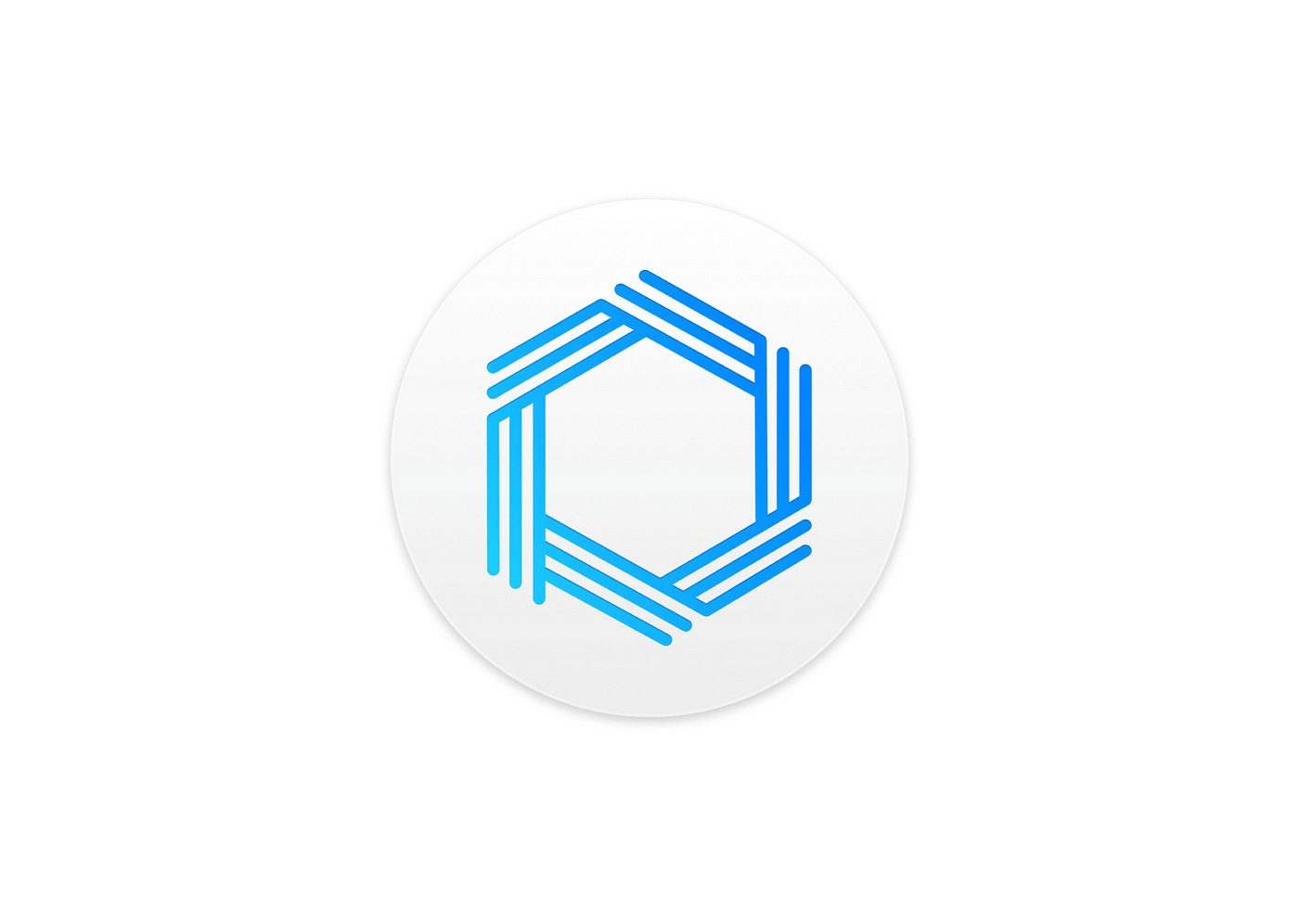
How to Modify JSON Responses With AnyProxy
How many times did you struggle to test out your frontend features on a staging environment because the backend dependencies were not in place yet? It happens all the time with me and it can often take days before external dependencies are resolved.
I recently stumbled upon AnyProxy.io — a configurable proxy written in NodeJS, created by the developers at Alibaba. With it, you can write rules in JavaScript to intercept specific network requests and make some modifications to them before they are returned to you.
So in this tutorial, we will take a look at how we can add missing JSON nodes to our requests so we can test out our feature outside of our local env, without having to wait for other teams to implement their changes.
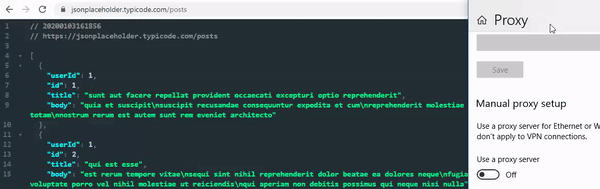
Setting Up AnyProxy
Your very first step is to install AnyProxy globally with the npm i -g anyproxy
command, so we can type anyproxy
into the command line to start a proxy server. It will fire up the proxy on port 8001 and a web interface on 8002. If you visit localhost:8002
you’ll be greeted with an empty dashboard:

This is where you’ll be able to inspect requests to see, which one you’ll need to override. By default, only HTTP requests are intercepted. To view decrypted information on HTTPS requests, you’ll have to configure some certificates.
Generating certificates
In its core, AnyProxy uses man-in-the-middle attacks to decrypt HTTPS requests. To avoid getting errors on the client-side we will have to generate and trust a CA certificate. Type in anyproxy-ca
to generate your certificates.
Inside Chrome, open chrome://settings/?search=manage+certificates
, you’ll see the option highlighted:

Open it up and go to the “Trusted Root Certification Authorities” tab and import the generated AnyProxy certificate. You may also want to chrome://restart
after to make sure the certificate takes effect.
Enabling the Proxy
Now you’ll need to turn on the proxy server and set your address to your IP and your port to 8001 — This is where AnyProxy listens. Type in “proxy” to your search bar and click on “Change proxy settings”. You’ll be greeted with the following window:

You can get your IP address by typing ipconfig
into your command line.
Intercepting HTTPS
Now you’re all set and done. If you start AnyProxy with the --intercept
flag it will start to catch every request for you:
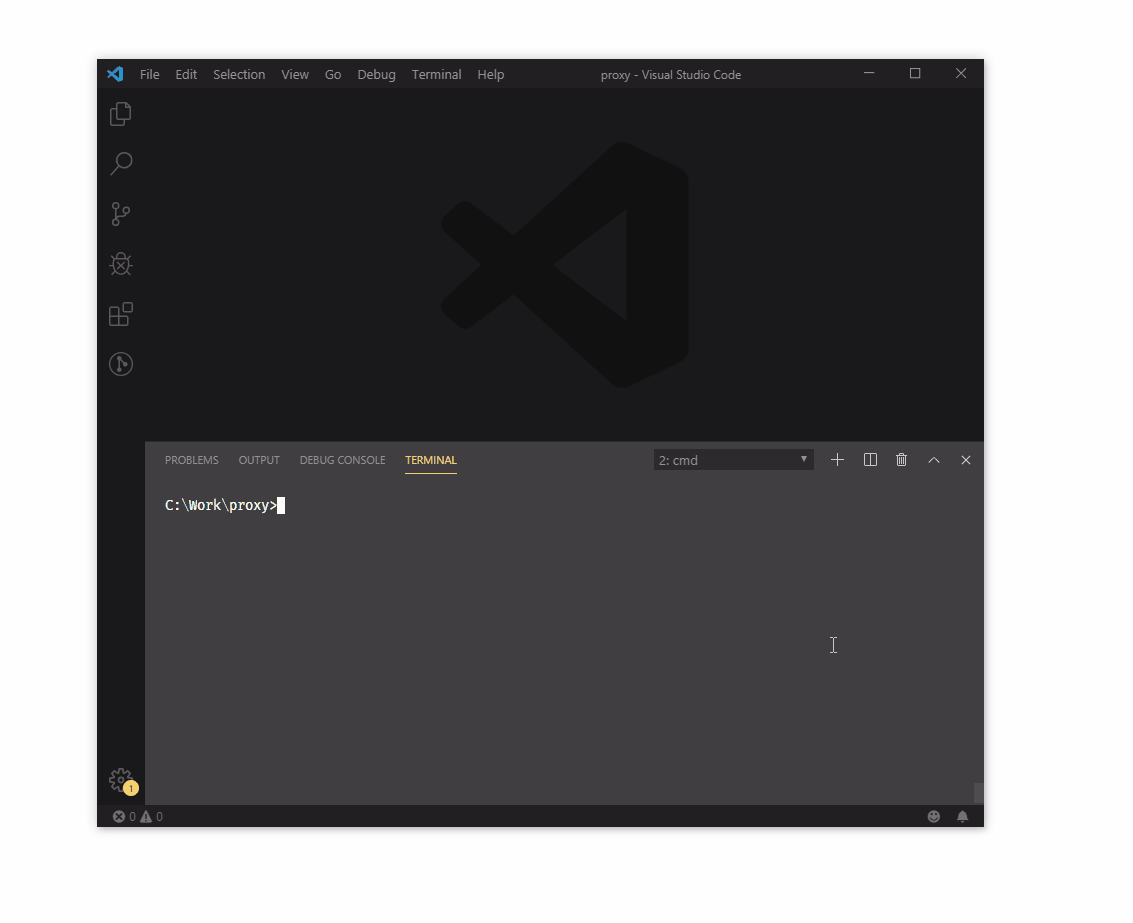
By clicking on a request you’ll see both the request and the response object along with all the information it contains, such as the header and the body.
Adding the Rewrite Rule
All that’s left to do is to create a rule so we can catch the single request we are interested in and rewrite its response. I’ve created a rule.js
file which exports a single function: beforeSendResponse
.
const fs = require('fs');
const data = fs.readFileSync('./data.json');
const url = 'https://jsonplaceholder.typicode.com/todos/1';
module.exports = {
*beforeSendResponse(requestDetail, responseDetail) {
if (requestDetail.url === url) {
responseDetail.response.body = data;
}
return {
response: responseDetail.response
};
}
};
This function will get executed right before sending the response down to the client. As you can see, we don’t do much here. We check the URL of the request and if it matches the one we specified, we rewrite response.body
with the data
variable.
And this data is coming from the file we read in with the fs
module which contains the updated JSON response, the one we would like to see. If you visit the URL right now, you’ll see it returns the following response:

We have some basic info on a todo item. But what if we are working on a feature that adds some metadata to the item under a meta
node? This would contain the creation date, the author, and a note about the todo. We want to see the changes we made on the frontend that uses this new node, but the backend part of the work is yet to be done.

Overriding Requests
I’ve created a data.json
in the same folder I have my rule.js
file. The contents are the following:
{
"userId": 1,
"id": 1,
"title": "delectus aut autem",
"completed": false,
"meta": {
"createdDate": "02/02/2020",
"author": "John",
"note": "Created to demonstrate the use of AnyProxy"
}
}
I’ve copied the original response and added the meta
node. This is what we would like to get back from the URL we provided in our rule.js
file. So how do we tell AnyProxy to use it while intercepting requests? It’s real simple. Start AnyProxy with a --rule
flag pointing at the rule file: anyproxy --intercept --rule rule.js

And that is how you intercept requests and try out your frontend changes on a staging env without having to worry about external dependencies. 🎉 Let me know in the comments below what are your favorite use cases of proxies. Thank you for taking the time to read this article, happy debugging!
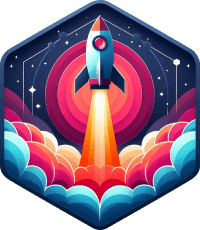
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: