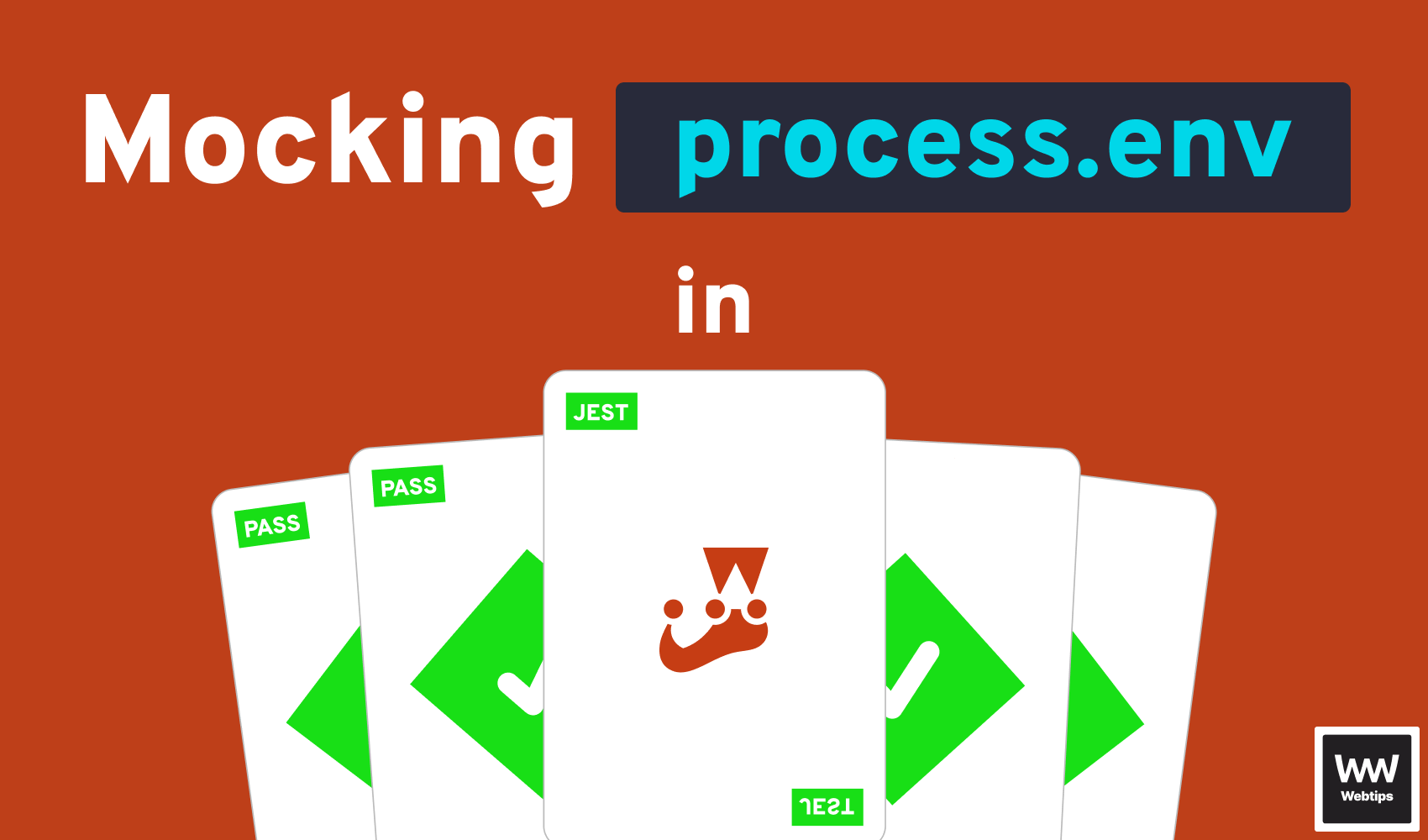
How to Mock process.env in Jest
Modern frontend applications depend on environment variables to present different behaviors in different setups. Most commonly, you will come across that process.env is used in a codebase to differentiate between production and development.
This means that when testing process.env
specific parts of your app, you may run into errors if it is not set to the correct state. You may be tempted to write:
it('should mock process.env', () => {
process.env.NODE_ENV = 'development'
})
However, this will cause unexpected behaviors, as you will override the process values for other test cases as well.
Need to mock global variables in Jest? Check out this tutorial.
Mocking process.env
To mock process.env
the right way, we need to set up before and after hooks to first make a copy of the original object, and then reset it after each test case:
describe('process.env', () => {
const env = process.env
beforeEach(() => {
jest.resetModules()
process.env = { ...env }
})
afterEach(() => {
process.env = env
})
})
For this, we can use the beforeEach
and afterEach
hooks. Inside the beforeEach
, make sure you call jest.resetModules
first to clear the cache for the required modules to avoid conflicting local states. Then we can make a copy of process.env
from the original object, and in the afterEach
hook, we also need to restore the old configuration to avoid conflicting states.
Testing the mocked values
Now that we have everything set up, we can add, remove, or reassign existing properties on process.env
, just like you normally would with an object:
it('should mock process.env', () => {
process.env.NODE_ENV = 'development'
console.log(process.env.NODE_ENV) // Will be "development"
})
it('should not mock process.env', () => {
console.log(process.env.NODE_ENV) // Will be "test"
})
Note that for subsequent it
calls, the process.env
will be reset to its original state because of the afterEach
hook. If you would like to provide a global setup for your process.env
, you can also create a setup file that you can reference in your Jest config, using the setupFiles
field:
module.exports = {
setupFilesAfterEnv: ['<rootDir>/setup-test-env.js']
}

Summary
In summary, to mock process.env
in Jest, you need to:
- Set up a
beforeEach
hook to clear the cache for the required modules, and make a copy ofprocess.env
- Set up an
afterEach
hook to resetprocess.env
to its original state - Add, delete, or reassign values to
process.env
in your test cases
Are you new to Jest and would like to learn more? Check out the following introduction tutorial below:

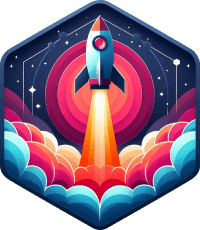
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies:
Courses

JavaScript Unit Testing

Unit Testing for TypeScript and Node.js Developers
