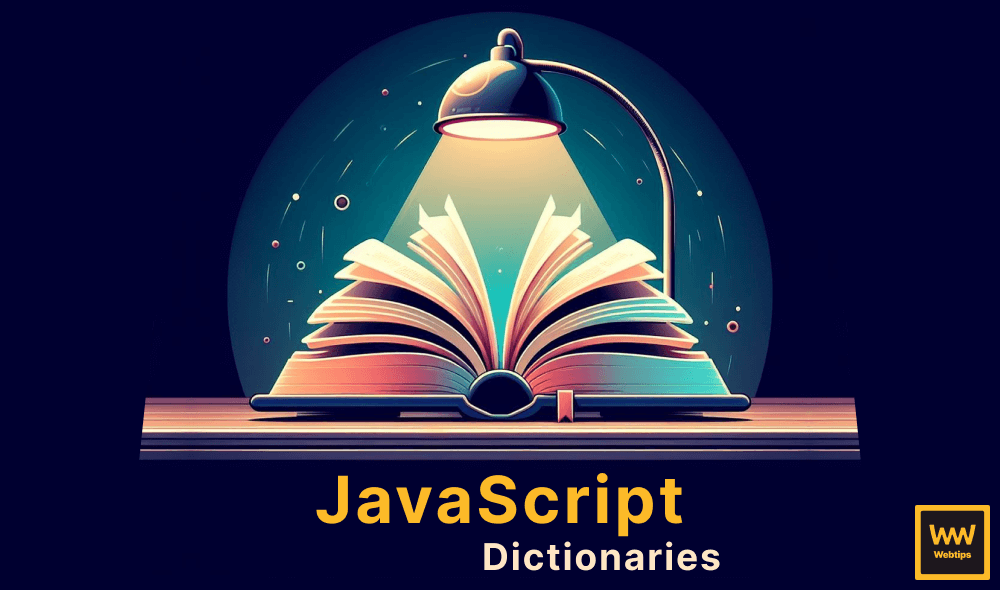
How to Create Dictionaries in JavaScript
In programming languages, a common data structure called a dictionary is often used for storing data in key-value pairs. It provides a flexible way to store and read data when it's presented with a key. Unlike in statically typed languages, however, there is no such type as Dictionary
in JavaScript. So how do we create one? We have a couple of options.
Creating a Dictionary in JavaScript
In JavaScript, dictionaries can be created through Objects
. To create a new object (among many other options), we can use an object literal:
// Using object literals
const obj = {
key: 'value'
}
Objects can be defined using curly braces. Each object can contain an arbitrary number of values, called properties. These properties are created using key-value pairs. If youβre interested in what are some other less commonly used ways to create objects, be sure to check out the following tutorial:
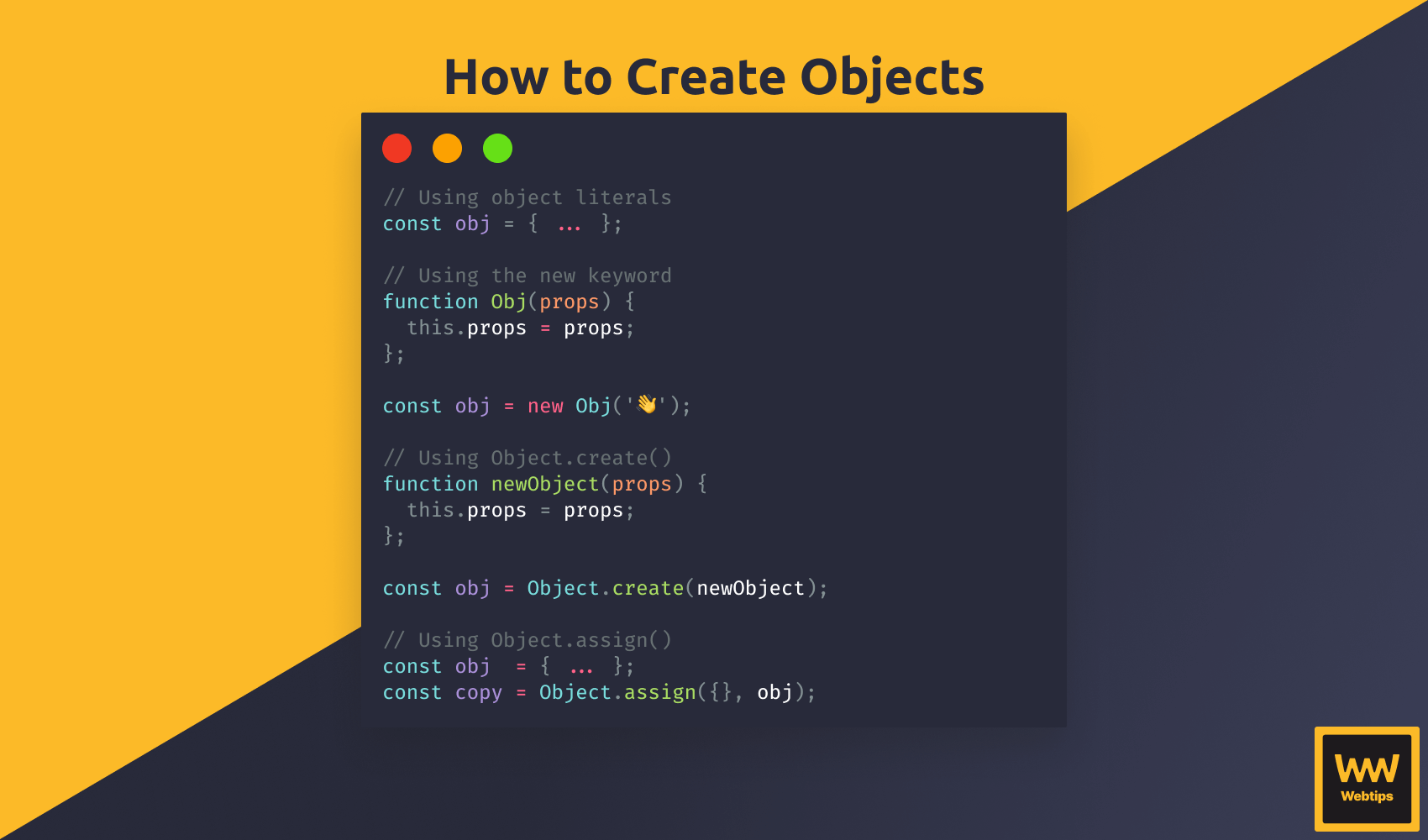
How to Add Values to a Dictionary
To add values to a dictionary β a JavaScript object β, we can initialize it with key-value pairs as in the previous example:
// Add default values during initialization
const dictionary = {
PUBLIC_API_KEY: '1c6aa69fb9d27cb4f29c81611d0b1ff5',
PRIVATE_API_KEY: '4fa758e6d388593f8637da4bdd697ef6'
}
Moving forward, we'll refer to dictionaries as "objects".
In this example, our object will have two properties: PUBLIC_API_KEY
and PRIVATE_API_KEY
. We can name object keys however we like, as long as they only contain valid characters from the English alphabet. We can also use different naming conventions, such as snake_case
in the above example. Most commonly, however, JavaScript uses camelCase
. The values of keys can be any valid JavaScript data type, including other objects. Take the following as an example:
In this case, our dictionary
variable has a user
key that references another object, which has a name
property. To populate our object with additional values after declaration, we can use indexes, bracket notation, or just call a property directly with dot notation:
If we try to log out the dictionary
variable to the console, we'll get the above output. Note that with bracket notation, we can also use a dash. This is not the case for the other options. If we try to set it through dot notation, we'll get the following error:

We also have the option to use other variables as a name for keys in the object, using bracket notation. This makes it possible to dynamically set properties. Simply pass the variable between brackets:
const key = 'blue-book'
const dictionary = {
[key]: 'π'
};
// You can also do
dictionary[key] = 'π'
Try to copy-paste the above code into the interactive editor and log out the value of the dictionary
variable to verify its properties.

How to Access Keys
If we want to access these values, we can use the same options as before, just without the assignment:
// Returns "c8b0c9e9b1618b4fb35c47ed4e2fadc7"
dictionary[2]
// Returns "π"
dictionary['blue-book']
// Returns "π"
dictionary.book
Note that if we want to access a property that has a dash or number in it, we have to use bracket notation again. Accessing properties with variables is also possible, just like creating new ones:
const key = 'blue-book'
// Returns "π"
dictionary[key]
When working with nested objects, the most common way to access the value is to use dot notation in the following way:
However, as we can see, we can also use bracket notation by combining multiple brackets after each other. In this case, we need to pass the keys as strings inside the brackets.
Note that in case one of the properties is undefined
, we'll run into an error. To get around this, we have several options that are outside the scope of this tutorial. However, if you're interested in how to resolve them, be sure to check out the following tutorial that discusses the possible options in detail:
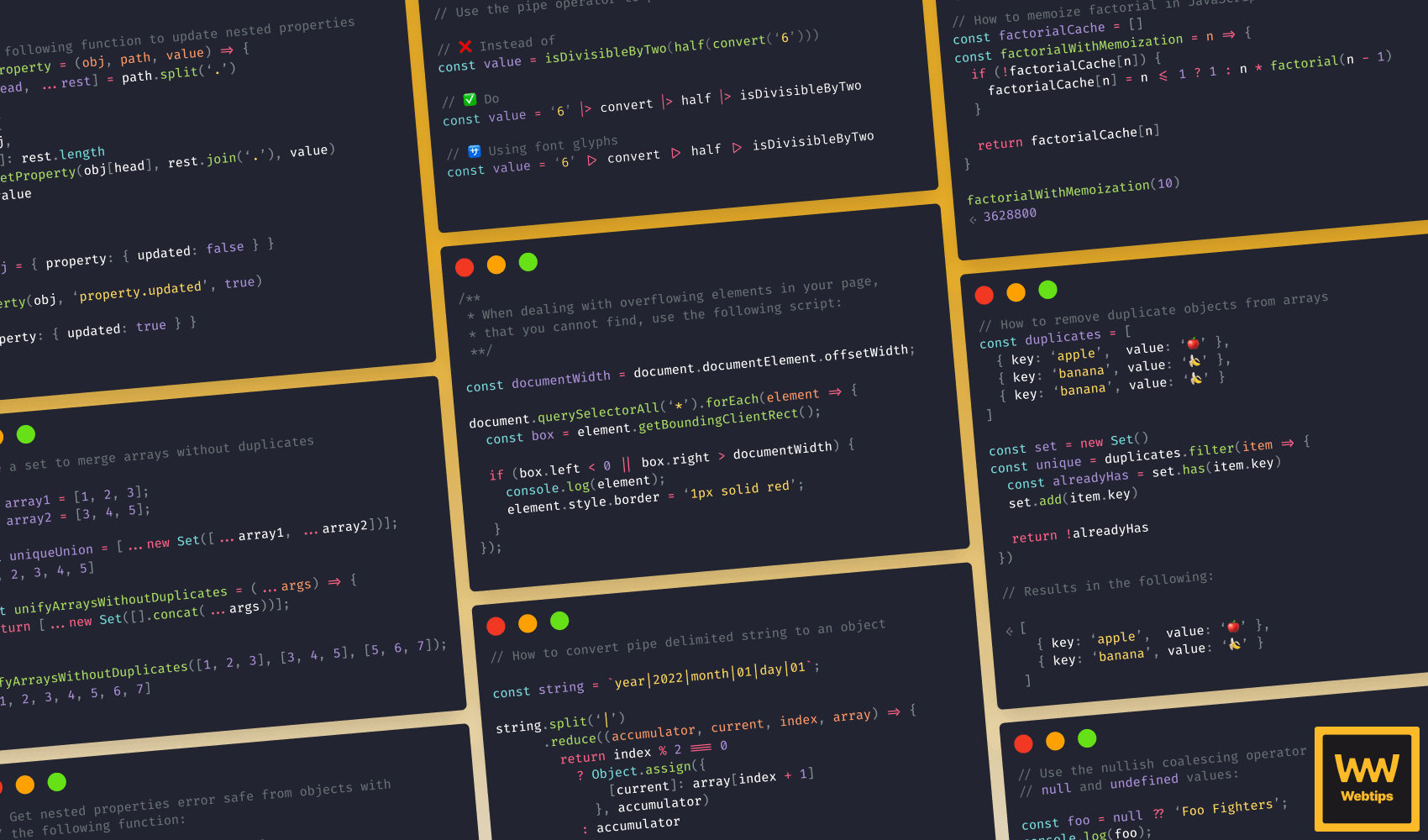
Iterating Through Dictionaries
If we want to loop through our whole object, we can use a for...in
loop in the following way:
To filter out inherited properties, we can use an if
statement to check whether the property we are working on belongs to the dictionary
. Note that we can access values inside the loop with bracket notation. We can also use a more declarative approach using Object.entries
combined with a forEach
loop:
Object.entries(dictionary).forEach(entry => {
console.log(`key: ${entry[0]}, value: ${entry[1]}`)
})
Try replacing the for loop in the interactive editor to verify that the output of the above code matches the output of the for loop. Object.entries
turn the object into an array of elements, each array containing a subarray that contains the key and the value. Try logging out only the value of Object.entries
to see its return value. You can reveal the solution on how to achieve this if you get stuck.
As Object.entries
return an array, we can chain the forEach
array method to loop through the elements and log the values to the console. This is called function chaining.
Dictionaries with Maps
Another data type in JavaScript that is similar to objects but comes with added benefits is maps. Just like objects, they hold key-value pairs, but they remember the insertion order of the keys, and they also come with helper methods. To create a new map, we can use the following code:
We initialize the dictionary variable to an empty map. To add values to a map, we can use the set
method that accepts two parameters: key and value. To reference these values later on, we need to use the get
method. Try to log the name
property of the map in the above editor. Make sure to pass the key as a string to the get
method.
Apart from set
and get
, maps also have the following helper methods to make working with them easier:
dictionary.has('name')
dictionary.size
dictionary.delete('age')
dictionary.clear()
has
: We can usehas
to check if the map has the passed key. It'll returntrue
if the key exists andfalse
if not.size
: Returns the number of key-value pairs in the map. In our example, it'll return two, as we have aname
andage
keys.delete
: Deletes the passed key from the map. If the key exists, it'll returntrue
. Otherwise, it'll returnfalse
. If we callhas
on a deleted key, it'll returnfalse
.clear
: Remove all key-value pairs from the map, leaving an empty map.
Maps also come with different methods that can be used for looping through them:
// Loop through keys and values:
for (const [key, value] of dictionary.entries()) {
console.log(key, value)
}
// Loop through keys only:
for (const key of dictionary.keys()) {
console.log(key)
}
// Loop through values only:
for (const value of dictionary.values()) {
console.log(value)
}
We can use the entries
method to loop on both keys and values, use the keys
method to loop only on keys or use the values
method to loop only on values.
Things to Keep in Mind
And that is how you can create dictionaries in JavaScript. Some things to keep in mind:
- Use object literals for better readability when creating new objects.
- Try to stick to one naming convention whether it be
camelCase
,snake_case
, orkebab-case
. - You can use variables to write and access properties.
- Make sure to include an
if
statement inside afor...in
loop if you want to filter out inherited properties. However, prefer usingObject.entries
for improved readability. - Only use maps over objects when you need to frequently modify a collection. Using the
set
anddelete
methods of maps can improve code readability and have better performance over regular objects:
Do you still have questions regarding objects in JavaScript? Let us know in the comments below so we can help! Thank you for reading through, happy coding! To learn more about JavaScript, continue with our guided roadmap:
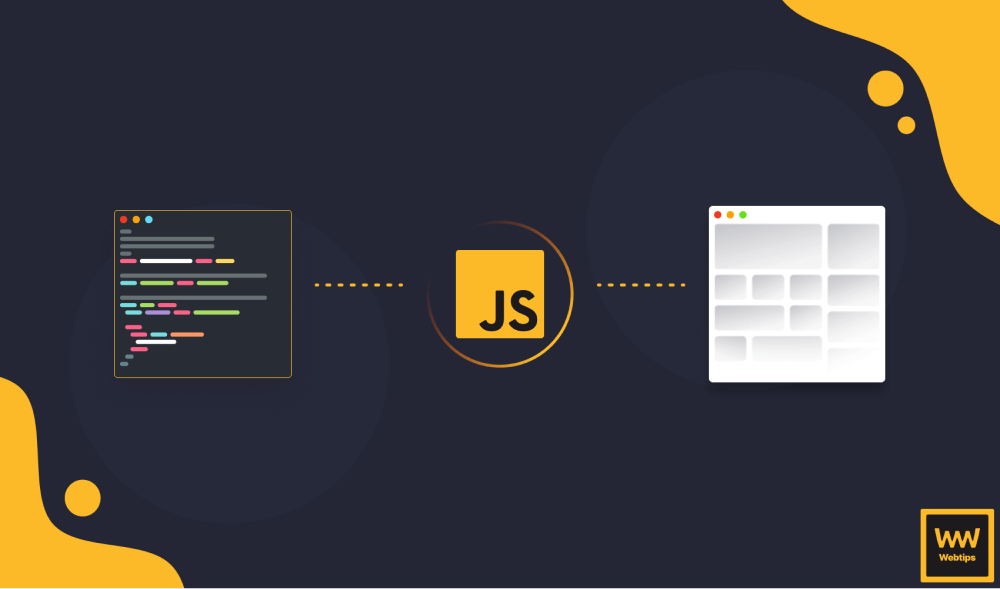
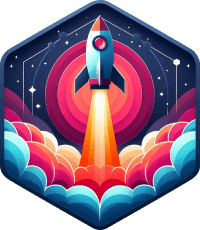
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: