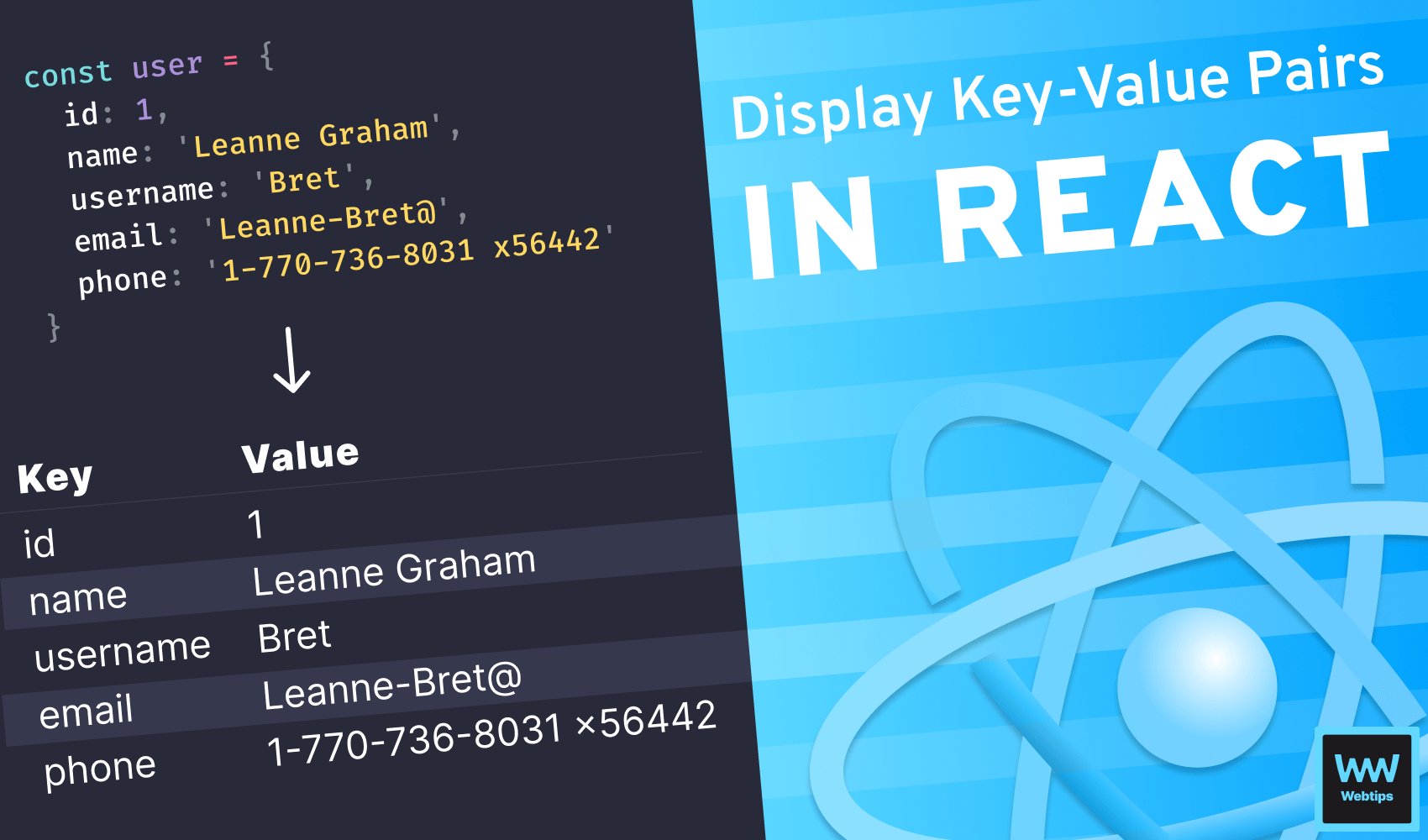
How to Display Key-Value Pairs in React in a Table
Displaying key-value pairs in React in a tabular format is a great way to quickly represent data without writing too much code. A custom component can also help you visualize objects and debug issues quickly.
To display key-value pairs, we can use a combination of the Object.keys
object method with a chained map
array method to loop through the keys:
import React from 'react'
const user = {
"id": 1,
"name": "Leanne Graham",
"username": "Bret",
"email": "Leanne-Bret@",
"phone": "1-770-736-8031 x56442"
}
const App = () => (
<table>
<thead>
<tr>
<th>Key</th>
<th>Value</th>
</tr>
</thead>
<tbody>
{Object.keys(user).map((key, index) => (
<tr key={index}>
<td>{key}</td>
<td>{user[key]}</td>
</tr>
))}
</tbody>
</table>
)
export default App
More example user data can be found on JSON Placeholder.
How It Works
Here, we have an example user that we want to display in a tabular way. The important parts are highlighted. We need to use a JSX expression and wrap the object we want to display (in this case, the user
variable) inside Object.keys
, which returns the keys for the passed object.
['id', 'name', 'username', ...]
Using this array, we chain map
from it to display a row for each property of the object. Don't forget to pass a unique key
prop to the tr
to let React keep track of the rendered elements.
Using the key
variable inside the map
, we can display the key of the object in the first column, and using bracket notation, we can display the corresponding value next to it with user[key]
. This will result in the following table:
Key | Value |
---|---|
id | 1 |
name | Leanne Graham |
username | Bret |
Leanne-Bret@ | |
phone | 1-770-736-8031 x56442 |
Using a Custom Component
We can also extract the above logic into a custom component to make it reusable throughout an application. Create a new component in your project called KeyValueTable
and add the following code:
import React from 'react'
const KeyValueTable = ({ obj }) => (
<table>
<thead>
<tr>
<th>Key</th>
<th>Value</th>
</tr>
</thead>
<tbody>
{Object.keys(obj).map((key, index) => (
<tr key={index}>
<td>{key}</td>
<td>{obj[key]}</td>
</tr>
))}
</tbody>
</table>
)
export default KeyValueTable
This component expects an obj
prop that we can use inside the table to display its keys and values. To use it anywhere, simply import the component and pass the object you want to display as the obj
prop:
import React from 'react'
import KeyValueTable from './KeyValueTable'
const user = {
"id": 1,
"name": "Leanne Graham",
"username": "Bret",
"email": "Leanne-Bret@",
"phone": "1-770-736-8031 x56442"
}
const App = () => (
<KeyValueTable obj={user} />
)
export default App

How to Dump JSON Data
In case you simply want to dump JSON data to the screen, you can use the built-in JSON.stringify
function with the following three parameters:
const App = () => (
<pre>{JSON.stringify(user, null, 4)}</pre>
)
user
: The object we want to display as a string.null
: The second parameter is for a replacer function that can alter the behavior of the stringification process. Since we want to display the data as is, we can keep this asnull
. A common example of the replacer function is a filtering functionality that filters unwanted values from being displayed.4
: The indentation we want to use, in this case, 4 spaces.
Make sure you wrap the whole expression into a pre
tag to ensure proper formatting. The above code example will result in the following output:
{ "id": 1, "name": "Leanne Graham", "username": "Bret", "email": "Leanne-Bret@", "phone": "1-770-736-8031 x56442" }
Summary
In summary, displaying key-value pairs in React can be done with a simple combination of Object.keys
and map
. If you would also like to learn how to take this example one step further, and fetch the data from a remote resource, be sure to check out the following tutorial:
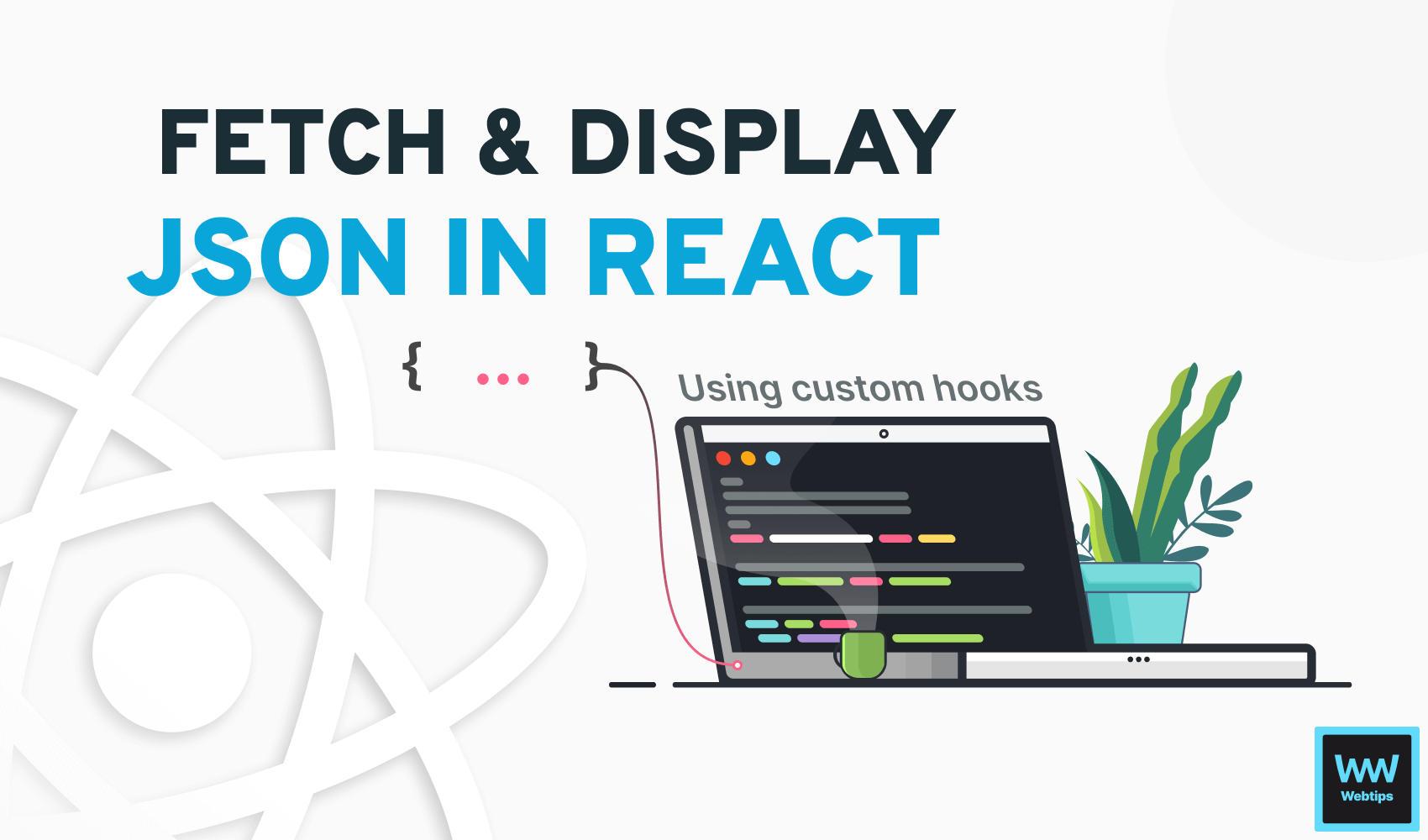
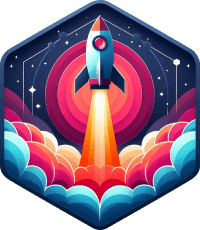
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies:
Courses
Recommended
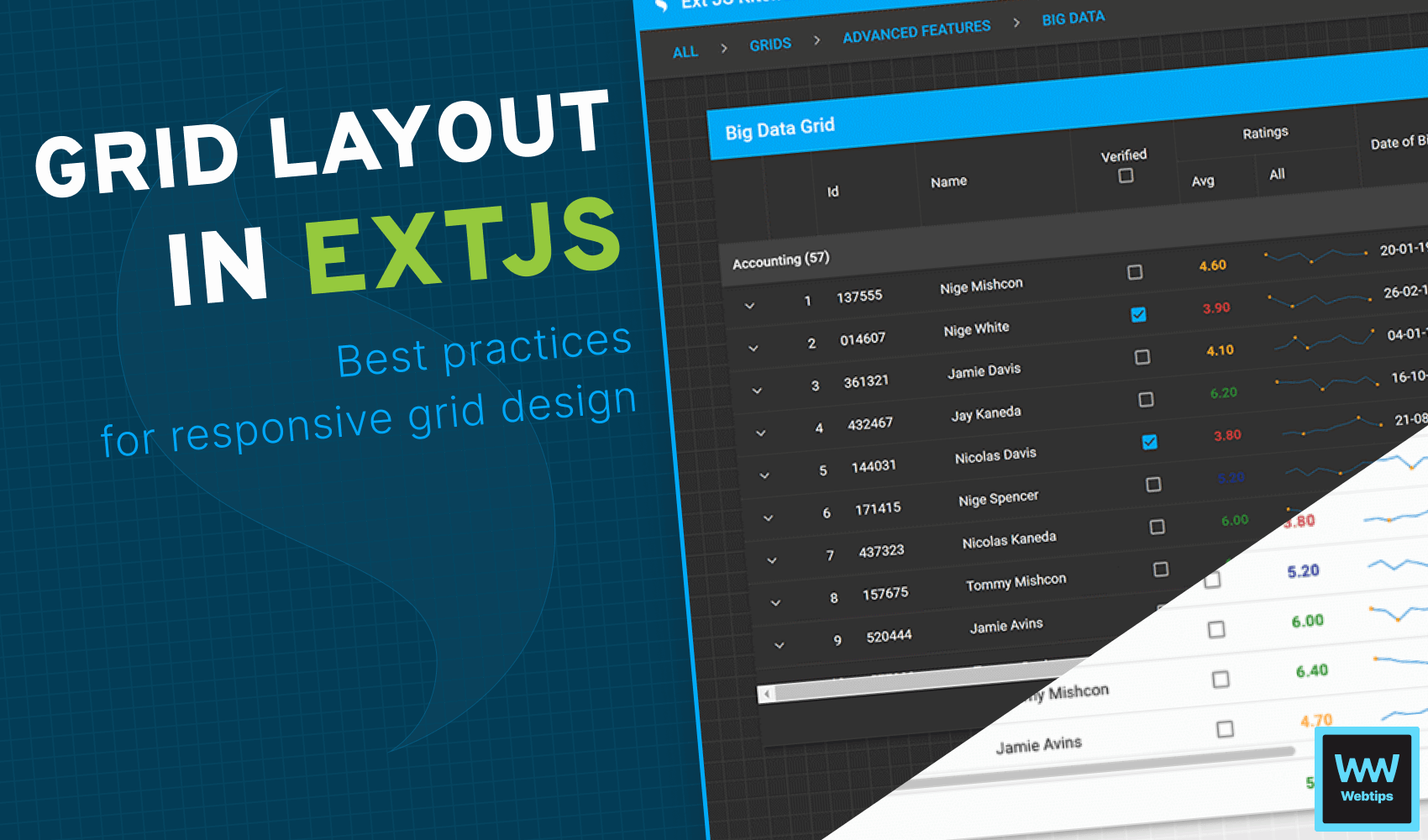
Effective Web App Development with Sencha Ext JS Grid Layout
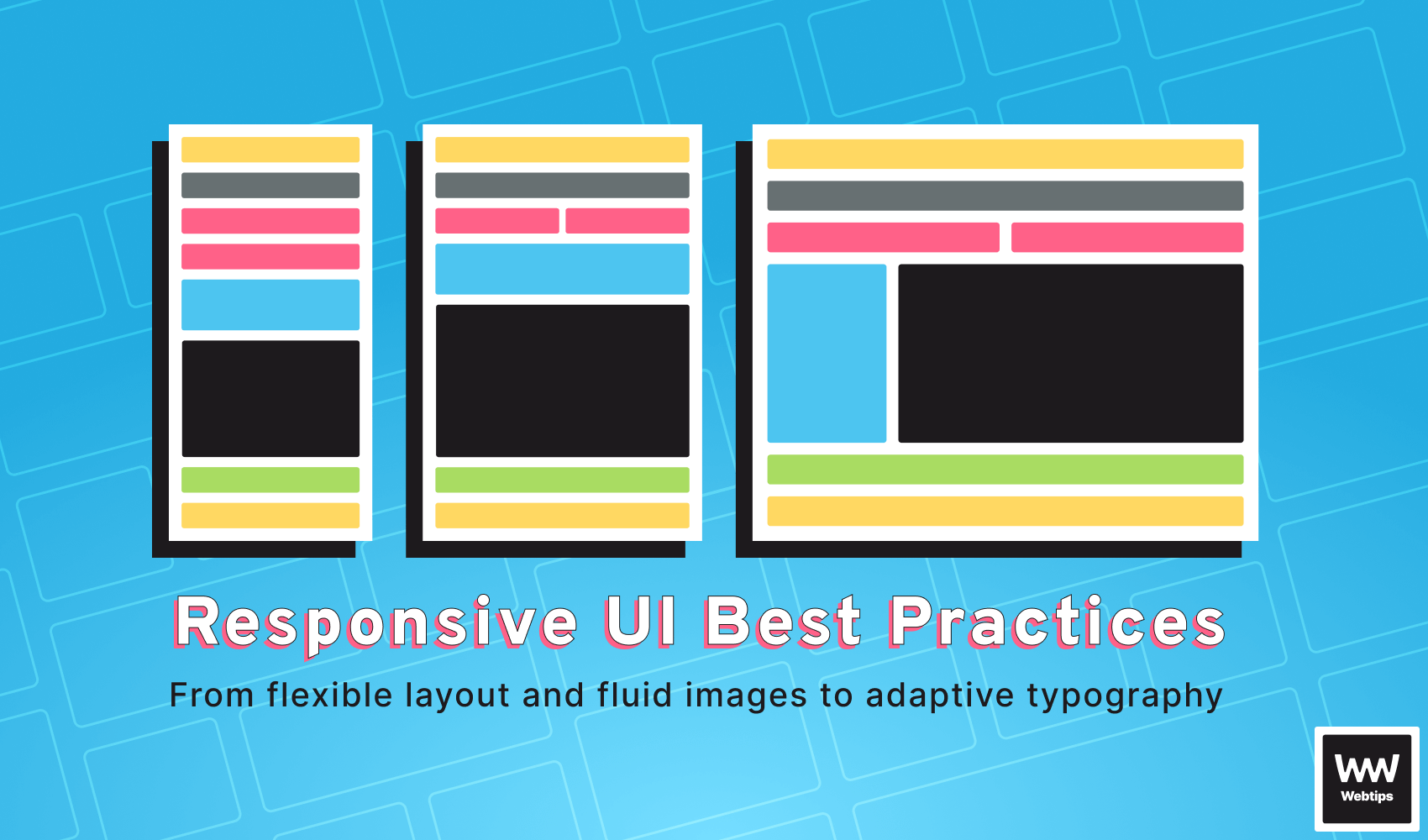
Best Practices for Responsive User Interfaces Using CSS
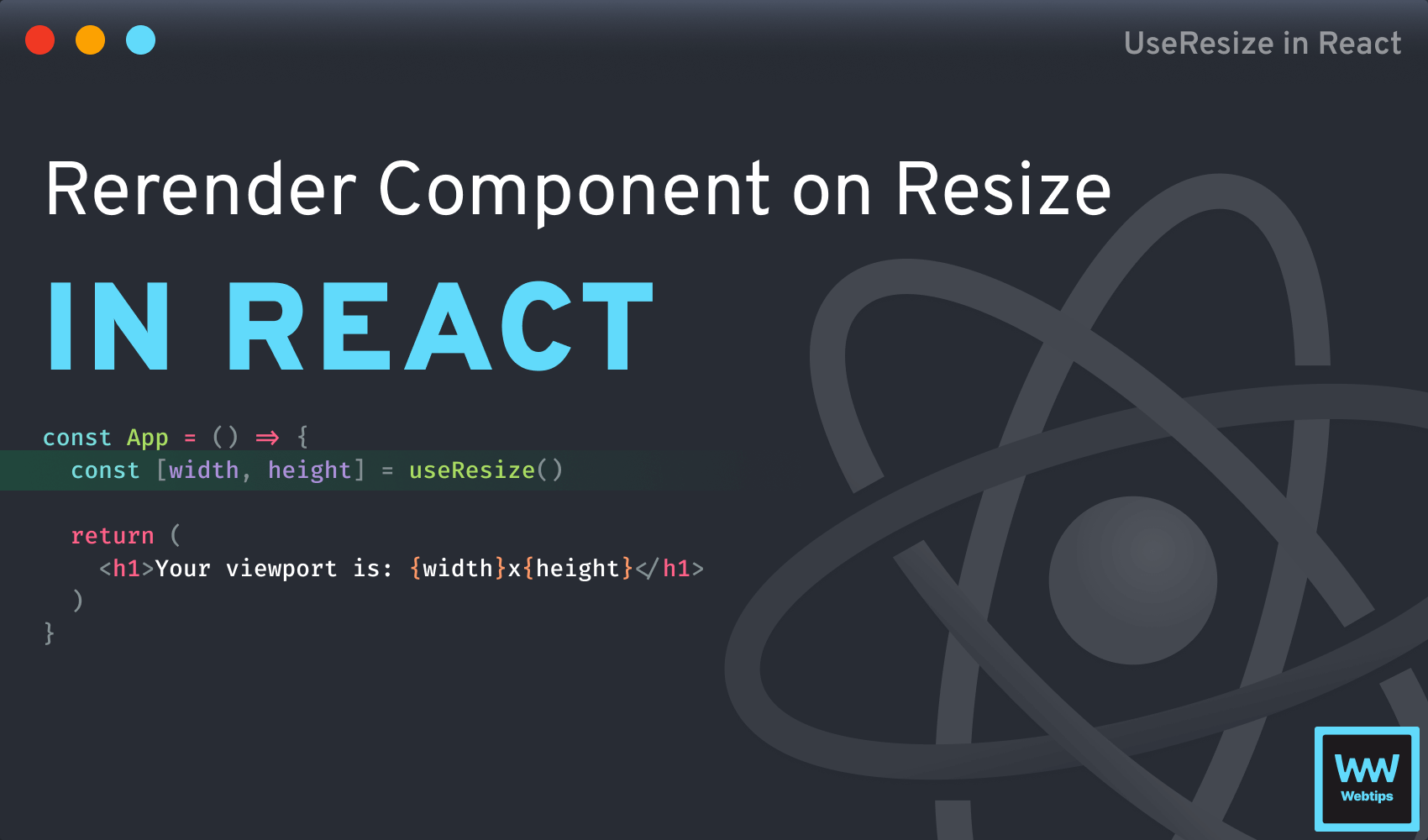