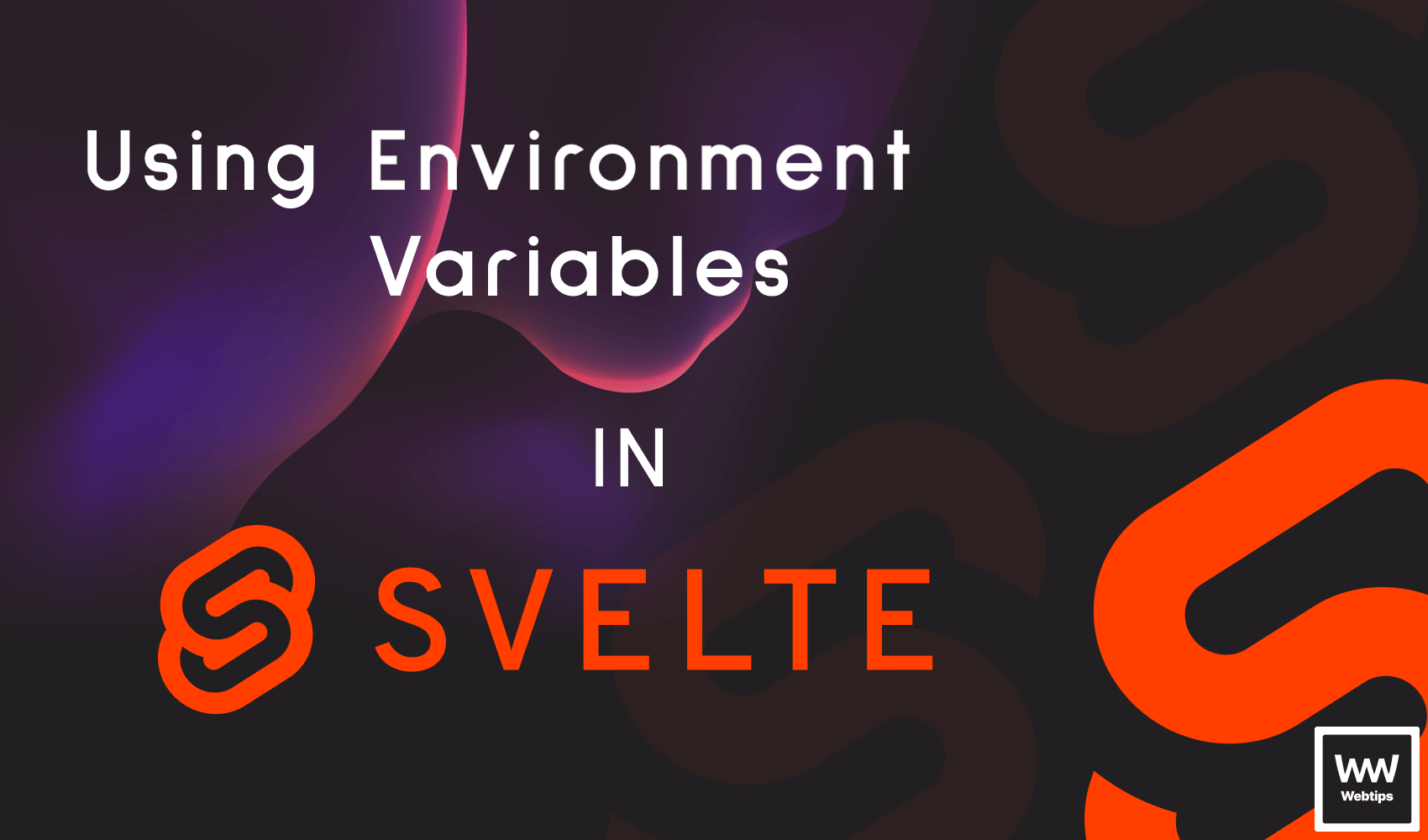
The Quick Way to Add Env Variables to Svelte using Vite
As Svelte uses Vite, and Vite uses dotenv
to load environment variables into the app, all we have to do is introduce a .env file at the root of our project, and add our environment variables from there. Take the following as an example:
VITE_API_ENDPOINT=/cms/api
API_SECRET=1234
We defined two different environment variables, one for the API endpoint, and one for the secret key. Notice the difference in naming. Anything prefixed with "VITE" will be publicly available, while others are not.
To access the above-defined environment variables from a Svelte component, we can reference import.meta.env
, plus the name of the environment variable.
<script>
// This will log out /cms/api
console.log(import.meta.env.VITE_API_ENDPOINT)
// This will log out undefined
console.log(import.meta.env.API_SECRET)
</script>
If we now try to log out the environment variable without the "VITE" prefix, it will be undefined
. This prevents accidentally leaking sensitive information to the client. Any environment variable that has the "VITE" prefix will end up in your client bundle too.
Never store sensitive information in publicly available environment variables.
The value of the prefix can be changed if needed using the envPrefix
property inside vite.config.js.
Default Environment Variables Exposed by Vite
Vite also exposes some other commonly used environment variables by default. You can see and access all of them if you log import.meta.env
to your console. The following environment variables are available in Vite by default:
{
BASE_URL: '/',
DEV: true,
MODE: 'development',
PROD: false,
SSR: false
}
BASE_URL
: The base URL as a string where the app is being served from. This can be changed using thebase
config.DEV
: A boolean flag for telling whether the app is running in development mode (always the opposite ofPROD
)MODE
: The mode (as a string) the app is running inPROD
: A boolean flag for telling whether the app is running in production mode (always the opposite ofDEV
)SSR
: A boolean flag for telling whether the app is running in the server
Using Different Environment Variables for Different Environments
It's also possible to use different environment variables for different environments. For example, you might want to have different sets of variables for development and production. You can use the following naming convention in order to prepare different env variables for different environments.
.env # Loaded in all cases
.env.local # Loaded in all cases, but ignored by git
.env.[mode] # Only loaded in the specified mode
.env.[mode].local # Only loaded in the specified mode, but ignored by git
.env.development # Only loaded in development mode
.env.production # Only loaded in production mode
More specific environment files will always take priority, meaning if you have both a .env and a .env.development file, then .env.development will take priority over the more generic .env file.
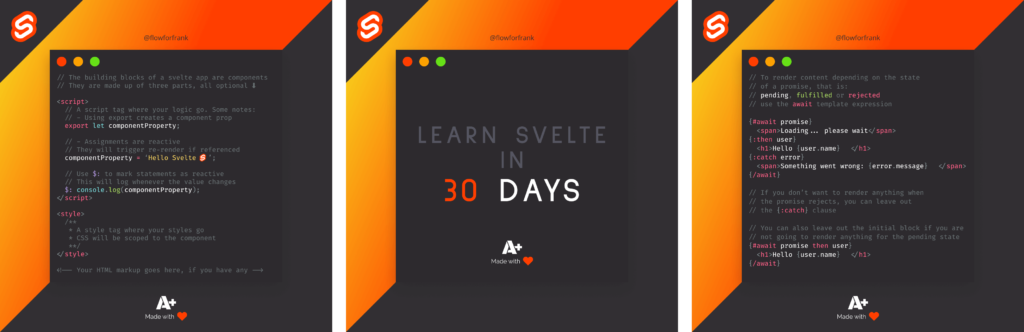
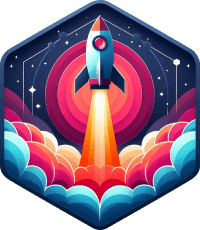
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: