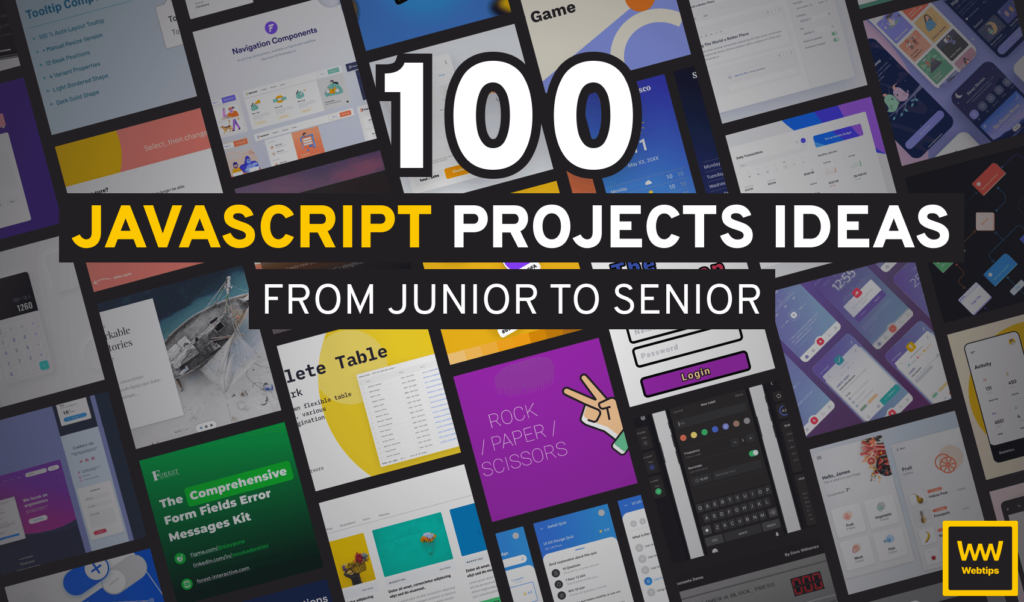
100 JavaScript Project Ideas
Letβs face it, you spend more time thinking about new project ideas than you should. Weβve all been there, whether you try to come up with something to practice a skill, challenge a candidate in an interview, or build the next billion-dollar app, weβve all spent some time thinking about project ideas.
In this article, Iβve collected 100 of them, so you can have an easier job choosing your next challenge. Iβve included designs for most of the projects so you donβt have to be a UX, and I will leave references to tutorials where appropriate, so you can cheat if you get stuck. Iβve also come up with a set of features that can act as acceptance criteria and listed some key takeaways so you know what can you learn by building each project.
With that being said, letβs see the 100 JavaScript project ideas, ordered by different types, such as small projects, apps, games, or components. Letβs start with the simple ones.
#1 β Create a To-Do App
By now, it would be a crime to leave out a to-do app from this list, so letβs quickly get this out of the way. The reason todo apps are so great for starter projects is that they have simple features, yet you can create a full-fledge, usable application in a matter of hours, while also learning key concepts in JavaScript. So what can you learn from this?
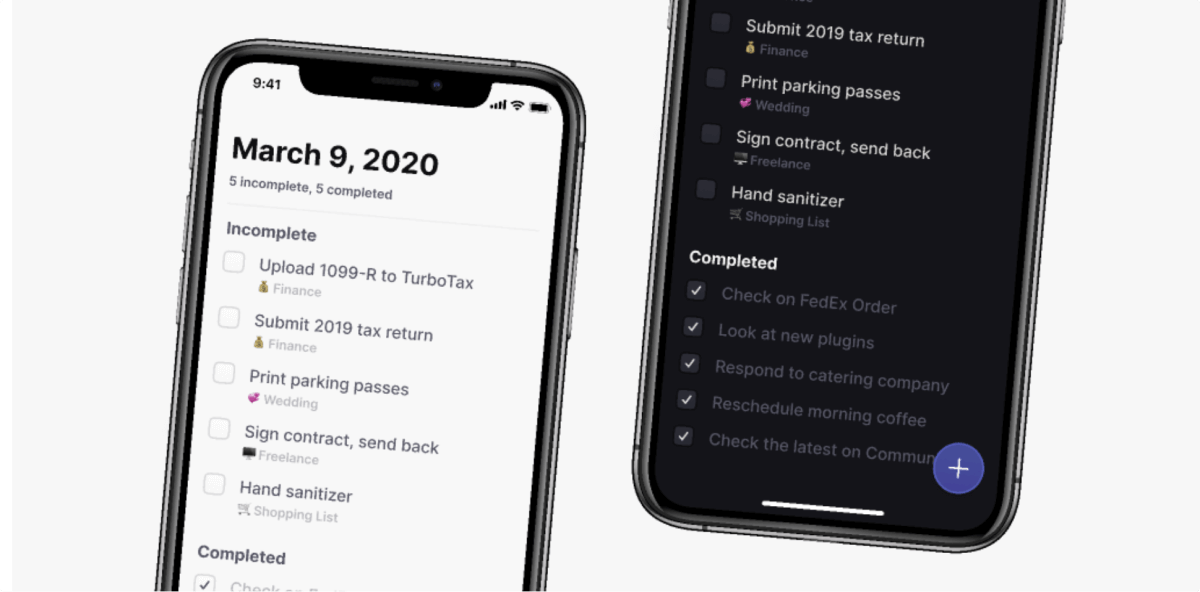
β¨ Features:
- Be able to add new elements to a list
- Be able to remove existing elements from a list
- Be able to rename existing elements in a list
- Be able to see the number of complete and incomplete elements
- Advanced:
- Be able to create separate lists
- Be able to tag and group elements
- Automatically sorting complete and incomplete elements
π§ You'll learn:
DOM manipulation
Interactions with checkboxes
For loops
Event listeners
π Resources:
#2 β Create a Counter
Building a counter is another great way to get started with JavaScript. Just as for the majority of the projects here, you can get familiar with DOM manipulation, as well as event listeners, and basic state management. You donβt need to worry about CSS too much, as we are more interested in the functionality here.
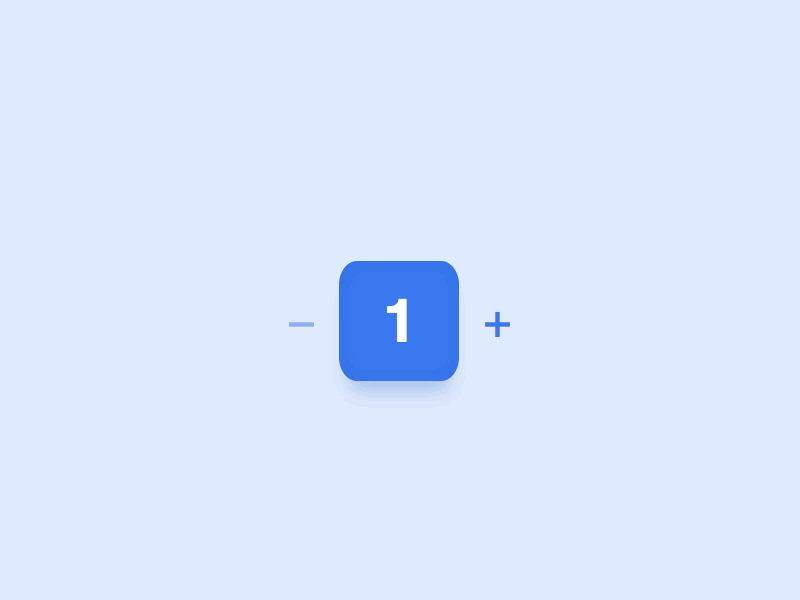
β¨ Features:
- Be able to increment and decrement a number
- Be able to directly input a number
- Advanced:
- Animate the number on change
π§ You'll learn:
State management
Change and click event listeners
Animations
π Resources:

#3 β Create a Calculator
Creating a simple calculator is yet another great way to get exposure to JavaScript. Here we are starting to have more and more functionality, meaning itβs a great starter project for learning how you can structure your code to make it more easily maintainable, scalable, and readable.
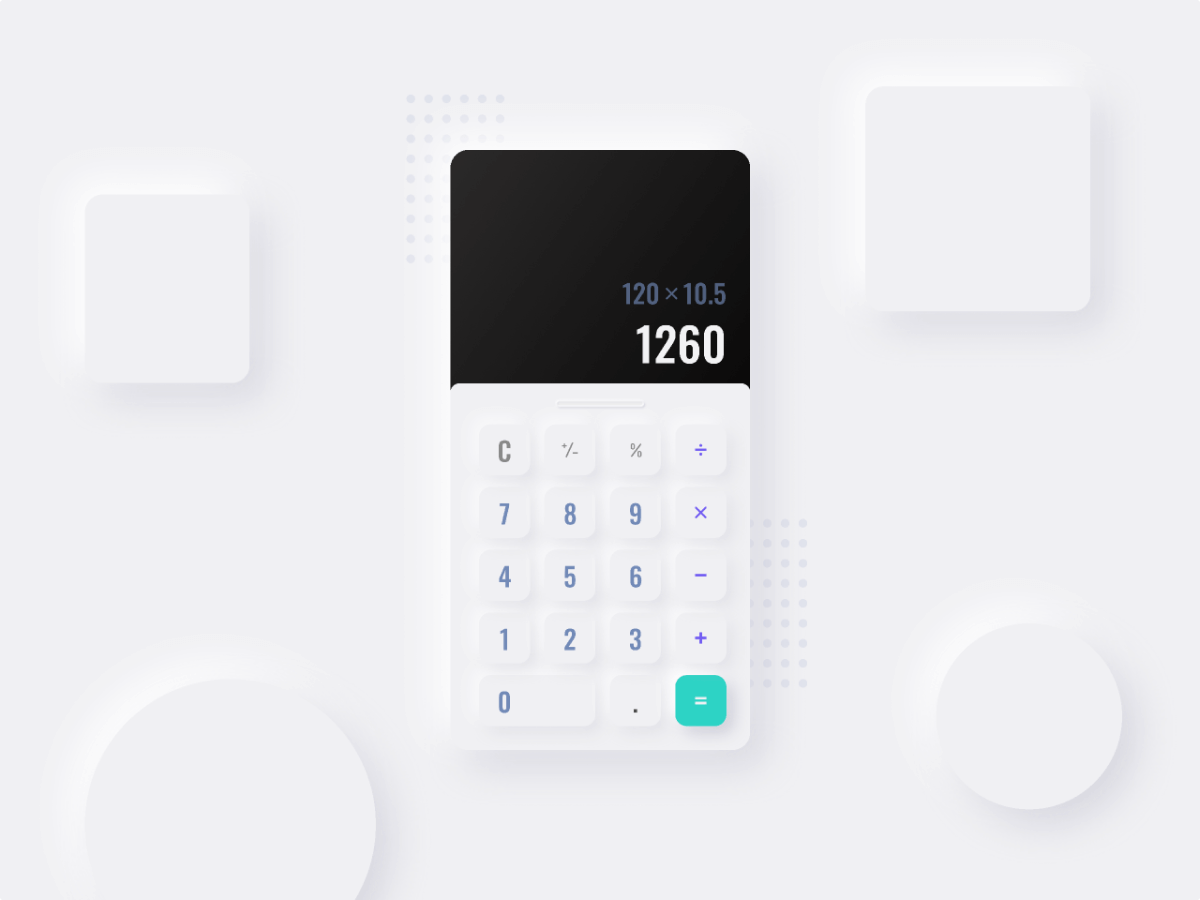
β¨ Features:
- Be able to add, subtract, divide, and multiply numbers together
- Be able to use decimals
- Be able to clear the result
- Advanced:
- Be able to use the modulus
- Be able to use exponentiation
- Be able to store calculation history
π§ You'll learn:
Classes and methods
Switch statements
Arithmetic operators
π Resources:
#4 β Create a Contact Form
A contact form lets you practice not just client-side JavaScript, but also server-side if you want to opt for the harder route. Of course, you can also create a functional contact form without having to worry about the backend, using only API calls.
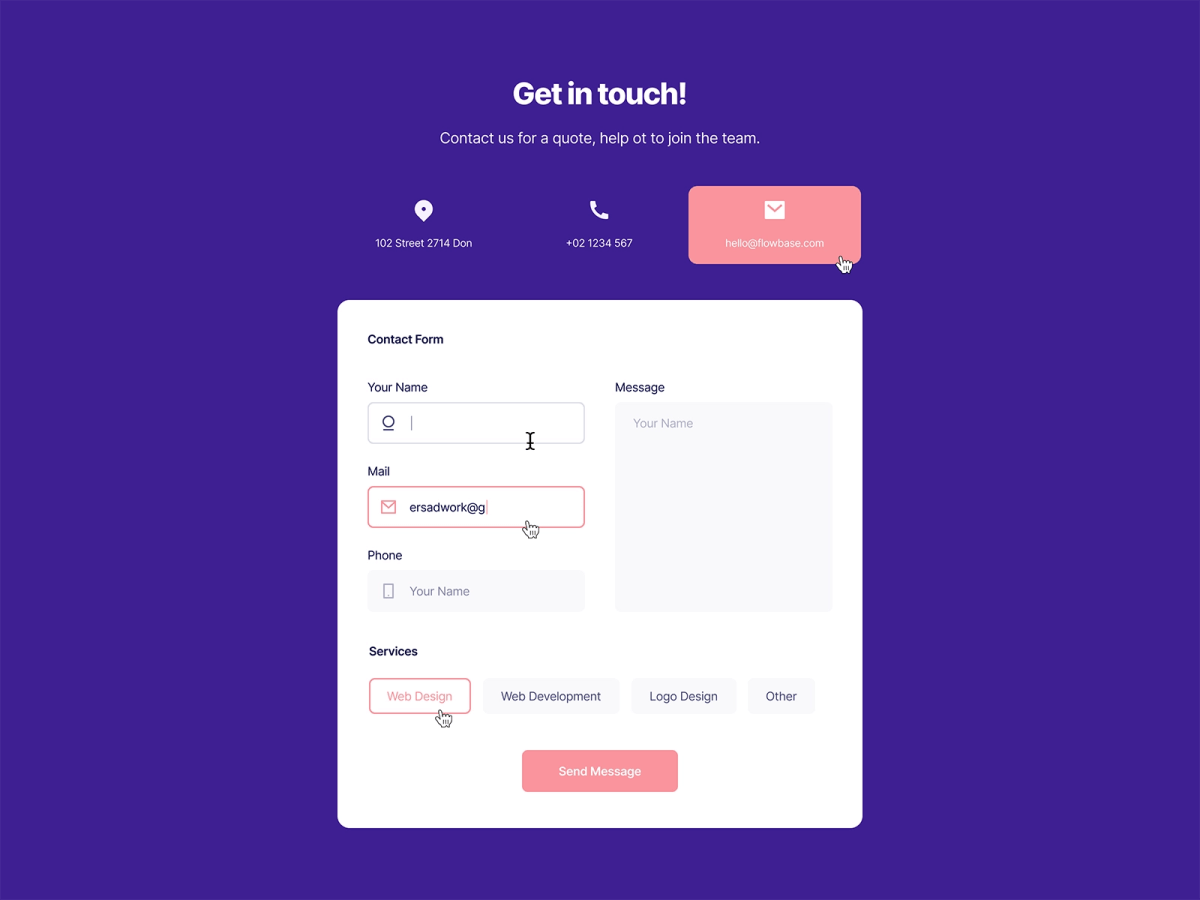
β¨ Features:
- Be able to fill out the contact form
- Validate that fields are not empty
- Validate that fields are valid
- Clear fields upon submission
- Be able to log the form values as a JSON object to the console
- Advanced:
- Be able to send an email with the details using an API call
π§ You'll learn:
Fetch API
Validating user input
Regex for input validation with pattern
Handling happy and failure paths from a promise
π Resources:
#5 β Create a Landing Page
Weβve yet to create a full webpage in one go, so as a next project, we can look into building a landing page. We can also integrate the contact form weβve built previously into the bottom of the page. Thereβs not so much JavaScript involved with the project, rather itβs about learning how to structure your HTML and layout your elements with CSS. But to get some JavaScript into the mix still, we can add smooth scrolling for anchors.
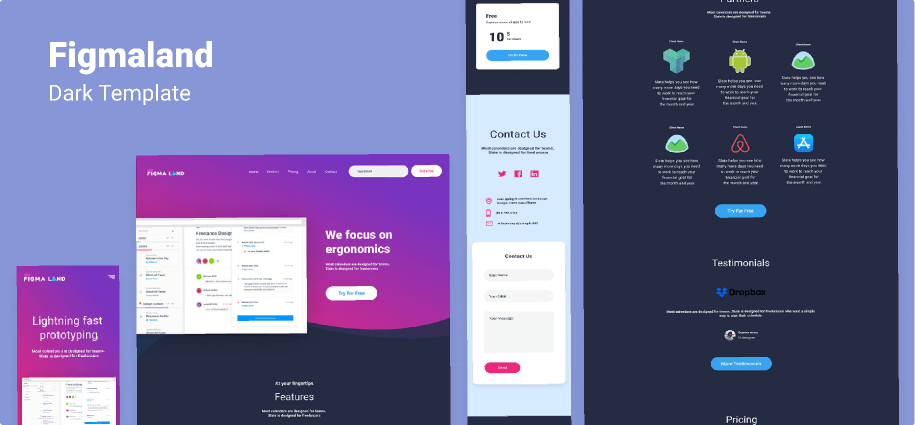
β¨ Features:
- Be able to smoothly scroll to other sections of the page with anchors
- Be able to resize the page without breaking the layout
π§ You'll learn:
Semantic HTML
Responsive CSS
Scroll events
π Resources:
#6 β Create a Toggleable Sidebar
Letβs take a look at some components now. Components can help you build reusable pieces of code, and they are just the right size in terms of complexity: they are small enough so you can practice key concepts in a short amount of time, but they are just large enough to give you some challenges while doing so. One of these projects is creating a toggleable sidebar.
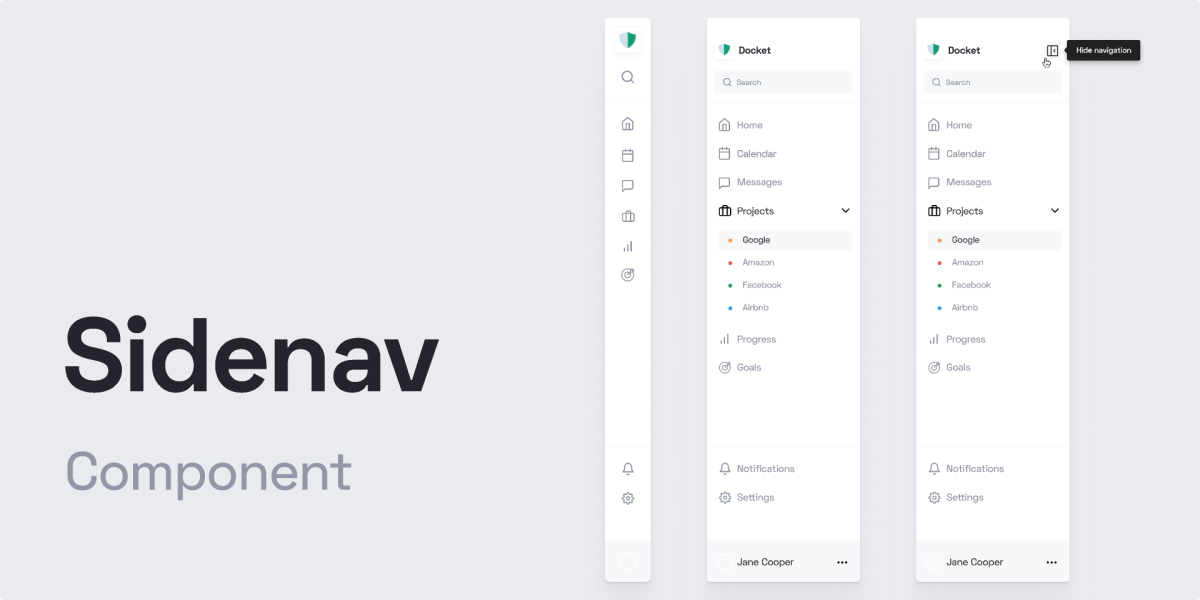
β¨ Features:
- Be able to toggle the sidebar on and off
- Be able to generate the menu items from a JavaScript object
- Advanced:
- Be able to navigate to different pages by interacting with the items
- Retains the selected state if a hash is present in the URL
π§ You'll learn:
Manipulating classes
Dynamically rendering elements
History API
Location object
π Resources:

#7 β Create a Tooltip Component
Another great challenge is to build a tooltip component. Tooltips can let you learn about hover effects, dynamically inserting content, and positioning. They should be positioned above the content. But should they also cover sticky elements, such as a fixed header?
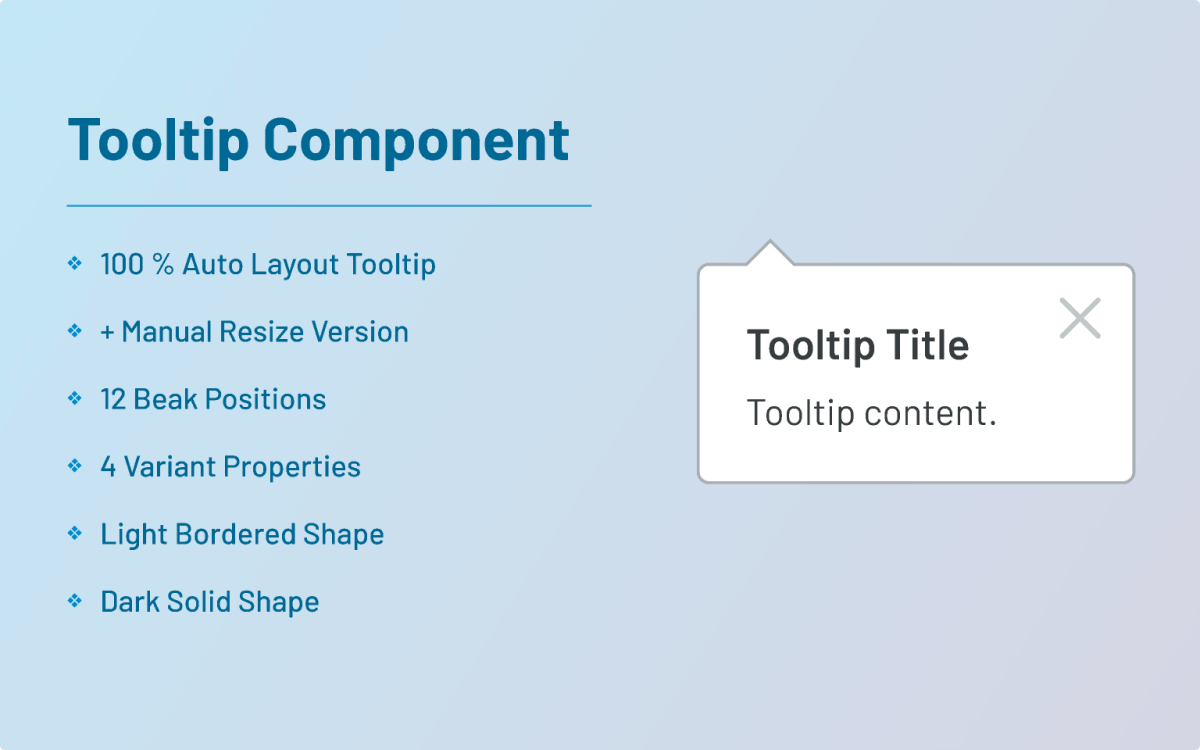
β¨ Features:
- Be able to create tooltips with a function call
- Be able to trigger tooltips on hover or on click
- Be able to close the tooltip on mouse leave or on close button click
- Be able to set the position of the tooltip anchor
tooltip({
selector: '.dom-element',
trigger: 'click',
position: 'top-left',
closeButton: true
});
π§ You'll learn:
Objects and methods
Layers and positioning
Dynamically inserting content
Mouse events
π Resources:
#8 β Create a Hamburger Menu
Building a hamburger menu is another great way to hone your JavaScript skills. You can combine this project with a sidebar component that can be toggled on and off using this menu. You can also throw in some animations into the mix if you would like to learn more about CSS too.
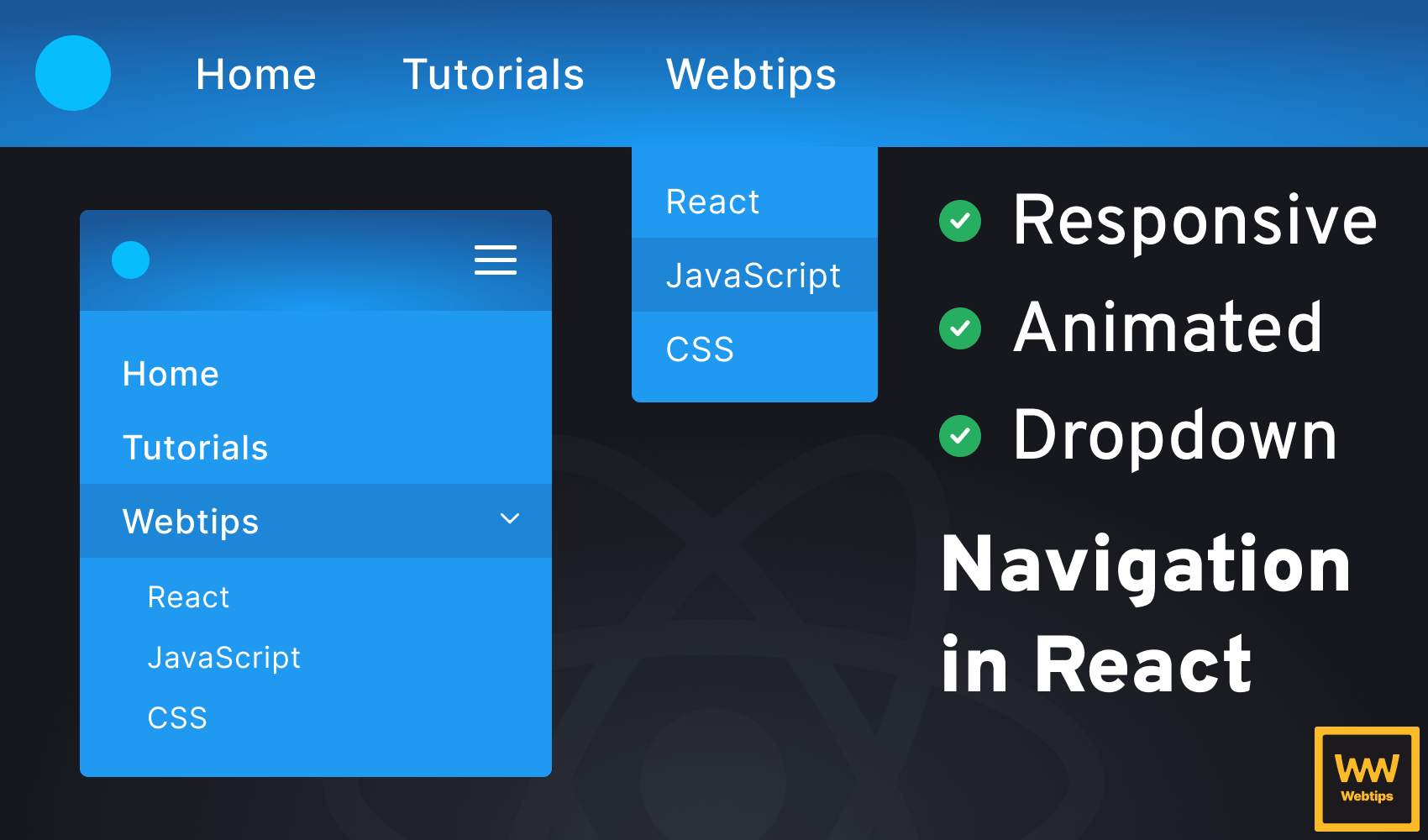
β¨ Features:
- Be able to toggle the state of a hamburger menu on and off
- The hamburger menu should be automatically closed when a menu item is interacted with
π§ You'll learn:
DOM manipulation
CSS animations
π Resources:
#9 β Create an Image Slider
An image slider is heavy in JavaScript, and if you want to make it customizable, then you have to go the extra mile. In this challenge, youβll learn about transitioning between elements and handling user interaction on desktop, as well as on mobile.
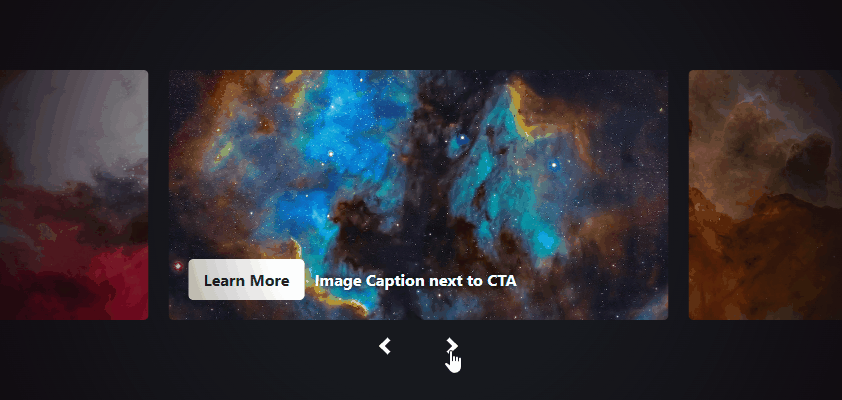
β¨ Features:
- Be able to navigate between images using dots or arrows
- Be able to swipe the images on mobile
- Be able to infinitely swipe to both sides
- Be able to dynamically define the slides
- Be able to choose between animations
slider({
selector: '.dom-element',
animation: 'slide',
arrows: true,
dots: true,
images: [...]
})
π§ You'll learn:
Animating elements
Handling swipes and click events
Working with function configurations
π Resources:
#10 β Create an FAQ Component
Next up, we have an FAQ component. FAQs are usually displayed in accordions which can act as a great base for learning how to toggle states in JavaScript. Itβs a simple, but fun project where you can also enhance your HTML and CSS skills.
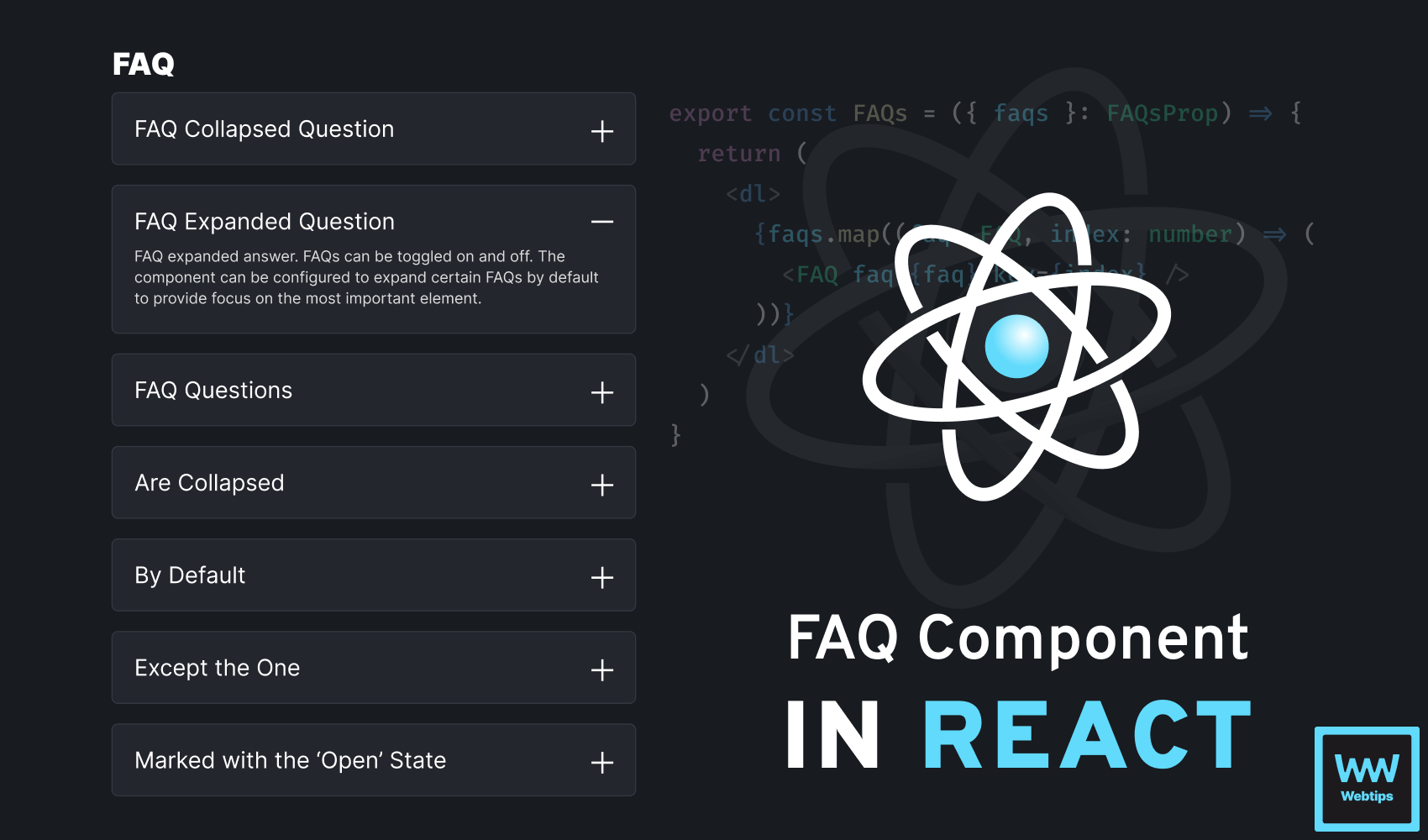
β¨ Features:
- Be able to toggle FAQs on and off
- Be able to automatically close opened sections if another section is toggled on
π§ You'll learn:
Handling on and off states
DOM Manipulation
CSS animations
Semantic HTML & Accessibility
π Resources:

#11 β Create a Customizable Modal
Designing and creating a customizable modal is another great way to get yourself in the habit of building reusable, flexible components that you can later take with you to other projects.
In this project, you will learn how to work with the dialog element and how you can use configuration objects to customize look and behavior. If you want to go the extra mile, you can also enable the use of callback functions for the buttons to execute custom events.
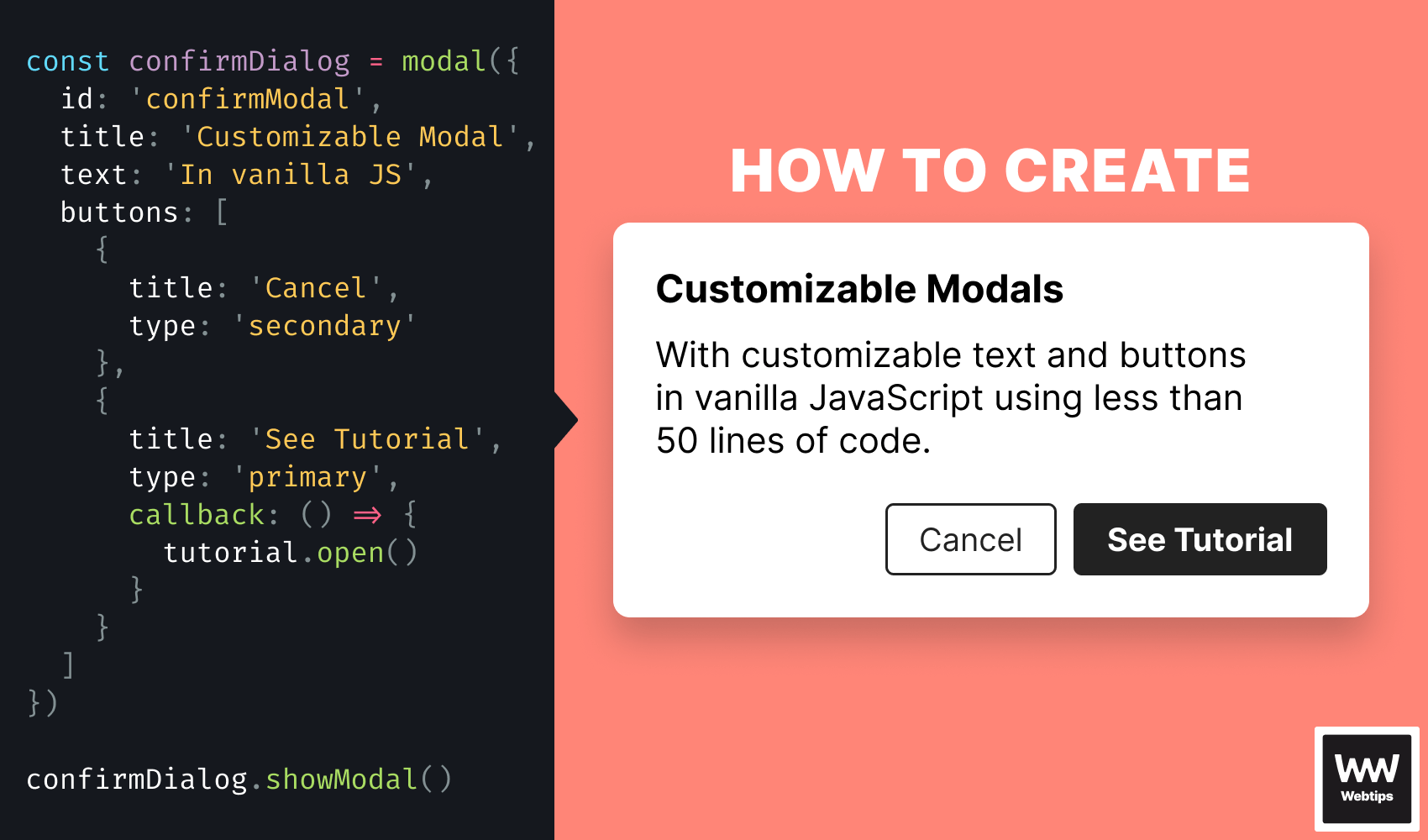
β¨ Features:
- Be able to define a header for the popup
- Be able to define a text for the popup
- Be able to define buttons with a callback function for the popup
modal({
id: 'confirmModal',
title: 'Are you sure?',
text: 'All your data will be deleted...',
buttons: [
{
title: 'Cancel',
type: 'secondary',
callback: () => { console.log('hide modal'); }
},
{
title: 'Yes, I\'m sure',
type: 'primary',
callback: () => { console.log('delete system32'); }
}
]
})
π§ You'll learn:
Event listeners
Custom data properties
Handling callback functions
Generating HTML elements
π Resources:
#12 β Create a Bookmarklet
If you rather want to improve your workflow while learnings JavaScript instead of building components, a great way to do that is to build a bookmarklet. A bookmarklet is like a bookmark that holds a piece of JavaScript code.
Once you click the bookmarklet, instead of opening a new page, you execute that code. You can implement various useful scripts this way, from highlighting outgoing links on the page to displaying extra information.
β¨ Features:
- Be able to append additional information to the DOM, based on elements on the page
π§ You'll learn:
Arrays
Querying and manipulating the DOM
Understanding how bookmarklets work
π Resources:
#13 β Create Tabs
Using tabs is a common way to organize, and display data. In this project, youβll learn about how you can switch out content by interacting with the tabs, and if you want to learn about the drag and drop API, you can also make the tabs draggable. Mixing this with a close button also lets you take a look at how to remove DOM elements completely.
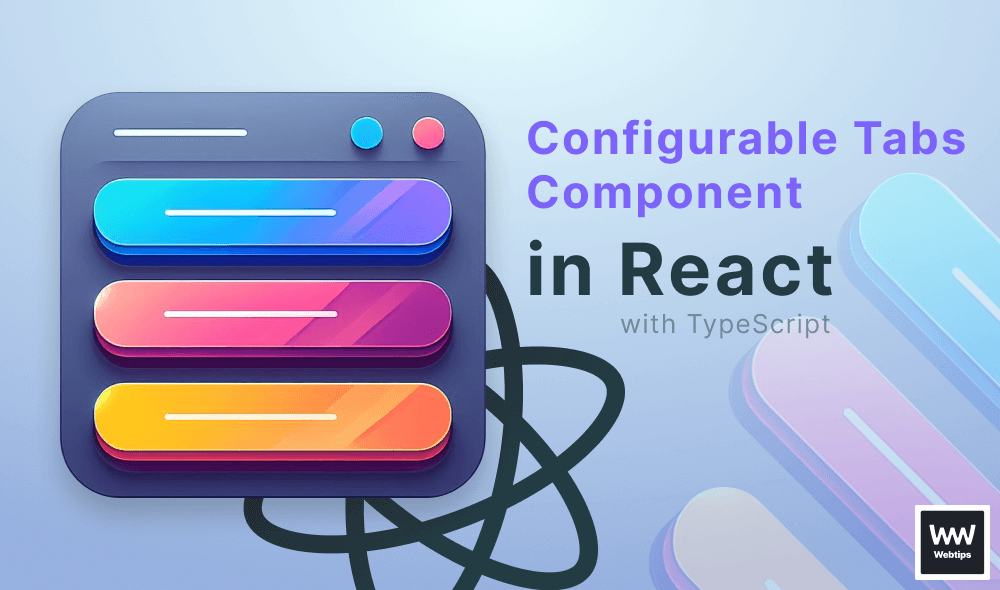
β¨ Features:
- Be able to navigate between tabs
- Advanced:
- Be able to close tabs
- Be able to order the tabs with drag and drop
π§ You'll learn:
DOM manipulation
Click events
Removing DOM elements
Drag and Drop API
π Resources:
#14 β Create a Download Progress Bar
Giving feedback to the user is one of your main jobs as a frontend developer. A download progress bar lets you do just that. Here you can learn more about promises, the different states they can take, the async/await
keywords, and how you can manage different states in order to reflect the current progress of a download or file upload on the UI.
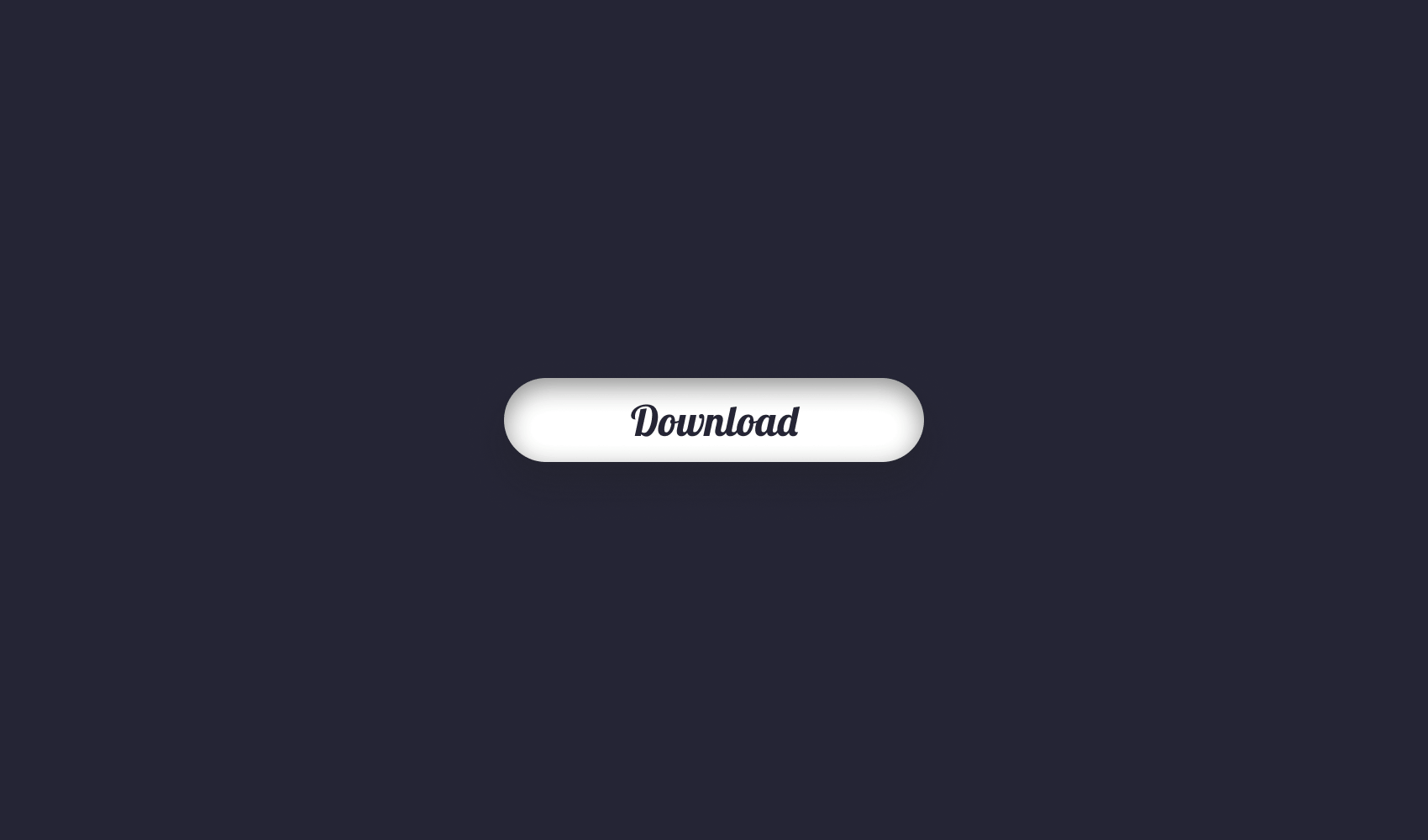
β¨ Features:
- Be able to see the progress of a file upload
- Be able to see the percentage of the progress
π§ You'll learn:
Promises
Async / Await
Axios
Managing state
π Resources:

#15 β Create a Form Validator
Form validation is another common task you will often find yourself dealing with. In this project, you can learn how to validate input fields, and give actionable error messages to users in case something goes wrong. You can also learn about regular expressions here, as they are often used to validate complex patterns. Always remember that you want to validate inputs on the server too.
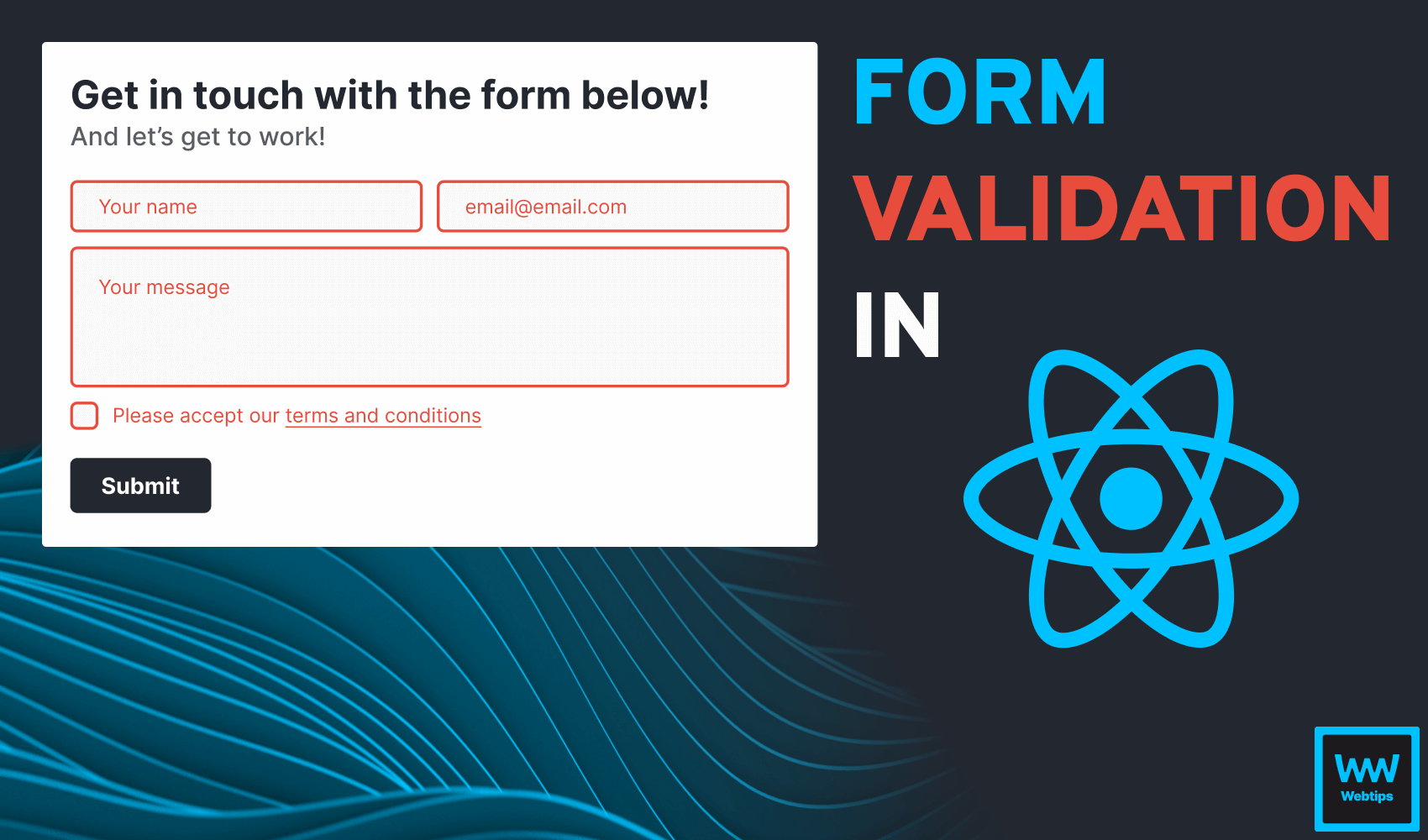
β¨ Features:
- Be able to set validation rules for an input
- Be able to show error state
const validationRules = {
name: /validation-rule-for-name/g,
email: /validation-rule-for-email/g,
message: /validation-rule-for-message/g
}
π§ You'll learn:
Handling form submissions
Querying the DOM
Input validation
Working with regex
π Resources:
#16 β Create a Calendar
Building a calendar can let you learn a lot about how to work with the Date API. Next to that, you can also learn how you can display data in a table, or even store it inside local storage if you want to assign time entries to days.
The design looks simple, but the implementation can quickly grow in complexity. How about adding different styles to holidays, or handling leap years?
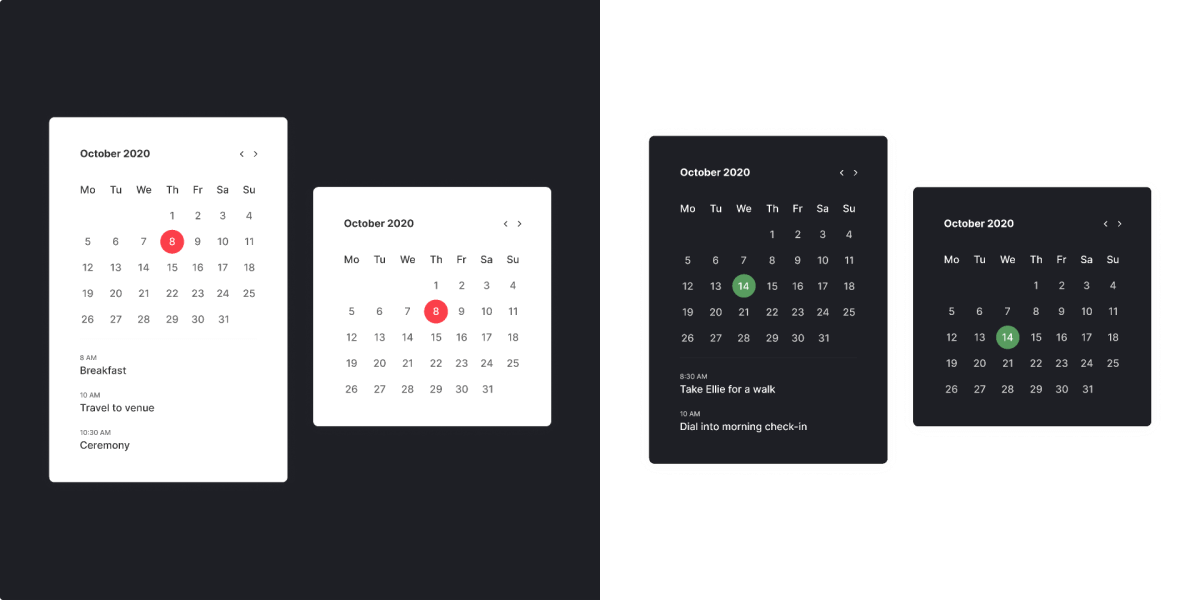
β¨ Features:
- Be able to navigate between months
- Be able to see today highlighted
- Advanced:
- Be able to set time entries on a day
- Be able to recall time entries from local storage
π§ You'll learn:
Tables and grids
Loops
Date API
Local Storage for storing time entries
π Resources:
#17 β Create a Timeline Component
Building a timeline is also a great challenge if you want to hone your CSS skills. By building this component, you can learn more about working with arrays and objects, loops, and learn about different array methods.
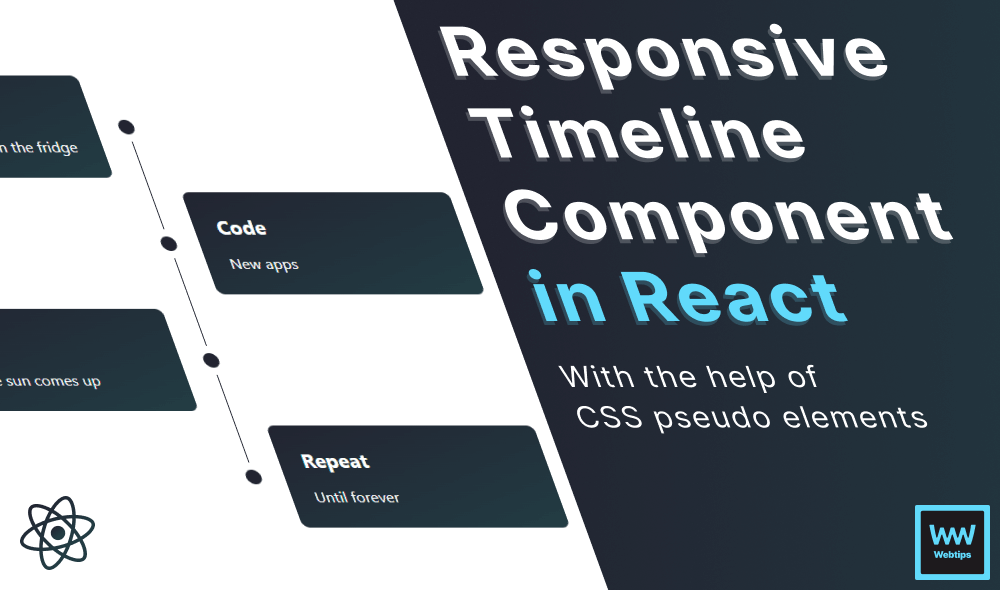
β¨ Features:
- Be able to generate a timeline from an array of objects
- Be able to set a title and text content for a timeline item
import { Timeline } from './Timeline'
export const App = () => {
const data = [
{
heading: 'Eat',
content: 'Everything you find in the fridge',
},
{ ... },
{ ... }
]
return <Timeline items={data} />
}
π§ You'll learn:
Array methods
Working with objects
Working with loops
Responsive CSS
π Resources:
#18 β Create an Event Countdown Component
Another great challenge you can do that is related to time, is building an event countdown component. With this, you can familiarize yourself with how the date API, and intervals work. If you would like to take the challenge a step further, make sure you are also able to call the timer with hours or minutes.
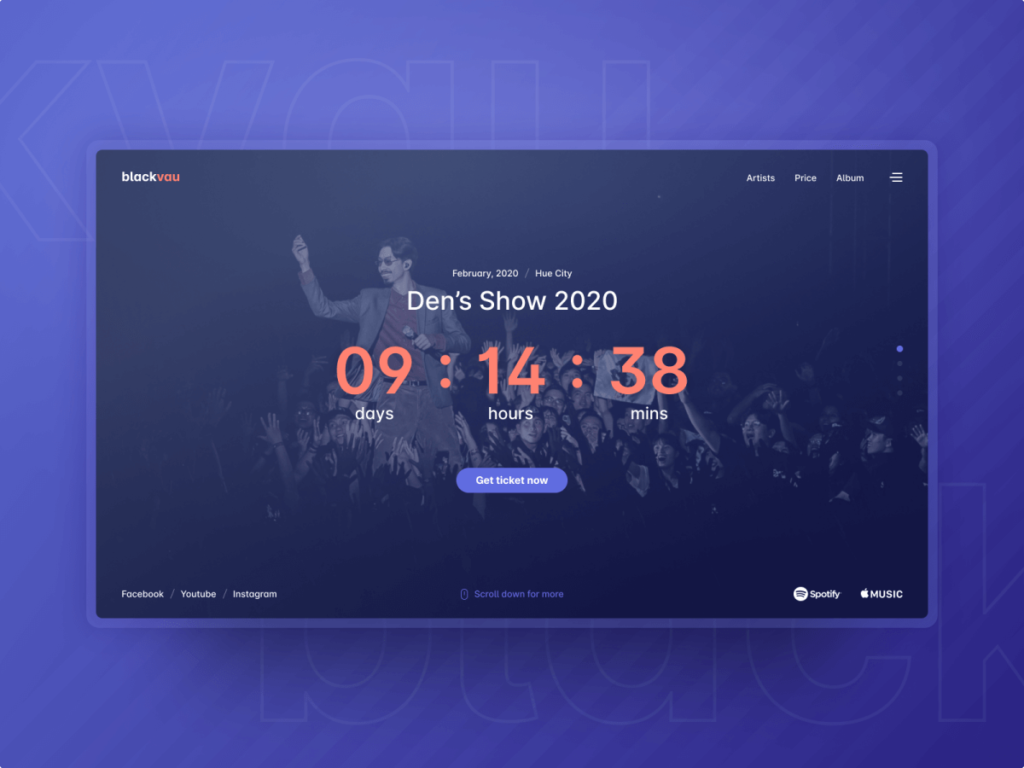
β¨ Features:
- Be able to call a function with the number of days that will display a countdown
- Be able to call the same function with the number of hours
- Be able to call the same function with the number of minutes
countdown(30); // Default to days
countdown(24, 'hours'); // Be able to use hours
countdown(30, 'minutes'); // Be able to use minutes
π§ You'll learn:
Date API
Intervals
π Resources:

#19 β Create a Star-rating Component
Using a star rating component is a common way to indicate votes or reviews. In this project, you will learn how to generate a number of icons based on an argument, as well as how to handle user interaction with these icons. If you also want to persist the data, you can look into using cookies or the local storage API.
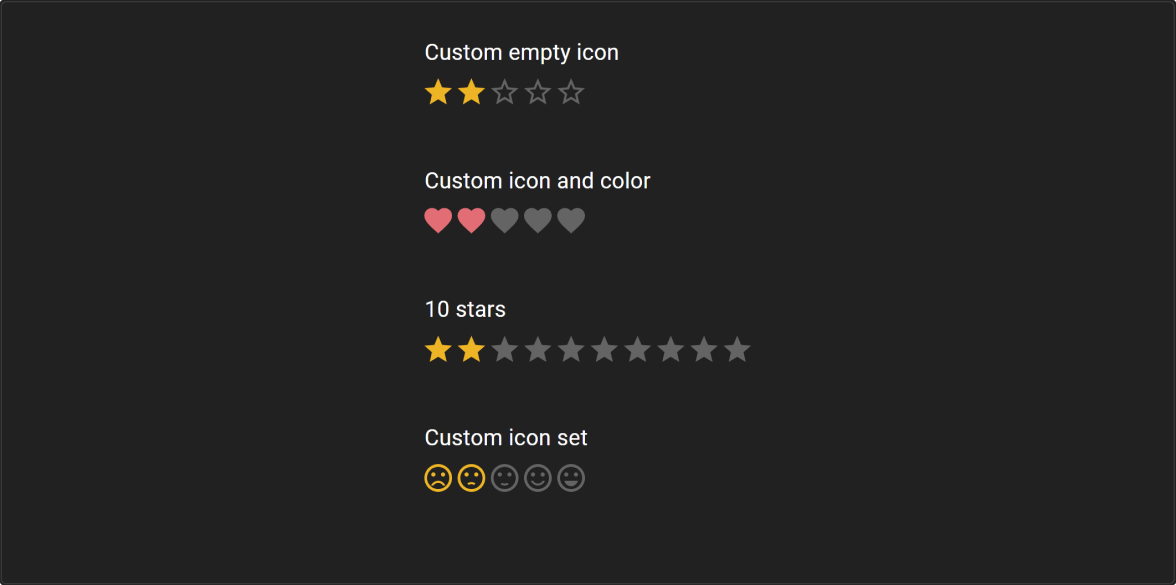
β¨ Features:
- Be able to interact with the star rating component
- When a star is clicked, it should retain state even after page reload
- Advanced:
- Be able to set a custom icon for the component
starRating(5); // Generates a 5-star rating component
starRating(10); // Generates a 10-star rating component
starRating(5, 'hearts'); // Use predefined icon
starRating(5, 'https://location.to/image/path.png'); // Use custom image
π§ You'll learn:
Working with loops
Persisting state through cookies / local storage API
π Resources:
#20 β Create a Breadcrumbs Menu
Showing navigation as breadcrumbs is a common way to visualize the relationship between pages. Building this component helps you dive into working with arrays and objects, as well as looking at generating navigation elements based on the JavaScript data.

β¨ Features:
- Be able to get a breadcrumb menu from an array of objects
- Be able to navigate to pages using the menu items
breadcrumbs([
{
menu: 'Home',
link: '/'
},
{ ... },
{ ... } // The last item - which is the current page - should not have a link
]);
π§ You'll learn:
Working with arrays and objects
Working with loops
Generating navigation elements through JavaScript
π Resources:
#21 β Create a Stepper Component
A stepper, or a multi-step form is a way to indicate the progress of filling out a form through a list of actions. With this project, you can learn how to manage an internal state, and work with object methods to externally update the component and trigger a change in steps.
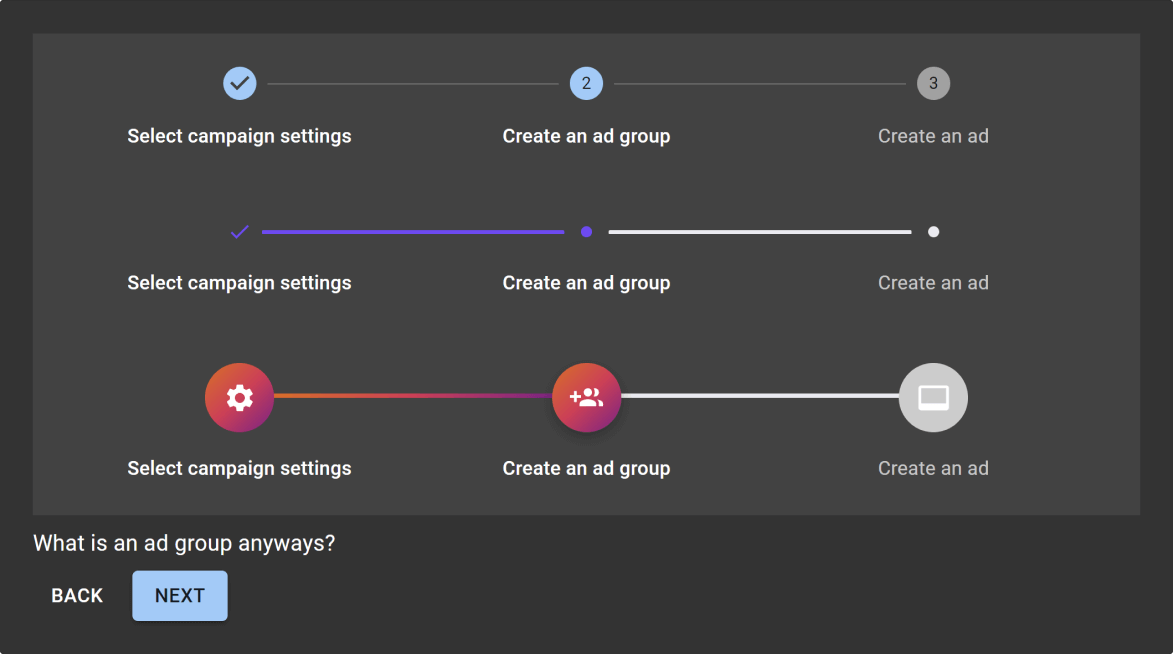
β¨ Features:
- Be able to define a list of steps with a name
- Be able to set a complete state of a step
- Be able to trigger a state update for the component
const stepperComponent = stepper({
selector: '.dom-wrapper',
steps: [
{
name: 'Select campaign settings',
completed: true
},
{
name: 'Create an ad group',
completed: false
},
]
});
stepperComponent.next(); // Trigger the component to move to the next step
stepperComponent.previous(); // Trigger the component to move to the previous step
π§ You'll learn:
Managing state
Working with methods
DOM manipulation
π Resources:
#22 β Create a Chips Component
Chips let you quickly assign predefined tags to an input. It works similarly to an autosuggest, but here, the input can take on multiple values. To make the project a little bit more complicated, you can also look into adding a remove button for the chips or let them have different states, eg.: make them clickable, create primary / secondary styles, or adding custom icons to them.
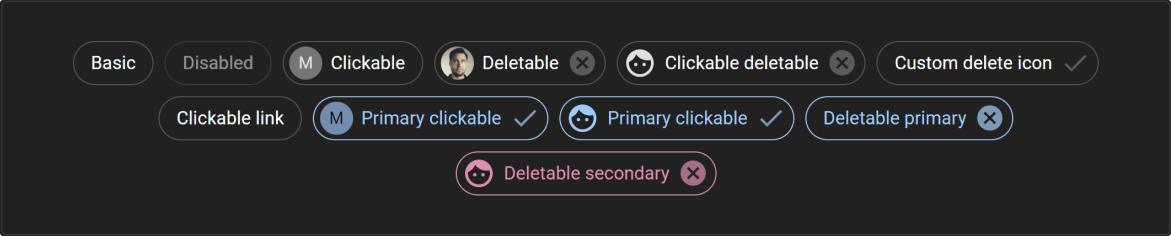
β¨ Features:
- Be able to add chips to an input field
- Be able to remove existing chips from the input field
- Advanced:
- Be able to set the chips to be either primary or secondary in style
- Be able to set a custom icon for the chips
- Be able to attach an
onChange
event listener to the component
chips({
selector: '.input-field',
value: ['chip1', 'chip2', 'chip3'],
items: ['remaining available items for selection', ...],
style: 'primary',
icon: 'icon/path'
onChange(value) { console.log('value changed to...'); }
});
π§ You'll learn:
Working with input fields
Click events
On change events
Working with loops
π Resources:

#23 β Create a Drop Down Menu
Using a drop-down menu is a common way to handle multi-level navigation. With this project, you can learn about correct positioning and displaying elements on hover. Want to go a bit more advanced? Try to integrate some sliding animation between navigating sub-menus, and you can also play with recursion if you want to implement a multi-level drop-down menu.
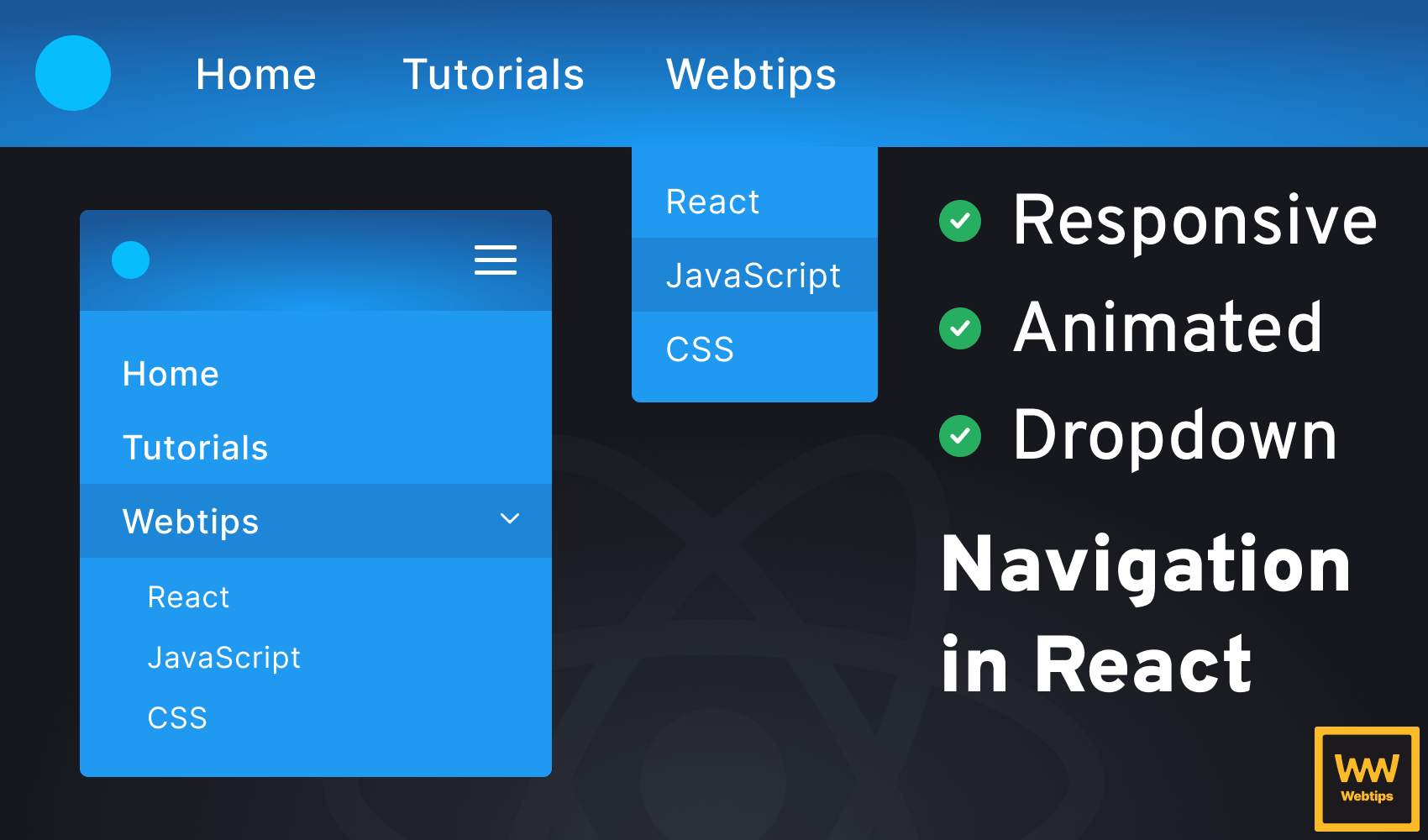
β¨ Features:
- Be able to see a drop-down menu when a top-level menu item is being hovered
- Be able to navigate to different pages when a sub-menu item is clicked
- Be able to get a multi-level drop-down menu from an array of JavaScript objects
π§ You'll learn:
Hover events
Animations
Recursion
π Resources:
#24 β Create an Autocomplete Search
Weβve looked at working with chips before, but thereβs also a similar component we havenβt touched yet. Creating an autocomplete search is a great way to learn more about how you can search through a dataset and filter it based on some user input.
With this project, you will learn a lot about array methods, and if you want to add some extra challenge, make sure you add support for keyboard navigation too.
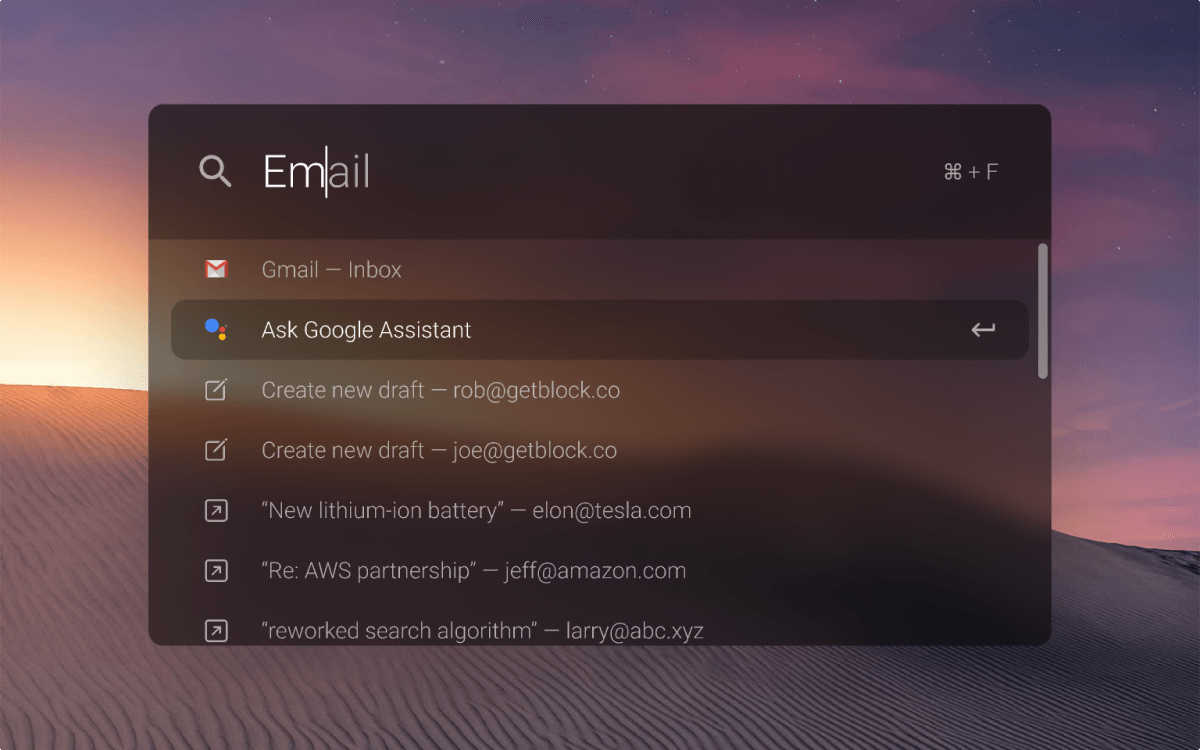
β¨ Features:
- Be able to filter an array of JavaScript objects based on an input field
- Be able to see autosuggestions on the filtered resultset
- Be able to select an element from the list of suggestions using the keyboard or mouse
π§ You'll learn:
Click event listeners
Keyboard event listeners
Searching and filtering data
π Resources:
#25 β Create a Pagination Component
Dealing with paginations is a common task that you will probably come across at one point in your career. This project can help you understand how can you break down a large set of data into smaller, more digestible pieces. How to offset the displayed elements, and how to set a limit, so only a predefined number of elements are rendered on the page at any given time.
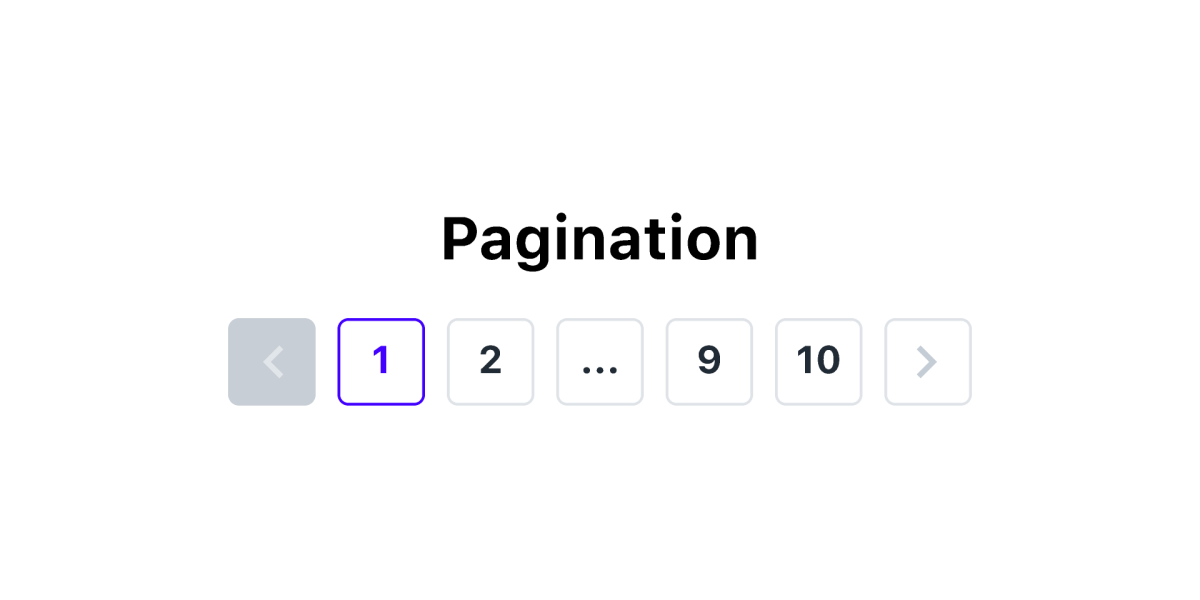
β¨ Features:
- Be able to break down an array into separate pages
- Be able to paginate between the items of the array
- Be able to use arrows to navigate to the next and previous page
π§ You'll learn:
Pagination
Arrays
Working with internal state
π Resources:
#26 β Create a Sortable and Filterable Table
Building upon the previous project, we can go one step further, and look at building a sortable and filterable table with a pagination component on the bottom. Along the way, you can deepen your knowledge on pagination, and also gain new skills on how to work with tabular data, or sort and filter a resultset.
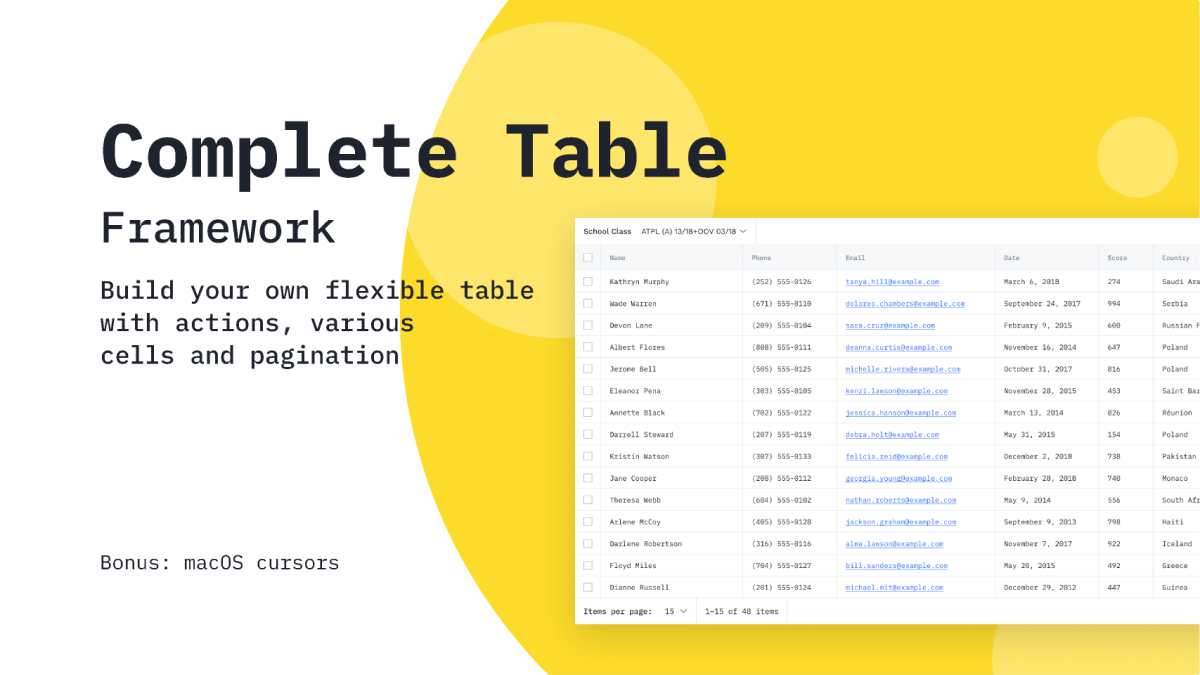
β¨ Features:
- Be able to see an array of JavaScript objects displayed in a table
- Be able to sort and filter the table
- Be able to paginate the data if the table has more than 10 elements
- Advanced:
- Be able to search the dataset through an input field
π§ You'll learn:
Array methods
Sorting and filtering
Pagination
π Resources:

#27 β Create a Blog From Scratch
Building a blog as a side project is a great way to get familiar with a handful of things. With the help of this project, you will be able to learn the basics of navigation, displaying data from an external source, working with grids, and paginating large data sets. It puts together multiple smaller projects into one big challenge you can take on.
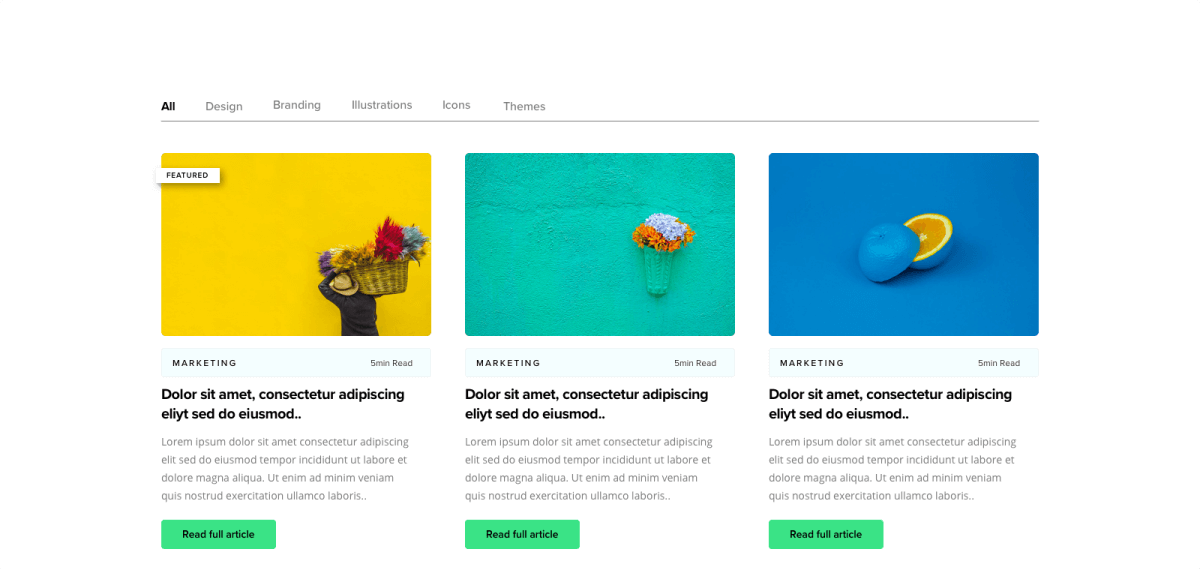
β¨ Features:
- Be able to see a list of posts (their cover image, title, excerpt, and CTA) displayed in a grid that is fetched from an API
- Be able to navigate to the articles by clicking on them
π§ You'll learn:
Routing
Grids
Pagination
Working with APIs
π Resources:
#28 β Create a Parallax Effect
Letβs take a look at some animations too. A parallax effect is a great way to illustrate the depth of elements on a page. It is triggered by scrolling, and if executed right, it can create a captivating effect. With this project, you can learn more about scroll events, how different layers can work together, and how translations can help you achieve it.
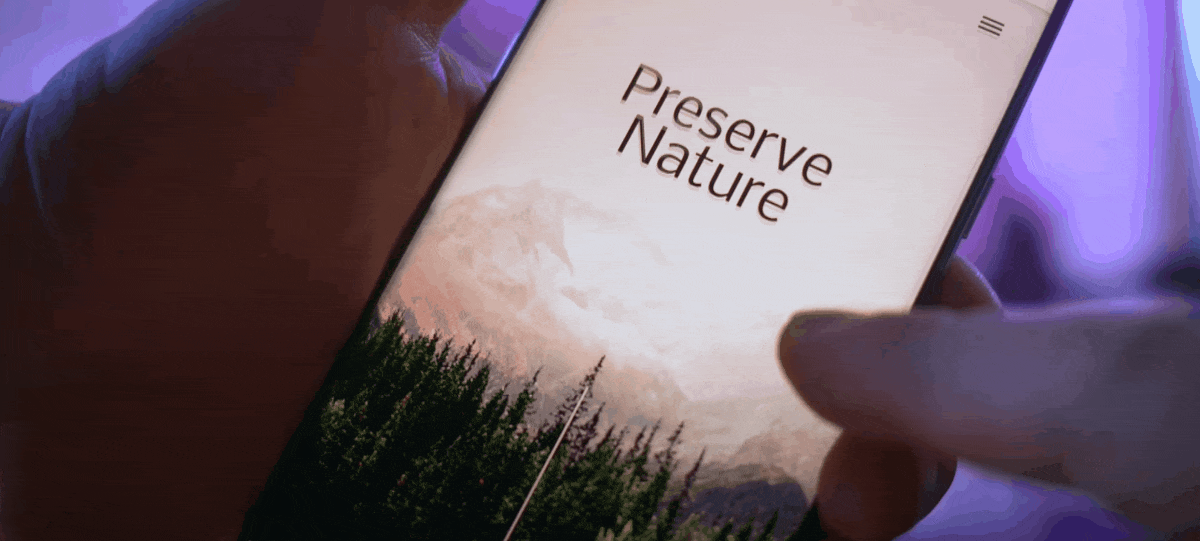
β¨ Features:
- Be able to see a parallax effect as I scroll down the page
π§ You'll learn:
Scroll events
Layers and translations
Data attributes
π Resources:
#29 β Create an Infinite Scroll Page
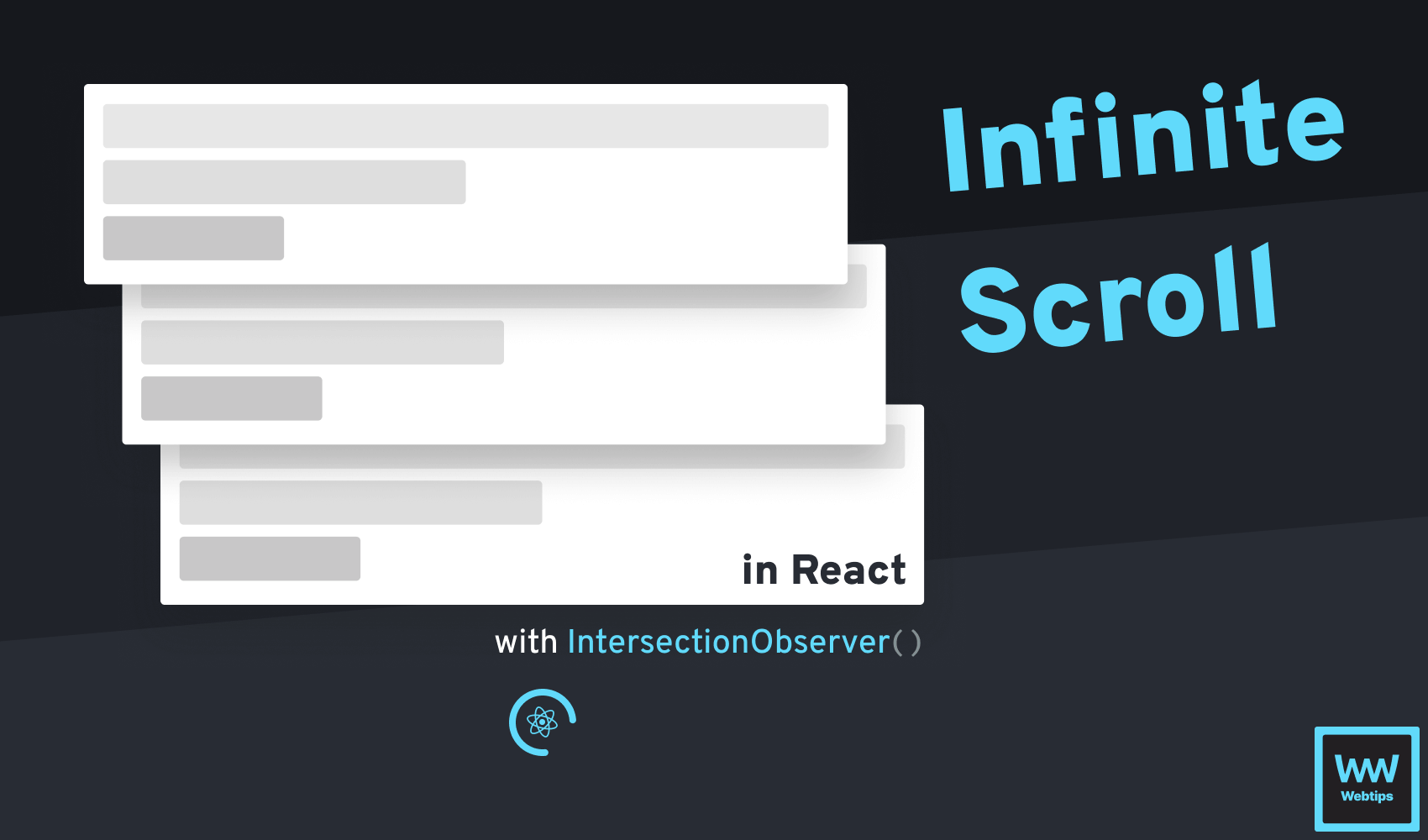
Just like pagination, infinite scroll works on the same logic, however, here we donβt have to interact with anything. As we scroll down a page, the next page is automatically loaded in after the other, creating the illusion that we can infinitely scroll down.
If you didnβt get pagination right for the first time, here you can learn more about it, how to work with scroll event listeners, and how to append elements to the DOM after requesting the next page from an API.
β¨ Features:
- Be able to infinitely scroll down the page
- Be able to see a loading indicator while the next page is fetched
- Be able to see a βYouβve reached the endβ message at the bottom of the page when there are no more pages to fetch
π§ You'll learn:
Pagination
Appending elements to the DOM
Scroll event listeners
IntersectionObserver API
π Resources:
#30 β Create a Scroll-animated Site
While we are talking about scrolling, another fun project to take on is building a site that is animated by the position of your scrollbar. With this project, you can get familiar with how to manipulate the CSS properties of different elements to add animations on scroll.
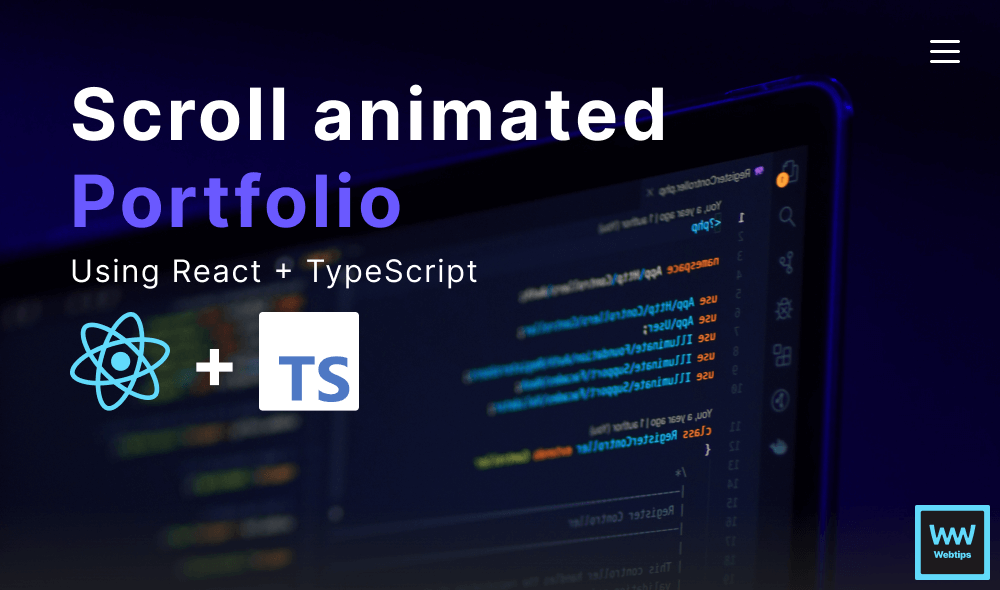
β¨ Features:
- Be able to control the style and position of elements on the page based on the position of the scrollbar
- Be able to fade elements in and out based on the scroll position
- Be able to manipulate CSS properties dynamically
π§ You'll learn:
Scroll event listeners
CSS Animations
Transitions and transforms
π Resources:

#31 β Create a Shopping Cart
Are you looking to learn how to build an e-commerce site? Then this project will be for you. A shopping cart is an integral part of any online store that connects your way from browsing products, to checking out. With the project, you can learn how to add and remove products from a cart, and how to display a sum based on the prices and quantity of the products inside the cart.
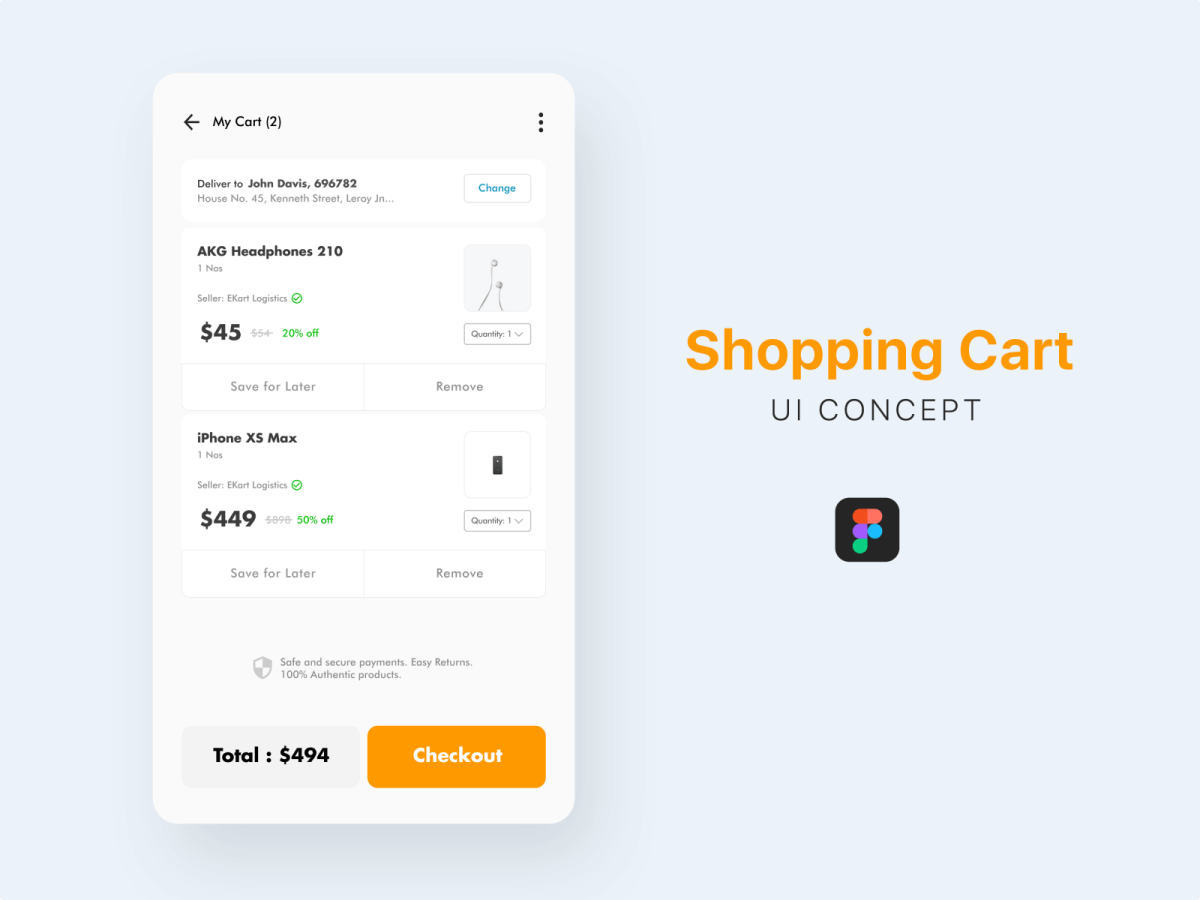
β¨ Features:
- Be able to add products to the cart
- Be able to remove products from the cart
- Be able to see the productβs name, quantity, and price inside the cart
- Be able to see the total price of the cart
π§ You'll learn:
DOM manipulation
Working with objects
State management
Cookies for storing cart information
π Resources:
#32 β Create a Color Generator
Next up, we have a color generator. Generating random colors in JavaScript can be done in a quick and straightforward manner. But what about generating shades of color, or generating entire color palettes?
Here you can learn about how to convert numbers into hexadecimal values, how to deal with random numbers, or how to copy strings to the clipboard for later use.
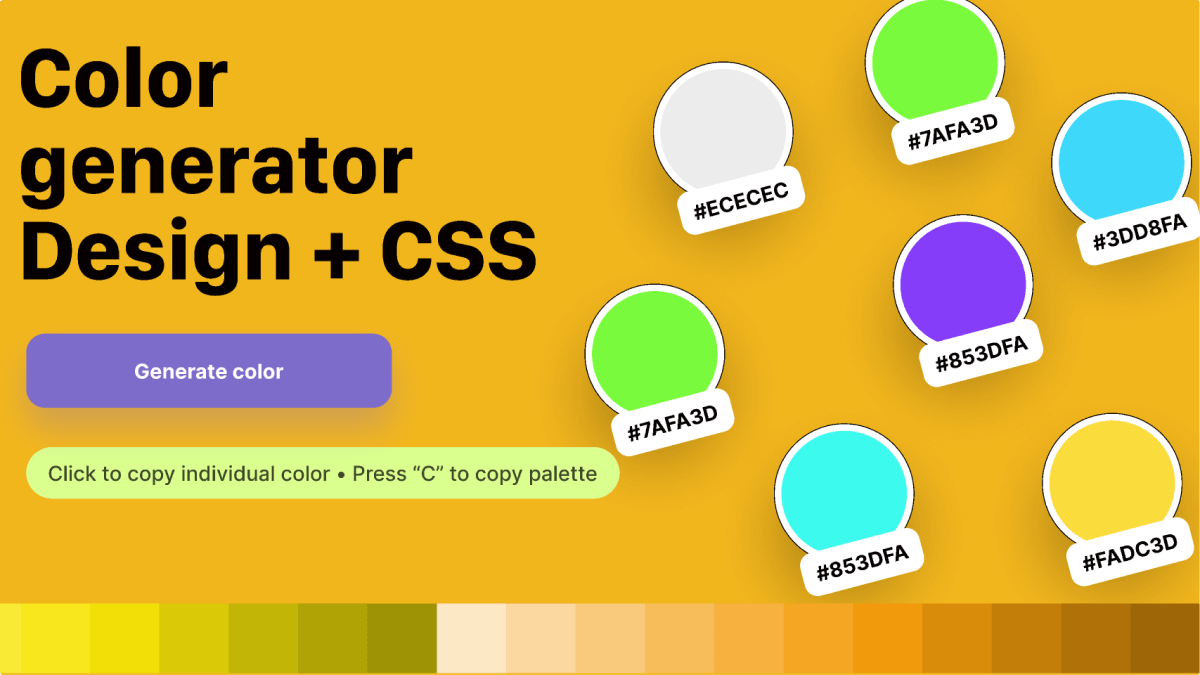
β¨ Features:
- Be able to randomly generate a color
- Be able to see the hex color code of the generated color
- Be able to copy the color code to the clipboard
- Advanced:
- Be able to generate x number of shades of a color
- Be able to generate color palettes
π§ You'll learn:
Working with
Math.random
Converting numbers to hexadecimal values
Copy to clipboard functionality
π Resources:
#33 β Create Feature Flags
Feature flags let you quickly test out new features in a production environment, and even turn off existing features, without having to deploy additional code changes.
With this project, you can learn more about how to work with the local storage API, how to toggle the state of objects on and off, and how to display features based on these states. You will also learn how you can quickly build a bookmarklet β like the one in the image below β that you can share with others to use in order to manage the features through an interface.
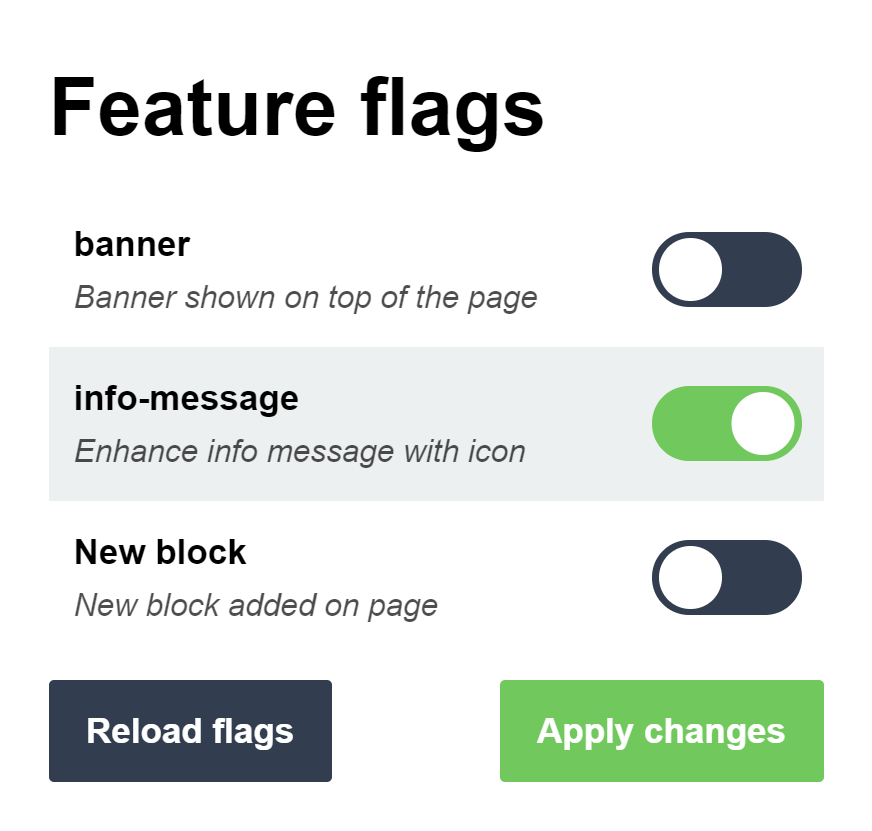
β¨ Features:
- Be able to toggle features on and off from an interface
- If a feature is toggled on, it should appear on the page
- If the feature is toggled off, it should either render a fallback or nothing
π§ You'll learn:
Local storage API
Working with toggles
How to create a bookmarklet
π Resources:
#34 β Create an Exit-Intent Popup
Building an exit-intent popup is a great way to get your audience to subscribe to your mailing list, but itβs also a great way to learn more about mouse and keyboard events. With this project, you will learn how to trigger a popup right when the mouse is about to leave the page, how to close the popup in three different ways, and how to work with cookies in order to prevent the popup from appearing again for every session.
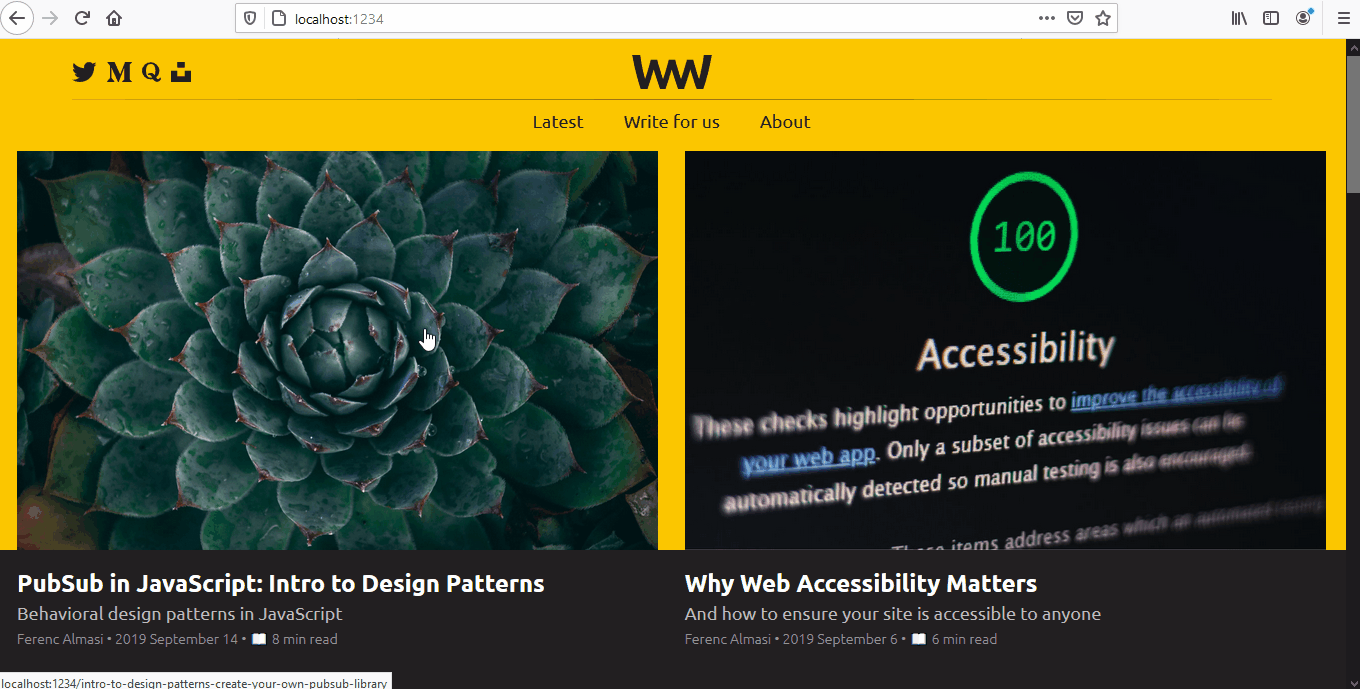
β¨ Features:
- Be able to trigger a popup on mouse leave
- Be able to close the popup with an x icon
- Be able to close the popup by clicking away
- Be able to close the popup by hitting the escape key
- Once the popup is triggered, it should not trigger again for the next 30 consecutive days
π§ You'll learn:
Mouse event listeners
Key event listeners
Cookies
π Resources:

#35 β Create an Animated Day and Night Toggle Switch
Do you want to make a dark theme for your app? You can look into implementing a day and night toggle switch for it to create a transition between the two themes using CSS, and some JavaScript for toggling the necessary classes, and creating a similar effect:
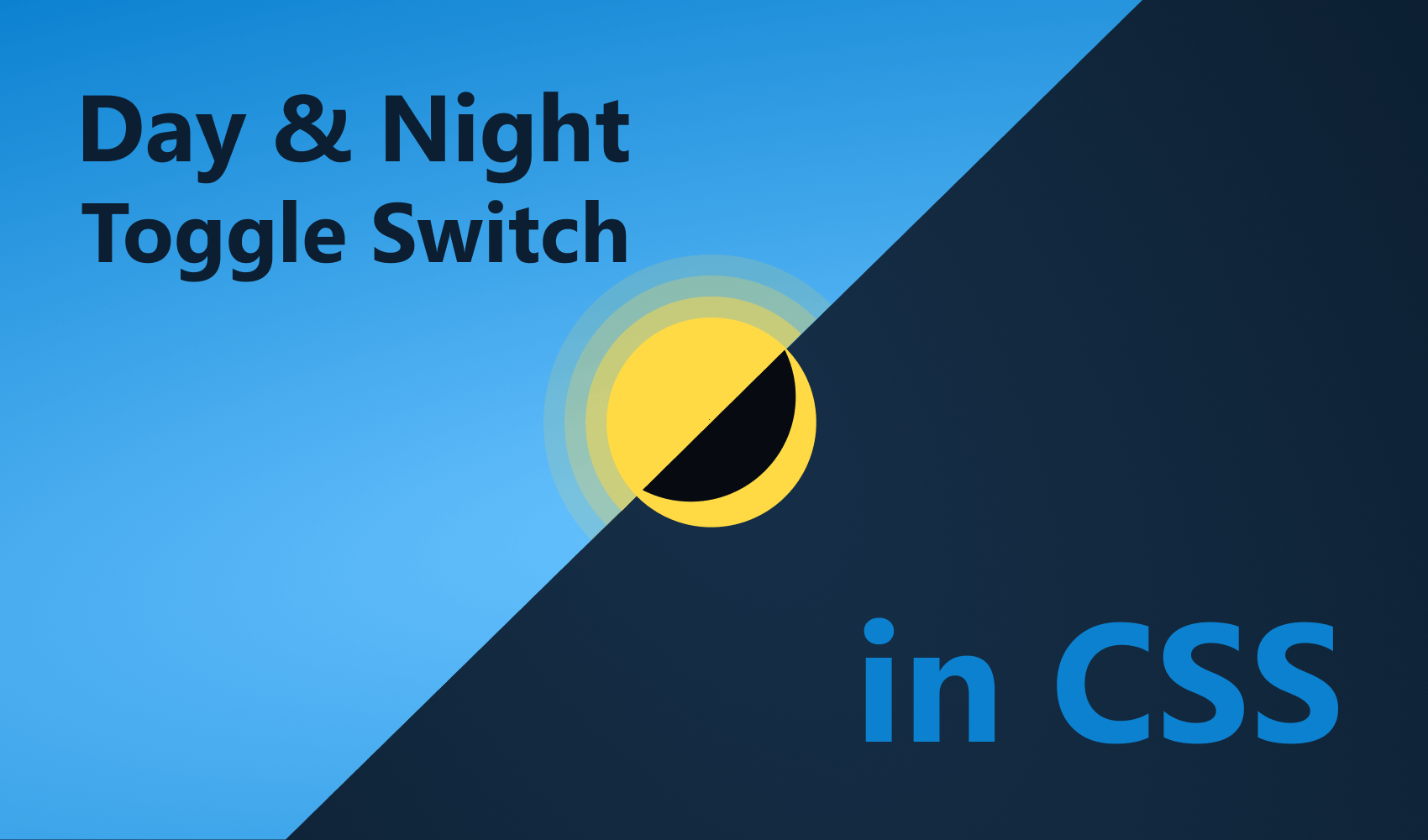
β¨ Features:
- Be able to toggle between a light and dark theme with a ripple effect
π§ You'll learn:
CSS transitions
Toggling states
π Resources:
#36 β Create a Network Status Notification Bar
A network status notification bar can help your users see status changes in their network connection. It comes especially useful if your app depends on a saving function, and suddenly the connection is dropped. You can let users know in this case that any changes made during this period are bound to be lost.
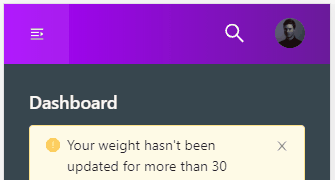
β¨ Features:
- Be able to see a banner when the network status changes to offline
- Be able to queue changes while being offline
- Be able to sync the queued changes after going online again
π§ You'll learn:
Detecting changes in network status
Queueing updates when being offline
Syncing with a database when going online
π Resources:
#37 β Create a Chrome Extension
If you want to look into enhancing your workflow, and a bookmarklet doesnβt suit you, then building a custom Chrome extension might help you with that. With this project, you will learn about the basic structure of a Chrome extension: how to create a custom popup, how to work with permissions and content scripts, and how to test everything.
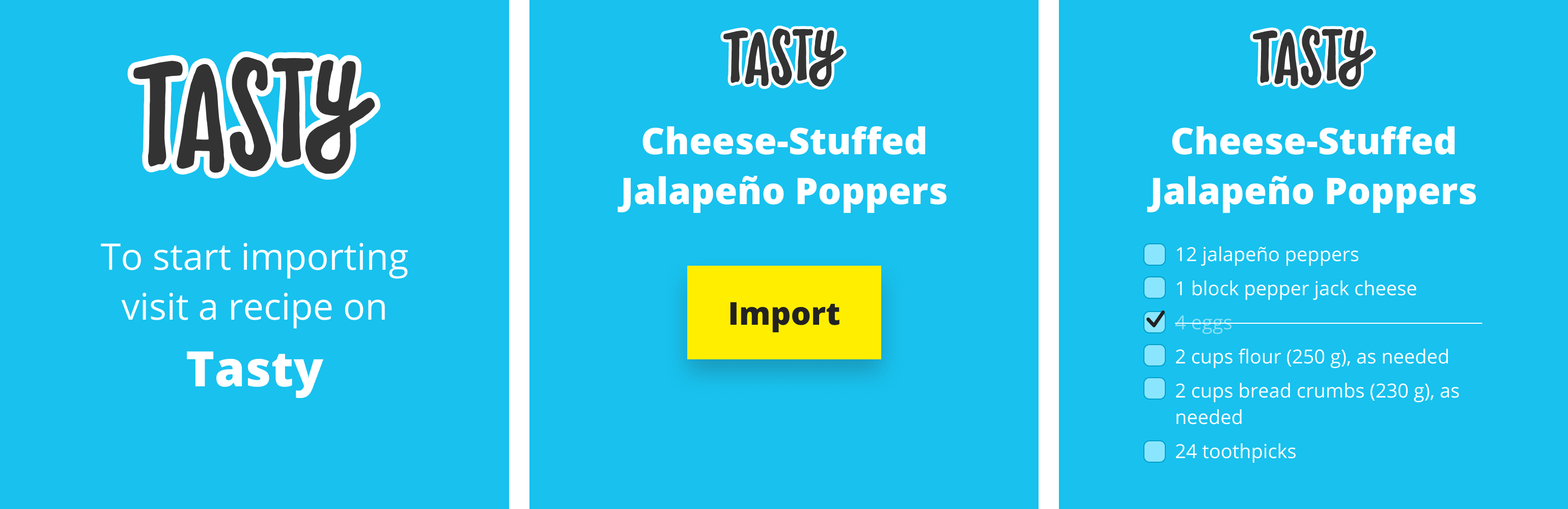
β¨ Features:
- Be able to import the ingredients of a recipe from a Tasty recipe page using a Chrome extension
- Be able to cross out ingredients from the list
- Be able to store the imported recipe in the Chrome extension
π§ You'll learn:
Chrome extensions
Extension popups
Content scripts
Chrome Permissions
π Resources:
#38 β Create a VSCode Extension
Rather not want to leave your code editor? Then building a VSCode extension is a good choice too. With this project, you will learn how you can create your own VSCode extension, how to create custom commands for it, and how to deploy it to the extensions marketplace so others can use it too.
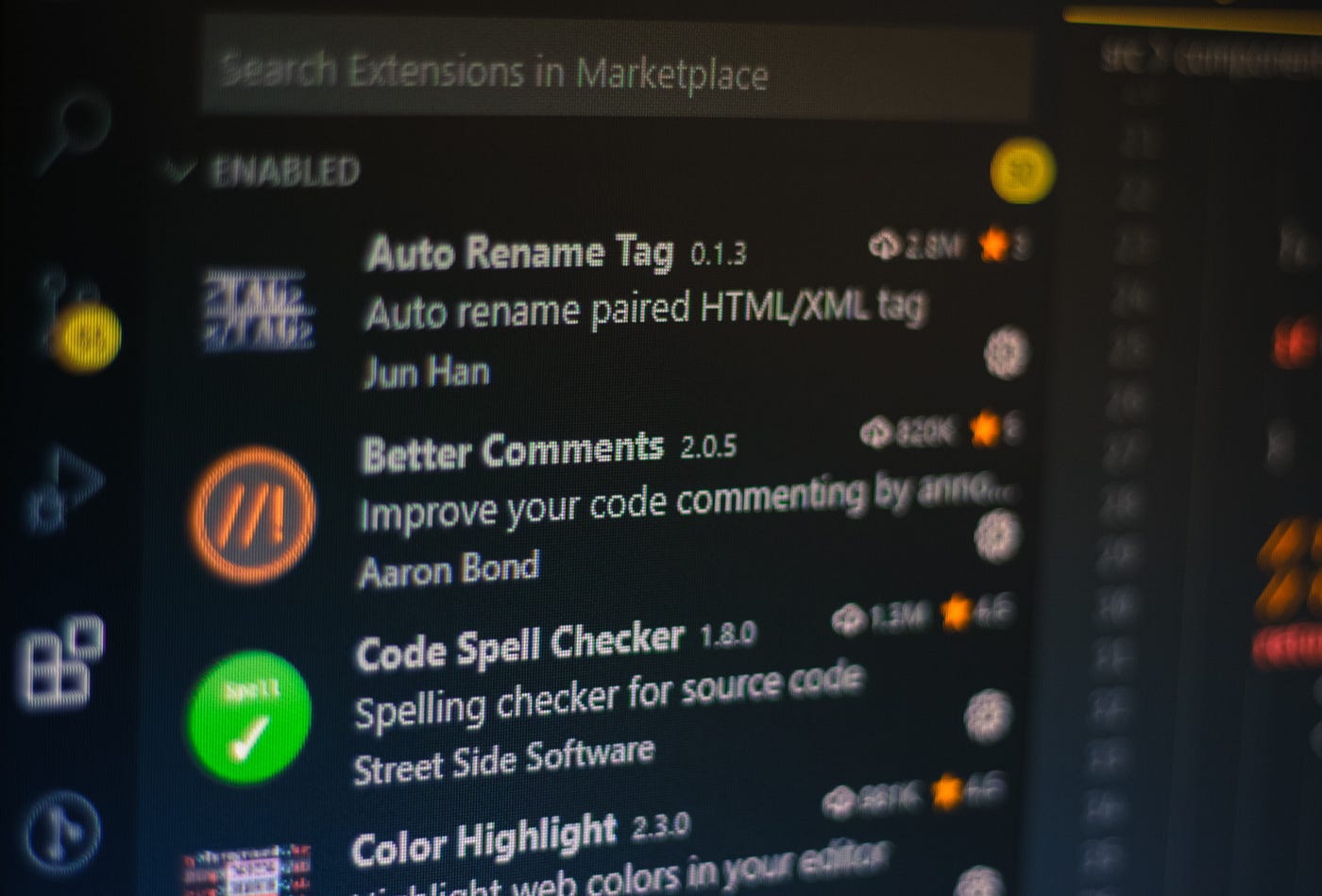
β¨ Features:
- Be able to install the extension through the Extensions tab
- Be able to call the extension with a custom command
π§ You'll learn:
Building a VSCode extension
Creating custom commands
Deploying the extension
π Resources:
Now letβs see some game ideas that you can easily (or not so easily) implement in JavaScript.

#39 β Create a Tic-Tac-Toe Game
Tic-tac-toe is a classic game that can be traced back to ancient Egypt, dating back around 1300 BC. Itβs a great starting project to learn about turn-based games, and how to handle game logic. As part of the project, you can also learn how to generate a grid, how to handle turns, and how to define a winning condition.
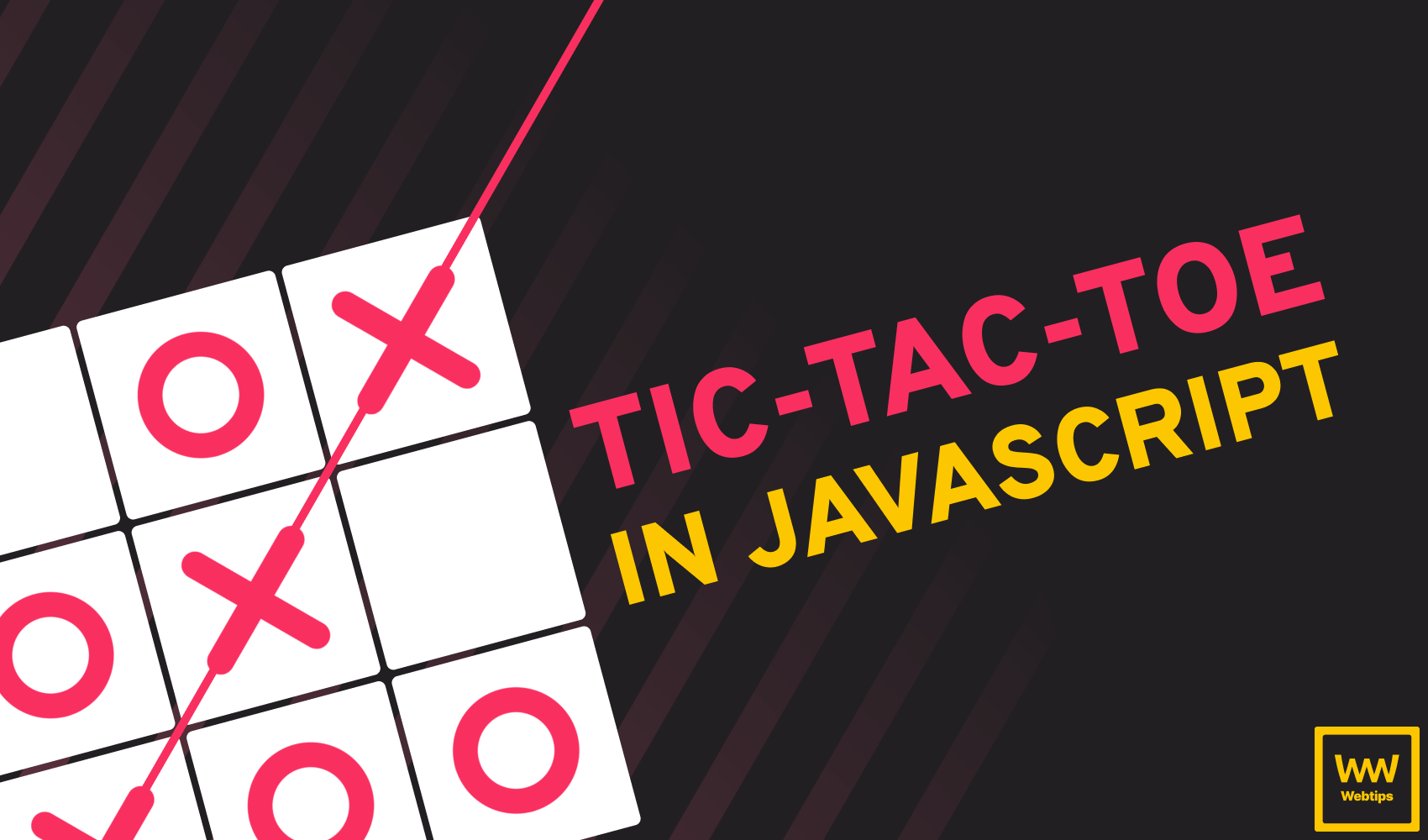
β¨ Features:
- Be able to generate a 3Γ3 grid
- Be able to take turns until one player wins (has their marks horizontal, vertical, or diagonal)
- Advanced:
- Be able to play against the computer
π§ You'll learn:
Generating grids
Click events
Handling game turns
π Resources:
#40 β Create a Rock, Paper, Scissors Game
Another classic game, this time, dating back to the Han dynasty around 200 BC is the rock, paper, scissors game. In this project, you will learn about working with random numbers and data attributes. After three turns, a winner should be nominated.
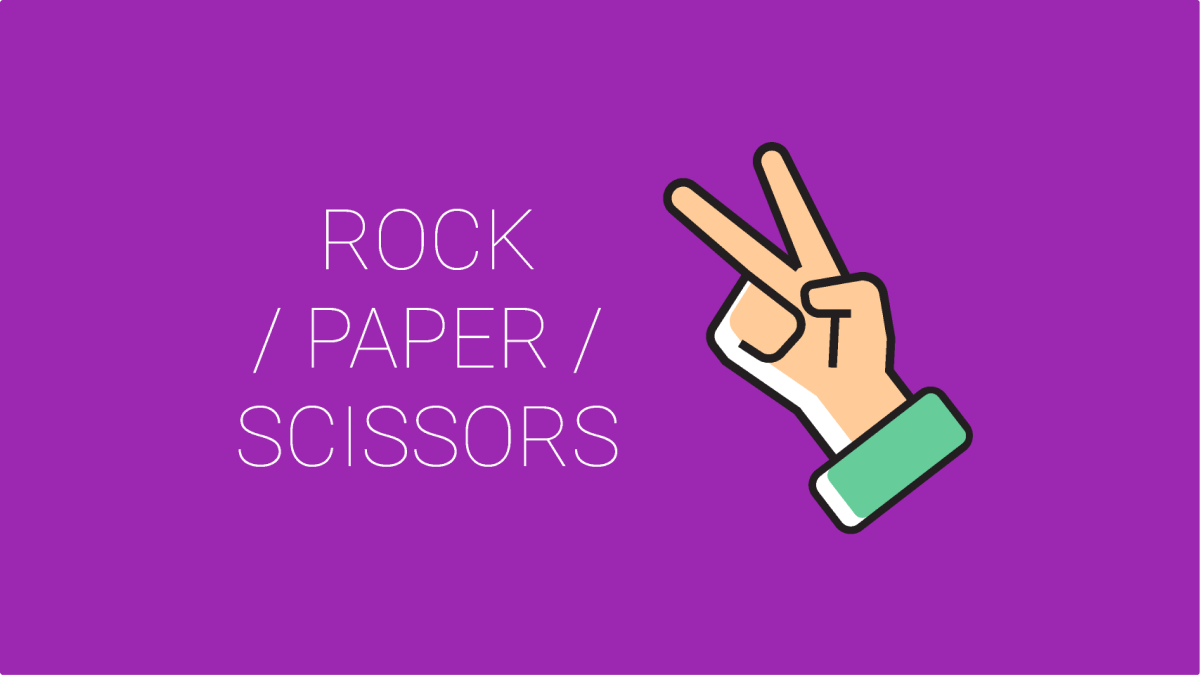
β¨ Features:
- Be able to draw either rock, paper, or scissors against the computer
- Be able to see the scores of each party
- Be able to see the outcome of previous turns
- A winner should be nominated after 3 turns based on the scores
π§ You'll learn:
Working with random numbers
Click event listeners
Data attributes
π Resources:
#41 β Create a Platformer Game
Want to look into a more advanced game? You can take a look at building a platformer, like an infinite side scroller with simple gameplay mechanics, such as Chromeβs jumping dinosaur, or a more complex one such as the famous Mario Bros from Nintendo. With these projects, you will learn a lot about how to build basic games with PhaserJS.
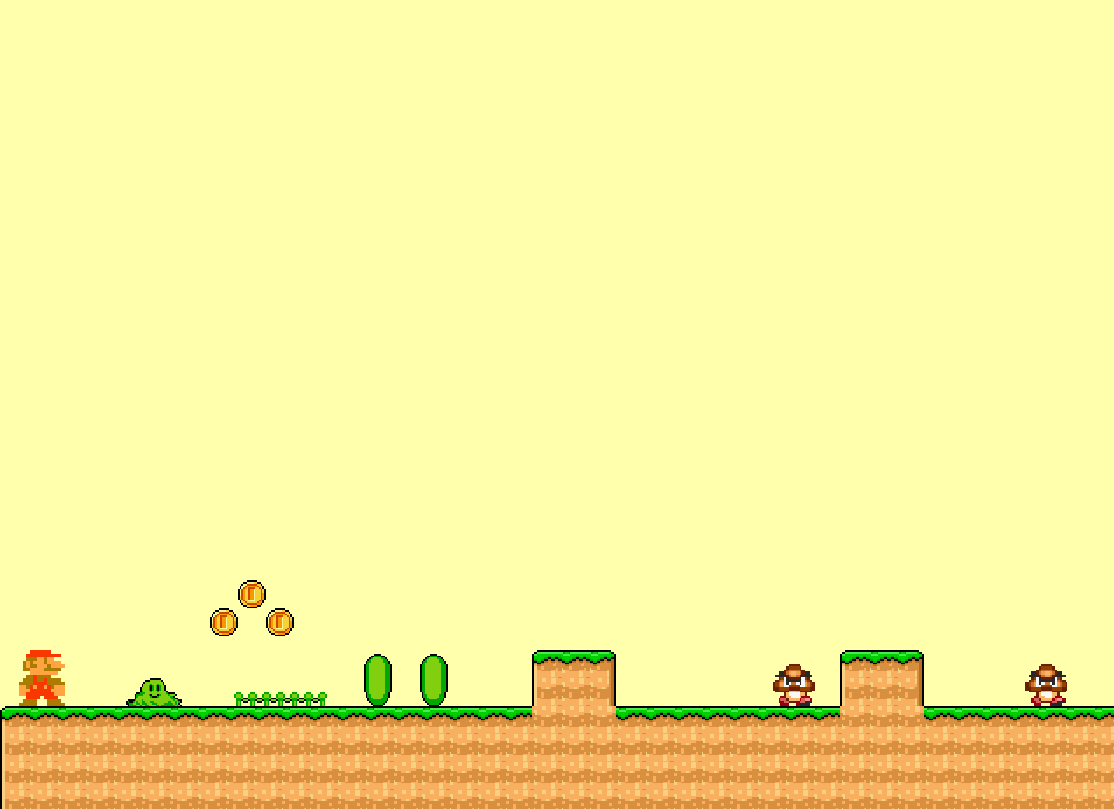
β¨ Features:
- Be able to control a 2D character on a map
- Be able to collect floating coins
- Be able to kill enemies by jumping on them
- When colliding with enemies from the sides, the game should be over
- Be able to win the game by reaching a flag at the end of the map
π§ You'll learn:
Working with PhaserJS
Working with game objects
Character movement control
Building maps from tilesets
Collision detection
Scoring mechanism
π Resources:
#42 β Create a Snake Game
You can also build games with JavaScript using only HTML elements. In this project, you will learn how you can build a snake game using nothing more, but checkboxes, and a radio button as an apple.
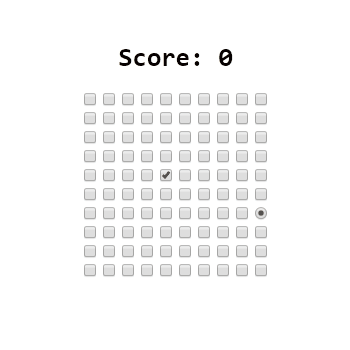
β¨ Features:
- Be able to control a checked checkbox using keyboard arrows
- Be able to collect an apple represented by a radio button
- The size of the snake should increase with each radio button hit
- The game should keep track of the score, increasing with each collected radio button
- The game should play a game over animation if the snake hits itself
π§ You'll learn:
Arrays
Keyboard inputs
Playing animations
Working with random numbers
π Resources:

#43 β Create Atariβs Breakout
Another famous game you can look into implementing is the famous Breakout from Atari. The aim of the game here is to hit every block with a ball, without allowing the ball to fall off. You have 3 lives, and you win the game once all blocks have been destroyed.
With this project, you will learn how to handle mouse events in Phaser, as well as how to handle arcade physics, tweens, or how to implement a scoring mechanism.
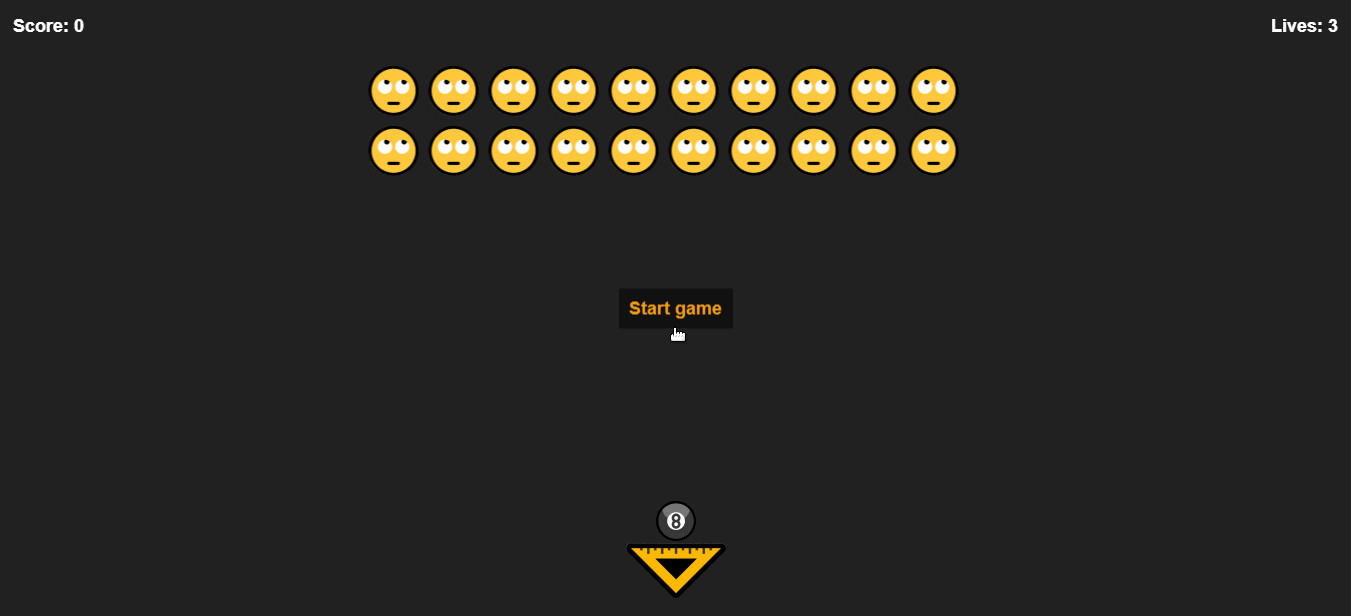
β¨ Features:
- Be able to shoot a ball from a paddle towards a grid of blocks
- Once a block is hit, it should be destroyed
- With each block destroyed, the player gets a score
- If the ball falls down, the player loses a health
- After losing three health points, the player loses the game
- After clearing all blocks from the screen, the player wins
π§ You'll learn:
Working with Phaser
Mouse events
Collision detection
Tweens
π Resources:
#44 β Create a Quiz Game
Rather work with HTML than the canvas, but still want to work on a game? Try looking into implementing a quiz game. Here you will learn more about working with an array of objects that describe each question and answer in the quiz, and how to implement a scoring system to see how good you are with a given quiz.
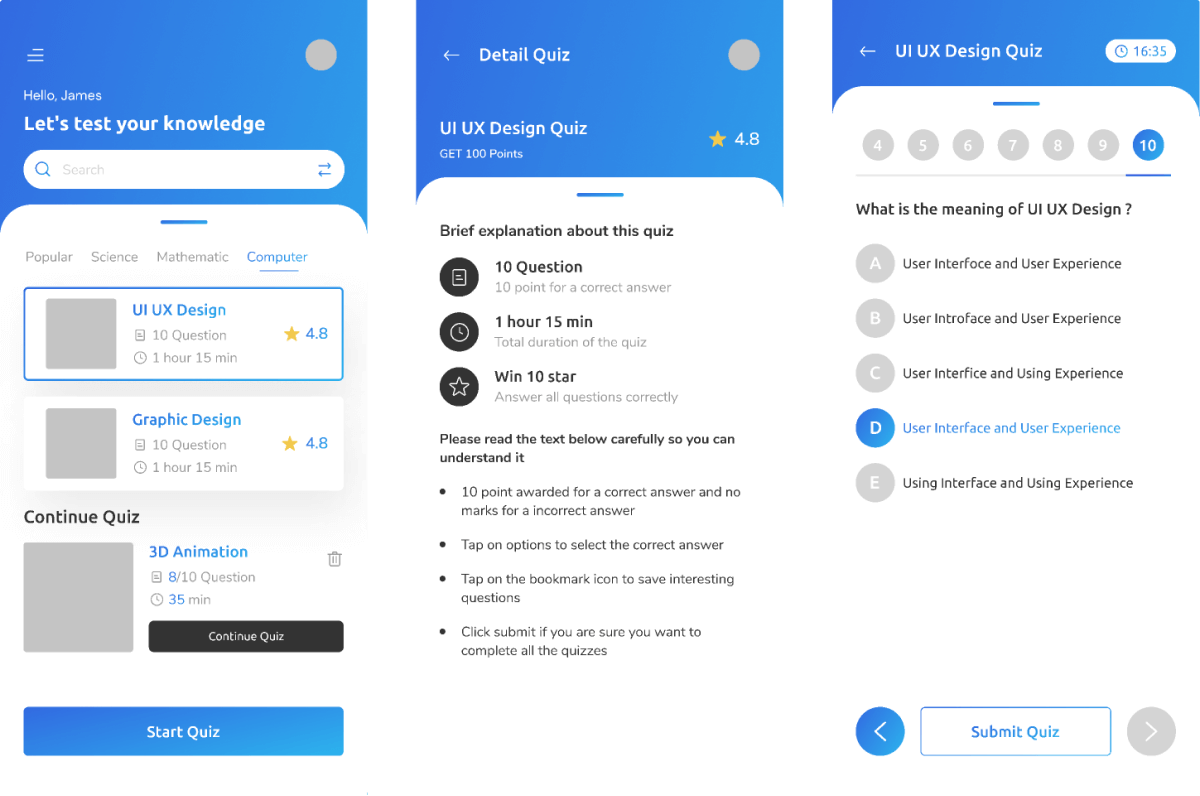
β¨ Features:
- Be able to choose an answer to a question from a list of available options
- Be able to see if the selected answer is correct
- Be able to see the number of scores based on correct answers
π§ You'll learn:
Click events
Array and objects
Scoring system
π Resources:
#45 β Create a Hangman
Another great game project where you can train your JavaScript skills is building the hangman. We all know how it goes, you have to guess the word before you run out of guesses, and the game is over. In this preview lesson from our JavaScript course, you will learn how to handle game assets, and handle different game states, such as win or lose.
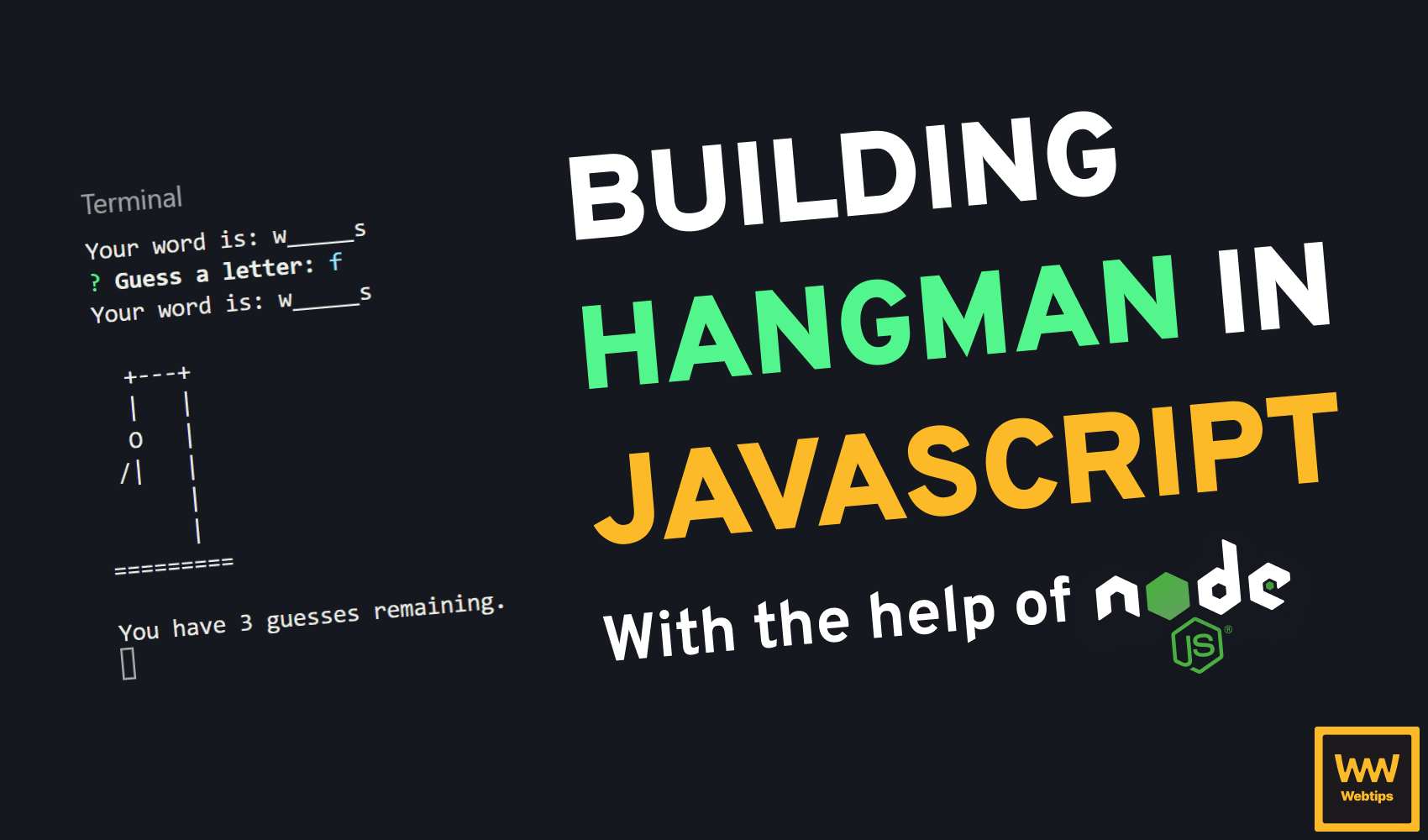
β¨ Features:
- Be able to see the number of letters a word has that needs to be guessed
- Be able to choose letters from the alphabet
- If there is a match, the letter should appear in the right place inside the word
- If there is no match, a part of the man should be drawn, and you should see the remaining number of wrong guesses
- If the word is guessed, you should be greeted by a win state
- If the word is not guessed, you should be greeted by a lost state
π§ You'll learn:
Keyboard events
Handling game states
Validating user inputs
π Resources:
#46 β Create a Memory Game
Building a memory game can not only improve your JavaScript knowledge but can also help you improve your memory. In this project, your task is to build a grid of cards that can be flipped over. If a match is found, the cards stay flipped, if not, they will turn back. If you run out of time, the game is over, but if you manage to find all matching cards, just like you would in a memory game, then you take the price.
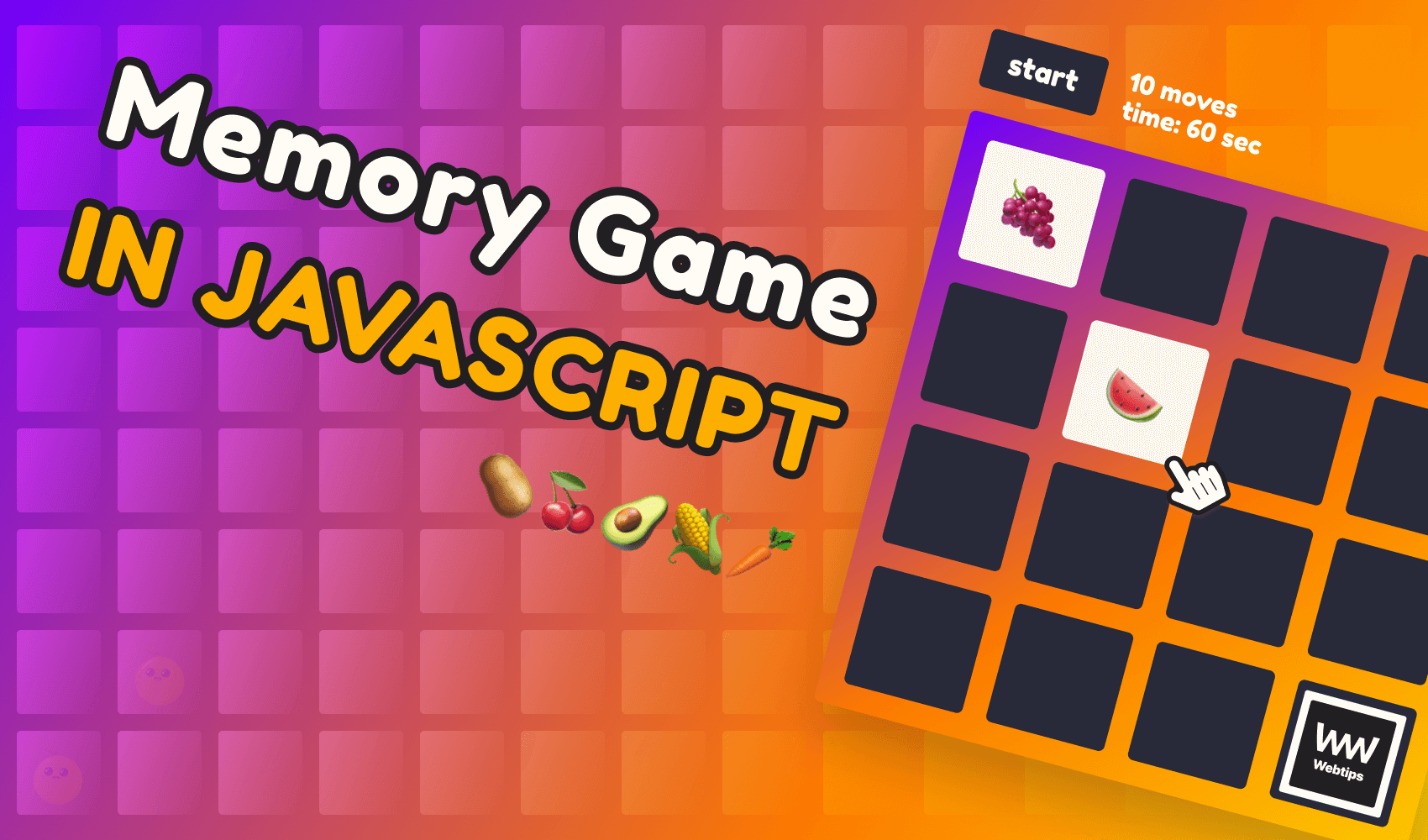
β¨ Features:
- Be able to flip cards that are displayed inside a grid
- When two cards are flipped and there is no match, the cards should turn back
- When two cards are flipped and there is a match, the cards should stay turned up
- Be able to see the number of flips
- When all cards are flipped, the player should get a victory screen
- When the time runs out, the player should get a game over screen
π§ You'll learn:
Click events
Handling game states
Timers
CSS transitions and animations
π Resources:

#47 β Create a Minesweeper Game
Another fun project to take on is developing the minesweeper game. The task of the player here is to uncover all fields that are free from any mines. If the player hits a mine, then the game is over. With this project, you can learn a lot about working with arrays, grids, and loops. Want to make the game more challenging? Implement a countdown so the game can only be solved under a certain amount of time.
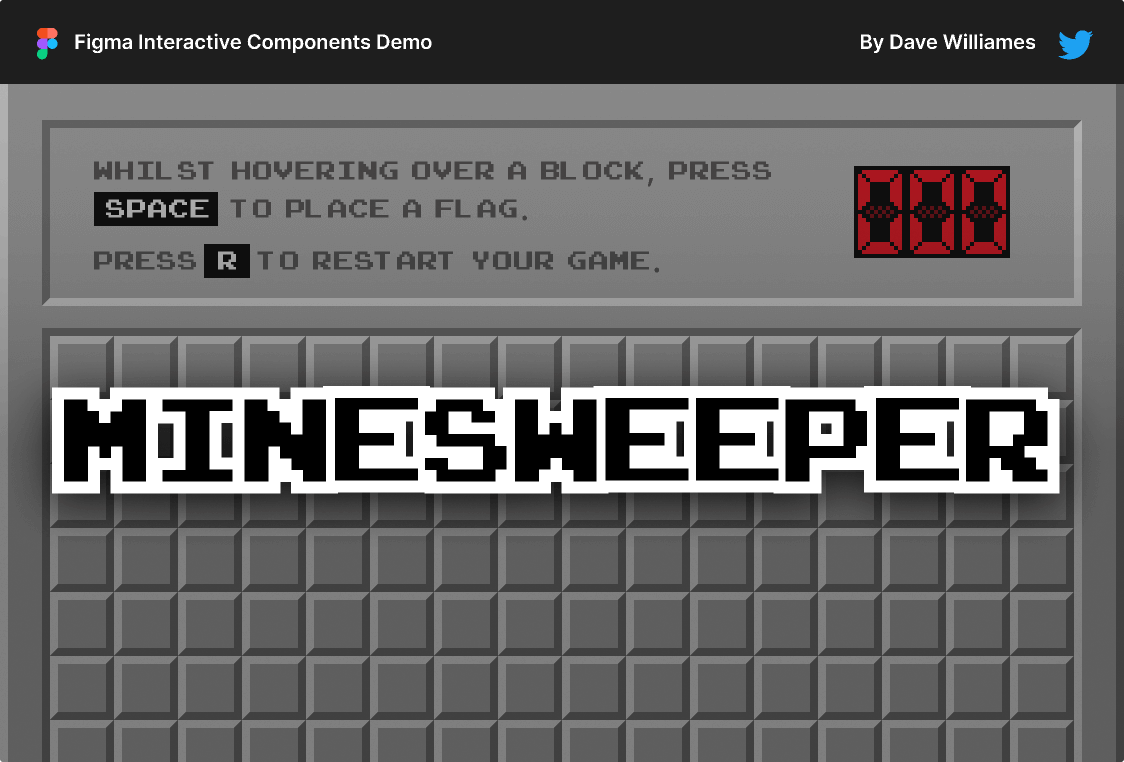
β¨ Features:
- Be able to click on a cell to uncover it
- Be able to flag a cell to indicate a potential mine location
- Be able to uncover flagged cells
- If a mine is uncovered, the game is over
- If all cells are uncovered that doesnβt have a mine, the player wins
π§ You'll learn:
Arrays
Loops
Grids
Working with random numbers
π Resources:
#48 β Create a Word Scramble Game
How about building a word scramble game? You can go as wild as on the design below and make them appear in a hexagonal grid, or you can simply switch words one after another with an input field where the user can type in their guesses. We all know the rules, you need to find all words to win the game. You will learn about working with keyboard events, manipulating the DOM, and implementing a scoring mechanism.
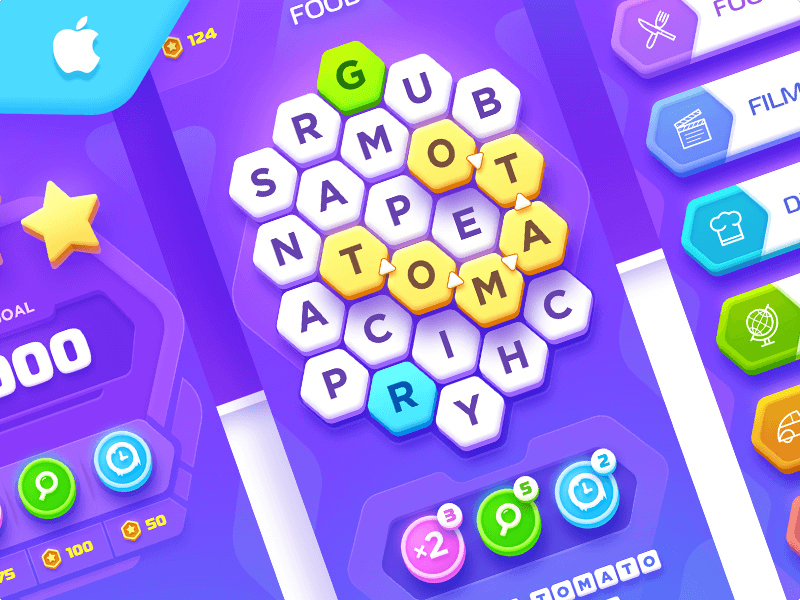
β¨ Features:
- Be able to see words scrambled
- Be able to type in the guessed word to an input
- Be able to see a score increasing or decreasing based on tries
- Be able to see the number of remaining guesses
- Be able to advance to the next level with longer words after finding all scrambled words
- If the player runs out of guesses, the game is over
π§ You'll learn:
Keyboard events
DOM manipulation
Implementing a scoring mechanism
π Resources:
#49 β Create Fireworks
As we are approaching the end of the year, itβs a perfect opportunity to start practicing how particles can be created in JavaScript. With this project, you will be building a mage with a wand that can shoot fireworks to the night sky, all done on a canvas element. You will learn how to handle different mouse events, and how to create physics-simulated particles by utilizing the Math object. This will be the final output of this project:
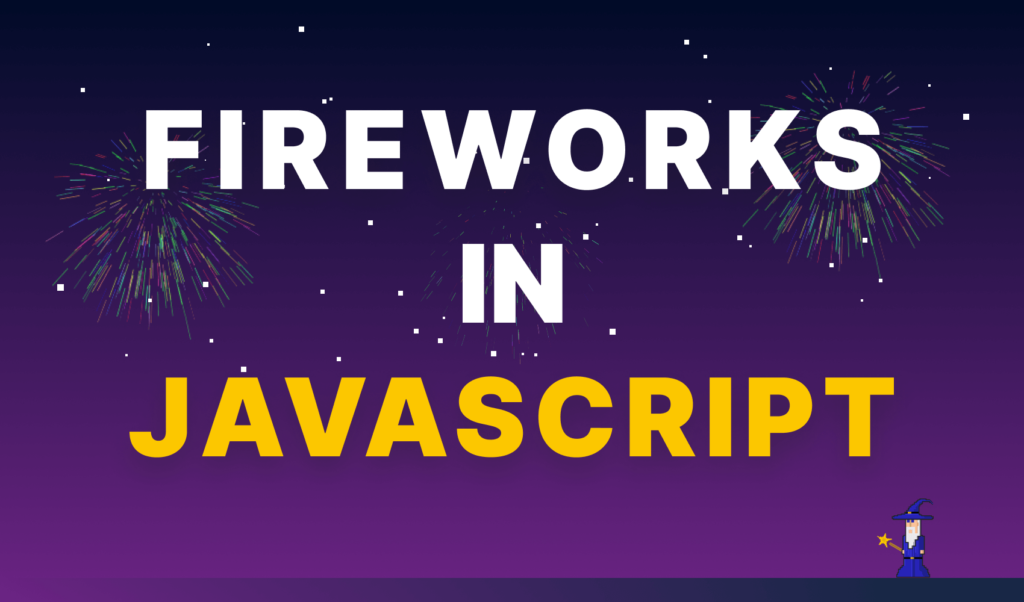
β¨ Features:
- Be able to control the rotation of the wand with the cursor
- Be able to shoot fireworks at the position of the mouse with clicks
π§ You'll learn:
Canvas API
Mouse events
Particle effects
requestAnimationFrame
Working with the
Math
object
π Resources:
#50 β Create a 3D Planet
If you want to look into 3D instead, then you might want to try out Three.js. It provides all the necessary APIs for you to more easily build 3D projects right inside your browser. In this project, you will be building a 3D replicate of Mars with lightning, textures, depth, and camera movement. Here is the final end result:
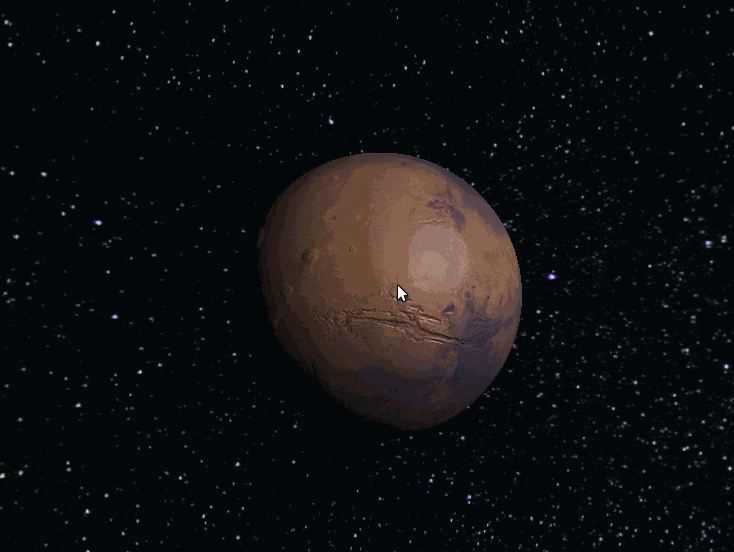
β¨ Features:
- Be able to see a 3D rotating planet on a canvas
- Be able to rotate the camera with the mouse
π§ You'll learn:
Canvas API
Working with ThreeJS
π Resources:

#51 β Create a 3D Rubikβs Cube
Looking for more challenging work? With the help of Three.js, you can also try building a 3D working Rubikβs cube. With this project, you can learn a lot about rotating objects and mouse events inside the scene. If you would like to go the extra make, try to let the cube auto shuffled, or completed by the computer itself.
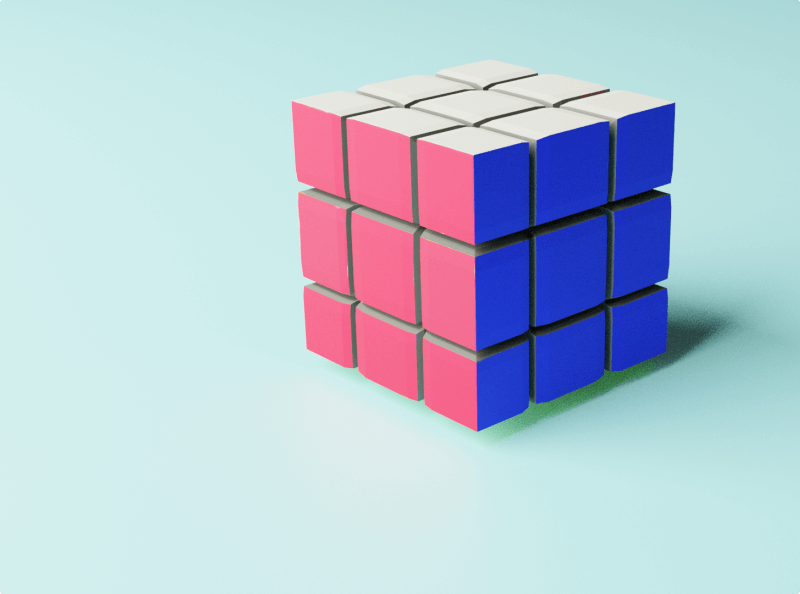
β¨ Features:
- Be able to see a 3D Rubikβs cube rendered on a canvas
- Be able to interact with the 3D Rubikβs cube and rotate the sides
- Advanced:
- Be able to automatically shuffle the Rubikβs cube
- Be able to automatically complete the Rubikβs cube
π§ You'll learn:
Canvas API
Working with 3D objects
Mouse events
π Resources:
Want something even more advanced? Letβs see a couple of examples that involve AI/machine learning.
#52 β Create an Image Classifier
One of the most common use-cases of machine learning is image classification. With this project, you will need to build an image classifier using the TensorFlow library, that should be able to accurately predict images based on a pre-trained model. Here is what we are going to build:
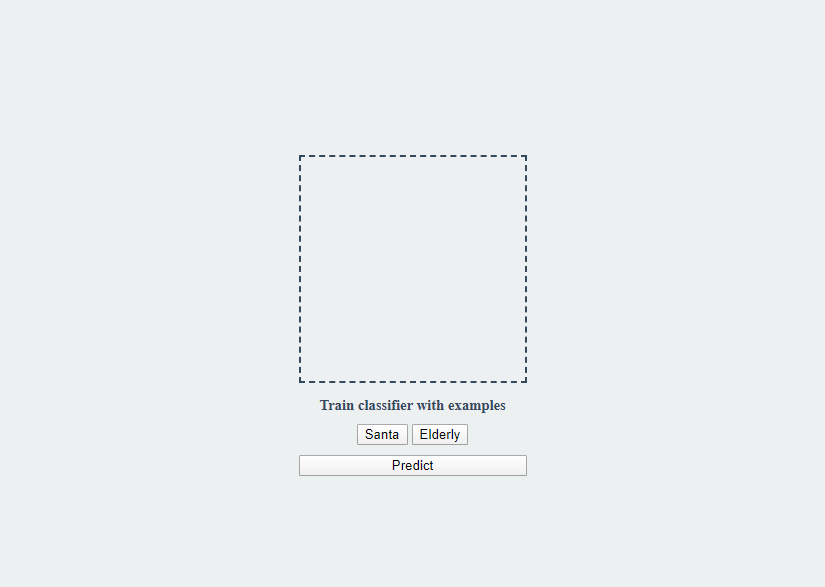
β¨ Features:
- Be able to drag and drop images to a page
- Be able to train a classifier with the dropped images
- Be able to predict previously unseen images
π§ You'll learn:
Working with TensorFlow
Working with a KNN classifier
Training models
π Resources:
#53 β Create a Chatbot
Want to learn about natural language processing? Then building a chatbot is a great way to get you started. Here youβll learn about the basics of NLP, how to work with the Natural library, and how to train a model based on a large data set, that will respond to even messages it has never seen before.

β¨ Features:
- Be able to run the code on CLI with a message, which should respond with an appropriate response from the training data
π§ You'll learn:
Natural language processing
Working with the Natural library
π Resources:
#54 β Create a Face Recognition App
Another great machine learning project that involves images is an app that can recognize faces and emotions. Here, we will take a look into how face-api works, and how you can use its models to detect faces on images, draw facial landmarks or even use it for emotion detection.
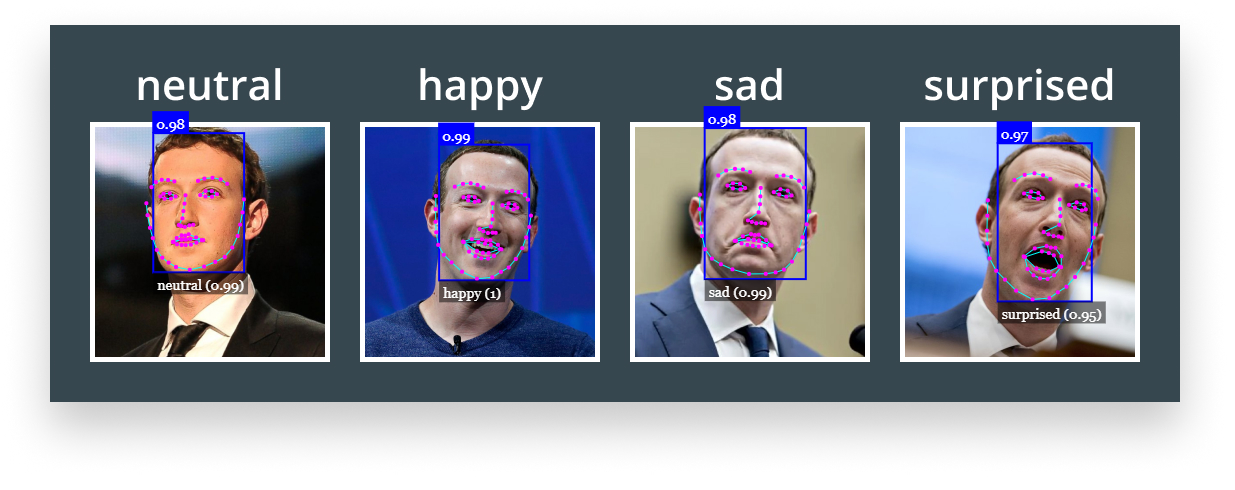
β¨ Features:
- Be able to provide an image on which the algorithm detects faces, landmarks, and emotions.
π§ You'll learn:
Image recognition
Emotion detection
Working with the face-api library
π Resources:
If youβre looking for more simple challenges, there are plenty of other app ideas out there you can implement, such as:

#55 β Create a Weather App
Probably the most common project you will come across is a weather app. The reason for that is that itβs simple even so that it can be built in a matter of hours, but it has all major technical challenges you can come across during your daily work. These include things such as requesting data from an API, displaying it to the user, or be able to search for weather forecasts.
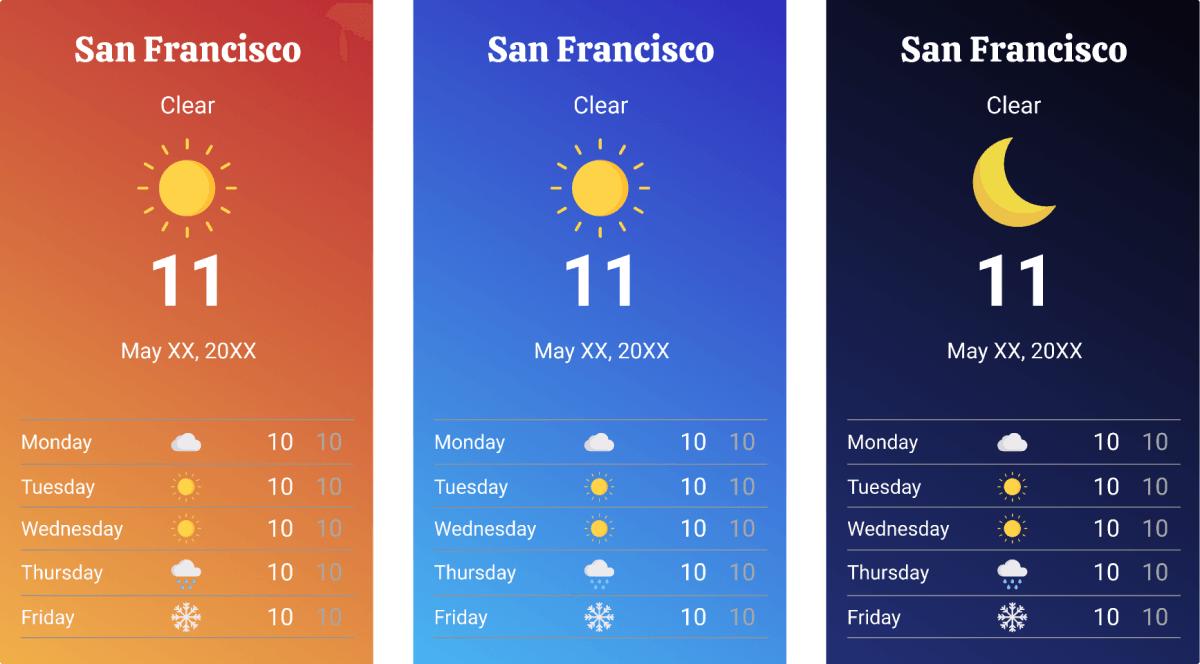
β¨ Features:
- Be able to see the weekly weather forecast for a city
- Be able to see the date
- Be able to see weather conditions
- Advanced:
- Be able to search for a city
- Be able to see wind speed and direction
π§ You'll learn:
Geolocation API
Fetch API
Working with an external weather API
π Resources:
#56 β Create a Clone
You can also look into implementing one of your favorite services out there. Want to learn about how e-commerce works? Build an Amazon clone. Want to learn how you can build complex search algorithms? Build a Google clone. Want to learn how to build a social network? Build a Facebook clone. You get the idea.
In this project, you will learn how to create a basic IMDB clone. You will learn about routing, fetching data from an API, and consuming it to display it in a meaningful way.
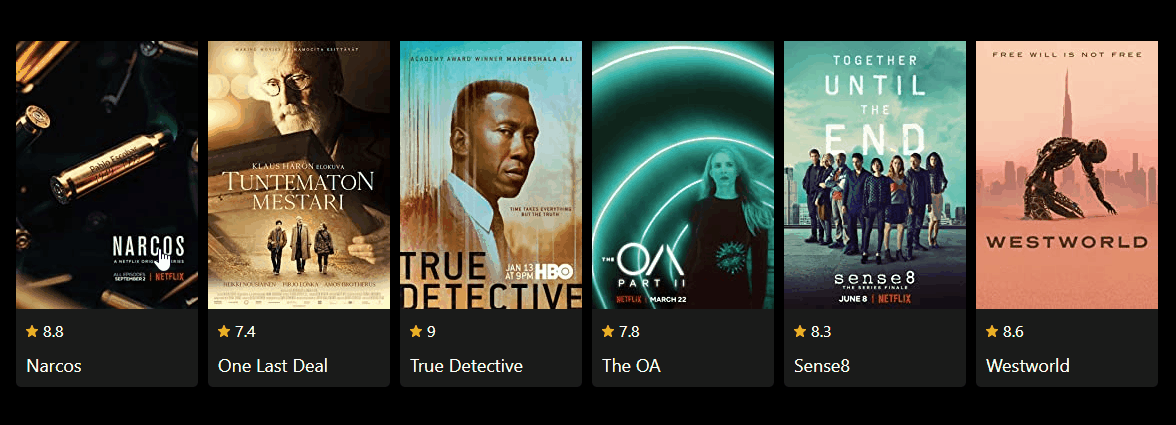
β¨ Features:
- Be able to see a list of movies with their cover, title, and rating
- Be able to navigate to individual movies where a description, creator, and actors are listed
- Be able to navigate back to the list
π§ You'll learn:
Routing
Fetch API
Requesting and consuming data from an endpoint
π Resources:
#57 β Create a Desktop Application
If you want to think outside of the browser, you can also look into building an actual desktop application. With the help of Electron, you can use technologies you are already familiar with: HTML, CSS, and JavaScript.
With this project, you will not only learn how to build a desktop app using Electron, but also how to work with cron jobs, and how to work with the native notification API.
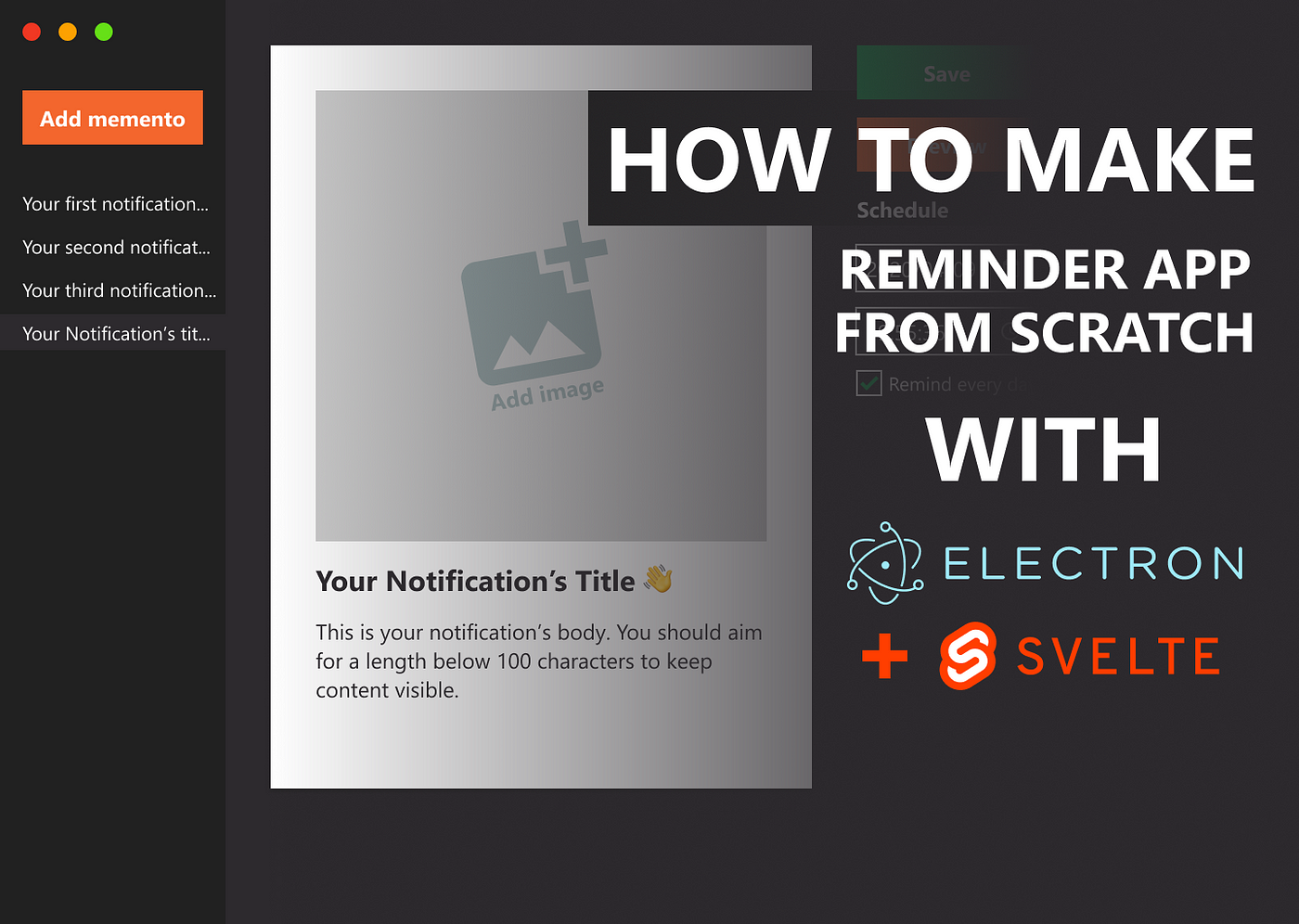
β¨ Features:
- Be able to create notifications in a desktop application
- Be able to set the image, title, and description of a notification
- Be able to schedule notifications
- Be able to edit existing notifications
- Be able to remove existing notifications
π§ You'll learn:
Electron
Cron jobs
Working with the native notification API
π Resources:
#58 β Create Bubble Charts
If you are into working with visualizing data, then the following two projects might be just for you. Here you will learn how to work with D3.js to build a bubble chart that represents what articles drive the most traffic to your site. You will also learn about creating a tooltip to showcase more data upon hovering a bubble, and you can also interact with them to navigate to different sections on your page.
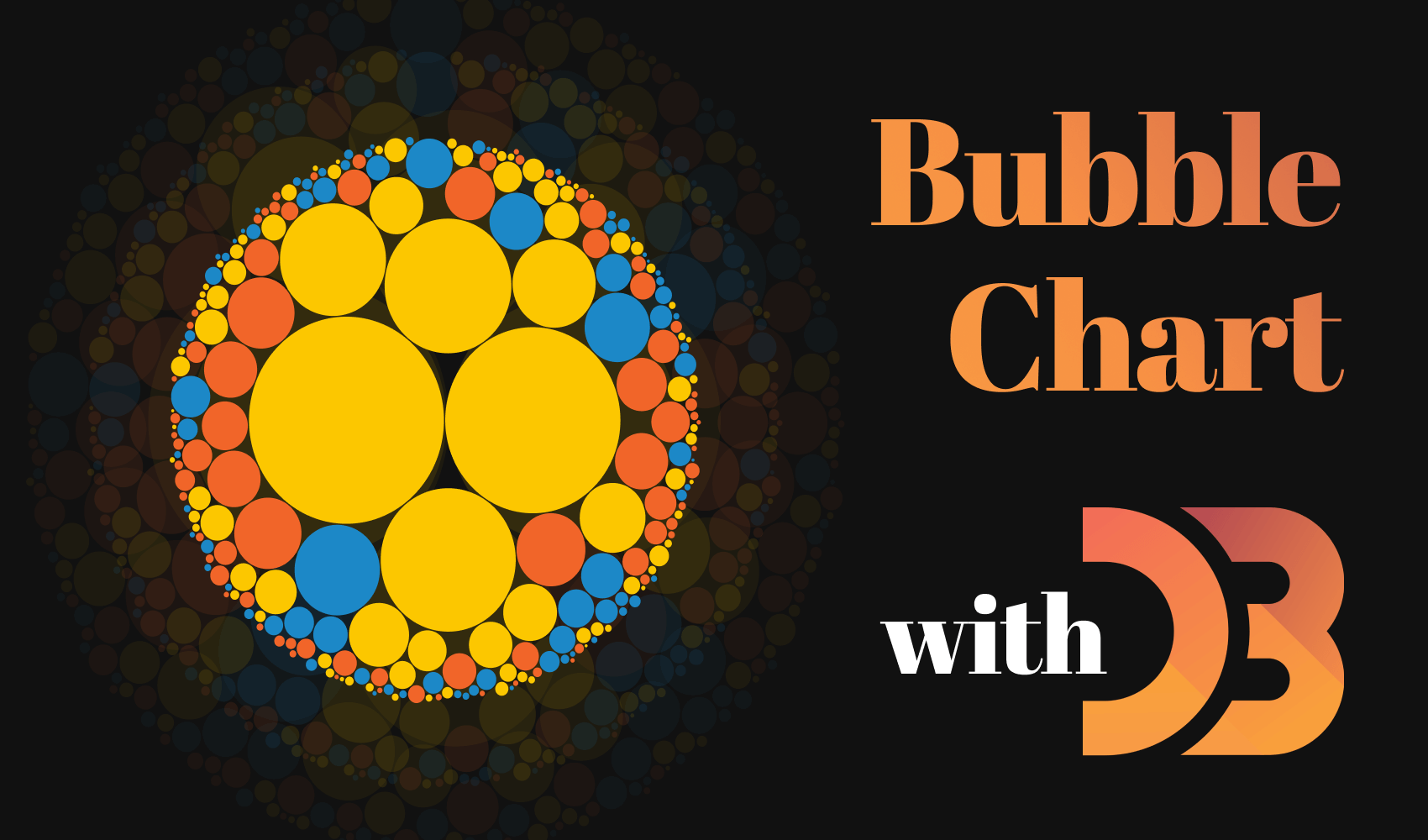
β¨ Features:
- Be able to generate a bubble chart from a JSON file
- The size of the bubbles should reflect the traffic each entry gets. Entries with higher traffic should be represented by a larger bubble
- Be able to interact with the bubbles and see additional information on it
- Be able to navigate to a new page by interacting with the tooltip that is shown upon hover of a bubble
π§ You'll learn:
D3.js
Animations
Working with SVGs
π Resources:

#59 β Create Treemaps
Another great way to get familiar with D3.js is by building a treemap. Here we will be looking at how to create a treemap from a JSON file. You will be able to visually see which files/folders take up the most space. You will be also able to navigate between different levels, and even change the styles of the treemap. If you ever wanted to learn more about recursions, then this is the right project as we will also look into that.
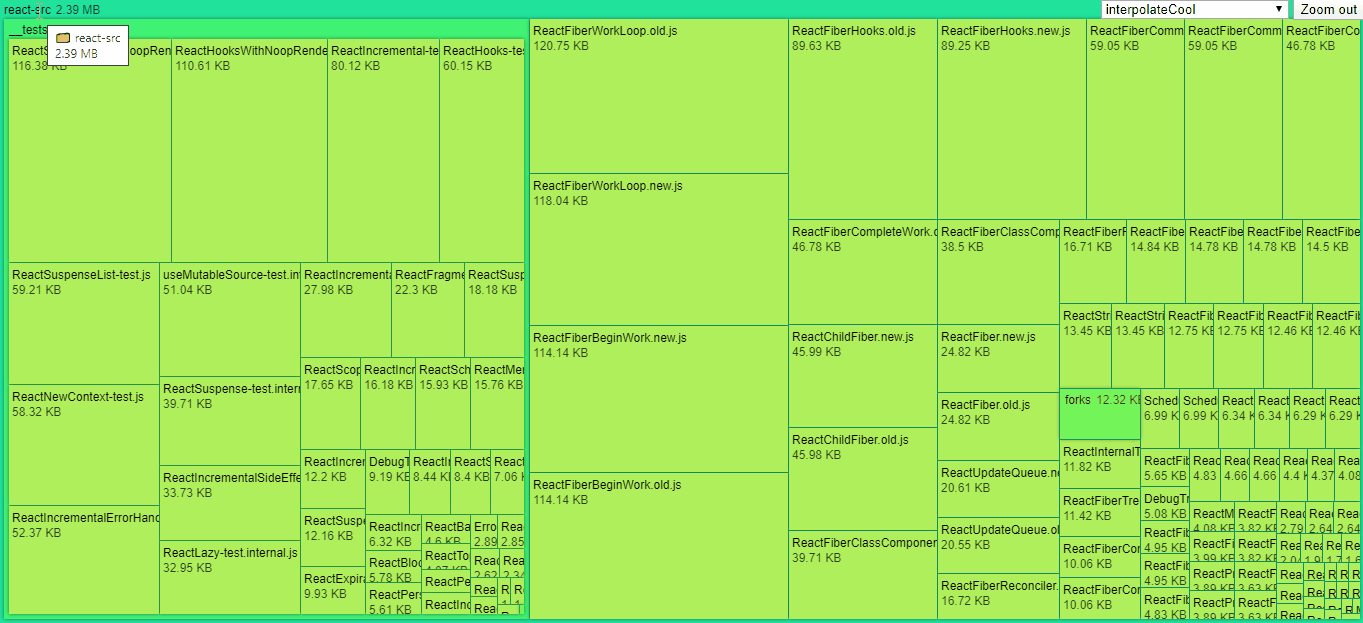
β¨ Features:
- Be able to generate a treemap from a JSON file
- Be able to navigate to sub-nodes
- Be able to navigate back to ancestors
- Be able to change the style of the treemap
π§ You'll learn:
D3.js
Recursion
Working with SVGs
π Resources:
#60 β Create a Real-Time Chat App
If you want to dive into the realm of real-time, then try building a real-time chat application with the ability to also see who is typing at the moment. In this project, you will learn more about web sockets, working with socket.io, and how you can emit and listen to events to update the UI accordingly.
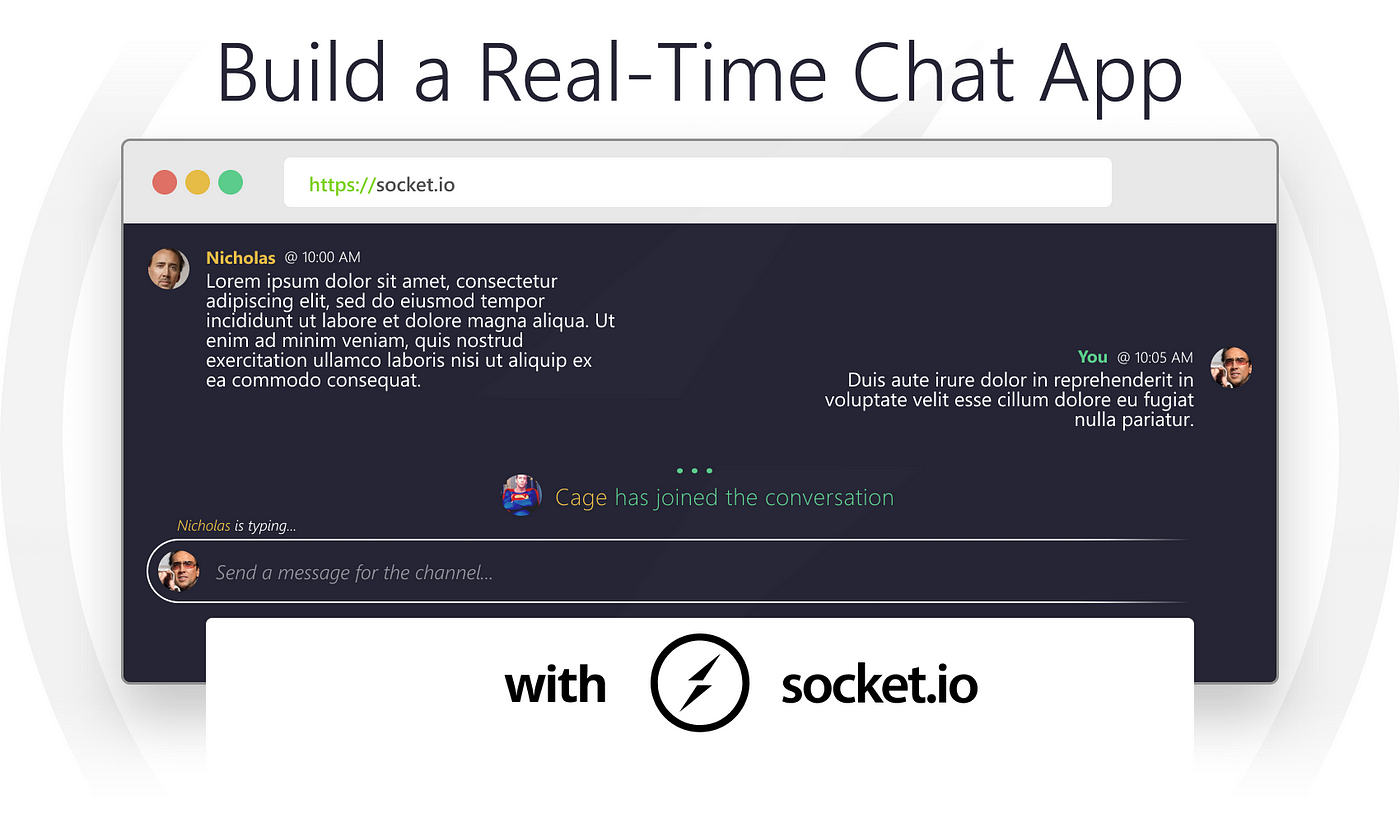
β¨ Features:
- Be able to join a chat conversation
- Be able to send and receive messages
- Be able to see messages appear in real-time
- Be able to see if someone is typing
π§ You'll learn:
Websockets
Working with Socket.IO
Emitting and listening to events
π Resources:
#61 β Create a Kanban Board
If you would like to learn more about how the drag and drop API works, then building a Kanban board is a great way to do that. Here you will learn about how you can drag and drop cards from one column to the other. If you want to persist the state of the cards after page reload, you can also learn about local storage or working with a database, depending on your use case.
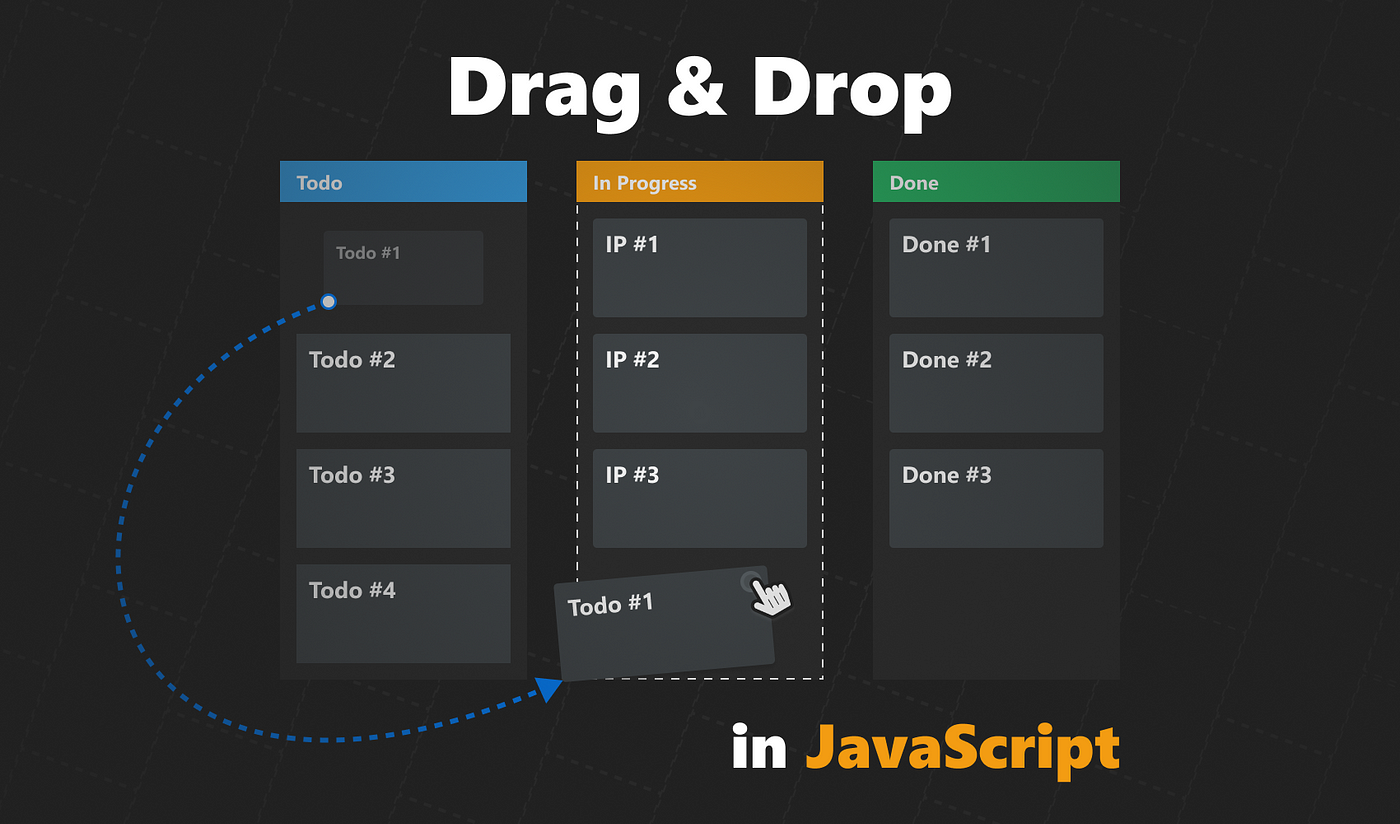
β¨ Features:
- Be able to drag cards from one column to the other
- Advanced:
- Be able to persist the state, even after page reload
π§ You'll learn:
Drag and Drop API
Local storage for persisting state
π Resources:
#62 β Create a Retro Board
We can also look into creating another kind of board, this time, a retro board. In this project, you will learn about adding, editing, and removing items from columns, as well as giving them likes in the form of claps. The end project will look something similar:
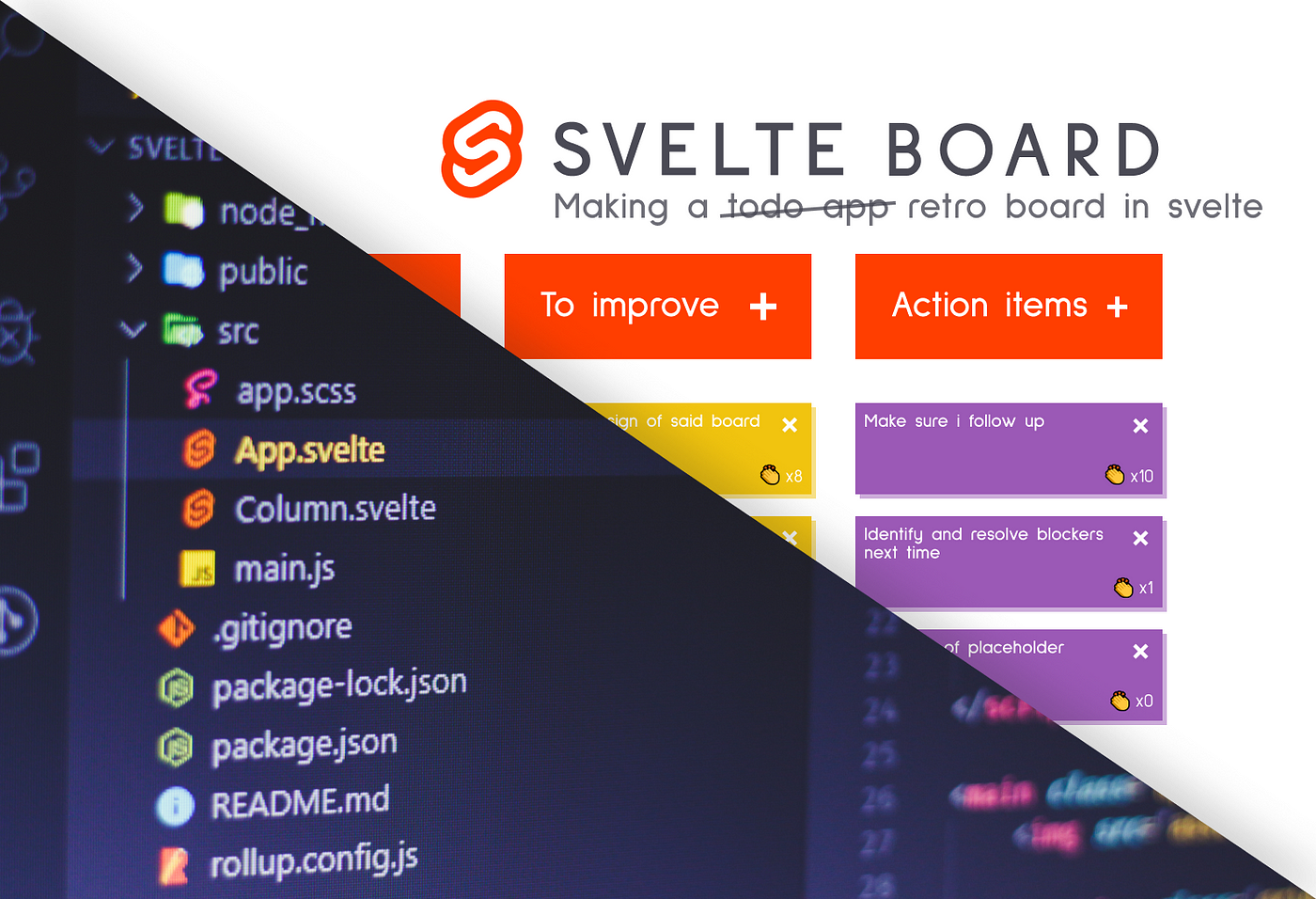
β¨ Features:
- Be able to add new items for each column
- Be able to remove existing items from columns
- Be able to edit the text inside the cards
- Be able to like cards by clapping them
- Be able to see the number of claps on each card
π§ You'll learn:
Dom manipulation
State management
Editable contents
π Resources:

#63 β Create a Reminder App
Building a reminder app from scratch can help you learn more about working with cron jobs and scheduling events. In this project, we will look into how you can work with push notifications in order to see reminders popping up on your screen, and we will also see how the whole application can be handled by a single global state.
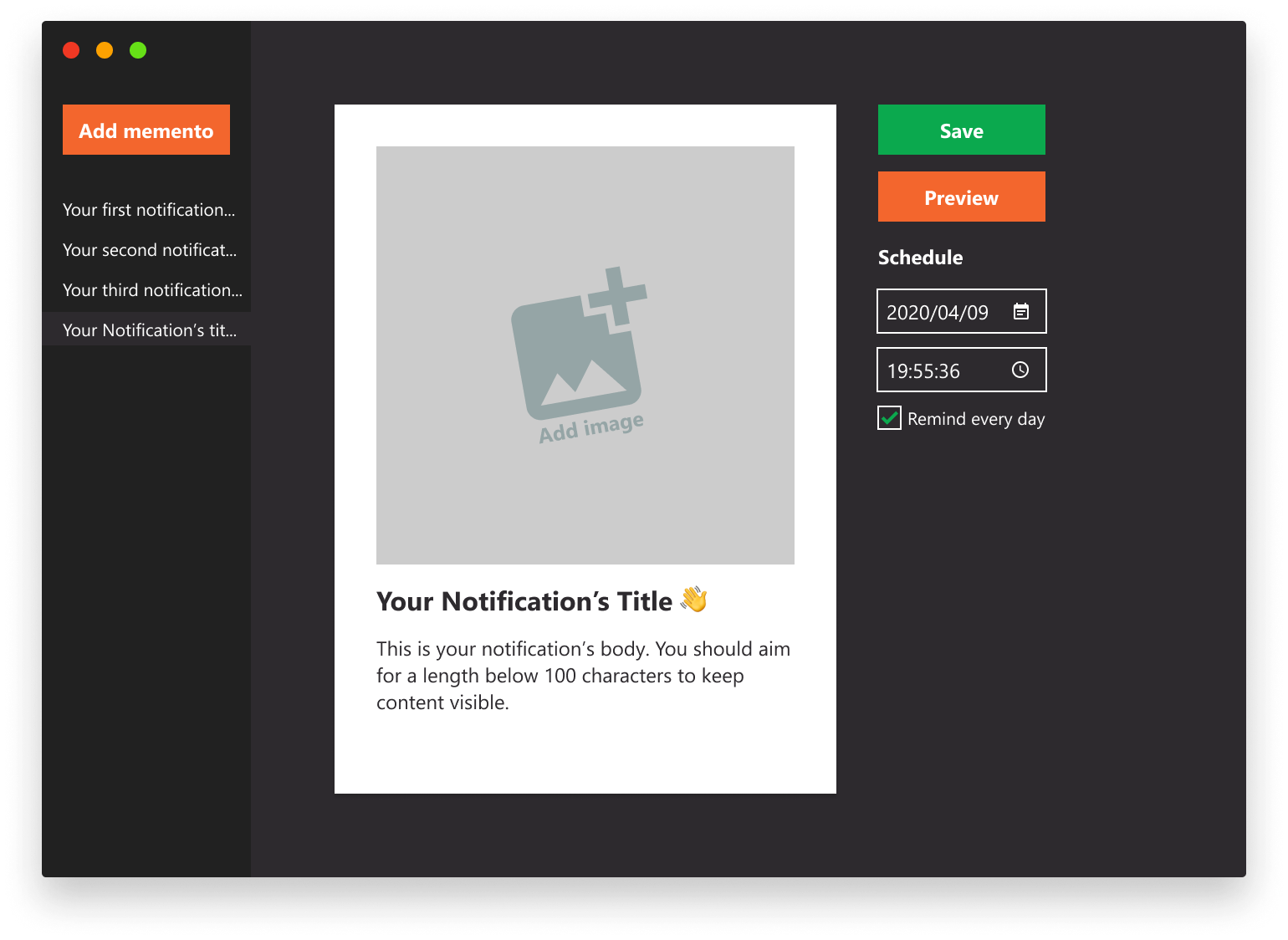
β¨ Features:
- Be able to create new notifications
- Be able to schedule notifications
- Be able to edit existing notifications
- Be able to remove existing notifications
π§ You'll learn:
Push notifications
Cron jobs
Working with global states
π Resources:
#64 β Create a Scheduler
If youβd rather want to work on your Node skills, and you donβt want to build an entire reminder app, then you can look into building a scheduler that works with the native push notification API. Works in a similar way as the previous project, only this time, without a user interface. As part of this project, you will also learn how you can create cron jobs to send scheduled notifications. By the end of the project, you will get the following behavior:
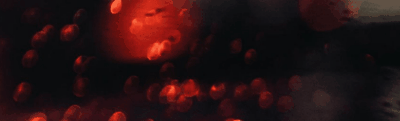
β¨ Features:
- Be able to schedule notification
- Be able to set a title and a message for the notification
- Be able to see the notification triggered at the scheduled time
π§ You'll learn:
Notification API
Cron jobs
Working with Node
π Resources:
#65 β Create a Note-Taking App
Next up, we have a note-taking app. With this project, you will learn how to display data using JSON files, work with global states, and implement CRUD operations so that you can create, update, and delete pages as you go. We will also look into integrating a WYSIWYG editor into the mix to make the app more accessible.
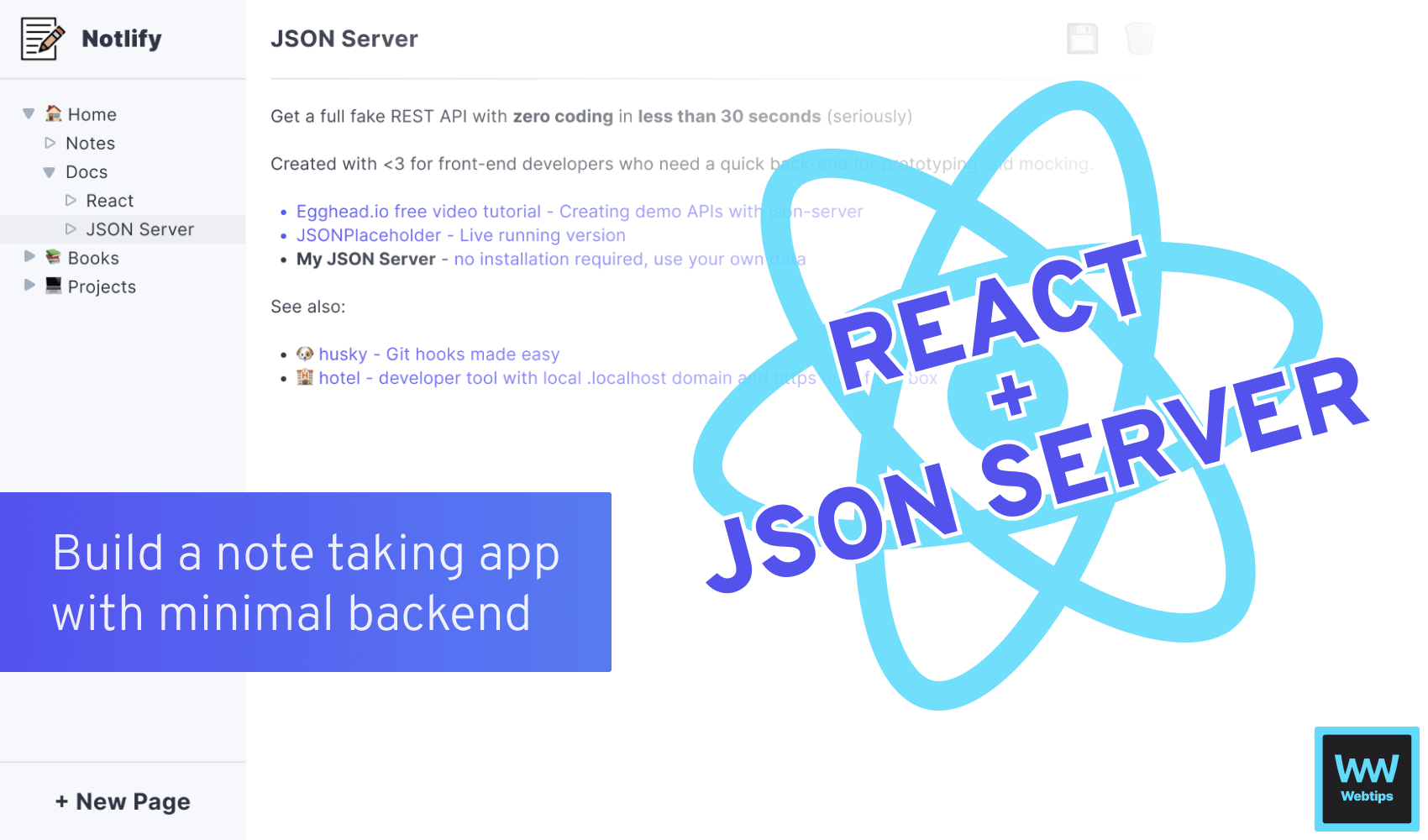
β¨ Features:
- Be able to create new pages
- Be able to remove existing pages
- Be able to take notes on pages with a WYSIWYG editor
π§ You'll learn:
CRUD operations
Building navigations
Working with a WYSIWYG editor
π Resources:
#66 β Create a Habit Tracker
Building new habits, and getting rid of old bad ones is not an easy take, but it is an essential part of becoming a better version of yourself. With this project, your task is to build a habit tracker so you can keep yourself more accountable. You will learn how to create new habits, define a frequency for them, and how to store everything in local storage. If you want to go wild, you can also look into visualizing it on a chart or calendar to see your progress.
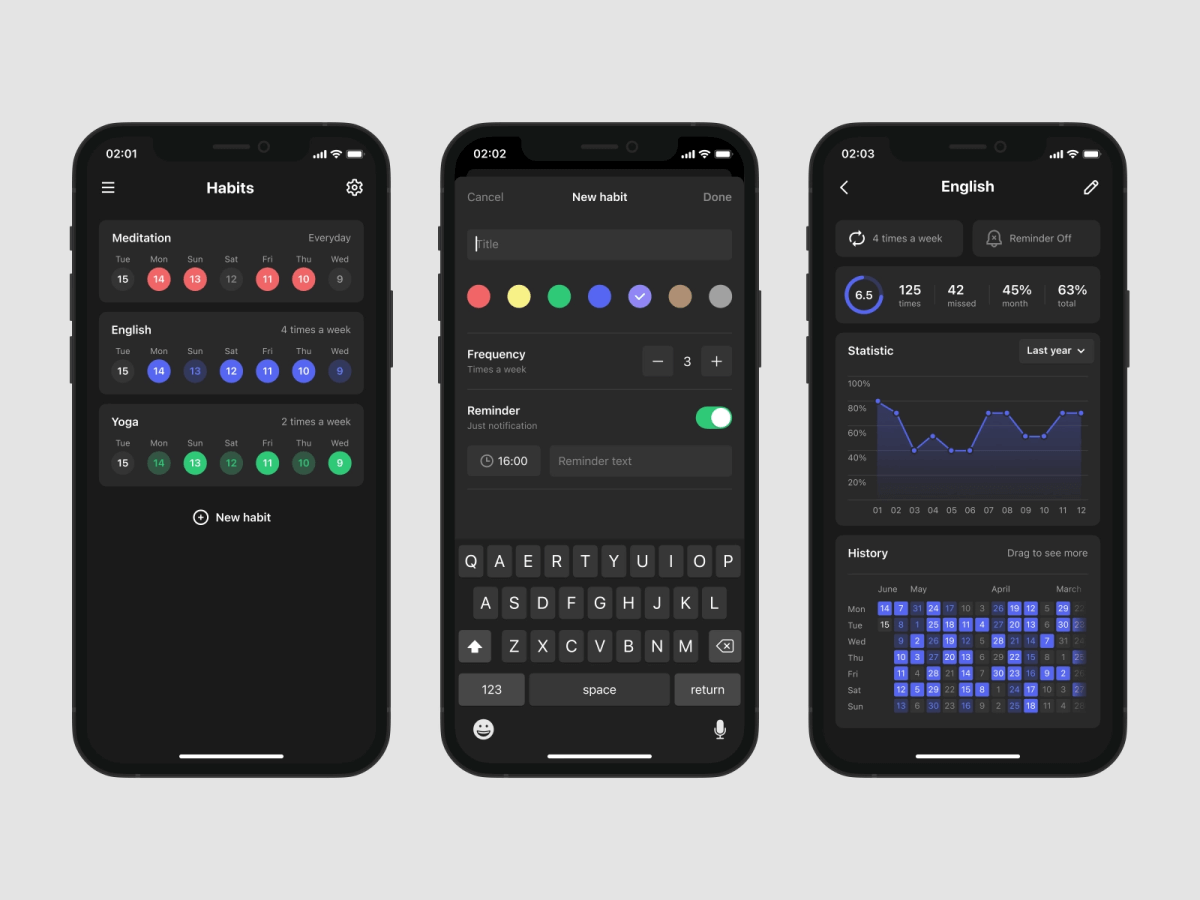
β¨ Features:
- Be able to add new habits
- Be able to see the list of habits created
- Be able to delete existing habits
- Be able to define a frequency for a habit
- Be able to store the habits in local storage
π§ You'll learn:
Arrays and objects
Local storage API
π Resources:

#67 β Create a Mobile App
Of course, you can not only build apps for the web or desktop with JavaScript but also build mobile applications. With this project, you will learn how you can make a checklist app with React Native with saving and loading functionality. You will also learn how to create list elements, how to handle their state, and how to store everything on your deviceβs storage.
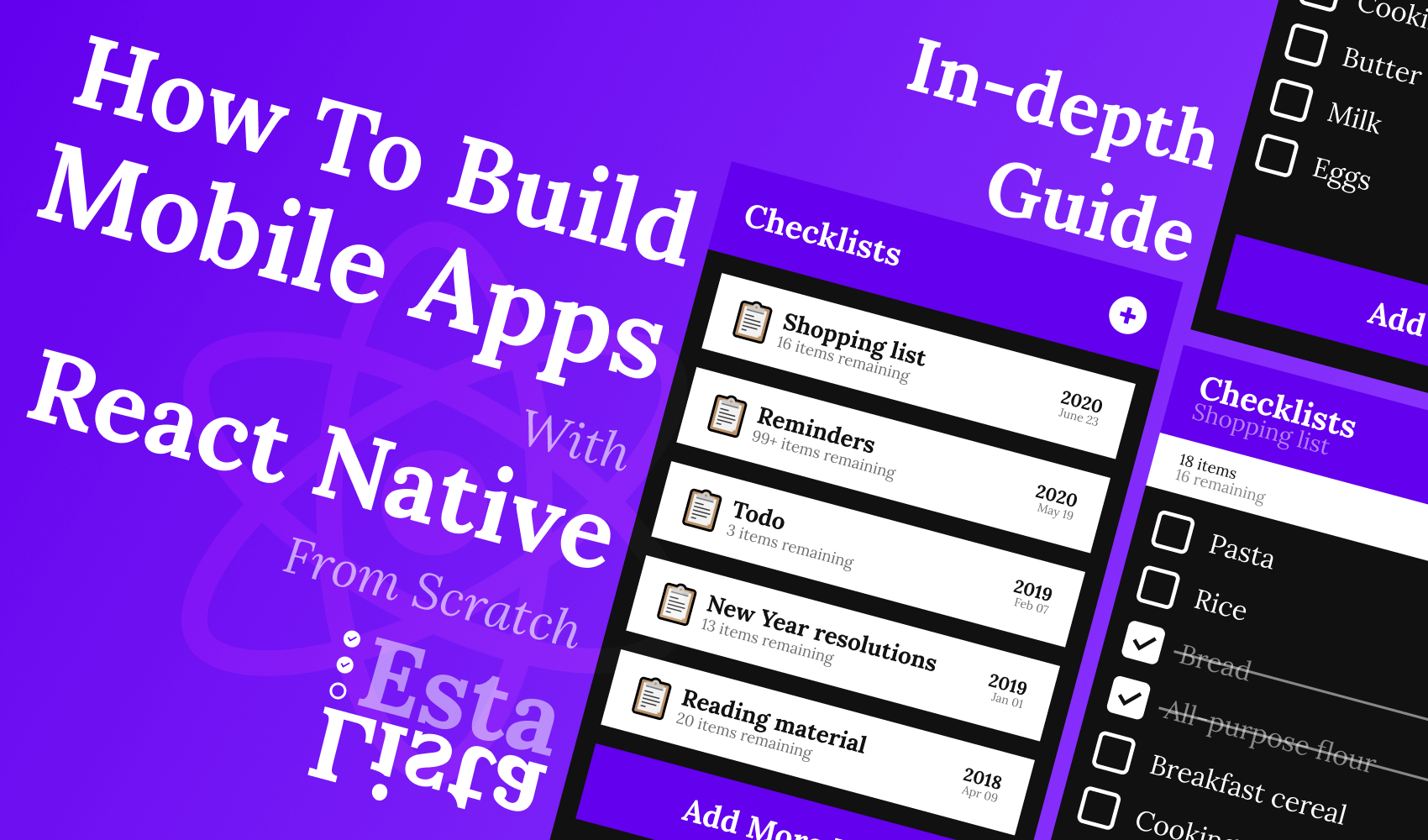
β¨ Features:
- Be able to add new checklists on the app
- Be able to remove existing checklists from the app
- Be able to check items from the checklist
- Be able to delete items from the checklist
- Advanced:
- Be able to see when the checklist was created
- Be able to see how many remaining items are inside a checklist
- Be able to see the total number of items inside a checklist
π§ You'll learn:
Building mobile applications
Routing
Working with states
Storing data on the device
π Resources:
#68 β Create a CMS
Looking for something complex, but for the web? Then you might want to take a look into building a content management system. You can start with a simple page and a WYSIWYG editor, and build out more features as you go to make it more complex and useful.
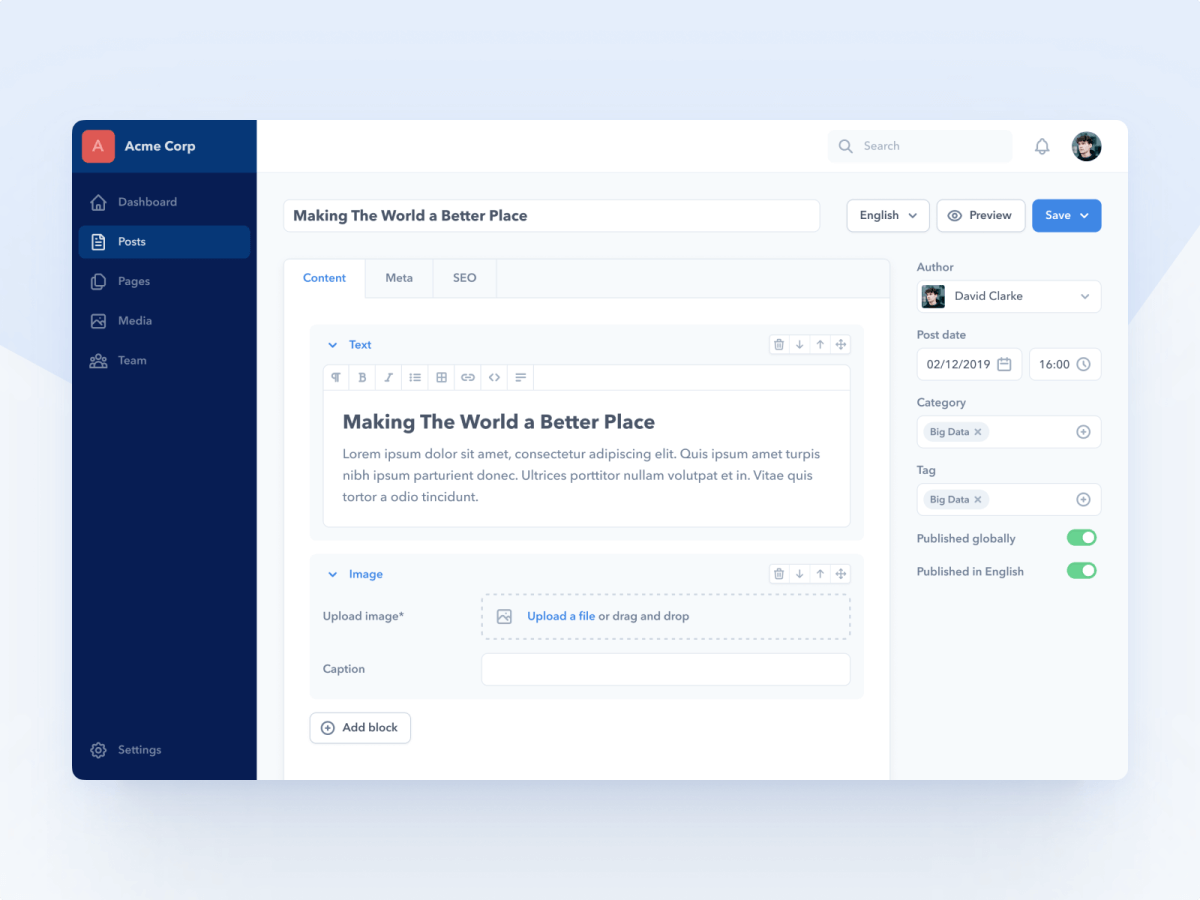
β¨ Features:
- Be able to create new posts with a WYSIWYG editor
- Be able to edit existing posts
- Be able to delete existing posts
- Be able to request the data through an API
π§ You'll learn:
CRUD operations
Authentication
Routing
Working with data from a database
π Resources:
#69 β Create a Budget Tracker
Building a budget tracker not only helps you become more aware of your spendings, but also helps you learn key concepts on how to implement CRUD operations in JavaScript, and really deepen your knowledge of arithmetic operators. Want to go a step further? You can also pull in a chart library to visualize the data, or even filter it with a date range.
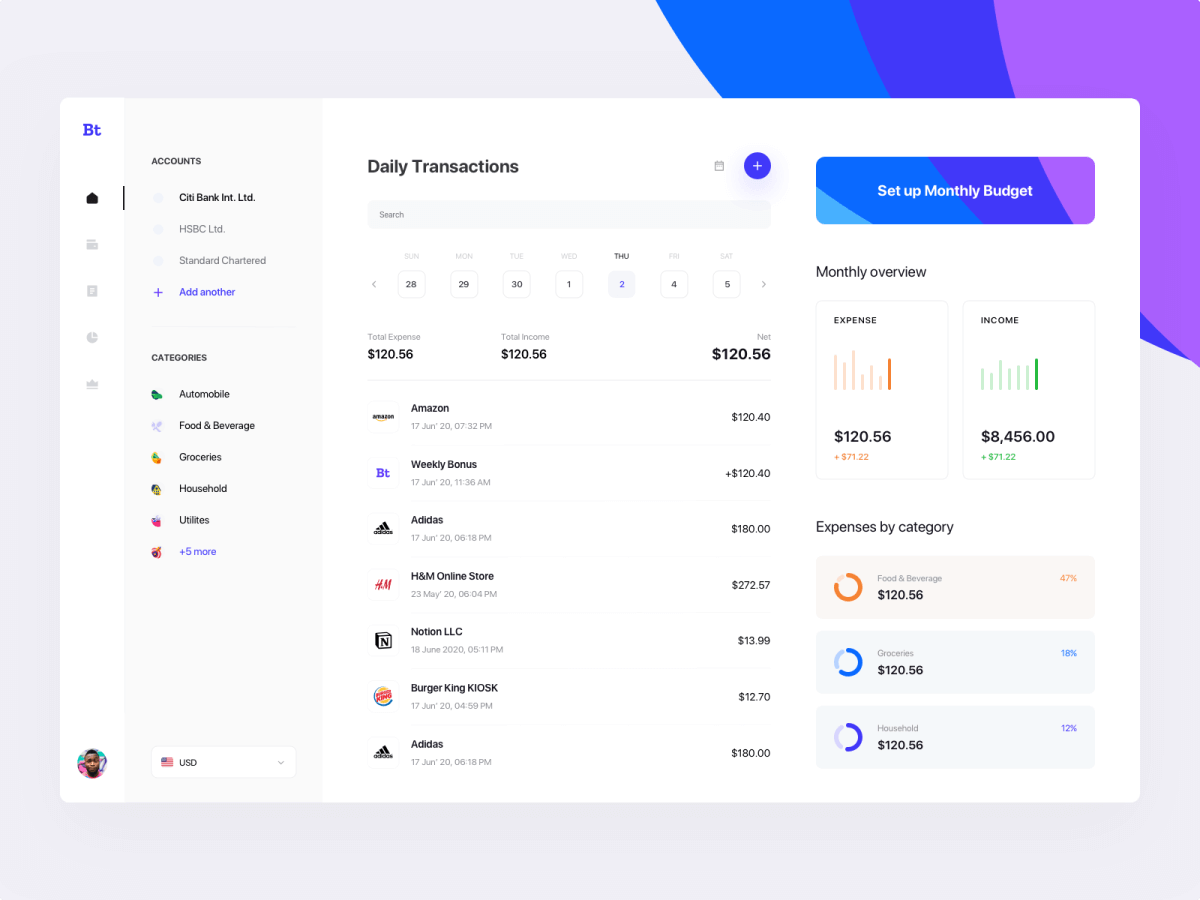
β¨ Features:
- Be able to record expenses and income
- Be able to set the date for an entry
- Be able to tag the entries to categorize them
- Be able to see a monthly overview of expenses and income
- Be able to see net worth
- Advanced:
- Be able to visualize the expenses and incomes through graphs
- Be able to select date ranges, eg.: previous month, or year to date
π§ You'll learn:
CRUD operations
Arithmetic operators
Charts if you want to go advanced
π Resources:
#70 β Create a Pomodoro App
The pomodoro technique helps you break down your tasks into smaller, more manageable chunks, so they become less daunting. Set a timer for 25 minutes, work on the task until the timer rings, then go for a break. You can also do this in cycles and repeat the steps a number of times to extend this to hours.
By building this app, you can learn more about how to handle time in JavaScript, how to work with loops, and how to work with the web audio API.
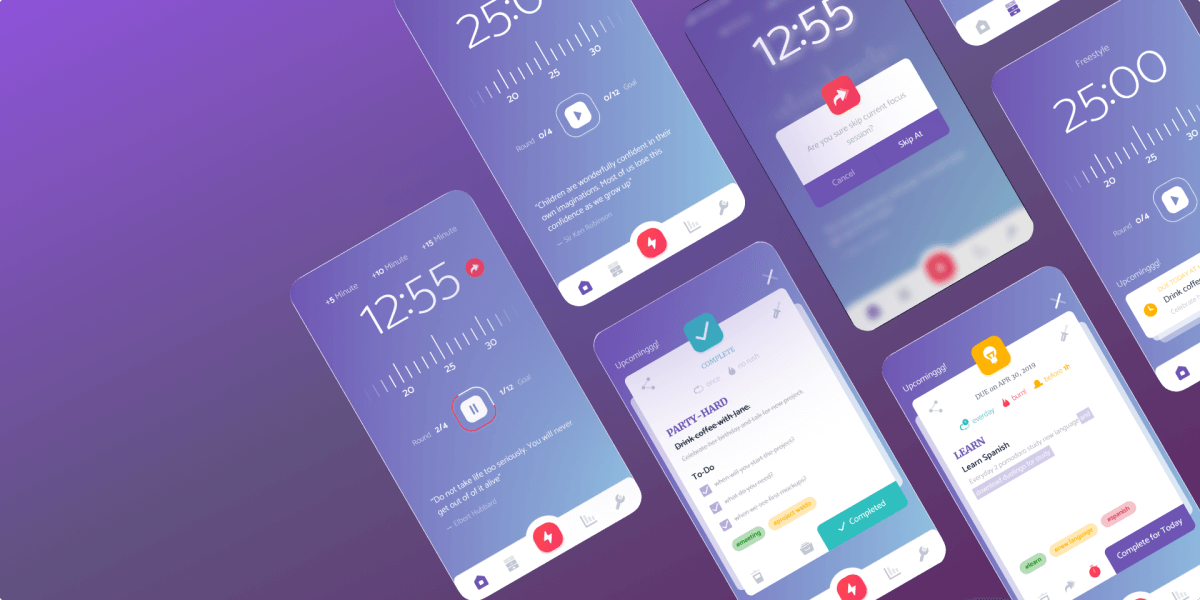
β¨ Features:
- Be able to set a timer in minutes
- Be able to set a break in minutes
- Be able to pause and play the timer
- Be able to reset the timer
- Advanced:
- Be able to set a cycle that repeats the pattern a number of times
π§ You'll learn:
Timers
Loops
Web Audio API
π Resources:

#71 β Create a Whatβs in my Fridge App
It probably happened to you before too that something expired in your fridge. What better way to battle this than building an app to keep track of the food inside?
This project lets you learn about CRUD operations, working with the date API, sorting the items so the food with the soonest expiry date is at the top, and optionally, working with routing if you want to store data in different categories.
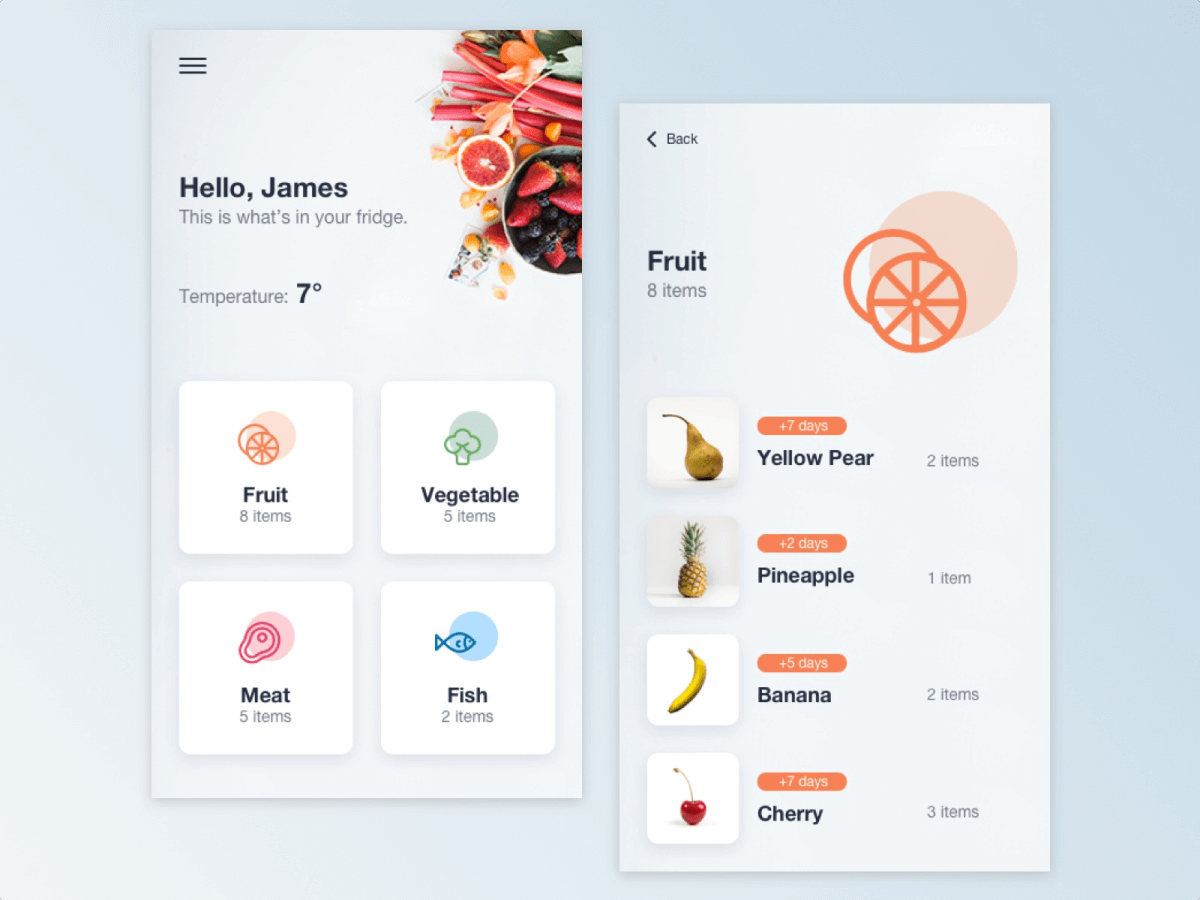
β¨ Features:
- Be able to add items with an expiration date
- Be able to remove existing items
- Be able to see the number of days until expiration
- Advanced:
- Be able to group items into different containers
π§ You'll learn:
CRUD operations
Date API
Sorting and filtering
Optional: routing
π Resources:
#72 β Create a Recipe App
Going one step further, we can look at creating a recipe app for storing our favorite foods in one place. Again, this can help you deepen your knowledge on CRUD operations, as well as persisting data through either local storage, or an actual database. You will also need to fetch data from an endpoint that you can display to the user, which helps you learn more about the fetch API and how promises work.
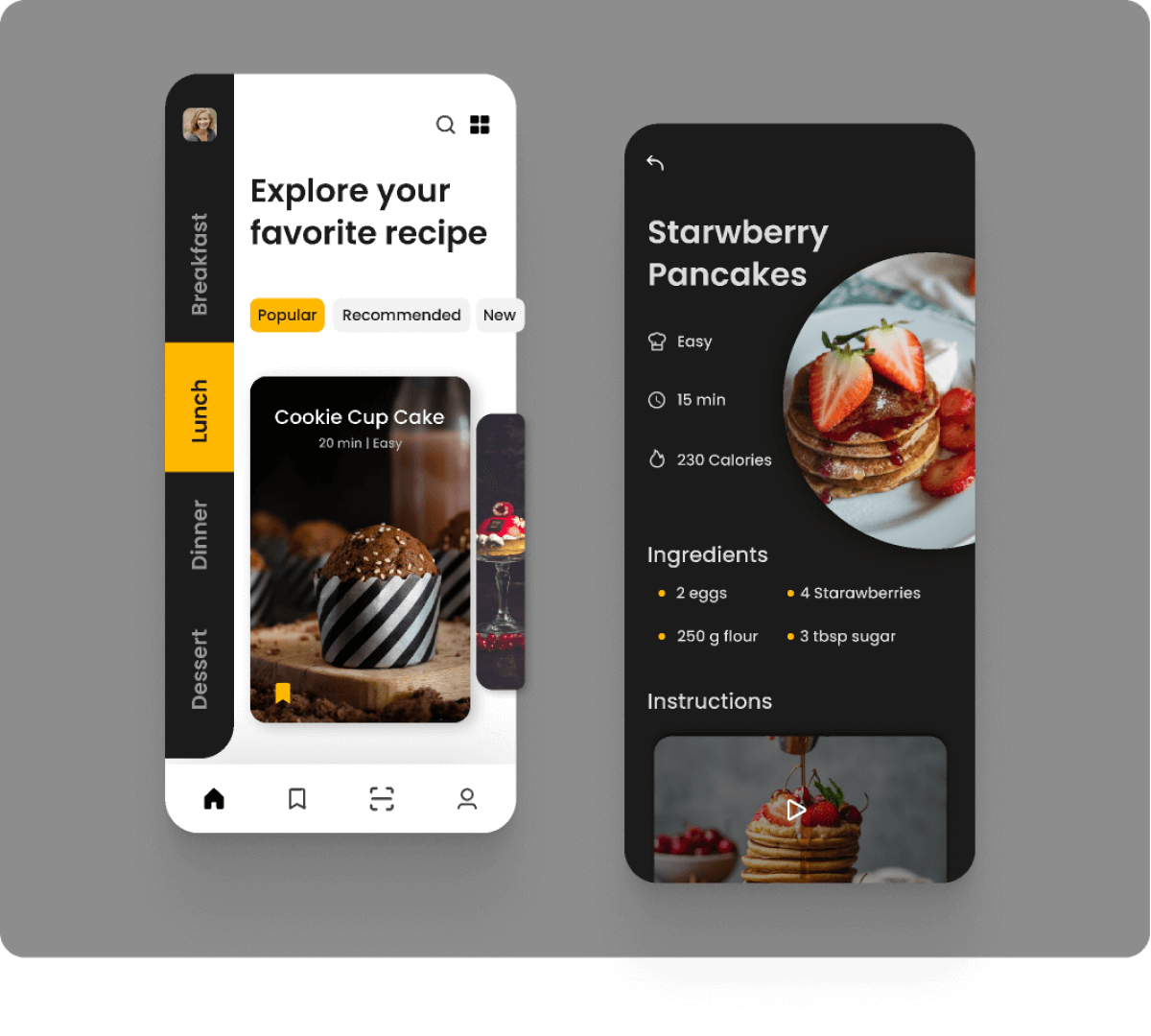
β¨ Features:
- Be able to add new recipes to a list
- Be able to specify a list of ingredients for the recipe
- Be able to specify the difficulty
- Be able to specify a prep time
- Be able to add an image for a recipe
π§ You'll learn:
Navigation between routes (recipes and a single recipe)
Displaying data from JavaScript through HTML
Data storage through the Local Storage API or a database
Fetching data from a remote data source
π Resources:
#73 β Create a Flashcard App
If you also want to learn something else while you are learning JavaScript, then you might want to look into building a flashcard application. You can also enhance this app with some gaming elements, such as a scoring system, or achievement board. With this project, you will learn about fetching data from an API and using that to generate the flashcards, as well as handling card states and click events.
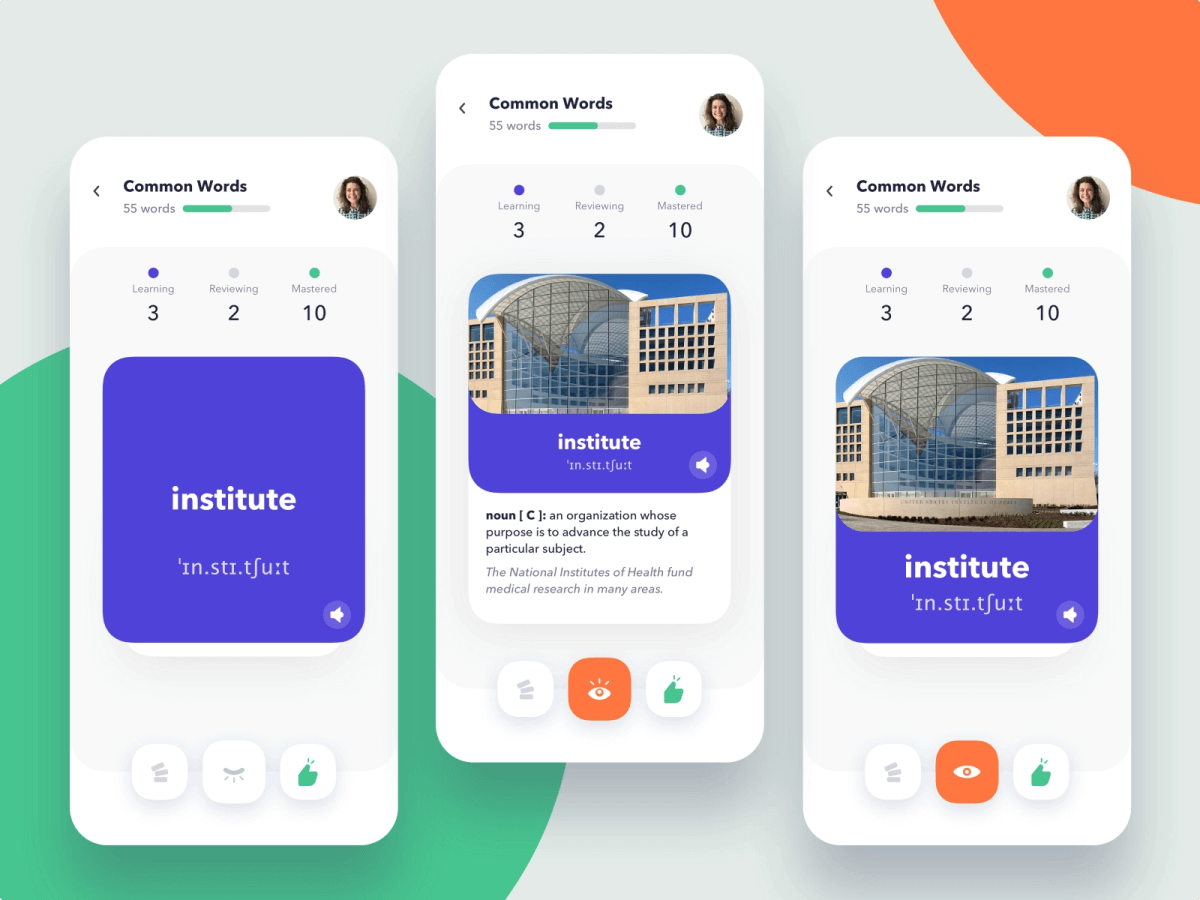
β¨ Features:
- Be able to scroll through a list of flashcards
- Be able to click on a flashcard and see the answer
- Advanced:
- Be able to select an answer from a list of predefined answers
- Be able to see scores based on the correct and incorrect answers
π§ You'll learn:
Fetching data from an API
Using the data to generate flashcards
Building components
Handling card states and click events
Implementing a scoring system
Storing scores
π Resources:
#74 β Create a Meditation App
The earliest records of meditation are found in the ancient Hindu writings called Vedas that are thought to be composed somewhere between 1500-1200 BE. It is an ancient practice that is known to have many positive effects on health, such as reduced stress levels, anxiety, depression, and pain.
So why not try to look into building a meditation app that can help us practice it? With this project, you can learn more about how to work with timers or how to play sounds using the web audio API.
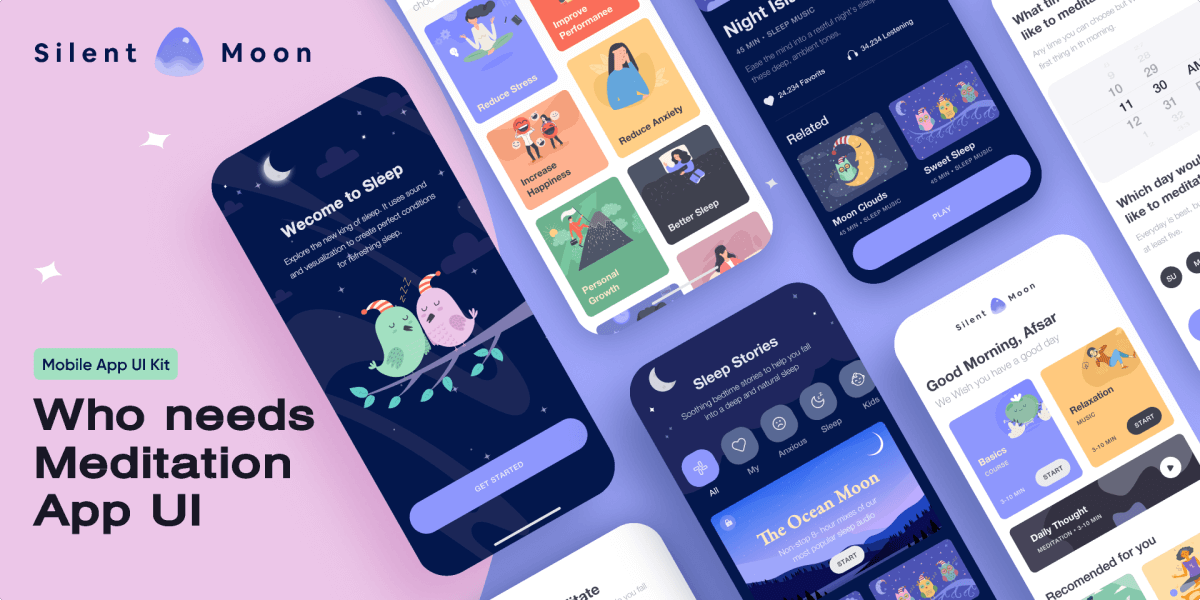
β¨ Features:
- Be able to set a timer for meditation
- Be able to play a set of meditation songs from a playlist
- Be able to save settings, such as the timer
- Advanced:
- Be able to create custom playlists
- Be able to modify existing playlists
π§ You'll learn:
Timers
Web Audio API
Local Storage API for persisting data
π Resources:

#75 β Create a Book Note-Taking App
Do you have a collection of books that you would like to take notes on? You can take on this project to build a book list, or a book note-taking app. You will be able to create, remove and store books for later use. With this project, you will learn about how to work with classes and persist your data throughout page reloads with the help of local storage.
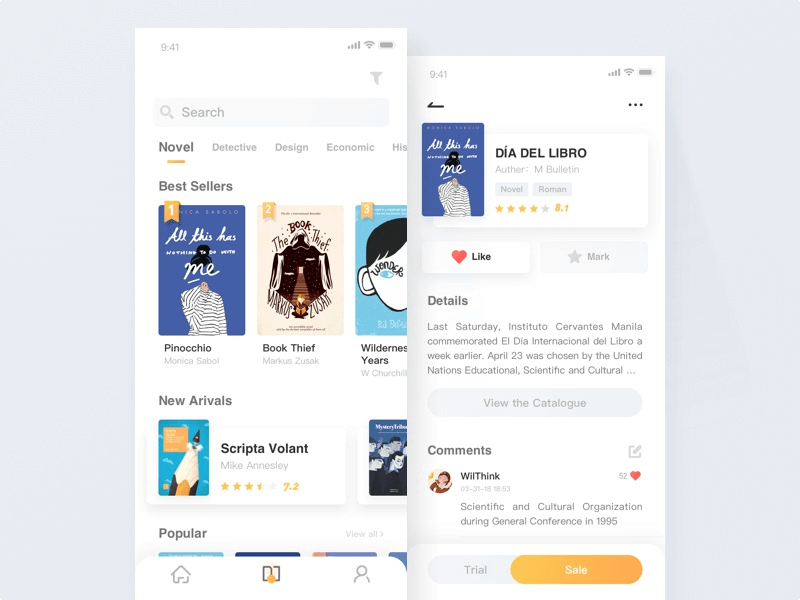
β¨ Features:
- Be able to add books to a list
- Be able to remove books from the list
- Be able to store the books in local storage
π§ You'll learn:
Classes
Event listeners
Local storage API
π Resources:
#76 β Create an Activity Tracker
An activity tracker helps you keep track of your activities in order to make you more aware of your everyday habits. Building this project can help you become more familiar with the Date API, and helps you understand how to work with charts and persisting data for later use.
A great tool that is similar to this project is called Webtime Tracker. It can help you discover your browsing habits so you can better understand where you spend the most time.
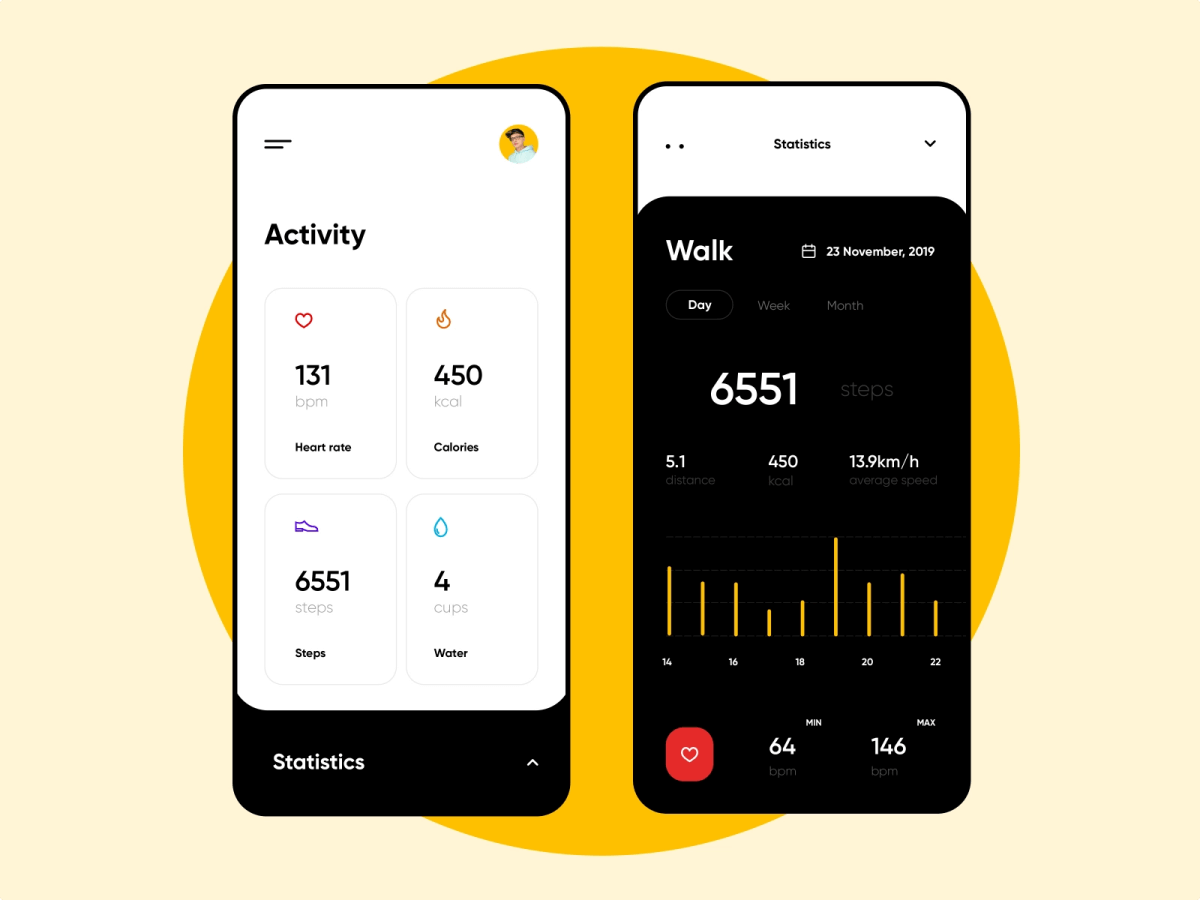
β¨ Features:
- Be able to track activities with a key (name), and value, eg.: Steps: 6551
- Be able to visually see the activity with a chart
- Advanced:
- Be able to switch between days, weeks, and months
π§ You'll learn:
Storing data in the browser or on a server
Date API
Visualizing data with charts
π Resources:
#77 β Create a Random JSON Response Generator
Rather want to create a tool that helps other developers or even your own workflow? Probably you will work a lot with JSON responses. Building a random JSON response generator can not only help you learn JavaScript through a project but can also help your own journey along the way.
In this project, you will mostly learn with string interpolation, and getting JavaScript to execute functions based on certain values. Eg.: {{name}}
should generate a random name, while {{email}}
should generate a random email address.
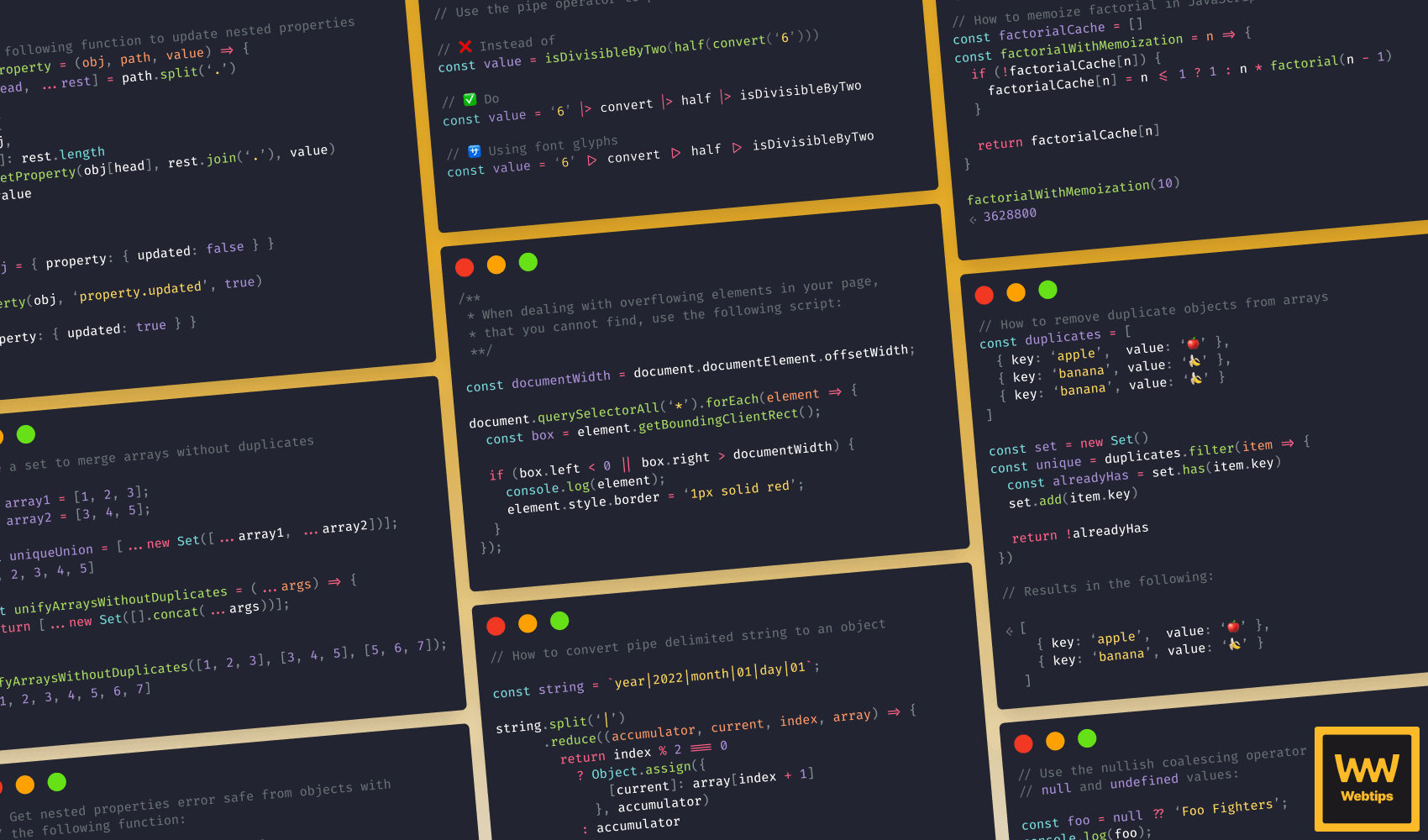
β¨ Features:
- Be able to generate random JSON responses given a schema
- Be able to download the generated response
{
name: "{{name}}",
age: "{{number(18, 40)}}"
email: "{{email}}",
achievements: "[[words(3)]]",
projects: [
{
generateThisObjectNumberOfTimes: 3,
codeName: "{{word}}",
progress: "{{percentage(0, 100)}}"
}
]
}
π§ You'll learn:
Loops
String interpolation
Working with JSON
π Resources:
#78 β Create a Piano
If you want to learn more about the web audio API, building a piano is a great way to start. With the help of this project, you will learn how to play different sounds through click and keyboard events. If you want to take this project one step further, you can also look into building the entire project out on a canvas.
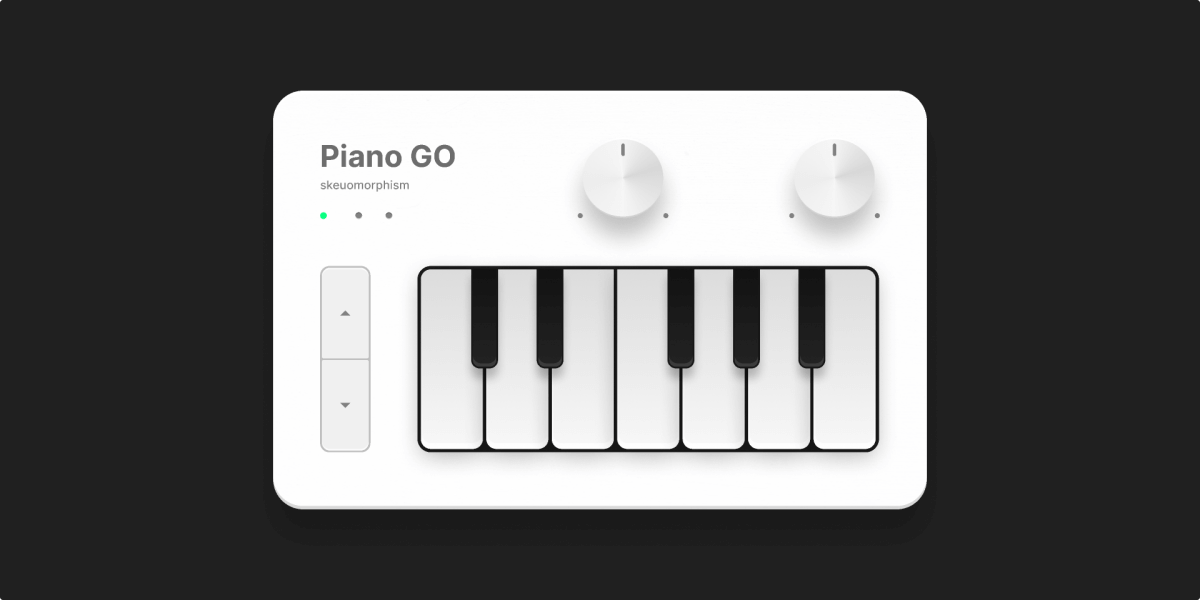
β¨ Features:
- Be able to click on the keyboard that plays back the appropriate sound
- Be able to play the piano with your keyboard
- Be able to adjust the volume of the sound
π§ You'll learn:
Web Audio API
Canvas API
π Resources:

#79 β Create an App for Writers
If you are an avid writer, then you may want to also look into a project that helps to improve your writings. Similar to the famous Hemingway app, you can take a look at how to highlight errors, sentences that are hard to read or long, or things like passive voice or fillers that donβt provide any information.
If you choose this project as your next challenge, you can learn a lot about working with a WYSIWYG editor and not to mention natural language processing.
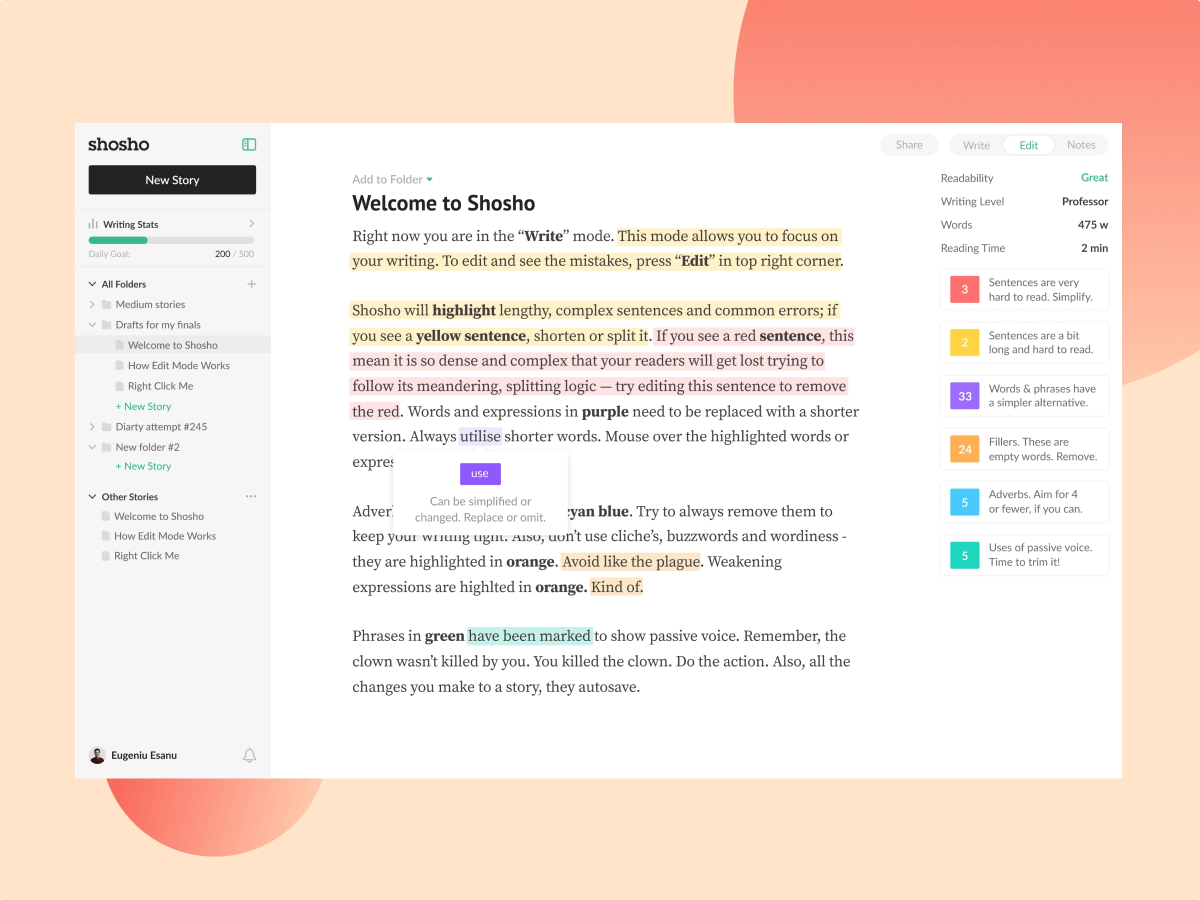
β¨ Features:
- Be able to use a WYSIWYG editor to write text
- Be able to see the word count
- Be able to see the reading time
- Be able to see the readability
- Advanced:
- Be able to see sentences highlighted in red that are very hard to read
- Be able to see sentences highlighted in yellow that are long and hard to read
- Be able to see words highlighted in purple that have simpler alternatives
- Be able to see empty words highlighted in orange
- Be able to see passive voice highlighted in green
π§ You'll learn:
Working with a WYSIWYG editor
Natural language processing
π Resources:
#80 β Create a JSON β CSV Converter
Want to deepen your knowledge of JSON? Try building a JSON to CSV converter that can prove to be useful for other situations as well. In this project, you will need to work with both JSON and CSV files, look at formatting and parsing data, and figuring out how to convert from one to another.
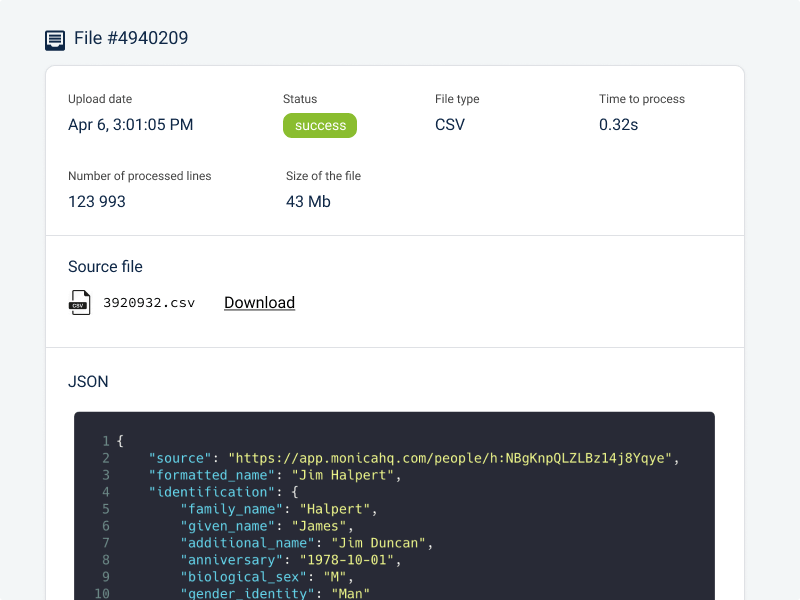
β¨ Features:
- Be able to convert JSON files to CSV
- Be able to format the JSON
- Be able to convert the CSV back to JSON
- Be able to download the generated file
π§ You'll learn:
Working with JSON
Working with CSV
Formatting data
Parsing data
π Resources:
#81 β Create a Markdown β HTML Converter
Weβve already looked at JSON to CSV, but you can also have fun with Markdown and HTML. You will learn how to parse Markdown, and based on the generated tokens, you can generate the HTML file. If you would like some more challenges, you can also look into generating HTML files back to Markdown.
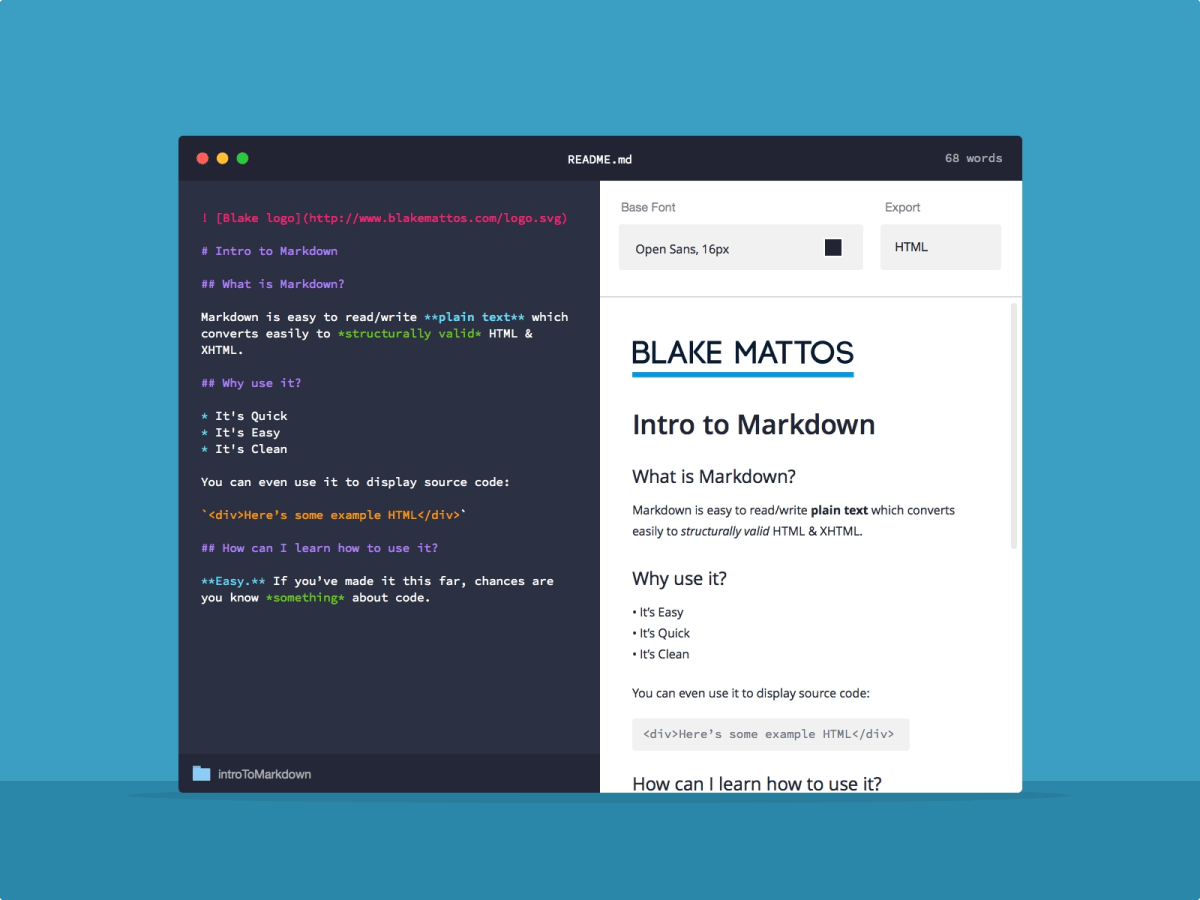
β¨ Features:
- Be able to convert Markdown into HTML
- Be able to preview the generated HTML
- Be able to download the generated HTML
- Advanced:
- Be able to convert HTML back to Markdown
π§ You'll learn:
Working with Markdown
Parsing data
π Resources:
#82 β Create an Image Filter App with CSS Filters
If you would rather work with images, then you might want to take a look into building an image filter app. Here you can learn more about the different CSS filters available, how to work with the Canvas API, and more specifically, how to work with image data.
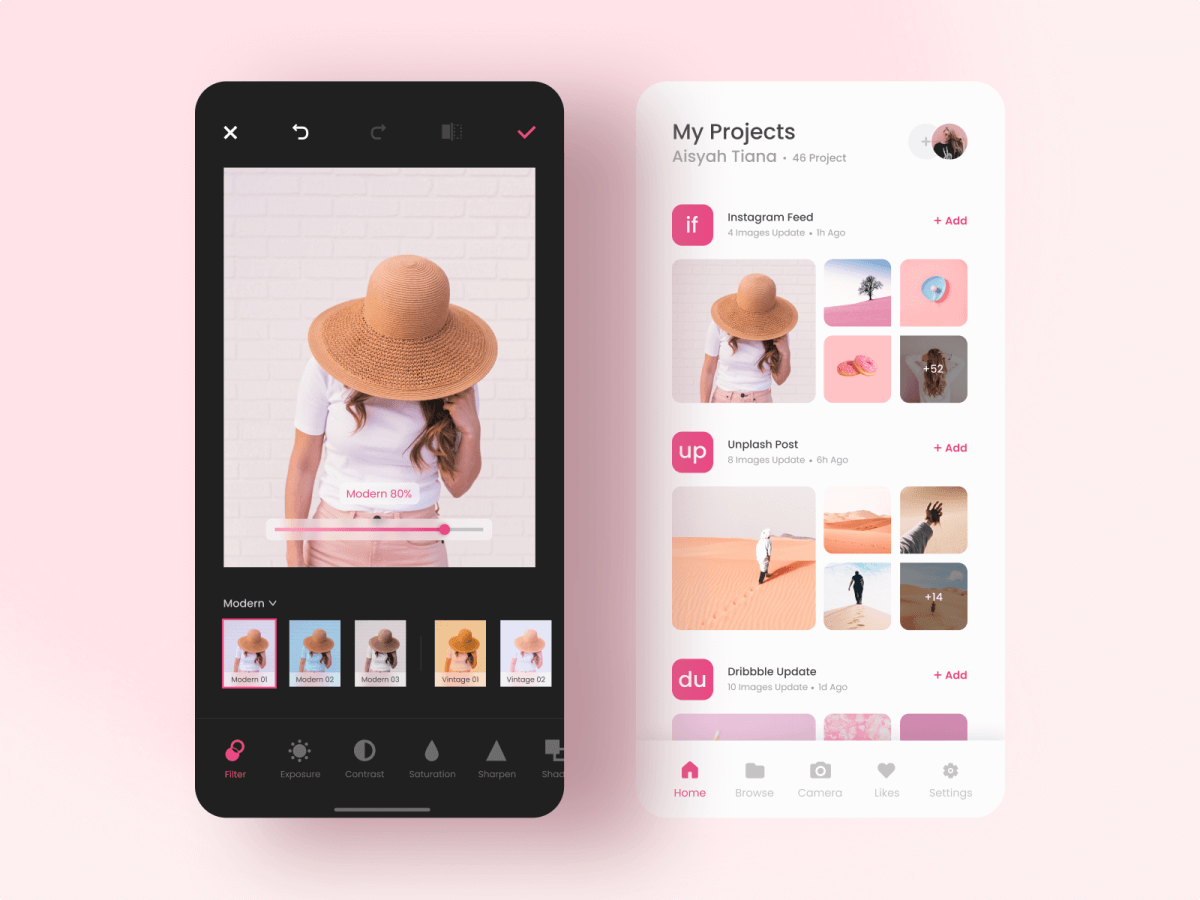
β¨ Features:
- Be able to select an image for filtering
- Be able to select the filter from a list of predefined effects
- Advanced:
- Be able to adjust the strength of the filter
- Be able to download the filtered image
π§ You'll learn:
CSS Filters
Canvas API
Working with image data
π Resources:

#83 β Create a Drawing App
Talking of canvas, another fun project is about building a drawing application. You can really go wild and implement all sorts of functionality to increase the complexity of this project. How about switching to different colors, or setting brush sizes and hardness? Donβt forget to also add the ability to download the masterpieces!
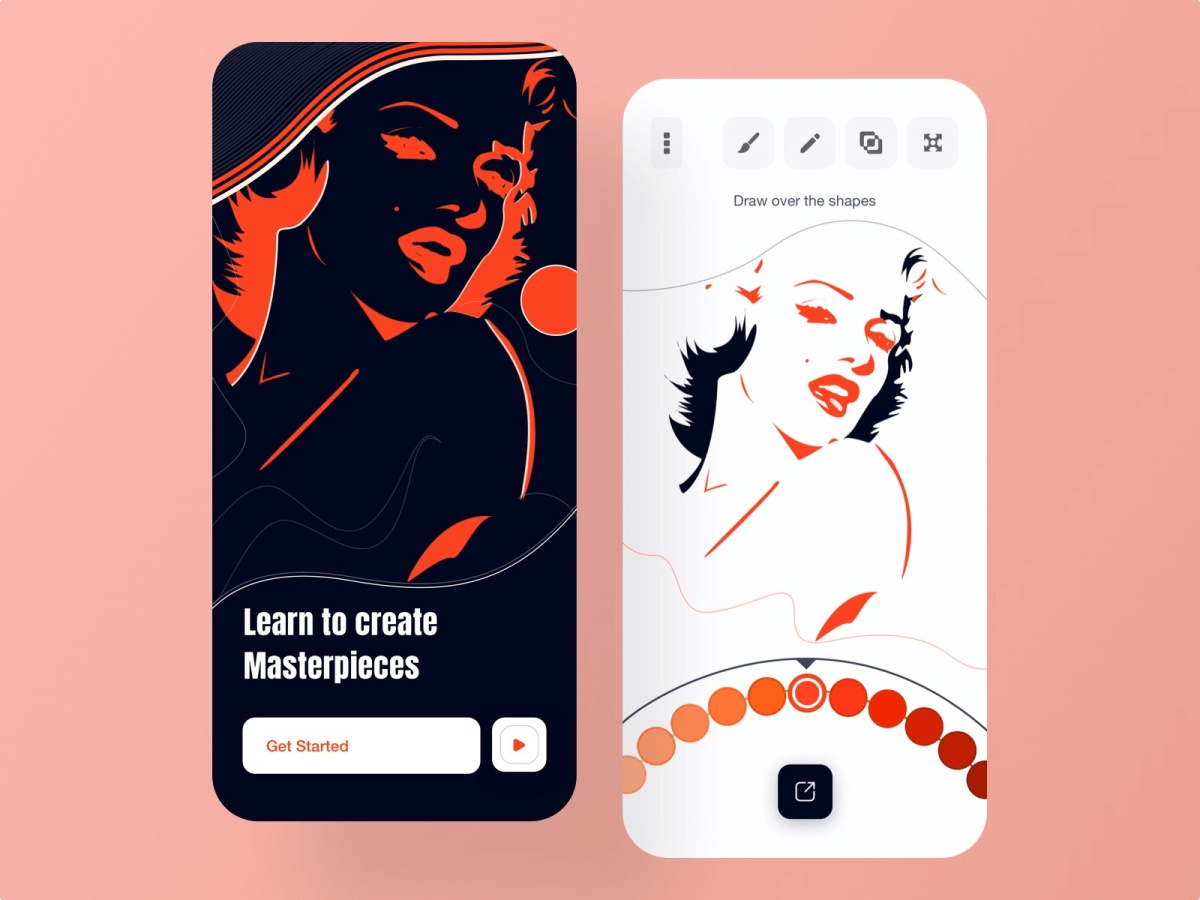
β¨ Features:
- Be able to draw on a canvas using a mouse
- Be able to switch between colors
- Advanced:
- Be able to set the size of the brush
- Be able to set the hardness of the brush (opacity)
- Be able to download the drawing
π§ You'll learn:
Canvas API
Event listeners
π Resources:
#84 β Create a Presentation App
We all used PowerPoint at one point in our life. With this project, we can take a look into how we can achieve the same using Reveal.js that also powers Slides which is built on top of that. With this project, you will learn how you can create slides and transition between them using JavaScript.
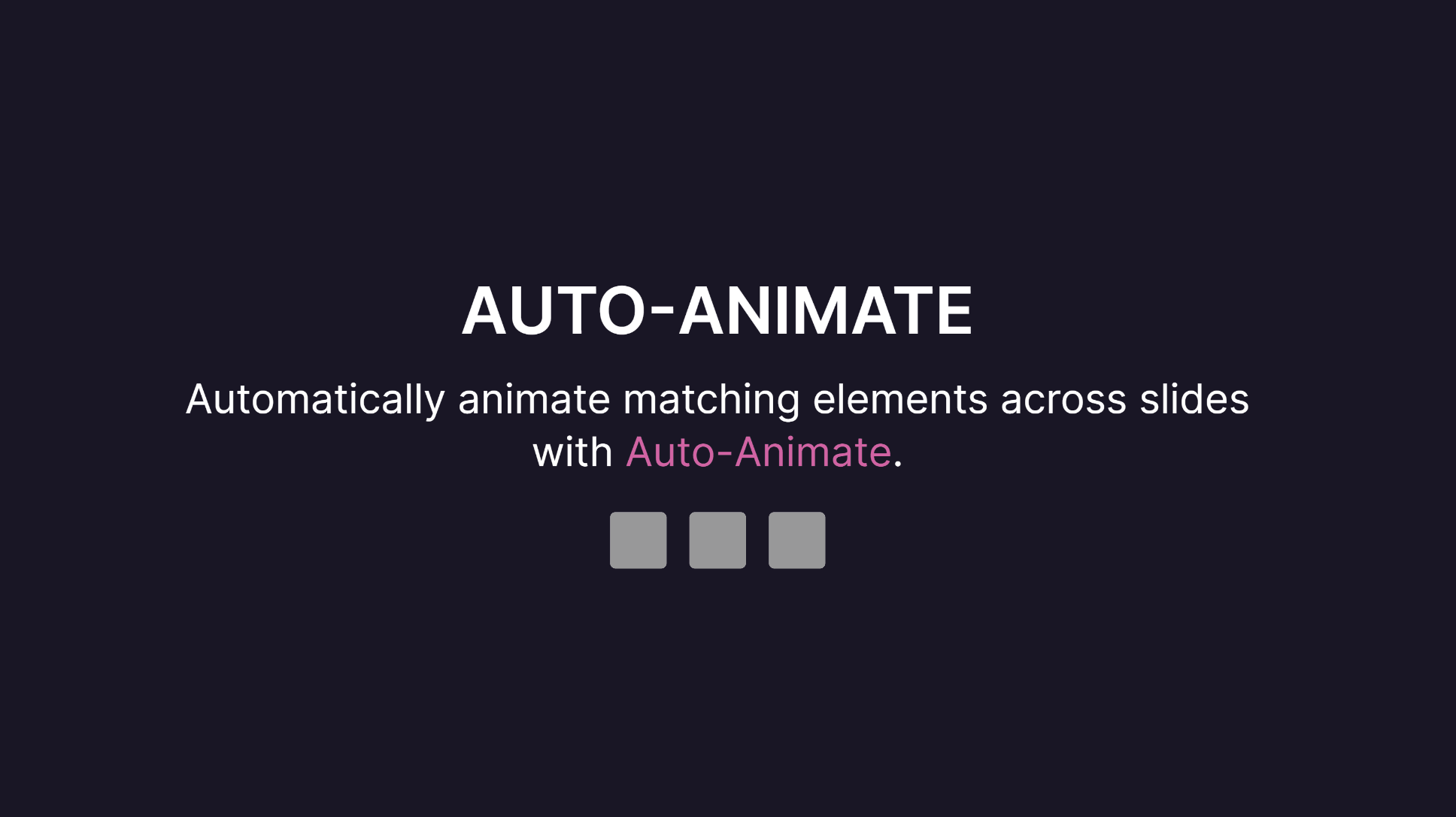
β¨ Features:
- Be able to create new slides using HTML
- Be able to transition between slides using mouse and keyboard navigation
π§ You'll learn:
Animations
Working with Reveal.js
π Resources:
#85 β Create a βBest Places to Goβ App
Want to find out what is the best place to go in your town? You can use Googleβs places API to request the data and display it as you like. In the meantime, you will learn about how to work with an external API, request the data you need, then sort and filter the dataset. The places API also returns a subset of the whole data, so you need to do pagination to get everything.
β¨ Features:
- Be able to fetch places from the Google Places API
- Be able to dynamically set a location using latitude and longitude values
- Be able to dynamically set a radius to use for the location
- Be able to dynamically set the type of location to fetch, eg.: restaurant, bar, etc.
π§ You'll learn:
Google Places API
Fetch API
Pagination
Filtering and sorting
Working with latitude and longitude
π Resources:
#86 β Create a Poll App
If you want to learn a bit about user identification, then you can think about building a poll app where each user can vote only one time. For storing the data, you probably want a server if you would like to make the app usable on every device, but if you are just trying to learn more about JavaScript, then itβs a great start to first implement this app using local storage.
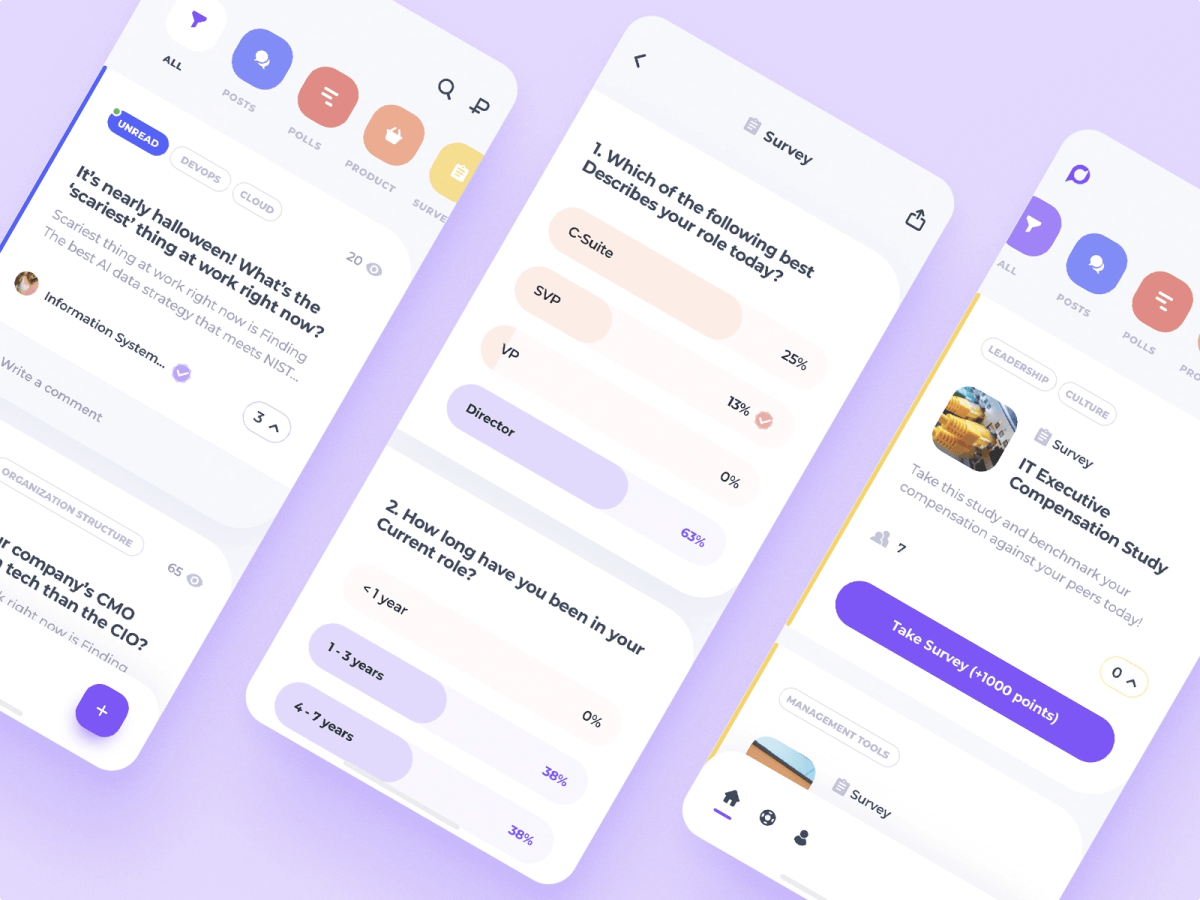
β¨ Features:
- Be able to generate polls from a JavaScript object
- Be able to see the distribution of votes in percentage
- Be able to vote on a single item only
- Be able to store votes and retrieve them, even after reload
- Advanced:
- Be able to prevent users from voting again, based on user identification
π§ You'll learn:
Local Storage API
Click events
User identification
Displaying data from JavaScript
π Resources:

#87 β Create an Image Cropper
If you are looking for more projects that deal with images, then an image cropper might just be the right fit for you. Here you can learn a lot about image manipulation: how to crop images, how to work with aspect ratios, with flips and rotation. Lastly, if you want to keep the result, you can also look into implementing a download functionality for the image.
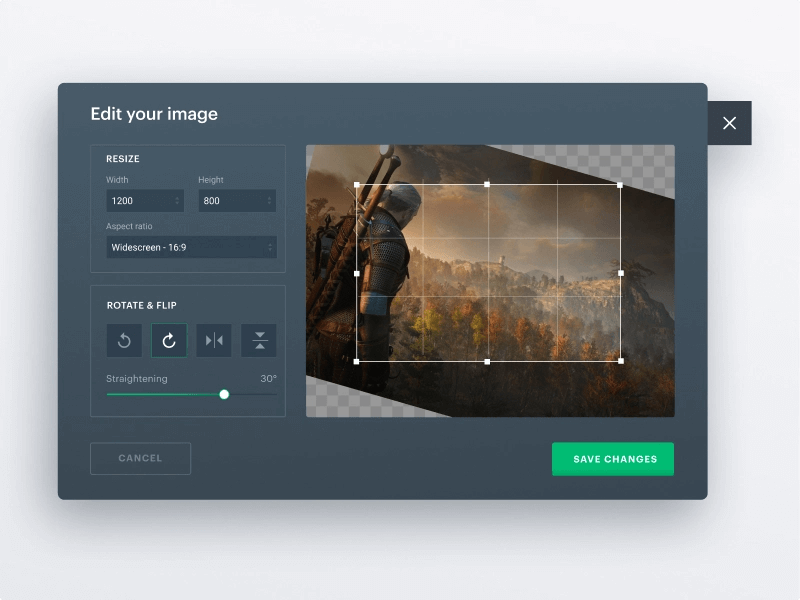
β¨ Features:
- Be able to select an image for cropping
- Be able to select the crop area for the image
- Be able to select an aspect ratio
- Advanced:
- Be able to flip and rotate the image
- Be able to save the changes to a file
π§ You'll learn:
Canvas API
Manipulating image data
π Resources:
#88 β Create a Web Scraper
Web scraping is done when a site doesnβt expose a public API from where we can query data from. It is done by requesting a website and then reading the DOM in order to get the necessary data from there. From this project, you can learn how to work with Cypress to scrape a website for data and collect all the necessary information into an array of objects.
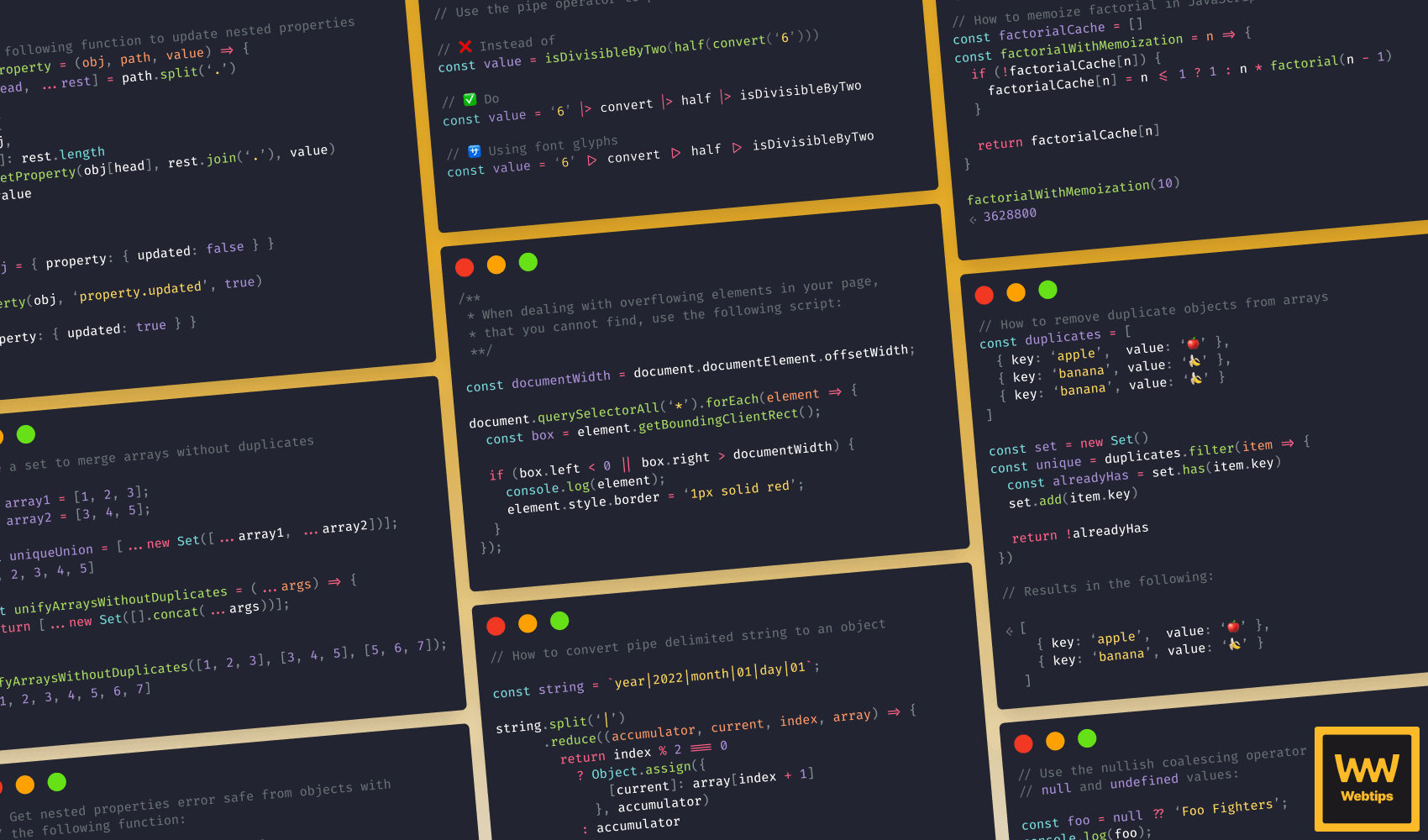
β¨ Features:
- Be able to hit a webpage and scrape data from it
- Be able to collect the data into JavaScript objects
- Advanced:
- Be able to convert the collected data into a JSON object that can be downloaded to a JSON file
π§ You'll learn:
Cypress
Reading and parsing the DOM
π Resources:
#89 β Create a News Aggregator
By creating a news aggregator, you can see all of the most important news in one feed. With this project, you can learn how to work with the fetch API in order to source and combine data from multiple sources, and display them in a feed.
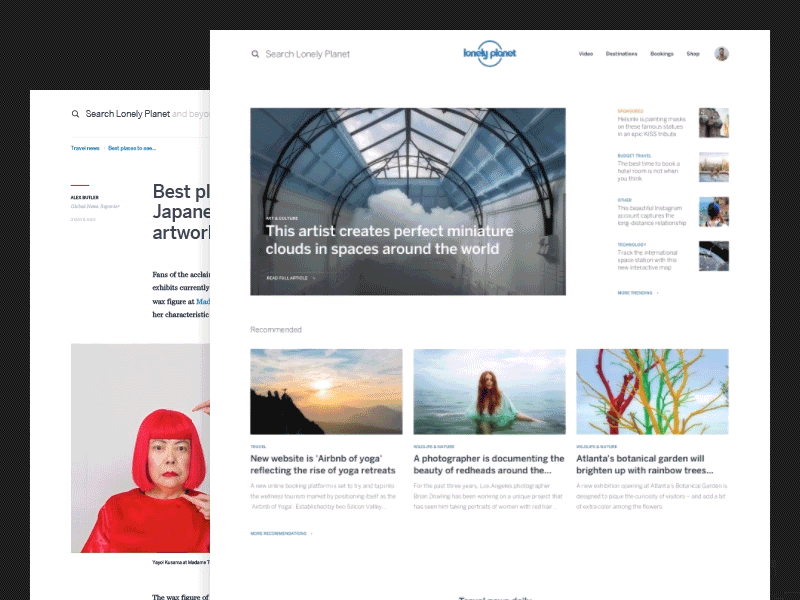
β¨ Features:
- Be able to collect news from multiple sources
- Be able to see the original source of the article
- Be able to see the title and a cover image for the story
π§ You'll learn:
Fetch API
Sourcing data from multiple endpoints
π Resources:
#90 β Create a Password Generator
Having a hard time coming up with new passwords? Try building a password generator that will let you quickly create a secure one. With this project, you will learn more about working with strings and arrays, how to implement sliders, and finally, how to generate a password based on the provided settings.
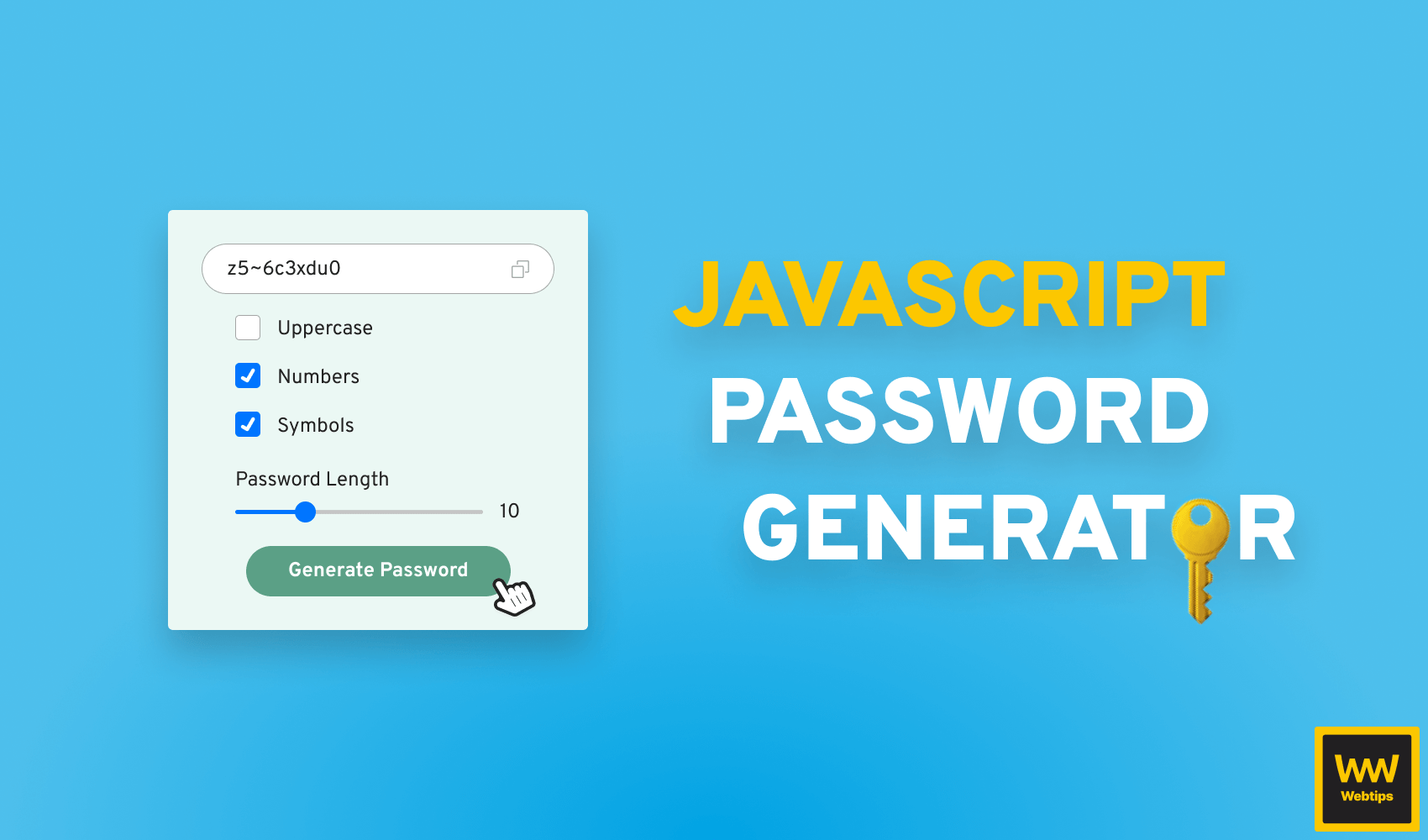
β¨ Features:
- Be able to generate a random password from the letters of the English alphabet
- Be able to use uppercase letters
- Be able to use numbers
- Be able to use symbols
- Be able to copy the generated passwords to the clipboard
- Advanced:
- Be able to set the length of the password
π§ You'll learn:
Strings and arrays
Sliders
Copying text to clipboard
π Resources:

#91 β Create a Resource Network App
Want to learn more about working with graphs and data visualization? You can look into building a resource network app where each document can be connected, and its connections are visualized by a network graph. Documents that are referenced the most are emphasized so you can easily spot cornerstone content at a glance.
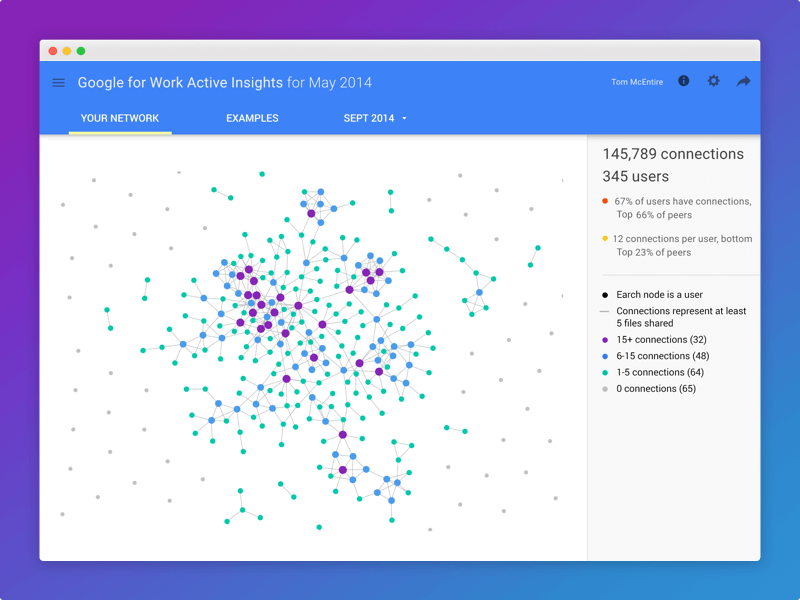
β¨ Features:
- Be able to create new documents
- Be able to edit existing documents
- Be able to remove existing documents
- Be able to link documents to each other
- Be able to visualize documents as a network
- Be able to see connections between documents
π§ You'll learn:
CRUD operations
Working with D3
Working with SVGs
π Resources:
#92 β Create a Journaling App
A great example of this project is Minidiary, which was also written in JavaScript. Unfortunately, the project is not maintained anymore, but we can try to replicate it ourselves and see what we can learn on the way.
Namely, you will be closely working with the Date API and a WYSIWYG editor. For keeping data, you can look at either the local storage API for a simple solution or if you want to take it to another level, implement it in Electron to create a desktop experience. This way, you can also learn more about the file system API.
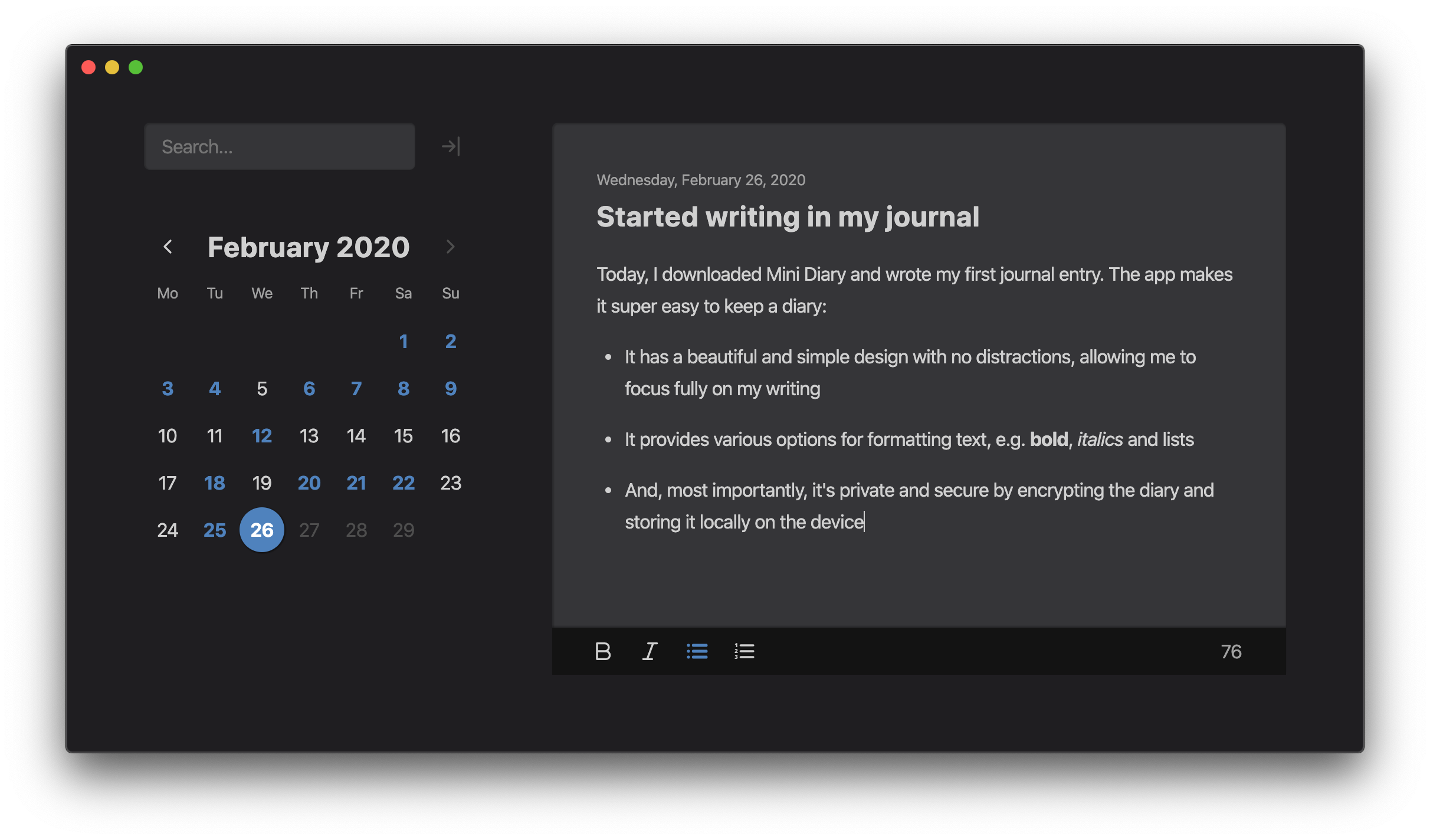
β¨ Features:
- Be able to create new entries
- Be able to format the text inside the entries
- Be able to visually see on the calendar which day contains an entry
- Be able to search for entries
- Advanced:
- Be able to see statistics about total word count, and number of entries
- Be able to store the data in a file
π§ You'll learn:
Date API
Working with a text editor
Local storage / File system API
π Resources:
#93 β Create a Time Tracker App
Want to time block your day to manage your time better? Build a time tracker app where you can start tracking activities, and you can see with an overview of what really takes up most of your time in a day. You can also become more mindful of distractions. You will learn about working with timers in this project, and if you want to go the extra mile, you can look into data visualization as well using charts.
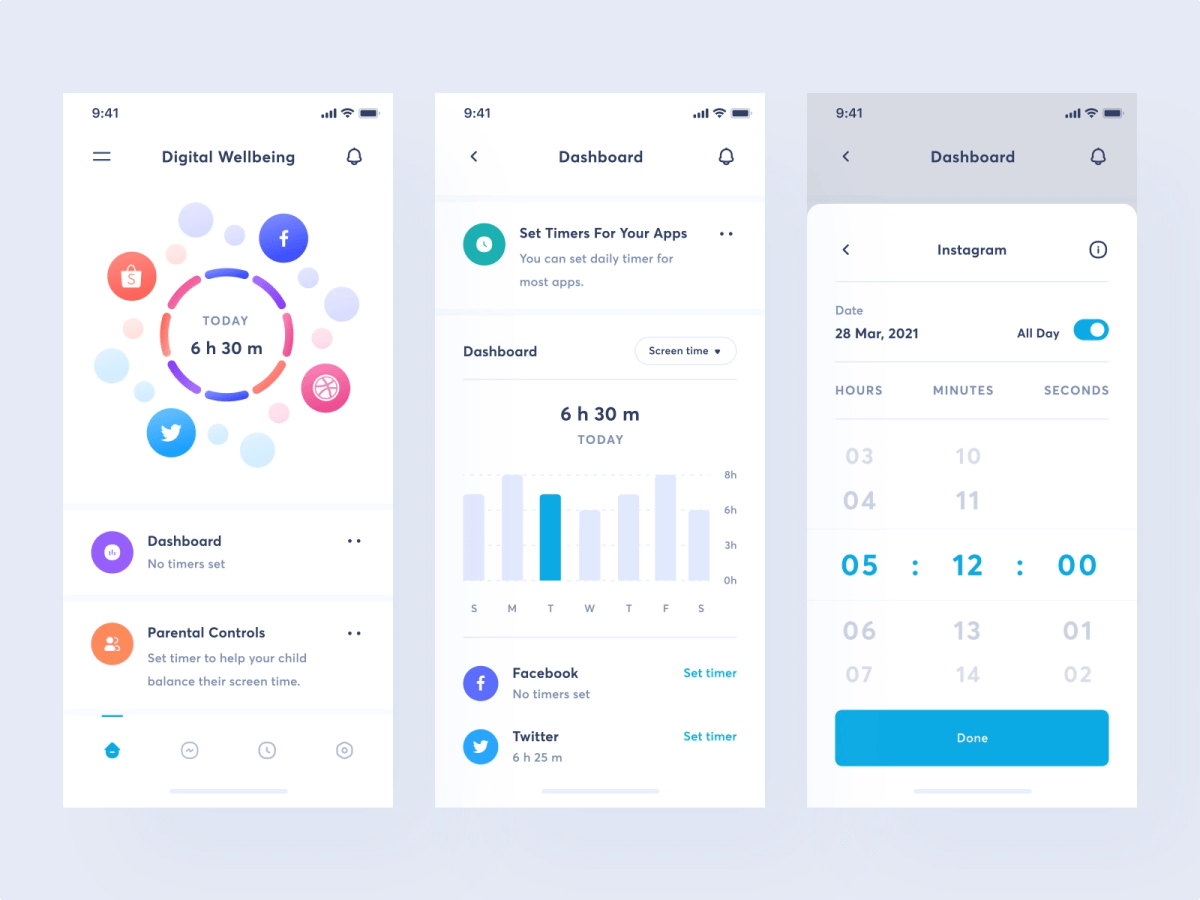
β¨ Features:
- Be able to start a timer
- Be able to stop the timer
- Be able to name the time block
- Be able to see in an ordered list which time block took the most time
π§ You'll learn:
Timers
Sorting arrays
Working with charts
π Resources:
#94 β Create a Mind Map
A mind map is a great way to visualize your ideas and see where you are now, and where do you want to be. With the help of this project, you can learn more about how to work with D3.js to visualize graphs and make them interactive.
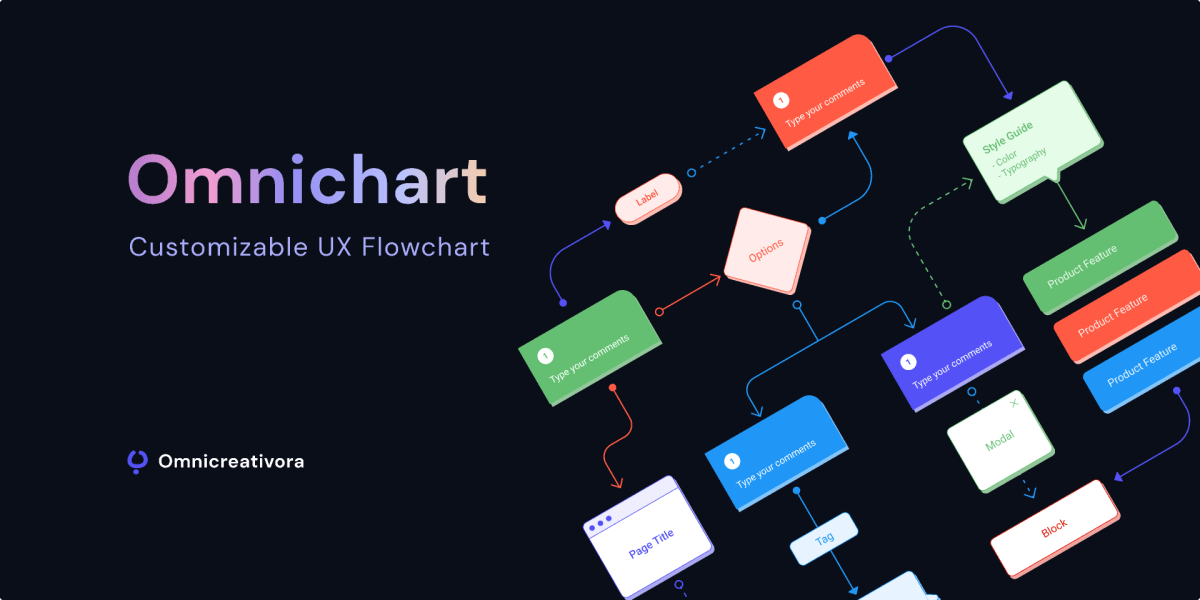
β¨ Features:
- Be able to generate a dendrogram based on an array of objects
- Be able to dynamically add new nodes to the dendrogram
- Be able to remove existing nodes from the dendrogram
π§ You'll learn:
Working with D3.js
Working with SVGs
π Resources:

#95 β Create a Code Challenge App
If you really want to dive into coding, then one of the best ways to do it is to build an app that challenges you to write functioning code. A similar tool to this is called CodeWars where you have to solve code challenges by following a set of instructions. As part of this project, try to build a similar tool where the user can write code that can be executed and verified.
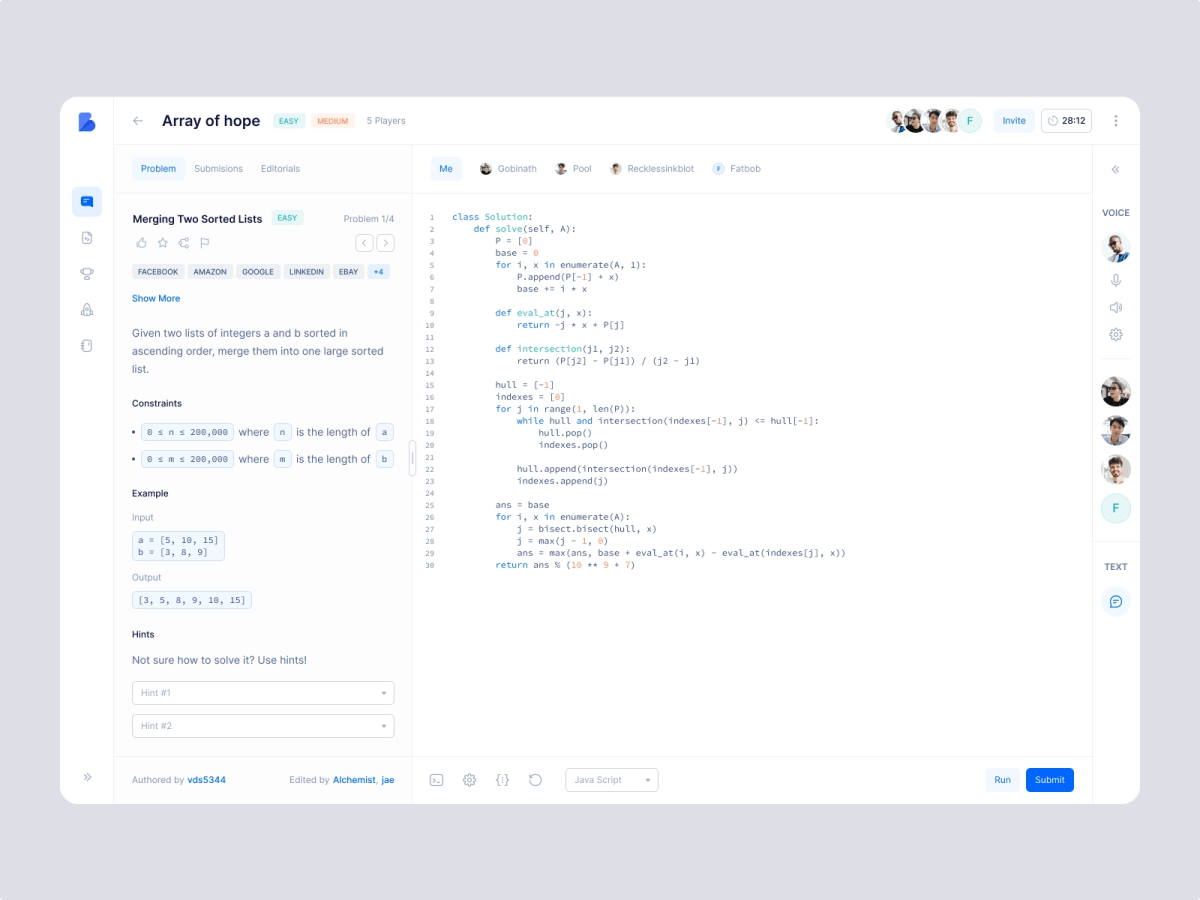
β¨ Features:
- Be able to execute written JavaScript code
- Be able to evaluate the output of the JavaScript code
- If the return value matches the expected value, the solution should get an accepted status
- If the return value doesnβt match the expected value, the solution should get an unaccepted status
π§ You'll learn:
Working with text editors
Executing strings as JavaScript
Evaluating return values
π Resources:
#96 β Create a Family Tree App
If you are the type of developer who is all into binary trees, then you need to scroll through all 95 projects to get to the one where you can finally work with one.
In this project, try to build an interactive family tree where you can create new branches by adding and editing members. You can also look into exporting and importing the data to JSON to make the data portable.
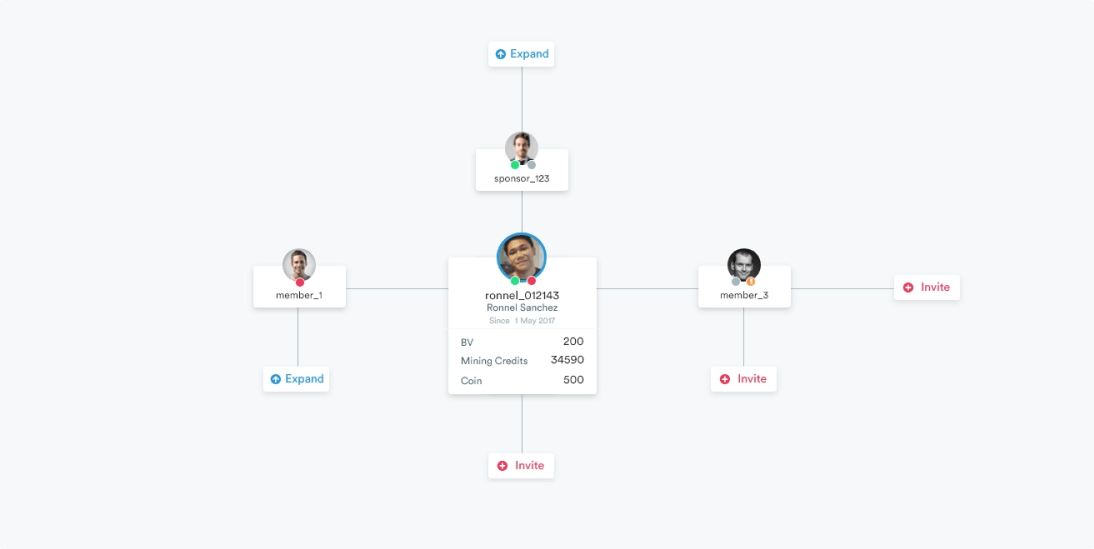
β¨ Features:
- Be able to create a new tree from scratch
- Be able to add members to the tree
- Be able to specify an image, a name, and a DOB for each entry
- Be able to edit existing members of the tree
- Be able to remove branches
- Advanced:
- Be able to export the data as a JSON file
- Be able to import data from a JSON file
π§ You'll learn:
Binary trees
Storing and managing JSON files
π Resources:
#97 β Create a PDF Generator
Want to dive deeper into how HTML works? You can look into building a PDF generator that will require you to parse HTML data in order to dynamically build the PDF files. If you want to make the app more accessible and usable by users, then you can also look into integrating a WYSIWYG editor to make the generated PDF editable, like in the screenshot below:
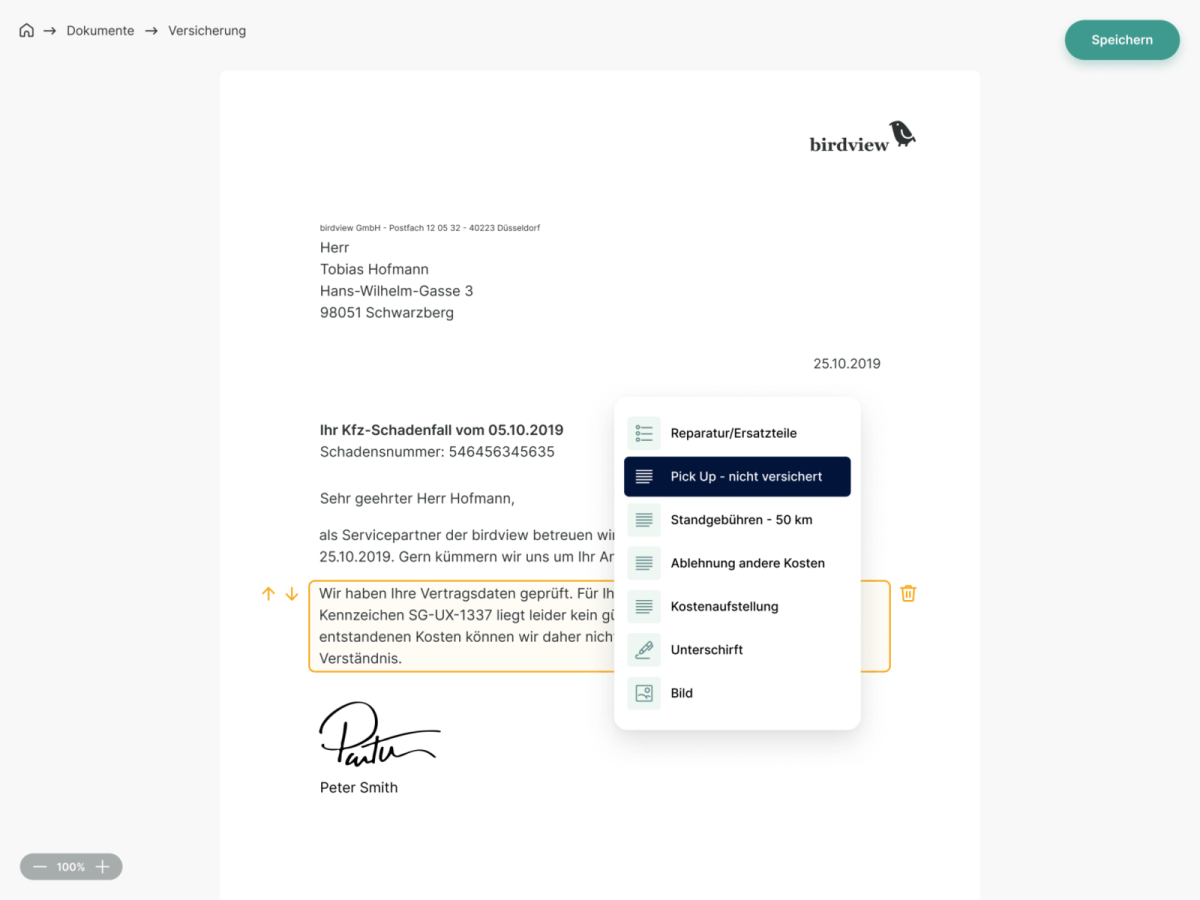
β¨ Features:
- Be able to fill in an A/4 card with content
- Be able to convert the card into a PDF
- Be able to download the generated PDF
π§ You'll learn:
Working with PDF
Working with a text editor
Parsing HTML
π Resources:
#98 β Create a Timetable App
If you have finished the calendar project, or you have already worked with a calendar before, then this project will feel similar to you. It is an extension of a calendar where you can visually see the different tasks you have throughout the day. By taking on this project, you will become deeply familiar with how the date API works, as well as how to work with tables and grids.
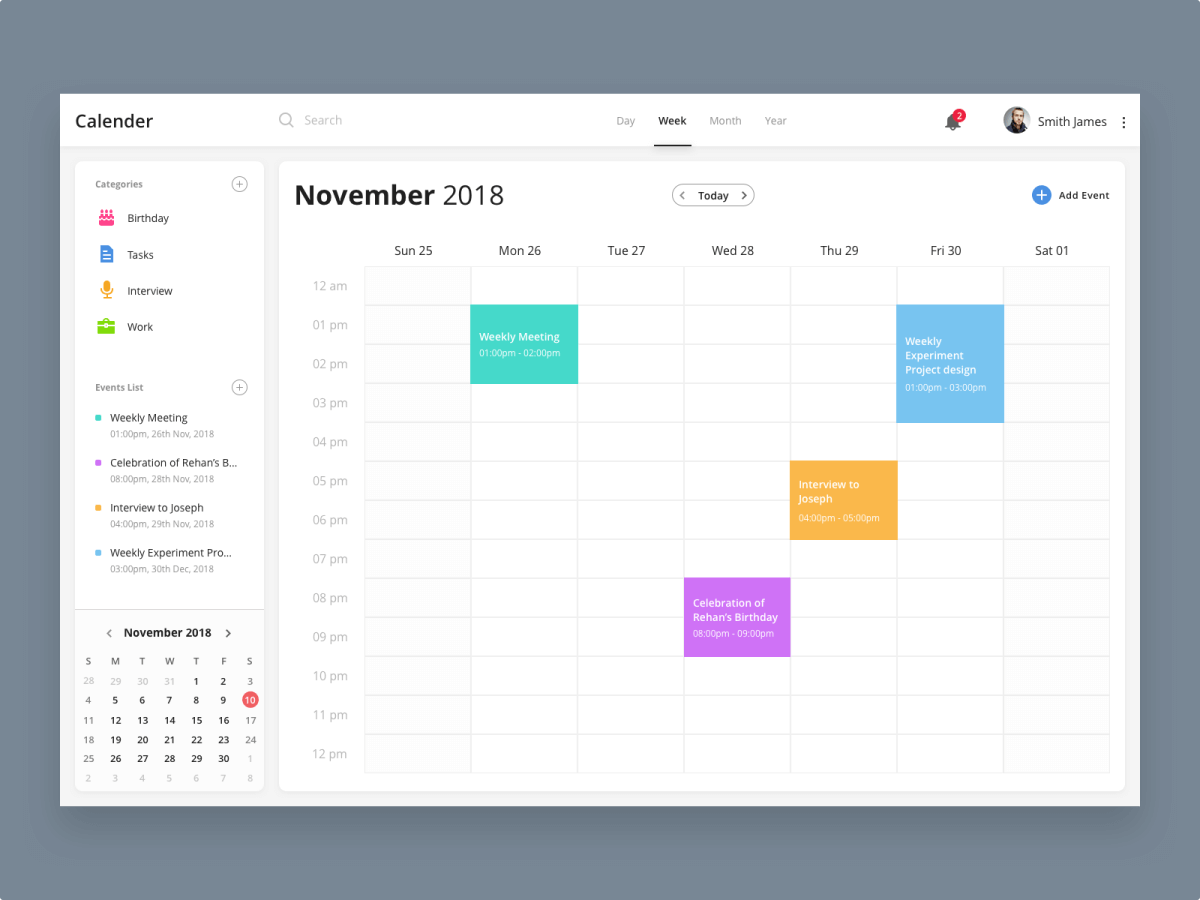
β¨ Features:
- Be able to create entries on a timetable
- Be able to update existing entries inside the calendar
- Be able to assign a color to an entry
- Be able to delete entries
- Be able to navigate the calendar
π§ You'll learn:
Date API
CRUD operations
Working with tables and grids
π Resources:

#99 β Create a Business Card Collector App
Keep losing your business cards? Why not keep them in one place digitally? By building this project, you can learn more about CRUD operations, and how to work with the local storage API to keep all of your data in your browser, even after leaving the page. Want to reach it from elsewhere? You can also look into hosting it in the cloud and uploading the data into a database.
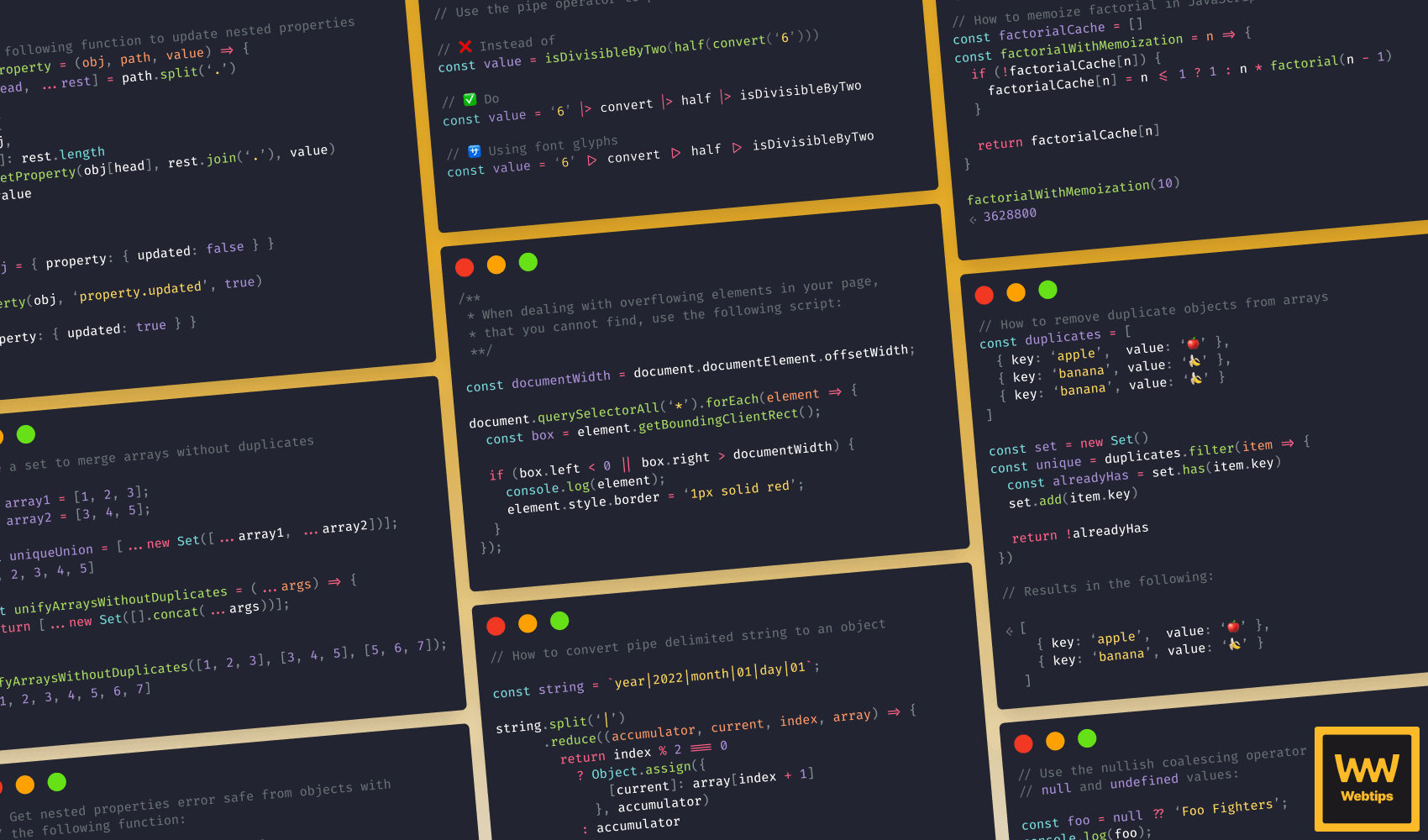
β¨ Features:
- Be able to upload new business cards
- Be able to remove existing business cards
- Be able to edit existing business cards
- Advanced:
- Be able to search for business cards
π§ You'll learn:
CRUD operations
Local storage API
π Resources:
#100 β Create a Frontend Project Challenge Generator
And weβve reached the final project idea, an app that generates project ideas just like this list. Creating the app came with its own challenges too. So what are the takeaways?
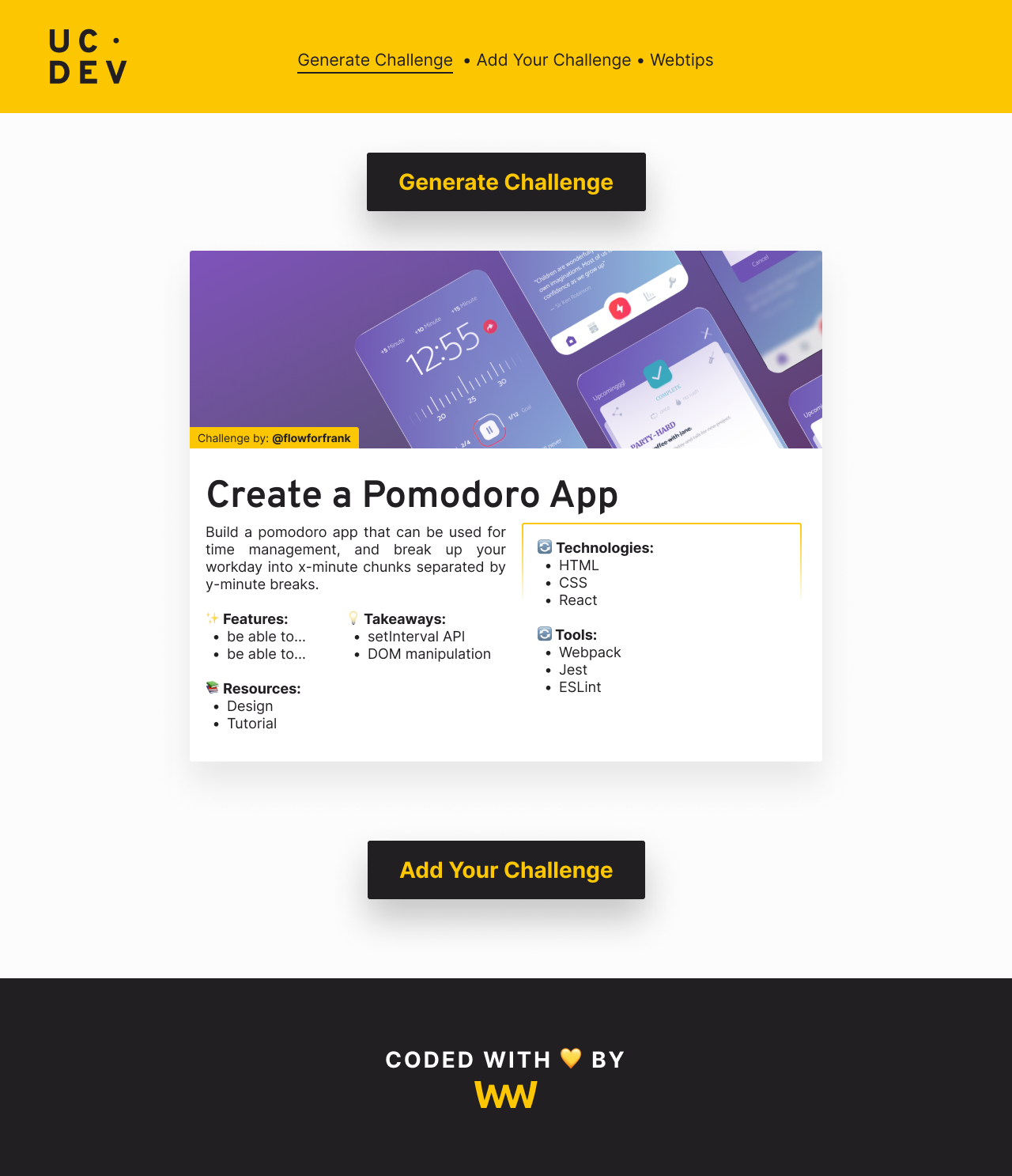
β¨ Features:
- Be able to see the number of total projects
- Be able to randomly choose a project from a list of projects
- Be able to randomly generate technologies and tools to use for the project
- Be able to send in new projects via email
π§ You'll learn:
Routing
Fetching data from a JSON
Working with random numbers
π Resources:
Conclusion
You can take this list one step further and convert these project ideas from JavaScript to your favorite framework or library, whether be it React, Angular, Vue, or Svelte. For extra difficulty, you can throw in some syntactic sugar with TypeScript, or use GraphQL to source your data.
Do you have any project ideas that you think are missing from this page? Let us know in the comments below. Have you already worked on one of these projects? Share them with us in the comments below! Thank you for taking the time to read through, happy coding!
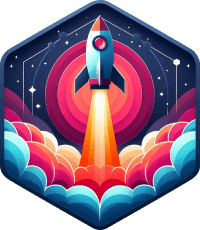
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: